Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
VectorwiseOp< ExpressionType, Direction > Class Template Reference
[Core module]
Pseudo expression providing partial reduction operations. More...
#include <VectorwiseOp.h>
Public Member Functions | |
template<typename BinaryOp > | |
const ReduxReturnType < BinaryOp >::Type | redux (const BinaryOp &func=BinaryOp()) const |
const ReturnType < internal::member_minCoeff > ::Type | minCoeff () const |
const ReturnType < internal::member_maxCoeff > ::Type | maxCoeff () const |
const ReturnType < internal::member_squaredNorm, RealScalar >::Type | squaredNorm () const |
const ReturnType < internal::member_norm, RealScalar >::Type | norm () const |
const ReturnType < internal::member_blueNorm, RealScalar >::Type | blueNorm () const |
const ReturnType < internal::member_stableNorm, RealScalar >::Type | stableNorm () const |
const ReturnType < internal::member_hypotNorm, RealScalar >::Type | hypotNorm () const |
const ReturnType < internal::member_sum >::Type | sum () const |
const ReturnType < internal::member_mean > ::Type | mean () const |
const ReturnType < internal::member_all >::Type | all () const |
const ReturnType < internal::member_any >::Type | any () const |
const PartialReduxExpr < ExpressionType, internal::member_count< Index > , Direction > | count () const |
const ReturnType < internal::member_prod > ::Type | prod () const |
const Reverse< ExpressionType, Direction > | reverse () const |
const ReplicateReturnType | replicate (Index factor) const |
template<int Factor> | |
const Replicate < ExpressionType,(IsVertical?Factor:1),(IsHorizontal?Factor:1)> | replicate (Index factor=Factor) const |
template<typename OtherDerived > | |
ExpressionType & | operator= (const DenseBase< OtherDerived > &other) |
Copies the vector other to each subvector of *this . | |
template<typename OtherDerived > | |
ExpressionType & | operator+= (const DenseBase< OtherDerived > &other) |
Adds the vector other to each subvector of *this . | |
template<typename OtherDerived > | |
ExpressionType & | operator-= (const DenseBase< OtherDerived > &other) |
Substracts the vector other to each subvector of *this . | |
template<typename OtherDerived > | |
ExpressionType & | operator*= (const DenseBase< OtherDerived > &other) |
Multiples each subvector of *this by the vector other. | |
template<typename OtherDerived > | |
ExpressionType & | operator/= (const DenseBase< OtherDerived > &other) |
Divides each subvector of *this by the vector other. | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE CwiseBinaryOp < internal::scalar_sum_op < Scalar >, const ExpressionTypeNestedCleaned, const typename ExtendedType < OtherDerived >::Type > | operator+ (const DenseBase< OtherDerived > &other) const |
Returns the expression of the sum of the vector other to each subvector of *this . | |
template<typename OtherDerived > | |
CwiseBinaryOp < internal::scalar_difference_op < Scalar >, const ExpressionTypeNestedCleaned, const typename ExtendedType < OtherDerived >::Type > | operator- (const DenseBase< OtherDerived > &other) const |
Returns the expression of the difference between each subvector of *this and the vector other. | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE CwiseBinaryOp < internal::scalar_product_op < Scalar >, const ExpressionTypeNestedCleaned, const typename ExtendedType < OtherDerived >::Type > | operator* (const DenseBase< OtherDerived > &other) const |
Returns the expression where each subvector is the product of the vector other by the corresponding subvector of *this . | |
template<typename OtherDerived > | |
CwiseBinaryOp < internal::scalar_quotient_op < Scalar >, const ExpressionTypeNestedCleaned, const typename ExtendedType < OtherDerived >::Type > | operator/ (const DenseBase< OtherDerived > &other) const |
Returns the expression where each subvector is the quotient of the corresponding subvector of *this by the vector other. | |
CwiseBinaryOp < internal::scalar_quotient_op < Scalar >, const ExpressionTypeNestedCleaned, const typename OppositeExtendedType< typename ReturnType < internal::member_norm, RealScalar >::Type >::Type > | normalized () const |
void | normalize () |
Normalize in-place each row or columns of the referenced matrix. | |
Homogeneous< ExpressionType, Direction > | homogeneous () const |
| |
template<typename OtherDerived > | |
const CrossReturnType | cross (const MatrixBase< OtherDerived > &other) const |
const HNormalizedReturnType | hnormalized () const |
|
Detailed Description
template<typename ExpressionType, int Direction>
class Eigen::VectorwiseOp< ExpressionType, Direction >
Pseudo expression providing partial reduction operations.
- Parameters:
-
ExpressionType the type of the object on which to do partial reductions Direction indicates the direction of the redux (Vertical or Horizontal)
This class represents a pseudo expression with partial reduction features. It is the return type of DenseBase::colwise() and DenseBase::rowwise() and most of the time this is the only way it is used.
Example:
Output:
- See also:
- DenseBase::colwise(), DenseBase::rowwise(), class PartialReduxExpr
Definition at line 166 of file VectorwiseOp.h.
