Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
AlignedBox< _Scalar, _AmbientDim > Class Template Reference
[Geometry module]
#include <AlignedBox.h>
Public Types | |
enum | CornerType { Min = 0 , BottomLeft = 0 , BottomLeftFloor = 0 } |
Define constants to name the corners of a 1D, 2D or 3D axis aligned bounding box. More... | |
Public Member Functions | |
AlignedBox () | |
Default constructor initializing a null box. | |
AlignedBox (Index _dim) | |
Constructs a null box with _dim the dimension of the ambient space. | |
template<typename OtherVectorType1 , typename OtherVectorType2 > | |
AlignedBox (const OtherVectorType1 &_min, const OtherVectorType2 &_max) | |
Constructs a box with extremities _min and _max. | |
template<typename Derived > | |
AlignedBox (const MatrixBase< Derived > &p) | |
Constructs a box containing a single point p. | |
Index | dim () const |
bool | isNull () const |
void | setNull () |
bool | isEmpty () const |
void | setEmpty () |
Makes *this an empty box. | |
const VectorType &() | min () const |
VectorType &() | min () |
const VectorType &() | max () const |
VectorType &() | max () |
const CwiseUnaryOp < internal::scalar_quotient1_op < Scalar >, const CwiseBinaryOp < internal::scalar_sum_op < Scalar >, const VectorType, const VectorType > > | center () const |
const CwiseBinaryOp < internal::scalar_difference_op < Scalar >, const VectorType, const VectorType > | sizes () const |
Scalar | volume () const |
CwiseBinaryOp < internal::scalar_difference_op < Scalar >, const VectorType, const VectorType > | diagonal () const |
VectorType | corner (CornerType corner) const |
VectorType | sample () const |
template<typename Derived > | |
bool | contains (const MatrixBase< Derived > &p) const |
bool | contains (const AlignedBox &b) const |
bool | intersects (const AlignedBox &b) const |
template<typename Derived > | |
AlignedBox & | extend (const MatrixBase< Derived > &p) |
Extends *this such that it contains the point p and returns a reference to *this . | |
AlignedBox & | extend (const AlignedBox &b) |
Extends *this such that it contains the box b and returns a reference to *this . | |
AlignedBox & | clamp (const AlignedBox &b) |
Clamps *this by the box b and returns a reference to *this . | |
AlignedBox | intersection (const AlignedBox &b) const |
Returns an AlignedBox that is the intersection of b and *this . | |
AlignedBox | merged (const AlignedBox &b) const |
Returns an AlignedBox that is the union of b and *this . | |
template<typename Derived > | |
AlignedBox & | translate (const MatrixBase< Derived > &a_t) |
Translate *this by the vector t and returns a reference to *this . | |
template<typename Derived > | |
Scalar | squaredExteriorDistance (const MatrixBase< Derived > &p) const |
Scalar | squaredExteriorDistance (const AlignedBox &b) const |
template<typename Derived > | |
NonInteger | exteriorDistance (const MatrixBase< Derived > &p) const |
NonInteger | exteriorDistance (const AlignedBox &b) const |
template<typename NewScalarType > | |
internal::cast_return_type < AlignedBox, AlignedBox < NewScalarType, AmbientDimAtCompileTime > >::type | cast () const |
template<typename OtherScalarType > | |
AlignedBox (const AlignedBox< OtherScalarType, AmbientDimAtCompileTime > &other) | |
Copy constructor with scalar type conversion. | |
bool | isApprox (const AlignedBox &other, const RealScalar &prec=ScalarTraits::dummy_precision()) const |
Detailed Description
template<typename _Scalar, int _AmbientDim>
class Eigen::AlignedBox< _Scalar, _AmbientDim >
An axis aligned box
- Template Parameters:
-
_Scalar the type of the scalar coefficients _AmbientDim the dimension of the ambient space, can be a compile time value or Dynamic.
This class represents an axis aligned box as a pair of the minimal and maximal corners.
- Warning:
- The result of most methods is undefined when applied to an empty box. You can check for empty boxes using isEmpty().
- See also:
- Global aligned box typedefs
Definition at line 30 of file AlignedBox.h.
Member Enumeration Documentation
enum CornerType |
Define constants to name the corners of a 1D, 2D or 3D axis aligned bounding box.
