Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Map< Quaternion< _Scalar >, _Options > Class Template Reference
[Geometry module]
Expression of a quaternion from a memory buffer. More...
#include <Quaternion.h>
Inherits QuaternionBase< Map< Quaternion< _Scalar >, _Options > >.
Public Types | |
typedef _Scalar | Scalar |
the scalar type of the coefficients | |
typedef Matrix< Scalar, 3, 1 > | Vector3 |
the type of a 3D vector | |
typedef Matrix< Scalar, 3, 3 > | Matrix3 |
the equivalent rotation matrix type | |
typedef AngleAxis< Scalar > | AngleAxisType |
the equivalent angle-axis type | |
typedef Matrix< Scalar, Dim, Dim > | RotationMatrixType |
corresponding linear transformation matrix type | |
Public Member Functions | |
EIGEN_INHERIT_ASSIGNMENT_OPERATORS(Map) using Base EIGEN_STRONG_INLINE | Map (Scalar *coeffs) |
Constructs a Mapped Quaternion object from the pointer coeffs. | |
Coefficients & | coeffs () |
const Coefficients & | coeffs () const |
Scalar | x () const |
Scalar & | x () |
Scalar | y () const |
Scalar & | y () |
Scalar | z () const |
Scalar & | z () |
Scalar | w () const |
Scalar & | w () |
const VectorBlock< const Coefficients, 3 > | vec () const |
VectorBlock< Coefficients, 3 > | vec () |
QuaternionBase & | setIdentity () |
Scalar | squaredNorm () const |
Scalar | norm () const |
void | normalize () |
Normalizes the quaternion *this . | |
Quaternion< Scalar > | normalized () const |
Scalar | dot (const QuaternionBase< OtherDerived > &other) const |
Matrix3 | toRotationMatrix () const |
Map< Quaternion< _Scalar > , _Options > & | setFromTwoVectors (const MatrixBase< Derived1 > &a, const MatrixBase< Derived2 > &b) |
Transform< Scalar, Dim, Isometry > | operator* (const Translation< Scalar, Dim > &t) const |
RotationMatrixType | operator* (const UniformScaling< Scalar > &s) const |
EIGEN_STRONG_INLINE internal::rotation_base_generic_product_selector < Map< Quaternion< _Scalar > , _Options >, OtherDerived, OtherDerived::IsVectorAtCompileTime > ::ReturnType | operator* (const EigenBase< OtherDerived > &e) const |
Transform< Scalar, Dim, Mode > | operator* (const Transform< Scalar, Dim, Mode, Options > &t) const |
Quaternion< Scalar > | inverse () const |
Quaternion< Scalar > | conjugate () const |
bool | isApprox (const QuaternionBase< OtherDerived > &other, const RealScalar &prec=NumTraits< Scalar >::dummy_precision()) const |
EIGEN_STRONG_INLINE Vector3 | _transformVector (const Vector3 &v) const |
return the result vector of v through the rotation | |
internal::cast_return_type < Map< Quaternion< _Scalar > , _Options >, Quaternion < NewScalarType > >::type | cast () const |
RotationMatrixType | matrix () const |
Static Public Member Functions | |
static Quaternion< Scalar > | Identity () |
Friends | |
RotationMatrixType | operator* (const EigenBase< OtherDerived > &l, const Map< Quaternion< _Scalar >, _Options > &r) |
Transform< Scalar, Dim, Affine > | operator* (const DiagonalMatrix< Scalar, Dim > &l, const Map< Quaternion< _Scalar >, _Options > &r) |
Detailed Description
template<typename _Scalar, int _Options>
class Eigen::Map< Quaternion< _Scalar >, _Options >
Expression of a quaternion from a memory buffer.
- Template Parameters:
-
_Scalar the type of the Quaternion coefficients _Options see class Map
This is a specialization of class Map for Quaternion. This class allows to view a 4 scalar memory buffer as an Eigen's Quaternion object.
- See also:
- class Map, class Quaternion, class QuaternionBase
Definition at line 373 of file Quaternion.h.
Member Typedef Documentation
typedef AngleAxis<Scalar> AngleAxisType [inherited] |
the equivalent angle-axis type
Definition at line 55 of file Quaternion.h.
the equivalent rotation matrix type
Definition at line 53 of file Quaternion.h.
typedef Matrix<Scalar,Dim,Dim> RotationMatrixType [inherited] |
corresponding linear transformation matrix type
Definition at line 37 of file RotationBase.h.
typedef _Scalar Scalar |
the scalar type of the coefficients
Reimplemented from QuaternionBase< Map< Quaternion< _Scalar >, _Options > >.
