Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
DenseCoeffsBase< Derived, DirectAccessors > Class Template Reference
[Core module]
Base class providing direct read-only coefficient access to matrices and arrays. More...
#include <DenseCoeffsBase.h>
Inherits Eigen::DenseCoeffsBase< Derived, ReadOnlyAccessors >.
Public Member Functions | |
Index | innerStride () const |
Index | outerStride () const |
Index | rowStride () const |
Index | colStride () const |
EIGEN_STRONG_INLINE CoeffReturnType | coeff (Index row, Index col) const |
Short version: don't use this function, use operator()(Index,Index) const instead. | |
EIGEN_STRONG_INLINE CoeffReturnType | coeff (Index index) const |
Short version: don't use this function, use operator[](Index) const instead. | |
EIGEN_STRONG_INLINE CoeffReturnType | operator() (Index row, Index col) const |
EIGEN_STRONG_INLINE CoeffReturnType | operator() (Index index) const |
EIGEN_STRONG_INLINE CoeffReturnType | operator[] (Index index) const |
EIGEN_STRONG_INLINE CoeffReturnType | x () const |
equivalent to operator[](0). | |
EIGEN_STRONG_INLINE CoeffReturnType | y () const |
equivalent to operator[](1). | |
EIGEN_STRONG_INLINE CoeffReturnType | z () const |
equivalent to operator[](2). | |
EIGEN_STRONG_INLINE CoeffReturnType | w () const |
equivalent to operator[](3). | |
Derived & | derived () |
const Derived & | derived () const |
Index | rows () const |
Index | cols () const |
Index | size () const |
Detailed Description
template<typename Derived>
class Eigen::DenseCoeffsBase< Derived, DirectAccessors >
Base class providing direct read-only coefficient access to matrices and arrays.
- Template Parameters:
-
Derived Type of the derived class DirectAccessors Constant indicating direct access
This class defines functions to work with strides which can be used to access entries directly. This class inherits DenseCoeffsBase<Derived, ReadOnlyAccessors> which defines functions to access entries read-only using operator()
.
- See also:
- TopicClassHierarchy
Definition at line 566 of file DenseCoeffsBase.h.
Member Function Documentation
EIGEN_STRONG_INLINE CoeffReturnType coeff | ( | Index | row, |
Index | col | ||
) | const [inherited] |
Short version: don't use this function, use operator()(Index,Index) const instead.
Long version: this function is similar to operator()(Index,Index) const , but without the assertion. Use this for limiting the performance cost of debugging code when doing repeated coefficient access. Only use this when it is guaranteed that the parameters row and col are in range.
If EIGEN_INTERNAL_DEBUGGING is defined, an assertion will be made, making this function equivalent to operator()(Index,Index) const .
- See also:
- operator()(Index,Index) const, coeffRef(Index,Index), coeff(Index) const
Definition at line 94 of file DenseCoeffsBase.h.
EIGEN_STRONG_INLINE CoeffReturnType coeff | ( | Index | index ) | const [inherited] |
Short version: don't use this function, use operator[](Index) const instead.
Long version: this function is similar to operator[](Index) const , but without the assertion. Use this for limiting the performance cost of debugging code when doing repeated coefficient access. Only use this when it is guaranteed that the parameter index is in range.
If EIGEN_INTERNAL_DEBUGGING is defined, an assertion will be made, making this function equivalent to operator[](Index) const .
- See also:
- operator[](Index) const, coeffRef(Index), coeff(Index,Index) const
Definition at line 134 of file DenseCoeffsBase.h.
Index cols | ( | void | ) | const [inherited] |
- Returns:
- the number of columns.
- See also:
- rows(), ColsAtCompileTime
Reimplemented in BandMatrix< _Scalar, Rows, Cols, Supers, Subs, Options >, PermutationBase< Derived >, SelfAdjointView< MatrixType, UpLo >, TriangularView< _MatrixType, _Mode >, HouseholderSequence< VectorsType, CoeffsType, Side >, BandMatrix< RealScalar, ColsAtCompileTime, ColsAtCompileTime, 1, 0 >, BandMatrix< Scalar, Size, Size, Options &SelfAdjoint?0:1, 1, Options|RowMajor >, PermutationBase< PermutationWrapper< _IndicesType > >, PermutationBase< Map< PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType >, _PacketAccess > >, and PermutationBase< PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType > >.
Definition at line 46 of file EigenBase.h.
