Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Eigen Namespace Reference
Namespace containing all symbols from the Eigen library. More...
Namespaces | |
namespace | internal |
Applies the clock wise 2D rotation j to the set of 2D vectors of cordinates x and y: | |
Data Structures | |
class | LDLT |
Robust Cholesky decomposition of a matrix with pivoting. More... | |
class | LLT |
Standard Cholesky decomposition (LL^T) of a matrix and associated features. More... | |
class | Array |
General-purpose arrays with easy API for coefficient-wise operations. More... | |
class | ArrayBase |
Base class for all 1D and 2D array, and related expressions. More... | |
class | ArrayWrapper |
Expression of a mathematical vector or matrix as an array object. More... | |
class | MatrixWrapper |
Expression of an array as a mathematical vector or matrix. More... | |
class | Block |
Expression of a fixed-size or dynamic-size block. More... | |
class | CommaInitializer |
Helper class used by the comma initializer operator. More... | |
class | CwiseBinaryOp |
Generic expression where a coefficient-wise binary operator is applied to two expressions. More... | |
class | CwiseNullaryOp |
Generic expression of a matrix where all coefficients are defined by a functor. More... | |
class | CwiseUnaryOp |
Generic expression where a coefficient-wise unary operator is applied to an expression. More... | |
class | CwiseUnaryView |
Generic lvalue expression of a coefficient-wise unary operator of a matrix or a vector. More... | |
class | DenseCoeffsBase< Derived, ReadOnlyAccessors > |
Base class providing read-only coefficient access to matrices and arrays. More... | |
class | DenseCoeffsBase< Derived, WriteAccessors > |
Base class providing read/write coefficient access to matrices and arrays. More... | |
class | DenseCoeffsBase< Derived, DirectAccessors > |
Base class providing direct read-only coefficient access to matrices and arrays. More... | |
class | DenseCoeffsBase< Derived, DirectWriteAccessors > |
Base class providing direct read/write coefficient access to matrices and arrays. More... | |
class | Diagonal |
Expression of a diagonal/subdiagonal/superdiagonal in a matrix. More... | |
class | DiagonalMatrix |
Represents a diagonal matrix with its storage. More... | |
class | DiagonalWrapper |
Expression of a diagonal matrix. More... | |
struct | EigenBase |
Common base class for all classes T such that MatrixBase has an operator=(T) and a constructor MatrixBase(T). More... | |
class | Flagged |
Expression with modified flags. More... | |
class | ForceAlignedAccess |
Enforce aligned packet loads and stores regardless of what is requested. More... | |
class | ProductReturnType |
Helper class to get the correct and optimized returned type of operator*. More... | |
class | IOFormat |
Stores a set of parameters controlling the way matrices are printed. More... | |
class | WithFormat |
Pseudo expression providing matrix output with given format. More... | |
class | Map |
A matrix or vector expression mapping an existing array of data. More... | |
class | Matrix |
The matrix class, also used for vectors and row-vectors. More... | |
class | MatrixBase |
Base class for all dense matrices, vectors, and expressions. More... | |
class | NestByValue |
Expression which must be nested by value. More... | |
class | NoAlias |
Pseudo expression providing an operator = assuming no aliasing. More... | |
class | NumTraits |
Holds information about the various numeric (i.e. More... | |
class | PermutationBase |
Base class for permutations. More... | |
class | PermutationMatrix |
Permutation matrix. More... | |
class | PermutationWrapper |
Class to view a vector of integers as a permutation matrix. More... | |
class | PlainObjectBase |
Dense storage base class for matrices and arrays. More... | |
class | Ref |
A matrix or vector expression mapping an existing expressions. More... | |
class | Replicate |
Expression of the multiple replication of a matrix or vector. More... | |
class | Reverse |
Expression of the reverse of a vector or matrix. More... | |
class | Select |
Expression of a coefficient wise version of the C++ ternary operator ?: More... | |
class | SelfAdjointView |
