Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
AngleAxis< _Scalar > Class Template Reference
[Geometry module]
#include <AngleAxis.h>
Inherits RotationBase< AngleAxis< _Scalar >, 3 >.
Public Types | |
typedef _Scalar | Scalar |
the scalar type of the coefficients | |
typedef Matrix< Scalar, Dim, Dim > | RotationMatrixType |
corresponding linear transformation matrix type | |
Public Member Functions | |
AngleAxis () | |
Default constructor without initialization. | |
template<typename Derived > | |
AngleAxis (const Scalar &angle, const MatrixBase< Derived > &axis) | |
Constructs and initialize the angle-axis rotation from an angle in radian and an axis which must be normalized. | |
template<typename QuatDerived > | |
AngleAxis (const QuaternionBase< QuatDerived > &q) | |
Constructs and initialize the angle-axis rotation from a quaternion q. | |
template<typename Derived > | |
AngleAxis (const MatrixBase< Derived > &m) | |
Constructs and initialize the angle-axis rotation from a 3x3 rotation matrix. | |
Scalar | angle () const |
Scalar & | angle () |
const Vector3 & | axis () const |
Vector3 & | axis () |
QuaternionType | operator* (const AngleAxis &other) const |
Concatenates two rotations. | |
QuaternionType | operator* (const QuaternionType &other) const |
Concatenates two rotations. | |
AngleAxis | inverse () const |
template<class QuatDerived > | |
AngleAxis & | operator= (const QuaternionBase< QuatDerived > &q) |
Set *this from a unit quaternion. | |
template<typename Derived > | |
AngleAxis & | operator= (const MatrixBase< Derived > &m) |
Set *this from a 3x3 rotation matrix mat. | |
template<typename Derived > | |
AngleAxis & | fromRotationMatrix (const MatrixBase< Derived > &m) |
Sets *this from a 3x3 rotation matrix. | |
Matrix3 | toRotationMatrix (void) const |
Constructs and. | |
template<typename NewScalarType > | |
internal::cast_return_type < AngleAxis, AngleAxis < NewScalarType > >::type | cast () const |
template<typename OtherScalarType > | |
AngleAxis (const AngleAxis< OtherScalarType > &other) | |
Copy constructor with scalar type conversion. | |
bool | isApprox (const AngleAxis &other, const typename NumTraits< Scalar >::Real &prec=NumTraits< Scalar >::dummy_precision()) const |
RotationMatrixType | matrix () const |
Transform< Scalar, Dim, Isometry > | operator* (const Translation< Scalar, Dim > &t) const |
RotationMatrixType | operator* (const UniformScaling< Scalar > &s) const |
EIGEN_STRONG_INLINE internal::rotation_base_generic_product_selector < AngleAxis< _Scalar > , OtherDerived, OtherDerived::IsVectorAtCompileTime > ::ReturnType | operator* (const EigenBase< OtherDerived > &e) const |
Transform< Scalar, Dim, Mode > | operator* (const Transform< Scalar, Dim, Mode, Options > &t) const |
Friends | |
QuaternionType | operator* (const QuaternionType &a, const AngleAxis &b) |
Concatenates two rotations. | |
RotationMatrixType | operator* (const EigenBase< OtherDerived > &l, const AngleAxis< _Scalar > &r) |
Transform< Scalar, Dim, Affine > | operator* (const DiagonalMatrix< Scalar, Dim > &l, const AngleAxis< _Scalar > &r) |
Detailed Description
template<typename _Scalar>
class Eigen::AngleAxis< _Scalar >
Represents a 3D rotation as a rotation angle around an arbitrary 3D axis
- Parameters:
-
_Scalar the scalar type, i.e., the type of the coefficients.
- Warning:
- When setting up an AngleAxis object, the axis vector must be normalized.
The following two typedefs are provided for convenience:
AngleAxisf
forfloat
AngleAxisd
fordouble
Combined with MatrixBase::Unit{X,Y,Z}, AngleAxis can be used to easily mimic Euler-angles. Here is an example:
Output:
- Note:
- This class is not aimed to be used to store a rotation transformation, but rather to make easier the creation of other rotation (Quaternion, rotation Matrix) and transformation objects.
- See also:
- class Quaternion, class Transform, MatrixBase::UnitX()
Definition at line 49 of file AngleAxis.h.
Member Typedef Documentation
typedef Matrix<Scalar,Dim,Dim> RotationMatrixType [inherited] |
corresponding linear transformation matrix type
Definition at line 37 of file RotationBase.h.
typedef _Scalar Scalar |
the scalar type of the coefficients
Reimplemented from RotationBase< AngleAxis< _Scalar >, 3 >.
Definition at line 59 of file AngleAxis.h.
Constructor & Destructor Documentation
AngleAxis | ( | ) |
Default constructor without initialization.
Definition at line 72 of file AngleAxis.h.
AngleAxis | ( | const Scalar & | angle, |
const MatrixBase< Derived > & | axis | ||
) |
Constructs and initialize the angle-axis rotation from an angle in radian and an axis which must be normalized.
- Warning:
- If the axis vector is not normalized, then the angle-axis object represents an invalid rotation.
Definition at line 79 of file AngleAxis.h.
AngleAxis | ( | const QuaternionBase< QuatDerived > & | q ) | [explicit] |
Constructs and initialize the angle-axis rotation from a quaternion q.