Definition at line 379 of file Quaternion.h.
the type of a 3D vector
Definition at line 51 of file Quaternion.h.
Constructor & Destructor Documentation
Constructs a Mapped Quaternion object from the pointer coeffs.
The pointer coeffs must reference the four coefficients of Quaternion in the following order:
If the template parameter _Options is set to Aligned, then the pointer coeffs must be aligned.
Definition at line 390 of file Quaternion.h.
Member Function Documentation
return the result vector of v through the rotation
Rotation of a vector by a quaternion.
- Remarks:
- If the quaternion is used to rotate several points (>1) then it is much more efficient to first convert it to a 3x3 Matrix. Comparison of the operation cost for n transformations:
- Quaternion2: 30n
- Via a Matrix3: 24 + 15n
internal::cast_return_type<Map< Quaternion< _Scalar >, _Options > ,Quaternion<NewScalarType> >::type cast | ( | ) | const [inherited] |
- Returns:
*this
with scalar type casted to NewScalarType
Note that if NewScalarType is equal to the current scalar type of *this
then this function smartly returns a const reference to *this
.
Definition at line 172 of file Quaternion.h.
Coefficients& coeffs | ( | ) |
- Returns:
- a vector expression of the coefficients (x,y,z,w)
Reimplemented from QuaternionBase< Map< Quaternion< _Scalar >, _Options > >.
Definition at line 392 of file Quaternion.h.
const Coefficients& coeffs | ( | ) | const |
- Returns:
- a read-only vector expression of the coefficients (x,y,z,w)
Reimplemented from QuaternionBase< Map< Quaternion< _Scalar >, _Options > >.
Definition at line 393 of file Quaternion.h.
Quaternion<Scalar> conjugate | ( | ) | const [inherited] |
- Returns:
- the conjugated quaternion
-
the conjugate of the
*this
which is equal to the multiplicative inverse if the quaternion is normalized. The conjugate of a quaternion represents the opposite rotation.
- See also:
- Quaternion2::inverse()
Scalar dot | ( | const QuaternionBase< OtherDerived > & | other ) | const [inherited] |
- Returns:
- the dot product of
*this
and other Geometrically speaking, the dot product of two unit quaternions corresponds to the cosine of half the angle between the two rotations.
- See also:
- angularDistance()
Definition at line 133 of file Quaternion.h.
static Quaternion<Scalar> Identity | ( | ) | [static, inherited] |
- Returns:
- a quaternion representing an identity rotation
- See also:
- MatrixBase::Identity()
Definition at line 105 of file Quaternion.h.
Quaternion<Scalar> inverse | ( | ) | const [inherited] |
- Returns:
- the quaternion describing the inverse rotation
-
the multiplicative inverse of
*this
Note that in most cases, i.e., if you simply want the opposite rotation, and/or the quaternion is normalized, then it is enough to use the conjugate.
- See also:
- QuaternionBase::conjugate()
Reimplemented from RotationBase< Map< Quaternion< _Scalar >, _Options >, 3 >.
bool isApprox | ( | const QuaternionBase< OtherDerived > & | other, |
const RealScalar & | prec = NumTraits<Scalar>::dummy_precision() |
||
) | const [inherited] |
- Returns:
true
if*this
is approximately equal to other, within the precision determined by prec.
- See also:
- MatrixBase::isApprox()
Definition at line 160 of file Quaternion.h.
RotationMatrixType matrix | ( | ) | const [inherited] |
- Returns:
- an equivalent rotation matrix This function is added to be conform with the Transform class' naming scheme.
Definition at line 50 of file RotationBase.h.
Scalar norm | ( | ) | const [inherited] |
- Returns:
- the norm of the quaternion's coefficients
- See also:
- QuaternionBase::squaredNorm(), MatrixBase::norm()
Definition at line 119 of file Quaternion.h.
void normalize | ( | void | ) | [inherited] |
Normalizes the quaternion *this
.
- See also:
- normalized(), MatrixBase::normalize()
Definition at line 123 of file Quaternion.h.
Quaternion<Scalar> normalized | ( | ) | const [inherited] |
- Returns:
- a normalized copy of
*this
- See also:
- normalize(), MatrixBase::normalized()
Definition at line 126 of file Quaternion.h.
Transform<Scalar,Dim,Mode> operator* | ( | const Transform< Scalar, Dim, Mode, Options > & | t ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a transformation t
Definition at line 89 of file RotationBase.h.
