Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Quaternion< _Scalar, _Options > Class Template Reference
[Geometry module]
#include <Quaternion.h>
Inherits QuaternionBase< Quaternion< _Scalar, _Options > >.
Public Types | |
typedef _Scalar | Scalar |
the scalar type of the coefficients | |
typedef Base::AngleAxisType | AngleAxisType |
the equivalent angle-axis type | |
typedef Matrix< Scalar, 3, 1 > | Vector3 |
the type of a 3D vector | |
typedef Matrix< Scalar, 3, 3 > | Matrix3 |
the equivalent rotation matrix type | |
typedef Matrix< Scalar, Dim, Dim > | RotationMatrixType |
corresponding linear transformation matrix type | |
Public Member Functions | |
Quaternion () | |
Default constructor leaving the quaternion uninitialized. | |
Quaternion (const Scalar &w, const Scalar &x, const Scalar &y, const Scalar &z) | |
Constructs and initializes the quaternion ![]() | |
Quaternion (const Scalar *data) | |
Constructs and initialize a quaternion from the array data. | |
template<class Derived > | |
EIGEN_STRONG_INLINE | Quaternion (const QuaternionBase< Derived > &other) |
Copy constructor. | |
Quaternion (const AngleAxisType &aa) | |
Constructs and initializes a quaternion from the angle-axis aa. | |
template<typename Derived > | |
Quaternion (const MatrixBase< Derived > &other) | |
Constructs and initializes a quaternion from either:
| |
template<typename OtherScalar , int OtherOptions> | |
Quaternion (const Quaternion< OtherScalar, OtherOptions > &other) | |
Explicit copy constructor with scalar conversion. | |
Coefficients & | coeffs () |
const Coefficients & | coeffs () const |
Scalar | x () const |
Scalar & | x () |
Scalar | y () const |
Scalar & | y () |
Scalar | z () const |
Scalar & | z () |
Scalar | w () const |
Scalar & | w () |
const VectorBlock< const Coefficients, 3 > | vec () const |
VectorBlock< Coefficients, 3 > | vec () |
QuaternionBase & | setIdentity () |
Scalar | squaredNorm () const |
Scalar | norm () const |
void | normalize () |
Normalizes the quaternion *this . | |
Quaternion< Scalar > | normalized () const |
Scalar | dot (const QuaternionBase< OtherDerived > &other) const |
Matrix3 | toRotationMatrix () const |
Quaternion< _Scalar, _Options > & | setFromTwoVectors (const MatrixBase< Derived1 > &a, const MatrixBase< Derived2 > &b) |
Transform< Scalar, Dim, Isometry > | operator* (const Translation< Scalar, Dim > &t) const |
RotationMatrixType | operator* (const UniformScaling< Scalar > &s) const |
EIGEN_STRONG_INLINE internal::rotation_base_generic_product_selector < Quaternion< _Scalar, _Options >, OtherDerived, OtherDerived::IsVectorAtCompileTime > ::ReturnType | operator* (const EigenBase< OtherDerived > &e) const |
Transform< Scalar, Dim, Mode > | operator* (const Transform< Scalar, Dim, Mode, Options > &t) const |
Quaternion< Scalar > | inverse () const |
Quaternion< Scalar > | conjugate () const |
bool | isApprox (const QuaternionBase< OtherDerived > &other, const RealScalar &prec=NumTraits< Scalar >::dummy_precision()) const |
EIGEN_STRONG_INLINE Vector3 | _transformVector (const Vector3 &v) const |
return the result vector of v through the rotation | |
internal::cast_return_type < Quaternion< _Scalar, _Options >, Quaternion < NewScalarType > >::type | cast () const |
RotationMatrixType | matrix () const |
Static Public Member Functions | |
template<typename Derived1 , typename Derived2 > | |
static Quaternion | FromTwoVectors (const MatrixBase< Derived1 > &a, const MatrixBase< Derived2 > &b) |
Returns a quaternion representing a rotation between the two arbitrary vectors a and b. | |
static Quaternion< Scalar > | Identity () |
Friends | |
RotationMatrixType | operator* (const EigenBase< OtherDerived > &l, const Quaternion< _Scalar, _Options > &r) |
Transform< Scalar, Dim, Affine > | operator* (const DiagonalMatrix< Scalar, Dim > &l, const Quaternion< _Scalar, _Options > &r) |
Detailed Description
template<typename _Scalar, int _Options>
class Eigen::Quaternion< _Scalar, _Options >
The quaternion class used to represent 3D orientations and rotations
- Template Parameters:
-
_Scalar the scalar type, i.e., the type of the coefficients _Options controls the memory alignment of the coefficients. Can be # AutoAlign or # DontAlign. Default is AutoAlign.
This class represents a quaternion that is a convenient representation of orientations and rotations of objects in three dimensions. Compared to other representations like Euler angles or 3x3 matrices, quaternions offer the following advantages:
- compact storage (4 scalars)
- efficient to compose (28 flops),
- stable spherical interpolation
The following two typedefs are provided for convenience:
Quaternionf
forfloat
Quaterniond
fordouble
- Warning:
- Operations interpreting the quaternion as rotation have undefined behavior if the quaternion is not normalized.