Member Function Documentation
const ReturnType<internal::member_all>::Type all | ( | void | ) | const |
- Returns:
- a row (or column) vector expression representing whether all coefficients of each respective column (or row) are
true
.
- See also:
- DenseBase::all()
Definition at line 371 of file VectorwiseOp.h.
const ReturnType<internal::member_any>::Type any | ( | void | ) | const |
- Returns:
- a row (or column) vector expression representing whether at least one coefficient of each respective column (or row) is
true
.
- See also:
- DenseBase::any()
Definition at line 378 of file VectorwiseOp.h.
const ReturnType<internal::member_blueNorm,RealScalar>::Type blueNorm | ( | ) | const |
- Returns:
- a row (or column) vector expression of the norm of each column (or row) of the referenced expression, using blue's algorithm.
- See also:
- DenseBase::blueNorm()
Definition at line 329 of file VectorwiseOp.h.
const PartialReduxExpr<ExpressionType, internal::member_count<Index>, Direction> count | ( | ) | const |
- Returns:
- a row (or column) vector expression representing the number of
true
coefficients of each respective column (or row).
Example:
Output:
- See also:
- DenseBase::count()
Definition at line 388 of file VectorwiseOp.h.
const VectorwiseOp< ExpressionType, Direction >::CrossReturnType cross | ( | const MatrixBase< OtherDerived > & | other ) | const |
- Returns:
- a matrix expression of the cross product of each column or row of the referenced expression with the other vector.
The referenced matrix must have one dimension equal to 3. The result matrix has the same dimensions than the referenced one.
- See also:
- MatrixBase::cross()
Definition at line 101 of file OrthoMethods.h.
const VectorwiseOp< ExpressionType, Direction >::HNormalizedReturnType hnormalized | ( | ) | const |
- Returns:
- an expression of the homogeneous normalized vector of
*this
Example:
Output:
- See also:
- MatrixBase::hnormalized()
Definition at line 176 of file Homogeneous.h.
Homogeneous< ExpressionType, Direction > homogeneous | ( | ) | const |
- Returns:
- a matrix expression of homogeneous column (or row) vectors
Example:
Output:
- See also:
- MatrixBase::homogeneous()
Definition at line 143 of file Homogeneous.h.
const ReturnType<internal::member_hypotNorm,RealScalar>::Type hypotNorm | ( | ) | const |
- Returns:
- a row (or column) vector expression of the norm of each column (or row) of the referenced expression, avoiding underflow and overflow using a concatenation of hypot() calls.
- See also:
- DenseBase::hypotNorm()
Definition at line 347 of file VectorwiseOp.h.
const ReturnType<internal::member_maxCoeff>::Type maxCoeff | ( | ) | const |
- Returns:
- a row (or column) vector expression of the largest coefficient of each column (or row) of the referenced expression.
- Warning:
- the result is undefined if
*this
contains NaN.
Example:
Output:
- See also:
- DenseBase::maxCoeff()
Definition at line 300 of file VectorwiseOp.h.
const ReturnType<internal::member_mean>::Type mean | ( | ) | const |
- Returns:
- a row (or column) vector expression of the mean of each column (or row) of the referenced expression.
- See also:
- DenseBase::mean()
Definition at line 364 of file VectorwiseOp.h.
const ReturnType<internal::member_minCoeff>::Type minCoeff | ( | ) | const |
- Returns:
- a row (or column) vector expression of the smallest coefficient of each column (or row) of the referenced expression.
- Warning:
- the result is undefined if
*this
contains NaN.
Example:
Output:
- See also:
- DenseBase::minCoeff()
Definition at line 288 of file VectorwiseOp.h.
const ReturnType<internal::member_norm,RealScalar>::Type norm | ( | ) | const |
- Returns:
- a row (or column) vector expression of the norm of each column (or row) of the referenced expression.
Example:
Output:
- See also:
- DenseBase::norm()
Definition at line 320 of file VectorwiseOp.h.
void normalize | ( | ) |
Normalize in-place each row or columns of the referenced matrix.
- See also:
- MatrixBase::normalize(), normalized()
Definition at line 548 of file VectorwiseOp.h.
CwiseBinaryOp<internal::scalar_quotient_op<Scalar>, const ExpressionTypeNestedCleaned, const typename OppositeExtendedType<typename ReturnType<internal::member_norm,RealScalar>::Type>::Type> normalized | ( | ) | const |
- Returns:
- an expression where each column of row of the referenced matrix are normalized. The referenced matrix is not modified.
- See also:
- MatrixBase::normalized(), normalize()
Definition at line 542 of file VectorwiseOp.h.
EIGEN_STRONG_INLINE CwiseBinaryOp<internal::scalar_product_op<Scalar>, const ExpressionTypeNestedCleaned, const typename ExtendedType<OtherDerived>::Type> operator* | ( | const DenseBase< OtherDerived > & | other ) | const |
Returns the expression where each subvector is the product of the vector other by the corresponding subvector of *this
.