Transform<Scalar,Dim,Isometry> operator* | ( | const Translation< Scalar, Dim > & | t ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a translation t
Definition at line 56 of file RotationBase.h.
RotationMatrixType operator* | ( | const UniformScaling< Scalar > & | s ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a uniform scaling s
Definition at line 60 of file RotationBase.h.
EIGEN_STRONG_INLINE internal::rotation_base_generic_product_selector<Map< Quaternion< _Scalar >, _Options > ,OtherDerived,OtherDerived::IsVectorAtCompileTime>::ReturnType operator* | ( | const EigenBase< OtherDerived > & | e ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a generic expression e e can be:- a DimxDim linear transformation matrix
- a DimxDim diagonal matrix (axis aligned scaling)
- a vector of size Dim
Definition at line 71 of file RotationBase.h.
Map< Quaternion< _Scalar >, _Options > & setFromTwoVectors | ( | const MatrixBase< Derived1 > & | a, |
const MatrixBase< Derived2 > & | b | ||
) | [inherited] |
Sets *this
to be a quaternion representing a rotation between the two arbitrary vectors a and b.
- Returns:
- the quaternion which transform a into b through a rotation
In other words, the built rotation represent a rotation sending the line of direction a to the line of direction b, both lines passing through the origin.
- Returns:
- a reference to
*this
.
Note that the two input vectors do not have to be normalized, and do not need to have the same norm.
QuaternionBase& setIdentity | ( | ) | [inherited] |
- See also:
- QuaternionBase::Identity(), MatrixBase::setIdentity()
Definition at line 109 of file Quaternion.h.
Scalar squaredNorm | ( | ) | const [inherited] |
- Returns:
- the squared norm of the quaternion's coefficients
- See also:
- QuaternionBase::norm(), MatrixBase::squaredNorm()
Definition at line 114 of file Quaternion.h.
Matrix3 toRotationMatrix | ( | void | ) | const [inherited] |
Convert the quaternion to a 3x3 rotation matrix.
- Returns:
- an equivalent 3x3 rotation matrix
The quaternion is required to be normalized, otherwise the result is undefined.
Reimplemented from RotationBase< Map< Quaternion< _Scalar >, _Options >, 3 >.
VectorBlock<Coefficients,3> vec | ( | ) | [inherited] |
- Returns:
- a vector expression of the imaginary part (x,y,z)
Definition at line 81 of file Quaternion.h.
const VectorBlock<const Coefficients,3> vec | ( | ) | const [inherited] |
- Returns:
- a read-only vector expression of the imaginary part (x,y,z)
Definition at line 78 of file Quaternion.h.
Scalar& w | ( | ) | [inherited] |
- Returns:
- a reference to the
w
coefficient
Definition at line 75 of file Quaternion.h.
Scalar w | ( | ) | const [inherited] |
- Returns:
- the
w
coefficient
Definition at line 66 of file Quaternion.h.
Scalar x | ( | ) | const [inherited] |
- Returns:
- the
x
coefficient
Definition at line 60 of file Quaternion.h.
Scalar& x | ( | ) | [inherited] |
- Returns:
- a reference to the
x
coefficient
Definition at line 69 of file Quaternion.h.
Scalar y | ( | ) | const [inherited] |
- Returns:
- the
y
coefficient
Definition at line 62 of file Quaternion.h.
Scalar& y | ( | ) | [inherited] |
- Returns:
- a reference to the
y
coefficient
Definition at line 71 of file Quaternion.h.
Scalar z | ( | ) | const [inherited] |
- Returns:
- the
z
coefficient
Definition at line 64 of file Quaternion.h.
Scalar& z | ( | ) | [inherited] |
- Returns:
- a reference to the
z
coefficient
Definition at line 73 of file Quaternion.h.
Friends And Related Function Documentation
RotationMatrixType operator* | ( | const EigenBase< OtherDerived > & | l, |
const Map< Quaternion< _Scalar >, _Options > & | r | ||
) | [friend, inherited] |
- Returns:
- the concatenation of a linear transformation l with the rotation r
Definition at line 76 of file RotationBase.h.
Transform<Scalar,Dim,Affine> operator* | ( | const DiagonalMatrix< Scalar, Dim > & | l, |
const Map< Quaternion< _Scalar >, _Options > & | r | ||
) | [friend, inherited] |
- Returns:
- the concatenation of a scaling l with the rotation r
Definition at line 80 of file RotationBase.h.
Generated on Tue Jul 12 2022 17:47:05 by
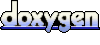