Definition at line 81 of file AngleAxis.h.
AngleAxis | ( | const MatrixBase< Derived > & | m ) | [explicit] |
Constructs and initialize the angle-axis rotation from a 3x3 rotation matrix.
Definition at line 84 of file AngleAxis.h.
Copy constructor with scalar type conversion.
Definition at line 135 of file AngleAxis.h.
Member Function Documentation
Scalar angle | ( | ) | const |
- Returns:
- the value of the rotation angle in radian
Definition at line 87 of file AngleAxis.h.
Scalar& angle | ( | ) |
- Returns:
- a read-write reference to the stored angle in radian
Definition at line 89 of file AngleAxis.h.
Vector3& axis | ( | ) |
- Returns:
- a read-write reference to the stored rotation axis.
- Warning:
- The rotation axis must remain a unit vector.
Definition at line 97 of file AngleAxis.h.
const Vector3& axis | ( | ) | const |
- Returns:
- the rotation axis
Definition at line 92 of file AngleAxis.h.
- Returns:
*this
with scalar type casted to NewScalarType
Note that if NewScalarType is equal to the current scalar type of *this
then this function smartly returns a const reference to *this
.
Definition at line 130 of file AngleAxis.h.
AngleAxis< Scalar > & fromRotationMatrix | ( | const MatrixBase< Derived > & | m ) |
Sets *this
from a 3x3 rotation matrix.
Definition at line 202 of file AngleAxis.h.
AngleAxis inverse | ( | ) | const |
- Returns:
- the inverse rotation, i.e., an angle-axis with opposite rotation angle
Reimplemented from RotationBase< AngleAxis< _Scalar >, 3 >.
Definition at line 112 of file AngleAxis.h.
bool isApprox | ( | const AngleAxis< _Scalar > & | other, |
const typename NumTraits< Scalar >::Real & | prec = NumTraits<Scalar>::dummy_precision() |
||
) | const |
- Returns:
true
if*this
is approximately equal to other, within the precision determined by prec.
- See also:
- MatrixBase::isApprox()
Definition at line 147 of file AngleAxis.h.
RotationMatrixType matrix | ( | ) | const [inherited] |
- Returns:
- an equivalent rotation matrix This function is added to be conform with the Transform class' naming scheme.
Definition at line 50 of file RotationBase.h.
QuaternionType operator* | ( | const AngleAxis< _Scalar > & | other ) | const |
Concatenates two rotations.
Definition at line 100 of file AngleAxis.h.
EIGEN_STRONG_INLINE internal::rotation_base_generic_product_selector<AngleAxis< _Scalar > ,OtherDerived,OtherDerived::IsVectorAtCompileTime>::ReturnType operator* | ( | const EigenBase< OtherDerived > & | e ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a generic expression e e can be:- a DimxDim linear transformation matrix
- a DimxDim diagonal matrix (axis aligned scaling)
- a vector of size Dim
Definition at line 71 of file RotationBase.h.
Transform<Scalar,Dim,Isometry> operator* | ( | const Translation< Scalar, Dim > & | t ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a translation t
Definition at line 56 of file RotationBase.h.
RotationMatrixType operator* | ( | const UniformScaling< Scalar > & | s ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a uniform scaling s
Definition at line 60 of file RotationBase.h.
Transform<Scalar,Dim,Mode> operator* | ( | const Transform< Scalar, Dim, Mode, Options > & | t ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a transformation t
Definition at line 89 of file RotationBase.h.
QuaternionType operator* | ( | const QuaternionType & | other ) | const |
Concatenates two rotations.
Definition at line 104 of file AngleAxis.h.
AngleAxis< Scalar > & operator= | ( | const MatrixBase< Derived > & | m ) |
Set *this
from a 3x3 rotation matrix mat.
Definition at line 190 of file AngleAxis.h.
AngleAxis< Scalar > & operator= | ( | const QuaternionBase< QuatDerived > & | q ) |
Set *this
from a unit quaternion.
The axis is normalized.
- Warning:
- As any other method dealing with quaternion, if the input quaternion is not normalized then the result is undefined.
Definition at line 166 of file AngleAxis.h.
Constructs and.
- Returns:
- an equivalent 3x3 rotation matrix.
Reimplemented from RotationBase< AngleAxis< _Scalar >, 3 >.
Definition at line 211 of file AngleAxis.h.
Friends And Related Function Documentation
QuaternionType operator* | ( | const QuaternionType & | a, |
const AngleAxis< _Scalar > & | b | ||
) | [friend] |
Concatenates two rotations.
Definition at line 108 of file AngleAxis.h.
Transform<Scalar,Dim,Affine> operator* | ( | const DiagonalMatrix< Scalar, Dim > & | l, |
const AngleAxis< _Scalar > & | r | ||
) | [friend, inherited] |
- Returns:
- the concatenation of a scaling l with the rotation r
Definition at line 80 of file RotationBase.h.
RotationMatrixType operator* | ( | const EigenBase< OtherDerived > & | l, |
const AngleAxis< _Scalar > & | r | ||
) | [friend, inherited] |
- Returns:
- the concatenation of a linear transformation l with the rotation r
Definition at line 76 of file RotationBase.h.
Generated on Tue Jul 12 2022 17:47:05 by
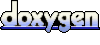