Expression of a selfadjoint matrix from a triangular part of a dense matrix. More... | |
class | Stride |
Holds strides information for Map. More... | |
class | InnerStride |
Convenience specialization of Stride to specify only an inner stride See class Map for some examples. More... | |
class | OuterStride |
Convenience specialization of Stride to specify only an outer stride See class Map for some examples. More... | |
class | Transpose |
Expression of the transpose of a matrix. More... | |
class | Transpositions |
Represents a sequence of transpositions (row/column interchange) More... | |
class | TriangularView |
Base class for triangular part in a matrix. More... | |
struct | Dense |
The type used to identify a dense storage. More... | |
struct | MatrixXpr |
The type used to identify a matrix expression. More... | |
struct | ArrayXpr |
The type used to identify an array expression. More... | |
class | aligned_allocator |
STL compatible allocator to use with with 16 byte aligned types. More... | |
class | VectorBlock |
Expression of a fixed-size or dynamic-size sub-vector. More... | |
class | PartialReduxExpr |
Generic expression of a partially reduxed matrix. More... | |
class | VectorwiseOp |
Pseudo expression providing partial reduction operations. More... | |
class | ComplexEigenSolver |
More... | |
class | ComplexSchur |
More... | |
class | EigenSolver |
More... | |
class | GeneralizedEigenSolver |
More... | |
class | GeneralizedSelfAdjointEigenSolver |
More... | |
class | HessenbergDecomposition |
More... | |
class | RealQZ |
More... | |
class | RealSchur |
More... | |
class | SelfAdjointEigenSolver |
More... | |
class | Tridiagonalization |
More... | |
class | AlignedBox |
More... | |
class | AngleAxis |
More... | |
class | Homogeneous |
More... | |
class | Hyperplane |
More... | |
class | ParametrizedLine |
More... | |
class | QuaternionBase |
More... | |
class | Quaternion |
More... | |
class | Map< const Quaternion< _Scalar >, _Options > |
Quaternion expression mapping a constant memory buffer. More... | |
class | Map< Quaternion< _Scalar >, _Options > |
Expression of a quaternion from a memory buffer. More... | |
class | Rotation2D |
More... | |
class | RotationBase |
Common base class for compact rotation representations. More... | |
class | Transform |
More... | |
class | Translation |
More... | |
class | HouseholderSequence |
More... | |
class | JacobiRotation |
More... | |
class | FullPivLU |
LU decomposition of a matrix with complete pivoting, and related features. More... | |
class | PartialPivLU |
LU decomposition of a matrix with partial pivoting, and related features. More... | |
class | ColPivHouseholderQR |
Householder rank-revealing QR decomposition of a matrix with column-pivoting. More... | |
class | FullPivHouseholderQR |
Householder rank-revealing QR decomposition of a matrix with full pivoting. More... | |
class | HouseholderQR |
Householder QR decomposition of a matrix. More... | |
class | JacobiSVD |
Two-sided Jacobi SVD decomposition of a rectangular matrix. More... | |
Typedefs | |
typedef AngleAxis< float > | AngleAxisf |
single precision angle-axis type | |
typedef AngleAxis< double > | AngleAxisd |
double precision angle-axis type | |
typedef Quaternion< float > | Quaternionf |
single precision quaternion type | |
typedef Quaternion< double > | Quaterniond |
double precision quaternion type | |
typedef Map< Quaternion< float >, 0 > | QuaternionMapf |
Map an unaligned array of single precision scalars as a quaternion. | |
typedef Map< Quaternion < double >, 0 > | QuaternionMapd |
Map an unaligned array of double precision scalars as a quaternion. | |
typedef Map< Quaternion< float > , Aligned > | QuaternionMapAlignedf |
Map a 16-byte aligned array of single precision scalars as a quaternion. | |
typedef Map< Quaternion < double >, Aligned > | QuaternionMapAlignedd |
Map a 16-byte aligned array of double precision scalars as a quaternion. | |
typedef Rotation2D< float > | Rotation2Df |
single precision 2D rotation type | |
typedef Rotation2D< double > | Rotation2Dd |
double precision 2D rotation type | |
typedef DiagonalMatrix< float, 2 > | AlignedScaling2f |
typedef DiagonalMatrix< double, 2 > | AlignedScaling2d |
typedef DiagonalMatrix< float, 3 > | AlignedScaling3f |
