Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
PlainObjectBase< Derived > Class Template Reference
Dense storage base class for matrices and arrays. More...
#include <PlainObjectBase.h>
Public Member Functions | |
EIGEN_STRONG_INLINE const Scalar * | data () const |
EIGEN_STRONG_INLINE Scalar * | data () |
EIGEN_STRONG_INLINE void | resize (Index nbRows, Index nbCols) |
Resizes *this to a rows x cols matrix. | |
void | resize (Index size) |
Resizes *this to a vector of length size. | |
void | resize (NoChange_t, Index nbCols) |
Resizes the matrix, changing only the number of columns. | |
void | resize (Index nbRows, NoChange_t) |
Resizes the matrix, changing only the number of rows. | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE void | resizeLike (const EigenBase< OtherDerived > &_other) |
Resizes *this to have the same dimensions as other. | |
EIGEN_STRONG_INLINE void | conservativeResize (Index nbRows, Index nbCols) |
Resizes the matrix to rows x cols while leaving old values untouched. | |
EIGEN_STRONG_INLINE void | conservativeResize (Index nbRows, NoChange_t) |
Resizes the matrix to rows x cols while leaving old values untouched. | |
EIGEN_STRONG_INLINE void | conservativeResize (NoChange_t, Index nbCols) |
Resizes the matrix to rows x cols while leaving old values untouched. | |
EIGEN_STRONG_INLINE void | conservativeResize (Index size) |
Resizes the vector to size while retaining old values. | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE void | conservativeResizeLike (const DenseBase< OtherDerived > &other) |
Resizes the matrix to rows x cols of other , while leaving old values untouched. | |
EIGEN_STRONG_INLINE Derived & | operator= (const PlainObjectBase &other) |
This is a special case of the templated operator=. | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE Derived & | lazyAssign (const DenseBase< OtherDerived > &other) |
EIGEN_STRONG_INLINE | PlainObjectBase (const PlainObjectBase &other) |
Copy constructor. | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE Derived & | operator= (const EigenBase< OtherDerived > &other) |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE | PlainObjectBase (const EigenBase< OtherDerived > &other) |
Derived & | setConstant (Index size, const Scalar &value) |
Resizes to the given size, and sets all coefficients in this expression to the given value. | |
Derived & | setConstant (Index rows, Index cols, const Scalar &value) |
Resizes to the given size, and sets all coefficients in this expression to the given value. | |
Derived & | setZero (Index size) |
Resizes to the given size, and sets all coefficients in this expression to zero. | |
Derived & | setZero (Index rows, Index cols) |
Resizes to the given size, and sets all coefficients in this expression to zero. | |
Derived & | setOnes (Index size) |
Resizes to the given newSize, and sets all coefficients in this expression to one. | |
Derived & | setOnes (Index rows, Index cols) |
Resizes to the given size, and sets all coefficients in this expression to one. | |
Derived & | setRandom (Index size) |
Resizes to the given newSize, and sets all coefficients in this expression to random values. | |
Derived & | setRandom (Index rows, Index cols) |
Resizes to the given size, and sets all coefficients in this expression to random values. | |
Static Public Member Functions | |
Map | |
static ConstMapType | Map (const Scalar *data) |
static MapType | Map (Scalar *data) |
static ConstMapType | Map (const Scalar *data, Index size) |
static MapType | Map (Scalar *data, Index size) |
static ConstMapType | Map (const Scalar *data, Index rows, Index cols) |
static MapType | Map (Scalar *data, Index rows, Index cols) |
static ConstAlignedMapType | MapAligned (const Scalar *data) |
static AlignedMapType | MapAligned (Scalar *data) |
static ConstAlignedMapType | MapAligned (const Scalar *data, Index size) |
static AlignedMapType | MapAligned (Scalar *data, Index size) |
static ConstAlignedMapType | MapAligned (const Scalar *data, Index rows, Index cols) |
static AlignedMapType | MapAligned (Scalar *data, Index rows, Index cols) |
template<int Outer, int Inner> | |
static StridedConstMapType < Stride< Outer, Inner > >::type | Map (const Scalar *data, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedMapType< Stride < Outer, Inner > >::type | Map (Scalar *data, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedConstMapType < Stride< Outer, Inner > >::type | Map (const Scalar *data, Index size, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedMapType< Stride < Outer, Inner > >::type | Map (Scalar *data, Index size, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedConstMapType < Stride< Outer, Inner > >::type | Map (const Scalar *data, Index rows, Index cols, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedMapType< Stride < Outer, Inner > >::type | Map (Scalar *data, Index rows, Index cols, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedConstAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (const Scalar *data, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (Scalar *data, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedConstAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (const Scalar *data, Index size, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (Scalar *data, Index size, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedConstAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (const Scalar *data, Index rows, Index cols, const Stride< Outer, Inner > &stride) |
template<int Outer, int Inner> | |
static StridedAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (Scalar *data, Index rows, Index cols, const Stride< Outer, Inner > &stride) |
Protected Member Functions | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE Derived & | _set (const DenseBase< OtherDerived > &other) |
Copies the value of the expression other into *this with automatic resizing. | |
Friends | |
class | Eigen::Map |
class | Eigen::Map< Derived, Unaligned > |
class | Eigen::Map< const Derived, Unaligned > |
class | Eigen::Map< Derived, Aligned > |
class | Eigen::Map< const Derived, Aligned > |
Detailed Description
template<typename Derived>
class Eigen::PlainObjectBase< Derived >
Dense storage base class for matrices and arrays.
