Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Hyperplane< _Scalar, _AmbientDim, _Options > Class Template Reference
[Geometry module]
#include <Hyperplane.h>
Public Member Functions | |
Hyperplane () | |
Default constructor without initialization. | |
Hyperplane (Index _dim) | |
Constructs a dynamic-size hyperplane with _dim the dimension of the ambient space. | |
Hyperplane (const VectorType &n, const VectorType &e) | |
Construct a plane from its normal n and a point e onto the plane. | |
Hyperplane (const VectorType &n, const Scalar &d) | |
Constructs a plane from its normal n and distance to the origin d such that the algebraic equation of the plane is ![]() | |
Hyperplane (const ParametrizedLine< Scalar, AmbientDimAtCompileTime > ¶metrized) | |
Constructs a hyperplane passing through the parametrized line parametrized. | |
Index | dim () const |
void | normalize (void) |
normalizes *this | |
Scalar | signedDistance (const VectorType &p) const |
Scalar | absDistance (const VectorType &p) const |
VectorType | projection (const VectorType &p) const |
ConstNormalReturnType | normal () const |
NormalReturnType | normal () |
const Scalar & | offset () const |
Scalar & | offset () |
const Coefficients & | coeffs () const |
Coefficients & | coeffs () |
VectorType | intersection (const Hyperplane &other) const |
template<typename XprType > | |
Hyperplane & | transform (const MatrixBase< XprType > &mat, TransformTraits traits=Affine) |
Applies the transformation matrix mat to *this and returns a reference to *this . | |
template<int TrOptions> | |
Hyperplane & | transform (const Transform< Scalar, AmbientDimAtCompileTime, Affine, TrOptions > &t, TransformTraits traits=Affine) |
Applies the transformation t to *this and returns a reference to *this . | |
template<typename NewScalarType > | |
internal::cast_return_type < Hyperplane, Hyperplane < NewScalarType, AmbientDimAtCompileTime, Options > >::type | cast () const |
template<typename OtherScalarType , int OtherOptions> | |
Hyperplane (const Hyperplane< OtherScalarType, AmbientDimAtCompileTime, OtherOptions > &other) | |
Copy constructor with scalar type conversion. | |
template<int OtherOptions> | |
bool | isApprox (const Hyperplane< Scalar, AmbientDimAtCompileTime, OtherOptions > &other, const typename NumTraits< Scalar >::Real &prec=NumTraits< Scalar >::dummy_precision()) const |
Static Public Member Functions | |
static Hyperplane | Through (const VectorType &p0, const VectorType &p1) |
Constructs a hyperplane passing through the two points. | |
static Hyperplane | Through (const VectorType &p0, const VectorType &p1, const VectorType &p2) |
Constructs a hyperplane passing through the three points. |
Detailed Description
template<typename _Scalar, int _AmbientDim, int _Options>
class Eigen::Hyperplane< _Scalar, _AmbientDim, _Options >
A hyperplane
A hyperplane is an affine subspace of dimension n-1 in a space of dimension n. For example, a hyperplane in a plane is a line; a hyperplane in 3-space is a plane.
- Parameters:
-
_Scalar the scalar type, i.e., the type of the coefficients _AmbientDim the dimension of the ambient space, can be a compile time value or Dynamic. Notice that the dimension of the hyperplane is _AmbientDim-1.
This class represents an hyperplane as the zero set of the implicit equation where
is a unit normal vector of the plane (linear part) and
is the distance (offset) to the origin.
Definition at line 34 of file Hyperplane.h.
Constructor & Destructor Documentation
Hyperplane | ( | ) |
Default constructor without initialization.
Definition at line 53 of file Hyperplane.h.
Hyperplane | ( | Index | _dim ) | [explicit] |
Constructs a dynamic-size hyperplane with _dim the dimension of the ambient space.
Definition at line 62 of file Hyperplane.h.
Hyperplane | ( | const VectorType & | n, |
const VectorType & | e | ||
) |
Construct a plane from its normal n and a point e onto the plane.
- Warning:
- the vector normal is assumed to be normalized.
Definition at line 67 of file Hyperplane.h.
Hyperplane | ( | const VectorType & | n, |
const Scalar & | d | ||
) |
Constructs a plane from its normal n and distance to the origin d such that the algebraic equation of the plane is .
- Warning:
- the vector normal is assumed to be normalized.
Definition at line 78 of file Hyperplane.h.
Hyperplane | ( | const ParametrizedLine< Scalar, AmbientDimAtCompileTime > & | parametrized ) | [explicit] |
Constructs a hyperplane passing through the parametrized line parametrized.
If the dimension of the ambient space is greater than 2, then there isn't uniqueness, so an arbitrary choice is made.
Definition at line 123 of file Hyperplane.h.
Hyperplane | ( | const Hyperplane< OtherScalarType, AmbientDimAtCompileTime, OtherOptions > & | other ) | [explicit] |
Copy constructor with scalar type conversion.