typedef DiagonalMatrix< double, 3 > | AlignedScaling3d |
Enumerations | |
enum | { Lower = 0x1, Upper = 0x2, UnitDiag = 0x4, ZeroDiag = 0x8, UnitLower = UnitDiag|Lower, UnitUpper = UnitDiag|Upper, StrictlyLower = ZeroDiag|Lower, StrictlyUpper = ZeroDiag|Upper, SelfAdjoint = 0x10, Symmetric = 0x20 } |
Enum containing possible values for the | |
enum | { Unaligned = 0, Aligned = 1 } |
Enum for indicating whether an object is aligned or not. More... | |
enum | CornerType |
Enum used by DenseBase::corner() in Eigen2 compatibility mode. More... | |
enum | DirectionType { Vertical, Horizontal, BothDirections } |
Enum containing possible values for the | |
enum | { ColMajor = 0, RowMajor = 0x1, AutoAlign = 0, DontAlign = 0x2 } |
Enum containing possible values for the | |
enum | { OnTheLeft = 1, OnTheRight = 2 } |
Enum for specifying whether to apply or solve on the left or right. More... | |
enum | AccessorLevels { ReadOnlyAccessors, WriteAccessors, DirectAccessors, DirectWriteAccessors } |
Used as template parameter in DenseCoeffBase and MapBase to indicate which accessors should be provided. More... | |
enum | DecompositionOptions { , ComputeFullU = 0x04, ComputeThinU = 0x08, ComputeFullV = 0x10, ComputeThinV = 0x20, EigenvaluesOnly = 0x40, ComputeEigenvectors = 0x80 , Ax_lBx = 0x100, ABx_lx = 0x200, BAx_lx = 0x400 } |
Enum with options to give to various decompositions. More... | |
enum | QRPreconditioners { NoQRPreconditioner, HouseholderQRPreconditioner, ColPivHouseholderQRPreconditioner, FullPivHouseholderQRPreconditioner } |
Possible values for the | |
enum | ComputationInfo { Success = 0, NumericalIssue = 1, NoConvergence = 2, InvalidInput = 3 } |
Enum for reporting the status of a computation. More... | |
enum | TransformTraits { Isometry = 0x1, Affine = 0x2, AffineCompact = 0x10 | Affine, Projective = 0x20 } |
Enum used to specify how a particular transformation is stored in a matrix. More... | |
Functions | |
template<typename Derived > | |
const Eigen::CwiseUnaryOp < Eigen::internal::scalar_inverse_mult_op < typename Derived::Scalar > , const Derived > | operator/ (const typename Derived::Scalar &s, const Eigen::ArrayBase< Derived > &a) |
Component-wise division of a scalar by array elements. | |
template<typename Derived , typename PermutationDerived > | |
const internal::permut_matrix_product_retval < PermutationDerived, Derived, OnTheRight > | operator* (const MatrixBase< Derived > &matrix, const PermutationBase< PermutationDerived > &permutation) |
template<typename Derived , typename PermutationDerived > | |
const internal::permut_matrix_product_retval < PermutationDerived, Derived, OnTheLeft > | operator* (const PermutationBase< PermutationDerived > &permutation, const MatrixBase< Derived > &matrix) |
std::ptrdiff_t | l1CacheSize () |
std::ptrdiff_t | l2CacheSize () |
void | setCpuCacheSizes (std::ptrdiff_t l1, std::ptrdiff_t l2) |
Set the cpu L1 and L2 cache sizes (in bytes). | |
void | initParallel () |
Must be call first when calling Eigen from multiple threads. | |
int | nbThreads () |
void | setNbThreads (int v) |
Sets the max number of threads reserved for Eigen. | |
template<typename Derived , typename TranspositionsDerived > | |
const internal::transposition_matrix_product_retval < TranspositionsDerived, Derived, OnTheRight > | operator* (const MatrixBase< Derived > &matrix, const TranspositionsBase< TranspositionsDerived > &transpositions) |
template<typename Derived , typename TranspositionDerived > | |
const internal::transposition_matrix_product_retval < TranspositionDerived, Derived, OnTheLeft > | operator* (const TranspositionsBase< TranspositionDerived > &transpositions, const MatrixBase< Derived > &matrix) |
static UniformScaling< float > | Scaling (float s) |
Constructs a uniform scaling from scale factor s. | |
static UniformScaling< double > | Scaling (double s) |
Constructs a uniform scaling from scale factor s. | |
template<typename RealScalar > | |
static UniformScaling < std::complex< RealScalar > > | Scaling (const std::complex< RealScalar > &s) |
Constructs a uniform scaling from scale factor s. | |
template<typename Scalar > | |
static DiagonalMatrix< Scalar, 2 > | Scaling (const Scalar &sx, const Scalar &sy) |
Constructs a 2D axis aligned scaling. | |