This class can be extended with the help of the plugin mechanism described on the page TopicCustomizingEigen by defining the preprocessor symbol EIGEN_PLAINOBJECTBASE_PLUGIN
.
- See also:
- TopicClassHierarchy
Definition at line 85 of file PlainObjectBase.h.
Constructor & Destructor Documentation
EIGEN_STRONG_INLINE PlainObjectBase | ( | const PlainObjectBase< Derived > & | other ) |
Copy constructor.
Definition at line 455 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE PlainObjectBase | ( | const EigenBase< OtherDerived > & | other ) |
- See also:
- MatrixBase::operator=(const EigenBase<OtherDerived>&)
Definition at line 489 of file PlainObjectBase.h.
Member Function Documentation
EIGEN_STRONG_INLINE Derived& _set | ( | const DenseBase< OtherDerived > & | other ) | [protected] |
Copies the value of the expression other into *this
with automatic resizing.
*this might be resized to match the dimensions of other. If *this was a null matrix (not already initialized), it will be initialized.
Note that copying a row-vector into a vector (and conversely) is allowed. The resizing, if any, is then done in the appropriate way so that row-vectors remain row-vectors and vectors remain vectors.
- See also:
- operator=(const MatrixBase<OtherDerived>&), _set_noalias()
Definition at line 628 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void conservativeResize | ( | Index | nbRows, |
Index | nbCols | ||
) |
Resizes the matrix to rows x cols while leaving old values untouched.
The method is intended for matrices of dynamic size. If you only want to change the number of rows and/or of columns, you can use conservativeResize(NoChange_t, Index) or conservativeResize(Index, NoChange_t).
Matrices are resized relative to the top-left element. In case values need to be appended to the matrix they will be uninitialized.
Definition at line 342 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void conservativeResize | ( | Index | nbRows, |
NoChange_t | |||
) |
Resizes the matrix to rows x cols while leaving old values untouched.
As opposed to conservativeResize(Index rows, Index cols), this version leaves the number of columns unchanged.
In case the matrix is growing, new rows will be uninitialized.
Definition at line 354 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void conservativeResize | ( | NoChange_t | , |
Index | nbCols | ||
) |
Resizes the matrix to rows x cols while leaving old values untouched.
As opposed to conservativeResize(Index rows, Index cols), this version leaves the number of rows unchanged.
In case the matrix is growing, new columns will be uninitialized.
Definition at line 367 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void conservativeResize | ( | Index | size ) |
Resizes the vector to size while retaining old values.
. This method does not work for partially dynamic matrices when the static dimension is anything other than 1. For example it will not work with Matrix<double, 2, Dynamic>.
When values are appended, they will be uninitialized.
Definition at line 381 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void conservativeResizeLike | ( | const DenseBase< OtherDerived > & | other ) |
Resizes the matrix to rows x cols of other
, while leaving old values untouched.
The method is intended for matrices of dynamic size. If you only want to change the number of rows and/or of columns, you can use conservativeResize(NoChange_t, Index) or conservativeResize(Index, NoChange_t).
Matrices are resized relative to the top-left element. In case values need to be appended to the matrix they will copied from other
.