Definition at line 226 of file Quaternion.h.
Member Typedef Documentation
typedef Base::AngleAxisType AngleAxisType |
the equivalent angle-axis type
Reimplemented from QuaternionBase< Quaternion< _Scalar, _Options > >.
Definition at line 238 of file Quaternion.h.
the equivalent rotation matrix type
Definition at line 53 of file Quaternion.h.
typedef Matrix<Scalar,Dim,Dim> RotationMatrixType [inherited] |
corresponding linear transformation matrix type
Definition at line 37 of file RotationBase.h.
typedef _Scalar Scalar |
the scalar type of the coefficients
Reimplemented from QuaternionBase< Quaternion< _Scalar, _Options > >.
Definition at line 232 of file Quaternion.h.
the type of a 3D vector
Definition at line 51 of file Quaternion.h.
Constructor & Destructor Documentation
Quaternion | ( | ) |
Default constructor leaving the quaternion uninitialized.
Definition at line 241 of file Quaternion.h.
Quaternion | ( | const Scalar & | w, |
const Scalar & | x, | ||
const Scalar & | y, | ||
const Scalar & | z | ||
) |
Constructs and initializes the quaternion from its four coefficients w, x, y and z.
- Warning:
- Note the order of the arguments: the real w coefficient first, while internally the coefficients are stored in the following order: [
x
,y
,z
,w
]
Definition at line 250 of file Quaternion.h.
Quaternion | ( | const Scalar * | data ) |
Constructs and initialize a quaternion from the array data.
Definition at line 253 of file Quaternion.h.
EIGEN_STRONG_INLINE Quaternion | ( | const QuaternionBase< Derived > & | other ) |
Copy constructor.
Definition at line 256 of file Quaternion.h.
Quaternion | ( | const AngleAxisType & | aa ) | [explicit] |
Constructs and initializes a quaternion from the angle-axis aa.
Definition at line 259 of file Quaternion.h.
Quaternion | ( | const MatrixBase< Derived > & | other ) | [explicit] |
Constructs and initializes a quaternion from either:
- a rotation matrix expression,
- a 4D vector expression representing quaternion coefficients.
Definition at line 266 of file Quaternion.h.
Quaternion | ( | const Quaternion< OtherScalar, OtherOptions > & | other ) | [explicit] |
Explicit copy constructor with scalar conversion.
Definition at line 270 of file Quaternion.h.
Member Function Documentation
return the result vector of v through the rotation
Rotation of a vector by a quaternion.
- Remarks:
- If the quaternion is used to rotate several points (>1) then it is much more efficient to first convert it to a 3x3 Matrix. Comparison of the operation cost for n transformations:
- Quaternion2: 30n
- Via a Matrix3: 24 + 15n
internal::cast_return_type<Quaternion< _Scalar, _Options > ,Quaternion<NewScalarType> >::type cast | ( | ) | const [inherited] |
- Returns:
*this
with scalar type casted to NewScalarType
Note that if NewScalarType is equal to the current scalar type of *this
then this function smartly returns a const reference to *this
.
Definition at line 172 of file Quaternion.h.
Coefficients& coeffs | ( | ) |
- Returns:
- a vector expression of the coefficients (x,y,z,w)
Reimplemented from QuaternionBase< Quaternion< _Scalar, _Options > >.
Definition at line 276 of file Quaternion.h.
const Coefficients& coeffs | ( | ) | const |
- Returns:
- a read-only vector expression of the coefficients (x,y,z,w)
Reimplemented from QuaternionBase< Quaternion< _Scalar, _Options > >.
Definition at line 277 of file Quaternion.h.
Quaternion<Scalar> conjugate | ( | ) | const [inherited] |
- Returns:
- the conjugated quaternion
-
the conjugate of the
*this
which is equal to the multiplicative inverse if the quaternion is normalized. The conjugate of a quaternion represents the opposite rotation.
- See also:
- Quaternion2::inverse()
Scalar dot | ( | const QuaternionBase< OtherDerived > & | other ) | const [inherited] |
- Returns:
- the dot product of
*this
and other Geometrically speaking, the dot product of two unit quaternions corresponds to the cosine of half the angle between the two rotations.
- See also:
- angularDistance()
Definition at line 133 of file Quaternion.h.
Quaternion< Scalar, Options > FromTwoVectors | ( | const MatrixBase< Derived1 > & | a, |
const MatrixBase< Derived2 > & | b | ||
) | [static] |
Returns a quaternion representing a rotation between the two arbitrary vectors a and b.
In other words, the built rotation represent a rotation sending the line of direction a to the line of direction b, both lines passing through the origin.
- Returns:
- resulting quaternion
Note that the two input vectors do not have to be normalized, and do not need to have the same norm.
Definition at line 621 of file Quaternion.h.
static Quaternion<Scalar> Identity | ( | ) | [static, inherited] |
- Returns:
- a quaternion representing an identity rotation
- See also:
- MatrixBase::Identity()
Definition at line 105 of file Quaternion.h.