Index colStride | ( | ) | const |
- Returns:
- the pointer increment between two consecutive columns.
- See also:
- innerStride(), outerStride(), rowStride()
Definition at line 618 of file DenseCoeffsBase.h.
Derived& derived | ( | ) | [inherited] |
- Returns:
- a reference to the derived object
Definition at line 34 of file EigenBase.h.
const Derived& derived | ( | ) | const [inherited] |
- Returns:
- a const reference to the derived object
Definition at line 36 of file EigenBase.h.
Index innerStride | ( | ) | const |
- Returns:
- the pointer increment between two consecutive elements within a slice in the inner direction.
- See also:
- outerStride(), rowStride(), colStride()
Definition at line 584 of file DenseCoeffsBase.h.
EIGEN_STRONG_INLINE CoeffReturnType operator() | ( | Index | row, |
Index | col | ||
) | const [inherited] |
- Returns:
- the coefficient at given the given row and column.
- See also:
- operator()(Index,Index), operator[](Index)
Definition at line 111 of file DenseCoeffsBase.h.
EIGEN_STRONG_INLINE CoeffReturnType operator() | ( | Index | index ) | const [inherited] |
- Returns:
- the coefficient at given index.
This is synonymous to operator[](Index) const.
This method is allowed only for vector expressions, and for matrix expressions having the LinearAccessBit.
- See also:
- operator[](Index), operator()(Index,Index) const, x() const, y() const, z() const, w() const
Definition at line 171 of file DenseCoeffsBase.h.
EIGEN_STRONG_INLINE CoeffReturnType operator[] | ( | Index | index ) | const [inherited] |
- Returns:
- the coefficient at given index.
This method is allowed only for vector expressions, and for matrix expressions having the LinearAccessBit.
- See also:
- operator[](Index), operator()(Index,Index) const, x() const, y() const, z() const, w() const
Definition at line 150 of file DenseCoeffsBase.h.
Index outerStride | ( | ) | const |
- Returns:
- the pointer increment between two consecutive inner slices (for example, between two consecutive columns in a column-major matrix).
- See also:
- innerStride(), rowStride(), colStride()
Definition at line 594 of file DenseCoeffsBase.h.
Index rows | ( | void | ) | const [inherited] |
- Returns:
- the number of rows.
- See also:
- cols(), RowsAtCompileTime
Reimplemented in BandMatrix< _Scalar, Rows, Cols, Supers, Subs, Options >, PermutationBase< Derived >, SelfAdjointView< MatrixType, UpLo >, TriangularView< _MatrixType, _Mode >, HouseholderSequence< VectorsType, CoeffsType, Side >, BandMatrix< RealScalar, ColsAtCompileTime, ColsAtCompileTime, 1, 0 >, BandMatrix< Scalar, Size, Size, Options &SelfAdjoint?0:1, 1, Options|RowMajor >, PermutationBase< PermutationWrapper< _IndicesType > >, PermutationBase< Map< PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType >, _PacketAccess > >, and PermutationBase< PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType > >.
Definition at line 44 of file EigenBase.h.
Index rowStride | ( | ) | const |
- Returns:
- the pointer increment between two consecutive rows.
- See also:
- innerStride(), outerStride(), colStride()
Definition at line 609 of file DenseCoeffsBase.h.
Index size | ( | ) | const [inherited] |
- Returns:
- the number of coefficients, which is rows()*cols().
Reimplemented in PermutationBase< Derived >, PermutationBase< PermutationWrapper< _IndicesType > >, PermutationBase< Map< PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType >, _PacketAccess > >, and PermutationBase< PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType > >.
Definition at line 49 of file EigenBase.h.
EIGEN_STRONG_INLINE CoeffReturnType w | ( | ) | const [inherited] |
equivalent to operator[](3).
Definition at line 195 of file DenseCoeffsBase.h.
EIGEN_STRONG_INLINE CoeffReturnType x | ( | ) | const [inherited] |
equivalent to operator[](0).
Definition at line 180 of file DenseCoeffsBase.h.
EIGEN_STRONG_INLINE CoeffReturnType y | ( | ) | const [inherited] |
equivalent to operator[](1).
Definition at line 185 of file DenseCoeffsBase.h.
EIGEN_STRONG_INLINE CoeffReturnType z | ( | ) | const [inherited] |
equivalent to operator[](2).
Definition at line 190 of file DenseCoeffsBase.h.
Generated on Tue Jul 12 2022 17:47:04 by
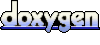