Definition at line 396 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE Scalar* data | ( | ) |
- Returns:
- a pointer to the data array of this matrix
Definition at line 216 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE const Scalar* data | ( | ) | const |
- Returns:
- a const pointer to the data array of this matrix
Definition at line 212 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE Derived& lazyAssign | ( | const DenseBase< OtherDerived > & | other ) |
- See also:
- MatrixBase::lazyAssign()
Definition at line 411 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE Derived& operator= | ( | const EigenBase< OtherDerived > & | other ) |
Reimplemented in Array< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols >, Matrix< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols >, Matrix< Scalar, RowsAtCompileTime, 1, Options, MaxRowsAtCompileTime, 1 >, Matrix< Index, 1, EIGEN_SIZE_MIN_PREFER_DYNAMIC(ColsAtCompileTime, RowsAtCompileTime), RowMajor, 1, EIGEN_SIZE_MIN_PREFER_FIXED(MaxColsAtCompileTime, MaxRowsAtCompileTime)>, Matrix< Scalar, 3, 1 >, Matrix< Scalar, AmbientDimAtCompileTime, 1, Options >, Matrix< internal::scalar_product_traits< Lhs::Scalar, Rhs::Scalar >::ReturnType, 1, 1 >, Matrix< ComplexScalar, ColsAtCompileTime, 1, Options &(~RowMajor), MaxColsAtCompileTime, 1 >, Matrix< Scalar, 1, Size, Options|RowMajor, 1, MaxSize >, Matrix< Scalar, 1, 1 >, Matrix< Scalar, Dynamic, 1 >, Matrix< Scalar, Dim, 1 >, Matrix< Scalar, DiagSizeAtCompileTime, DiagSizeAtCompileTime, MatrixOptions, MaxDiagSizeAtCompileTime, MaxDiagSizeAtCompileTime >, Matrix< Scalar, SizeMinusOne, 1, Options &~RowMajor, MaxSizeMinusOne, 1 >, Matrix< Scalar, ColsAtCompileTime, RowsAtCompileTime, Options, MaxColsAtCompileTime, MaxRowsAtCompileTime >, Matrix< Scalar, ColsAtCompileTime, 1, Options &~RowMajor, MaxColsAtCompileTime, 1 >, Matrix< ComplexScalar, ColsAtCompileTime, 1, Options &~RowMajor, MaxColsAtCompileTime, 1 >, Matrix< Scalar, 1, RowsAtCompileTime, RowMajor, 1, MaxRowsAtCompileTime >, Matrix< Scalar, Size, Size, ColMajor, MaxColsAtCompileTime, MaxColsAtCompileTime >, Matrix< Scalar, RowsAtCompileTime, RowsAtCompileTime, MatrixOptions, MaxRowsAtCompileTime, MaxRowsAtCompileTime >, Matrix< Scalar, AmbientDimAtCompileTime, 1 >, Matrix< Scalar, ColsAtCompileTime, ColsAtCompileTime, MatrixOptions, MaxColsAtCompileTime, MaxColsAtCompileTime >, Matrix< ComplexScalar, RowsAtCompileTime, ColsAtCompileTime, Options, MaxRowsAtCompileTime, MaxColsAtCompileTime >, and Matrix< Scalar, Index(AmbientDimAtCompileTime)==Dynamic?Dynamic:Index(AmbientDimAtCompileTime)+1, 1, Options >.
Definition at line 480 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE Derived& operator= | ( | const PlainObjectBase< Derived > & | other ) |
This is a special case of the templated operator=.
Its purpose is to prevent a default operator= from hiding the templated operator=.
Definition at line 404 of file PlainObjectBase.h.
void resize | ( | NoChange_t | , |
Index | nbCols | ||
) |
Resizes the matrix, changing only the number of columns.
For the parameter of type NoChange_t, just pass the special value NoChange
as in the example below.
Example:
Output:
- See also:
- resize(Index,Index)
Definition at line 289 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void resize | ( | Index | nbRows, |
Index | nbCols | ||
) |
Resizes *this
to a rows x cols matrix.
This method is intended for dynamic-size matrices, although it is legal to call it on any matrix as long as fixed dimensions are left unchanged. If you only want to change the number of rows and/or of columns, you can use resize(NoChange_t, Index), resize(Index, NoChange_t).
If the current number of coefficients of *this
exactly matches the product rows * cols, then no memory allocation is performed and the current values are left unchanged. In all other cases, including shrinking, the data is reallocated and all previous values are lost.
Example:
Output:
- See also:
- resize(Index) for vectors, resize(NoChange_t, Index), resize(Index, NoChange_t)
Definition at line 235 of file PlainObjectBase.h.
void resize | ( | Index | size ) |
Resizes *this
to a vector of length size.