template<typename Scalar > | |
static DiagonalMatrix< Scalar, 3 > | Scaling (const Scalar &sx, const Scalar &sy, const Scalar &sz) |
Constructs a 3D axis aligned scaling. | |
template<typename Derived > | |
static const DiagonalWrapper < const Derived > | Scaling (const MatrixBase< Derived > &coeffs) |
Constructs an axis aligned scaling expression from vector expression coeffs This is an alias for coeffs.asDiagonal() | |
template<typename Derived , typename OtherDerived > | |
internal::umeyama_transform_matrix_type < Derived, OtherDerived > ::type | umeyama (const MatrixBase< Derived > &src, const MatrixBase< OtherDerived > &dst, bool with_scaling=true) |
| |
template<typename OtherDerived , typename VectorsType , typename CoeffsType , int Side> | |
internal::matrix_type_times_scalar_type < typename VectorsType::Scalar, OtherDerived >::Type | operator* (const MatrixBase< OtherDerived > &other, const HouseholderSequence< VectorsType, CoeffsType, Side > &h) |
Computes the product of a matrix with a Householder sequence. | |
template<typename VectorsType , typename CoeffsType > | |
HouseholderSequence < VectorsType, CoeffsType > | householderSequence (const VectorsType &v, const CoeffsType &h) |
\ | |
template<typename VectorsType , typename CoeffsType > | |
HouseholderSequence < VectorsType, CoeffsType, OnTheRight > | rightHouseholderSequence (const VectorsType &v, const CoeffsType &h) |
\ | |
Variables | |
const int | Dynamic = -1 |
This value means that a positive quantity (e.g., a size) is not known at compile-time, and that instead the value is stored in some runtime variable. | |
const int | DynamicIndex = 0xffffff |
This value means that a signed quantity (e.g., a signed index) is not known at compile-time, and that instead its value has to be specified at runtime. | |
const int | Infinity = -1 |
This value means +Infinity; it is currently used only as the p parameter to MatrixBase::lpNorm<int>(). | |
const unsigned int | RowMajorBit = 0x1 |
for a matrix, this means that the storage order is row-major. | |
const unsigned int | EvalBeforeNestingBit = 0x2 |
means the expression should be evaluated by the calling expression | |
const unsigned int | EvalBeforeAssigningBit = 0x4 |
means the expression should be evaluated before any assignment | |
const unsigned int | PacketAccessBit = 0x8 |
Short version: means the expression might be vectorized. | |
const unsigned int | ActualPacketAccessBit = PacketAccessBit |
If vectorization is enabled (EIGEN_VECTORIZE is defined) this constant is set to the value PacketAccessBit. | |
const unsigned int | LinearAccessBit = 0x10 |
Short version: means the expression can be seen as 1D vector. | |
const unsigned int | LvalueBit = 0x20 |
Means the expression has a coeffRef() method, i.e. | |
const unsigned int | DirectAccessBit = 0x40 |
Means that the underlying array of coefficients can be directly accessed as a plain strided array. | |
const unsigned int | AlignedBit = 0x80 |
means the first coefficient packet is guaranteed to be aligned |
Detailed Description
Namespace containing all symbols from the Eigen library.
Function Documentation
void Eigen::initParallel | ( | ) |
Must be call first when calling Eigen from multiple threads.
Definition at line 48 of file Parallelizer.h.
std::ptrdiff_t Eigen::l1CacheSize | ( | ) |
- Returns:
- the currently set level 1 cpu cache size (in bytes) used to estimate the ideal blocking size parameters.
- See also:
- setCpuCacheSize
Definition at line 1313 of file GeneralBlockPanelKernel.h.
std::ptrdiff_t Eigen::l2CacheSize | ( | ) |
- Returns:
- the currently set level 2 cpu cache size (in bytes) used to estimate the ideal blocking size parameters.
- See also:
- setCpuCacheSize
Definition at line 1322 of file GeneralBlockPanelKernel.h.
int Eigen::nbThreads | ( | ) |
- Returns:
- the max number of threads reserved for Eigen
- See also:
- setNbThreads
Definition at line 58 of file Parallelizer.h.
internal::matrix_type_times_scalar_type<typename VectorsType::Scalar,OtherDerived>::Type Eigen::operator* | ( | const MatrixBase< OtherDerived > & | other, |
const HouseholderSequence< VectorsType, CoeffsType, Side > & | h | ||
) |
Computes the product of a matrix with a Householder sequence.