Definition at line 513 of file VectorwiseOp.h.
ExpressionType& operator*= | ( | const DenseBase< OtherDerived > & | other ) |
Multiples each subvector of *this
by the vector other.
Definition at line 463 of file VectorwiseOp.h.
EIGEN_STRONG_INLINE CwiseBinaryOp<internal::scalar_sum_op<Scalar>, const ExpressionTypeNestedCleaned, const typename ExtendedType<OtherDerived>::Type> operator+ | ( | const DenseBase< OtherDerived > & | other ) | const |
Returns the expression of the sum of the vector other to each subvector of *this
.
Definition at line 488 of file VectorwiseOp.h.
ExpressionType& operator+= | ( | const DenseBase< OtherDerived > & | other ) |
Adds the vector other to each subvector of *this
.
Definition at line 445 of file VectorwiseOp.h.
CwiseBinaryOp<internal::scalar_difference_op<Scalar>, const ExpressionTypeNestedCleaned, const typename ExtendedType<OtherDerived>::Type> operator- | ( | const DenseBase< OtherDerived > & | other ) | const |
Returns the expression of the difference between each subvector of *this
and the vector other.
Definition at line 500 of file VectorwiseOp.h.
ExpressionType& operator-= | ( | const DenseBase< OtherDerived > & | other ) |
Substracts the vector other to each subvector of *this
.
Definition at line 454 of file VectorwiseOp.h.
CwiseBinaryOp<internal::scalar_quotient_op<Scalar>, const ExpressionTypeNestedCleaned, const typename ExtendedType<OtherDerived>::Type> operator/ | ( | const DenseBase< OtherDerived > & | other ) | const |
Returns the expression where each subvector is the quotient of the corresponding subvector of *this
by the vector other.
Definition at line 527 of file VectorwiseOp.h.
ExpressionType& operator/= | ( | const DenseBase< OtherDerived > & | other ) |
Divides each subvector of *this
by the vector other.
Definition at line 474 of file VectorwiseOp.h.
ExpressionType& operator= | ( | const DenseBase< OtherDerived > & | other ) |
Copies the vector other to each subvector of *this
.
Definition at line 435 of file VectorwiseOp.h.
const ReturnType<internal::member_prod>::Type prod | ( | ) | const |
- Returns:
- a row (or column) vector expression of the product of each column (or row) of the referenced expression.
Example:
Output:
- See also:
- DenseBase::prod()
Definition at line 398 of file VectorwiseOp.h.
const ReduxReturnType<BinaryOp>::Type redux | ( | const BinaryOp & | func = BinaryOp() ) |
const |
- Returns:
- a row or column vector expression of
*this
reduxed by func
The template parameter BinaryOp is the type of the functor of the custom redux operator. Note that func must be an associative operator.
- See also:
- class VectorwiseOp, DenseBase::colwise(), DenseBase::rowwise()
Definition at line 276 of file VectorwiseOp.h.
const VectorwiseOp< ExpressionType, Direction >::ReplicateReturnType replicate | ( | Index | factor ) | const |
- Returns:
- an expression of the replication of each column (or row) of
*this
Example:
Output:
- See also:
- VectorwiseOp::replicate(), DenseBase::replicate(), class Replicate
Definition at line 169 of file Replicate.h.
const Replicate<ExpressionType,(IsVertical?Factor:1),(IsHorizontal?Factor:1)> replicate | ( | Index | factor = Factor ) |
const |
- Returns:
- an expression of the replication of each column (or row) of
*this
Example:
Output:
- See also:
- VectorwiseOp::replicate(Index), DenseBase::replicate(), class Replicate
Definition at line 425 of file VectorwiseOp.h.
const Reverse<ExpressionType, Direction> reverse | ( | ) | const |
- Returns:
- a matrix expression where each column (or row) are reversed.
Example:
Output:
- See also:
- DenseBase::reverse()
Definition at line 409 of file VectorwiseOp.h.
const ReturnType<internal::member_squaredNorm,RealScalar>::Type squaredNorm | ( | ) | const |
- Returns:
- a row (or column) vector expression of the squared norm of each column (or row) of the referenced expression.
Example:
Output:
- See also:
- DenseBase::squaredNorm()
Definition at line 310 of file VectorwiseOp.h.
const ReturnType<internal::member_stableNorm,RealScalar>::Type stableNorm | ( | ) | const |
- Returns:
- a row (or column) vector expression of the norm of each column (or row) of the referenced expression, avoiding underflow and overflow.
- See also:
- DenseBase::stableNorm()
Definition at line 338 of file VectorwiseOp.h.
const ReturnType<internal::member_sum>::Type sum | ( | ) | const |
- Returns:
- a row (or column) vector expression of the sum of each column (or row) of the referenced expression.
Example:
Output:
- See also:
- DenseBase::sum()
Definition at line 357 of file VectorwiseOp.h.
Generated on Tue Jul 12 2022 17:47:05 by
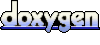