- Enumerator:
Min 1D names
BottomLeft Identifier for 2D corner.
BottomLeftFloor Identifier for 3D corner.
Definition at line 43 of file AlignedBox.h.
Constructor & Destructor Documentation
AlignedBox | ( | ) |
Default constructor initializing a null box.
Definition at line 64 of file AlignedBox.h.
AlignedBox | ( | Index | _dim ) | [explicit] |
Constructs a null box with _dim the dimension of the ambient space.
Definition at line 68 of file AlignedBox.h.
AlignedBox | ( | const OtherVectorType1 & | _min, |
const OtherVectorType2 & | _max | ||
) |
Constructs a box with extremities _min and _max.
- Warning:
- If either component of _min is larger than the same component of _max, the constructed box is empty.
Definition at line 74 of file AlignedBox.h.
AlignedBox | ( | const MatrixBase< Derived > & | p ) | [explicit] |
Constructs a box containing a single point p.
Definition at line 78 of file AlignedBox.h.
AlignedBox | ( | const AlignedBox< OtherScalarType, AmbientDimAtCompileTime > & | other ) | [explicit] |
Copy constructor with scalar type conversion.
Definition at line 292 of file AlignedBox.h.
Member Function Documentation
internal::cast_return_type<AlignedBox, AlignedBox<NewScalarType,AmbientDimAtCompileTime> >::type cast | ( | ) | const |
- Returns:
*this
with scalar type casted to NewScalarType
Note that if NewScalarType is equal to the current scalar type of *this
then this function smartly returns a const reference to *this
.
Definition at line 284 of file AlignedBox.h.
const CwiseUnaryOp<internal::scalar_quotient1_op<Scalar>, const CwiseBinaryOp<internal::scalar_sum_op<Scalar>, const VectorType, const VectorType> > center | ( | ) | const |
- Returns:
- the center of the box
Definition at line 116 of file AlignedBox.h.
AlignedBox& clamp | ( | const AlignedBox< _Scalar, _AmbientDim > & | b ) |
Clamps *this
by the box b and returns a reference to *this
.
- Note:
- If the boxes don't intersect, the resulting box is empty.
- See also:
- intersection(), intersects()
Definition at line 220 of file AlignedBox.h.
bool contains | ( | const AlignedBox< _Scalar, _AmbientDim > & | b ) | const |
- Returns:
- true if the box b is entirely inside the box
*this
.
Definition at line 189 of file AlignedBox.h.
bool contains | ( | const MatrixBase< Derived > & | p ) | const |
- Returns:
- true if the point p is inside the box
*this
.
Definition at line 182 of file AlignedBox.h.
VectorType corner | ( | CornerType | corner ) | const |
- Returns:
- the vertex of the bounding box at the corner defined by the corner-id corner. It works only for a 1D, 2D or 3D bounding box. For 1D bounding boxes corners are named by 2 enum constants: BottomLeft and BottomRight. For 2D bounding boxes, corners are named by 4 enum constants: BottomLeft, BottomRight, TopLeft, TopRight. For 3D bounding boxes, the following names are added: BottomLeftCeil, BottomRightCeil, TopLeftCeil, TopRightCeil.
Definition at line 146 of file AlignedBox.h.
CwiseBinaryOp< internal::scalar_difference_op<Scalar>, const VectorType, const VectorType> diagonal | ( | ) | const |
- Returns:
- an expression for the bounding box diagonal vector if the length of the diagonal is needed: diagonal().norm() will provide it.
Definition at line 134 of file AlignedBox.h.
Index dim | ( | ) | const |
- Returns:
- the dimension in which the box holds
Definition at line 84 of file AlignedBox.h.
AlignedBox& extend | ( | const MatrixBase< Derived > & | p ) |
Extends *this
such that it contains the point p and returns a reference to *this
.
- See also:
- extend(const AlignedBox&)
Definition at line 200 of file AlignedBox.h.
AlignedBox& extend | ( | const AlignedBox< _Scalar, _AmbientDim > & | b ) |
Extends *this
such that it contains the box b and returns a reference to *this
.
- See also:
- merged, extend(const MatrixBase&)
Definition at line 210 of file AlignedBox.h.
NonInteger exteriorDistance | ( | const AlignedBox< _Scalar, _AmbientDim > & | b ) | const |
- Returns:
- the distance between the boxes b and
*this
, and zero if the boxes intersect.