Definition at line 262 of file Hyperplane.h.
Member Function Documentation
Scalar absDistance | ( | const VectorType & | p ) | const |
- Returns:
- the absolute distance between the plane
*this
and a point p.
- See also:
- signedDistance()
Definition at line 148 of file Hyperplane.h.
internal::cast_return_type<Hyperplane, Hyperplane<NewScalarType,AmbientDimAtCompileTime,Options> >::type cast | ( | ) | const |
- Returns:
*this
with scalar type casted to NewScalarType
Note that if NewScalarType is equal to the current scalar type of *this
then this function smartly returns a const reference to *this
.
Definition at line 254 of file Hyperplane.h.
const Coefficients& coeffs | ( | ) | const |
- Returns:
- a constant reference to the coefficients c_i of the plane equation:
Definition at line 176 of file Hyperplane.h.
Coefficients& coeffs | ( | ) |
- Returns:
- a non-constant reference to the coefficients c_i of the plane equation:
Definition at line 181 of file Hyperplane.h.
Index dim | ( | ) | const |
- Returns:
- the dimension in which the plane holds
Definition at line 132 of file Hyperplane.h.
VectorType intersection | ( | const Hyperplane< _Scalar, _AmbientDim, _Options > & | other ) | const |
- Returns:
- the intersection of *this with other.
- Warning:
- The ambient space must be a plane, i.e. have dimension 2, so that
*this
and other are lines.
- Note:
- If other is approximately parallel to *this, this method will return any point on *this.
Definition at line 189 of file Hyperplane.h.
bool isApprox | ( | const Hyperplane< Scalar, AmbientDimAtCompileTime, OtherOptions > & | other, |
const typename NumTraits< Scalar >::Real & | prec = NumTraits<Scalar>::dummy_precision() |
||
) | const |
- Returns:
true
if*this
is approximately equal to other, within the precision determined by prec.
- See also:
- MatrixBase::isApprox()
Definition at line 270 of file Hyperplane.h.
ConstNormalReturnType normal | ( | ) | const |
- Returns:
- a constant reference to the unit normal vector of the plane, which corresponds to the linear part of the implicit equation.
Definition at line 157 of file Hyperplane.h.
NormalReturnType normal | ( | ) |
- Returns:
- a non-constant reference to the unit normal vector of the plane, which corresponds to the linear part of the implicit equation.
Definition at line 162 of file Hyperplane.h.
void normalize | ( | void | ) |
normalizes *this
Definition at line 135 of file Hyperplane.h.
const Scalar& offset | ( | ) | const |
- Returns:
- the distance to the origin, which is also the "constant term" of the implicit equation
- Warning:
- the vector normal is assumed to be normalized.
Definition at line 167 of file Hyperplane.h.
Scalar& offset | ( | ) |
- Returns:
- a non-constant reference to the distance to the origin, which is also the constant part of the implicit equation
Definition at line 171 of file Hyperplane.h.
VectorType projection | ( | const VectorType & | p ) | const |
- Returns:
- the projection of a point p onto the plane
*this
.
Definition at line 152 of file Hyperplane.h.
Scalar signedDistance | ( | const VectorType & | p ) | const |
- Returns:
- the signed distance between the plane
*this
and a point p.
- See also:
- absDistance()
Definition at line 143 of file Hyperplane.h.
static Hyperplane Through | ( | const VectorType & | p0, |
const VectorType & | p1, | ||
const VectorType & | p2 | ||
) | [static] |
Constructs a hyperplane passing through the three points.
The dimension of the ambient space is required to be exactly 3.
Definition at line 99 of file Hyperplane.h.
static Hyperplane Through | ( | const VectorType & | p0, |
const VectorType & | p1 | ||
) | [static] |
Constructs a hyperplane passing through the two points.
If the dimension of the ambient space is greater than 2, then there isn't uniqueness, so an arbitrary choice is made.
Definition at line 88 of file Hyperplane.h.
Hyperplane& transform | ( | const Transform< Scalar, AmbientDimAtCompileTime, Affine, TrOptions > & | t, |
TransformTraits | traits = Affine |
||
) |
Applies the transformation t to *this
and returns a reference to *this
.
- Parameters:
-
t the transformation of dimension Dim traits specifies whether the transformation t represents an Isometry or a more generic Affine transformation. The default is Affine. Other kind of transformations are not supported.
Definition at line 239 of file Hyperplane.h.
Hyperplane& transform | ( | const MatrixBase< XprType > & | mat, |
TransformTraits | traits = Affine |
||
) |
Applies the transformation matrix mat to *this
and returns a reference to *this
.
- Parameters:
-
mat the Dim x Dim transformation matrix traits specifies whether the matrix mat represents an Isometry or a more generic Affine transformation. The default is Affine.
Definition at line 218 of file Hyperplane.h.
Generated on Tue Jul 12 2022 17:47:05 by
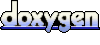