- Parameters:
-
[in] other Matrix being multiplied. [in] h HouseholderSequence being multiplied.
- Returns:
- Expression object representing the product.
This function computes where
is the matrix
other
and is the Householder sequence represented by
h
.
Definition at line 409 of file HouseholderSequence.h.
const internal::transposition_matrix_product_retval<TranspositionDerived, Derived, OnTheLeft> Eigen::operator* | ( | const TranspositionsBase< TranspositionDerived > & | transpositions, |
const MatrixBase< Derived > & | matrix | ||
) |
- Returns:
- the matrix with the transpositions applied to the rows.
Definition at line 344 of file Transpositions.h.
const internal::permut_matrix_product_retval<PermutationDerived, Derived, OnTheRight> Eigen::operator* | ( | const MatrixBase< Derived > & | matrix, |
const PermutationBase< PermutationDerived > & | permutation | ||
) |
- Returns:
- the matrix with the permutation applied to the columns.
Definition at line 539 of file PermutationMatrix.h.
const internal::permut_matrix_product_retval<PermutationDerived, Derived, OnTheLeft> Eigen::operator* | ( | const PermutationBase< PermutationDerived > & | permutation, |
const MatrixBase< Derived > & | matrix | ||
) |
- Returns:
- the matrix with the permutation applied to the rows.
Definition at line 552 of file PermutationMatrix.h.
const internal::transposition_matrix_product_retval<TranspositionsDerived, Derived, OnTheRight> Eigen::operator* | ( | const MatrixBase< Derived > & | matrix, |
const TranspositionsBase< TranspositionsDerived > & | transpositions | ||
) |
- Returns:
- the matrix with the transpositions applied to the columns.
Definition at line 331 of file Transpositions.h.
const Eigen::CwiseUnaryOp<Eigen::internal::scalar_inverse_mult_op<typename Derived::Scalar>, const Derived> Eigen::operator/ | ( | const typename Derived::Scalar & | s, |
const Eigen::ArrayBase< Derived > & | a | ||
) |
Component-wise division of a scalar by array elements.
Definition at line 74 of file GlobalFunctions.h.
static const DiagonalWrapper<const Derived> Eigen::Scaling | ( | const MatrixBase< Derived > & | coeffs ) | [static] |
static DiagonalMatrix<Scalar,2> Eigen::Scaling | ( | const Scalar & | sx, |
const Scalar & | sy | ||
) | [static] |
static DiagonalMatrix<Scalar,3> Eigen::Scaling | ( | const Scalar & | sx, |
const Scalar & | sy, | ||
const Scalar & | sz | ||
) | [static] |
static UniformScaling<std::complex<RealScalar> > Eigen::Scaling | ( | const std::complex< RealScalar > & | s ) | [static] |
static UniformScaling<double> Eigen::Scaling | ( | double | s ) | [static] |
static UniformScaling<float> Eigen::Scaling | ( | float | s ) | [static] |
void Eigen::setCpuCacheSizes | ( | std::ptrdiff_t | l1, |
std::ptrdiff_t | l2 | ||
) |
Set the cpu L1 and L2 cache sizes (in bytes).
These values are use to adjust the size of the blocks for the algorithms working per blocks.
- See also:
- computeProductBlockingSizes
Definition at line 1334 of file GeneralBlockPanelKernel.h.
void Eigen::setNbThreads | ( | int | v ) |
Sets the max number of threads reserved for Eigen.
- See also:
- nbThreads
Definition at line 67 of file Parallelizer.h.
Variable Documentation
const int Dynamic = -1 |
This value means that a positive quantity (e.g., a size) is not known at compile-time, and that instead the value is stored in some runtime variable.
Changing the value of Dynamic breaks the ABI, as Dynamic is often used as a template parameter for Matrix.
Definition at line 21 of file Constants.h.
const int DynamicIndex = 0xffffff |
This value means that a signed quantity (e.g., a signed index) is not known at compile-time, and that instead its value has to be specified at runtime.
Definition at line 26 of file Constants.h.
const int Infinity = -1 |
This value means +Infinity; it is currently used only as the p parameter to MatrixBase::lpNorm<int>().
The value Infinity there means the L-infinity norm.
Definition at line 31 of file Constants.h.
Generated on Tue Jul 12 2022 17:47:02 by
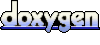