- See also:
- squaredExteriorDistance(const AlignedBox&), exteriorDistance(const MatrixBase&)
Definition at line 274 of file AlignedBox.h.
NonInteger exteriorDistance | ( | const MatrixBase< Derived > & | p ) | const |
- Returns:
- the distance between the point p and the box
*this
, and zero if p is inside the box.
- See also:
- squaredExteriorDistance(const MatrixBase&), exteriorDistance(const AlignedBox&)
Definition at line 267 of file AlignedBox.h.
AlignedBox intersection | ( | const AlignedBox< _Scalar, _AmbientDim > & | b ) | const |
Returns an AlignedBox that is the intersection of b and *this
.
- Note:
- If the boxes don't intersect, the resulting box is empty.
- See also:
- intersects(), clamp, contains()
Definition at line 230 of file AlignedBox.h.
bool intersects | ( | const AlignedBox< _Scalar, _AmbientDim > & | b ) | const |
- Returns:
- true if the box b is intersecting the box
*this
.
- See also:
- intersection, clamp
Definition at line 194 of file AlignedBox.h.
bool isApprox | ( | const AlignedBox< _Scalar, _AmbientDim > & | other, |
const RealScalar & | prec = ScalarTraits::dummy_precision() |
||
) | const |
- Returns:
true
if*this
is approximately equal to other, within the precision determined by prec.
- See also:
- MatrixBase::isApprox()
Definition at line 302 of file AlignedBox.h.
bool isEmpty | ( | ) | const |
bool isNull | ( | ) | const |
Definition at line 87 of file AlignedBox.h.
const VectorType&() max | ( | ) | const |
- Returns:
- the maximal corner
Definition at line 109 of file AlignedBox.h.
VectorType&() max | ( | ) |
- Returns:
- a non const reference to the maximal corner
Definition at line 111 of file AlignedBox.h.
AlignedBox merged | ( | const AlignedBox< _Scalar, _AmbientDim > & | b ) | const |
Returns an AlignedBox that is the union of b and *this
.
- Note:
- Merging with an empty box may result in a box bigger than
*this
.
- See also:
- extend(const AlignedBox&)
Definition at line 236 of file AlignedBox.h.
const VectorType&() min | ( | ) | const |
- Returns:
- the minimal corner
Definition at line 105 of file AlignedBox.h.
VectorType&() min | ( | ) |
- Returns:
- a non const reference to the minimal corner
Definition at line 107 of file AlignedBox.h.
VectorType sample | ( | ) | const |
- Returns:
- a random point inside the bounding box sampled with a uniform distribution
Definition at line 164 of file AlignedBox.h.
void setEmpty | ( | ) |
void setNull | ( | ) |
Definition at line 90 of file AlignedBox.h.
const CwiseBinaryOp< internal::scalar_difference_op<Scalar>, const VectorType, const VectorType> sizes | ( | ) | const |
- Returns:
- the lengths of the sides of the bounding box. Note that this function does not get the same result for integral or floating scalar types: see
Definition at line 123 of file AlignedBox.h.
Scalar squaredExteriorDistance | ( | const AlignedBox< _Scalar, _AmbientDim > & | b ) | const |
- Returns:
- the squared distance between the boxes b and
*this
, and zero if the boxes intersect.
- See also:
- exteriorDistance(const AlignedBox&), squaredExteriorDistance(const MatrixBase&)
Definition at line 336 of file AlignedBox.h.
Scalar squaredExteriorDistance | ( | const MatrixBase< Derived > & | p ) | const |
- Returns:
- the squared distance between the point p and the box
*this
, and zero if p is inside the box.
- See also:
- exteriorDistance(const MatrixBase&), squaredExteriorDistance(const AlignedBox&)
Definition at line 314 of file AlignedBox.h.
AlignedBox& translate | ( | const MatrixBase< Derived > & | a_t ) |
Translate *this
by the vector t and returns a reference to *this
.
Definition at line 241 of file AlignedBox.h.
Scalar volume | ( | ) | const |
- Returns:
- the volume of the bounding box
Definition at line 127 of file AlignedBox.h.
Generated on Tue Jul 12 2022 17:47:05 by
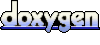