Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Translation< _Scalar, _Dim > Class Template Reference
[Geometry module]
#include <Translation.h>
Public Types | |
typedef _Scalar | Scalar |
the scalar type of the coefficients | |
typedef Matrix< Scalar, Dim, 1 > | VectorType |
corresponding vector type | |
typedef Matrix< Scalar, Dim, Dim > | LinearMatrixType |
corresponding linear transformation matrix type | |
typedef Transform< Scalar, Dim, Affine > | AffineTransformType |
corresponding affine transformation type | |
typedef Transform< Scalar, Dim, Isometry > | IsometryTransformType |
corresponding isometric transformation type | |
Public Member Functions | |
EIGEN_MAKE_ALIGNED_OPERATOR_NEW_IF_VECTORIZABLE_FIXED_SIZE (_Scalar, _Dim) enum | |
dimension of the space | |
Translation () | |
Default constructor without initialization. | |
Translation (const VectorType &vector) | |
Constructs and initialize the translation transformation from a vector of translation coefficients. | |
Scalar | x () const |
Retruns the x-translation by value. | |
Scalar | y () const |
Retruns the y-translation by value. | |
Scalar | z () const |
Retruns the z-translation by value. | |
Scalar & | x () |
Retruns the x-translation as a reference. | |
Scalar & | y () |
Retruns the y-translation as a reference. | |
Scalar & | z () |
Retruns the z-translation as a reference. | |
Translation | operator* (const Translation &other) const |
Concatenates two translation. | |
AffineTransformType | operator* (const UniformScaling< Scalar > &other) const |
Concatenates a translation and a uniform scaling. | |
template<typename OtherDerived > | |
AffineTransformType | operator* (const EigenBase< OtherDerived > &linear) const |
Concatenates a translation and a linear transformation. | |
template<typename Derived > | |
IsometryTransformType | operator* (const RotationBase< Derived, Dim > &r) const |
Concatenates a translation and a rotation. | |
template<int Mode, int Options> | |
Transform< Scalar, Dim, Mode > | operator* (const Transform< Scalar, Dim, Mode, Options > &t) const |
Concatenates a translation and a transformation. | |
VectorType | operator* (const VectorType &other) const |
Applies translation to vector. | |
Translation | inverse () const |
template<typename NewScalarType > | |
internal::cast_return_type < Translation, Translation < NewScalarType, Dim > >::type | cast () const |
template<typename OtherScalarType > | |
Translation (const Translation< OtherScalarType, Dim > &other) | |
Copy constructor with scalar type conversion. | |
bool | isApprox (const Translation &other, const typename NumTraits< Scalar >::Real &prec=NumTraits< Scalar >::dummy_precision()) const |
Friends | |
template<typename OtherDerived > | |
AffineTransformType | operator* (const EigenBase< OtherDerived > &linear, const Translation &t) |
Detailed Description
template<typename _Scalar, int _Dim>
class Eigen::Translation< _Scalar, _Dim >
Represents a translation transformation
- Parameters:
-
_Scalar the scalar type, i.e., the type of the coefficients. _Dim the dimension of the space, can be a compile time value or Dynamic
- Note:
- This class is not aimed to be used to store a translation transformation, but rather to make easier the constructions and updates of Transform objects.
Definition at line 30 of file Translation.h.
Member Typedef Documentation
typedef Transform<Scalar,Dim,Affine> AffineTransformType |
corresponding affine transformation type
Definition at line 43 of file Translation.h.
typedef Transform<Scalar,Dim,Isometry> IsometryTransformType |
corresponding isometric transformation type
Definition at line 45 of file Translation.h.
typedef Matrix<Scalar,Dim,Dim> LinearMatrixType |
corresponding linear transformation matrix type
Definition at line 41 of file Translation.h.
typedef _Scalar Scalar |
the scalar type of the coefficients
Definition at line 35 of file Translation.h.
typedef Matrix<Scalar,Dim,1> VectorType |
corresponding vector type
Definition at line 39 of file Translation.h.
Constructor & Destructor Documentation
Translation | ( | ) |
Default constructor without initialization.
Definition at line 54 of file Translation.h.
Translation | ( | const VectorType & | vector ) | [explicit] |
Constructs and initialize the translation transformation from a vector of translation coefficients.
Definition at line 71 of file Translation.h.
Translation | ( | const Translation< OtherScalarType, Dim > & | other ) | [explicit] |
Copy constructor with scalar type conversion.
Definition at line 158 of file Translation.h.
Member Function Documentation
internal::cast_return_type<Translation,Translation<NewScalarType,Dim> >::type cast | ( | ) | const |
- Returns:
*this
with scalar type casted to NewScalarType
Note that if NewScalarType is equal to the current scalar type of *this
then this function smartly returns a const reference to *this
.
Definition at line 153 of file Translation.h.
EIGEN_MAKE_ALIGNED_OPERATOR_NEW_IF_VECTORIZABLE_FIXED_SIZE | ( | _Scalar | , |
_Dim | |||
) |
dimension of the space
Definition at line 33 of file Translation.h.
Translation inverse | ( | ) | const |
- Returns:
- the inverse translation (opposite)
Definition at line 137 of file Translation.h.
bool isApprox | ( | const Translation< _Scalar, _Dim > & | other, |
const typename NumTraits< Scalar >::Real & | prec = NumTraits<Scalar>::dummy_precision() |
||
) | const |
- Returns:
true
if*this
is approximately equal to other, within the precision determined by prec.
- See also:
- MatrixBase::isApprox()
Definition at line 165 of file Translation.h.
Concatenates a translation and a transformation.
Definition at line 125 of file Translation.h.
VectorType operator* | ( | const VectorType & | other ) | const |
Applies translation to vector.
Definition at line 133 of file Translation.h.
Translation operator* | ( | const Translation< _Scalar, _Dim > & | other ) | const |
Concatenates two translation.
Definition at line 94 of file Translation.h.
IsometryTransformType operator* | ( | const RotationBase< Derived, Dim > & | r ) | const |
Concatenates a translation and a rotation.
Definition at line 106 of file Translation.h.
Translation< Scalar, Dim >::AffineTransformType operator* | ( | const UniformScaling< Scalar > & | other ) | const |
Concatenates a translation and a uniform scaling.
Definition at line 180 of file Translation.h.
Translation< Scalar, Dim >::AffineTransformType operator* | ( | const EigenBase< OtherDerived > & | linear ) | const |
Concatenates a translation and a linear transformation.
Definition at line 193 of file Translation.h.
Scalar& x | ( | ) |
Retruns the x-translation as a reference.
Definition at line 81 of file Translation.h.
Scalar x | ( | ) | const |
Retruns the x-translation by value.
Definition at line 74 of file Translation.h.
Scalar& y | ( | ) |
Retruns the y-translation as a reference.
Definition at line 83 of file Translation.h.
Scalar y | ( | ) | const |
Retruns the y-translation by value.
Definition at line 76 of file Translation.h.
Scalar z | ( | ) | const |
Retruns the z-translation by value.
Definition at line 78 of file Translation.h.
Scalar& z | ( | ) |
Retruns the z-translation as a reference.
Definition at line 85 of file Translation.h.
Friends And Related Function Documentation
AffineTransformType operator* | ( | const EigenBase< OtherDerived > & | linear, |
const Translation< _Scalar, _Dim > & | t | ||
) | [friend] |
- Returns:
- the concatenation of a linear transformation l with the translation t
Definition at line 112 of file Translation.h.
Generated on Tue Jul 12 2022 17:47:05 by
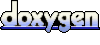