. This method does not work for partially dynamic matrices when the static dimension is anything other than 1. For example it will not work with Matrix<double, 2, Dynamic>.
Example:
Output:
Definition at line 265 of file PlainObjectBase.h.
void resize | ( | Index | nbRows, |
NoChange_t | |||
) |
Resizes the matrix, changing only the number of rows.
For the parameter of type NoChange_t, just pass the special value NoChange
as in the example below.
Example:
Output:
- See also:
- resize(Index,Index)
Definition at line 302 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void resizeLike | ( | const EigenBase< OtherDerived > & | _other ) |
Resizes *this
to have the same dimensions as other.
Takes care of doing all the checking that's needed.
Note that copying a row-vector into a vector (and conversely) is allowed. The resizing, if any, is then done in the appropriate way so that row-vectors remain row-vectors and vectors remain vectors.
Definition at line 315 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE Derived & setConstant | ( | Index | nbRows, |
Index | nbCols, | ||
const Scalar & | val | ||
) |
Resizes to the given size, and sets all coefficients in this expression to the given value.
- Parameters:
-
nbRows the new number of rows nbCols the new number of columns val the value to which all coefficients are set
Example:
Output:
- See also:
- MatrixBase::setConstant(const Scalar&), setConstant(Index,const Scalar&), class CwiseNullaryOp, MatrixBase::Constant(const Scalar&)
Definition at line 367 of file CwiseNullaryOp.h.
EIGEN_STRONG_INLINE Derived & setConstant | ( | Index | size, |
const Scalar & | val | ||
) |
Resizes to the given size, and sets all coefficients in this expression to the given value.
Example:
Output:
- See also:
- MatrixBase::setConstant(const Scalar&), setConstant(Index,Index,const Scalar&), class CwiseNullaryOp, MatrixBase::Constant(const Scalar&)
Definition at line 348 of file CwiseNullaryOp.h.
EIGEN_STRONG_INLINE Derived & setOnes | ( | Index | nbRows, |
Index | nbCols | ||
) |
Resizes to the given size, and sets all coefficients in this expression to one.
- Parameters:
-
nbRows the new number of rows nbCols the new number of columns
Example:
Output:
- See also:
- MatrixBase::setOnes(), setOnes(Index), class CwiseNullaryOp, MatrixBase::Ones()
Definition at line 659 of file CwiseNullaryOp.h.
EIGEN_STRONG_INLINE Derived & setOnes | ( | Index | newSize ) |
Resizes to the given newSize, and sets all coefficients in this expression to one.
Example:
Output:
- See also:
- MatrixBase::setOnes(), setOnes(Index,Index), class CwiseNullaryOp, MatrixBase::Ones()
Definition at line 641 of file CwiseNullaryOp.h.
EIGEN_STRONG_INLINE Derived & setRandom | ( | Index | newSize ) |
Resizes to the given newSize, and sets all coefficients in this expression to random values.
Example:
Output:
- See also:
- MatrixBase::setRandom(), setRandom(Index,Index), class CwiseNullaryOp, MatrixBase::Random()
EIGEN_STRONG_INLINE Derived & setRandom | ( | Index | nbRows, |
Index | nbCols | ||
) |
Resizes to the given size, and sets all coefficients in this expression to random values.
- Parameters:
-
nbRows the new number of rows nbCols the new number of columns
Example:
Output:
- See also:
- MatrixBase::setRandom(), setRandom(Index), class CwiseNullaryOp, MatrixBase::Random()
EIGEN_STRONG_INLINE Derived & setZero | ( | Index | nbRows, |
Index | nbCols | ||
) |
Resizes to the given size, and sets all coefficients in this expression to zero.
- Parameters:
-
nbRows the new number of rows nbCols the new number of columns
Example:
Output:
- See also:
- DenseBase::setZero(), setZero(Index), class CwiseNullaryOp, DenseBase::Zero()
Definition at line 533 of file CwiseNullaryOp.h.
EIGEN_STRONG_INLINE Derived & setZero | ( | Index | newSize ) |
Resizes to the given size, and sets all coefficients in this expression to zero.
Example:
Output:
- See also:
- DenseBase::setZero(), setZero(Index,Index), class CwiseNullaryOp, DenseBase::Zero()
Definition at line 515 of file CwiseNullaryOp.h.
Generated on Tue Jul 12 2022 17:47:04 by
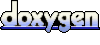