Quaternion<Scalar> inverse | ( | ) | const [inherited] |
- Returns:
- the quaternion describing the inverse rotation
-
the multiplicative inverse of
*this
Note that in most cases, i.e., if you simply want the opposite rotation, and/or the quaternion is normalized, then it is enough to use the conjugate.
- See also:
- QuaternionBase::conjugate()
Reimplemented from RotationBase< Quaternion< _Scalar, _Options >, 3 >.
bool isApprox | ( | const QuaternionBase< OtherDerived > & | other, |
const RealScalar & | prec = NumTraits<Scalar>::dummy_precision() |
||
) | const [inherited] |
- Returns:
true
if*this
is approximately equal to other, within the precision determined by prec.
- See also:
- MatrixBase::isApprox()
Definition at line 160 of file Quaternion.h.
RotationMatrixType matrix | ( | ) | const [inherited] |
- Returns:
- an equivalent rotation matrix This function is added to be conform with the Transform class' naming scheme.
Definition at line 50 of file RotationBase.h.
Scalar norm | ( | ) | const [inherited] |
- Returns:
- the norm of the quaternion's coefficients
- See also:
- QuaternionBase::squaredNorm(), MatrixBase::norm()
Definition at line 119 of file Quaternion.h.
void normalize | ( | void | ) | [inherited] |
Normalizes the quaternion *this
.
- See also:
- normalized(), MatrixBase::normalize()
Definition at line 123 of file Quaternion.h.
Quaternion<Scalar> normalized | ( | ) | const [inherited] |
- Returns:
- a normalized copy of
*this
- See also:
- normalize(), MatrixBase::normalized()
Definition at line 126 of file Quaternion.h.
RotationMatrixType operator* | ( | const UniformScaling< Scalar > & | s ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a uniform scaling s
Definition at line 60 of file RotationBase.h.
Transform<Scalar,Dim,Isometry> operator* | ( | const Translation< Scalar, Dim > & | t ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a translation t
Definition at line 56 of file RotationBase.h.
EIGEN_STRONG_INLINE internal::rotation_base_generic_product_selector<Quaternion< _Scalar, _Options > ,OtherDerived,OtherDerived::IsVectorAtCompileTime>::ReturnType operator* | ( | const EigenBase< OtherDerived > & | e ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a generic expression e e can be:- a DimxDim linear transformation matrix
- a DimxDim diagonal matrix (axis aligned scaling)
- a vector of size Dim
Definition at line 71 of file RotationBase.h.
Transform<Scalar,Dim,Mode> operator* | ( | const Transform< Scalar, Dim, Mode, Options > & | t ) | const [inherited] |
- Returns:
- the concatenation of the rotation
*this
with a transformation t
Definition at line 89 of file RotationBase.h.
Quaternion< _Scalar, _Options > & setFromTwoVectors | ( | const MatrixBase< Derived1 > & | a, |
const MatrixBase< Derived2 > & | b | ||
) | [inherited] |
Sets *this
to be a quaternion representing a rotation between the two arbitrary vectors a and b.
- Returns:
- the quaternion which transform a into b through a rotation
In other words, the built rotation represent a rotation sending the line of direction a to the line of direction b, both lines passing through the origin.
- Returns:
- a reference to
*this
.
Note that the two input vectors do not have to be normalized, and do not need to have the same norm.
QuaternionBase& setIdentity | ( | ) | [inherited] |
- See also:
- QuaternionBase::Identity(), MatrixBase::setIdentity()
Definition at line 109 of file Quaternion.h.
Scalar squaredNorm | ( | ) | const [inherited] |
- Returns:
- the squared norm of the quaternion's coefficients
- See also:
- QuaternionBase::norm(), MatrixBase::squaredNorm()
Definition at line 114 of file Quaternion.h.
Matrix3 toRotationMatrix | ( | void | ) | const [inherited] |
Convert the quaternion to a 3x3 rotation matrix.
- Returns:
- an equivalent 3x3 rotation matrix
The quaternion is required to be normalized, otherwise the result is undefined.
Reimplemented from RotationBase< Quaternion< _Scalar, _Options >, 3 >.
const VectorBlock<const Coefficients,3> vec | ( | ) | const [inherited] |
- Returns:
- a read-only vector expression of the imaginary part (x,y,z)
Definition at line 78 of file Quaternion.h.
VectorBlock<Coefficients,3> vec | ( | ) | [inherited] |
- Returns:
- a vector expression of the imaginary part (x,y,z)
Definition at line 81 of file Quaternion.h.
Scalar& w | ( | ) | [inherited] |
- Returns:
- a reference to the
w
coefficient
Definition at line 75 of file Quaternion.h.
Scalar w | ( | ) | const [inherited] |
- Returns:
- the
w
coefficient
Definition at line 66 of file Quaternion.h.
Scalar x | ( | ) | const [inherited] |
- Returns:
- the
x
coefficient
Definition at line 60 of file Quaternion.h.
Scalar& x | ( | ) | [inherited] |
- Returns:
- a reference to the
x
coefficient
Definition at line 69 of file Quaternion.h.
Scalar& y | ( | ) | [inherited] |
- Returns:
- a reference to the
y
coefficient
Definition at line 71 of file Quaternion.h.
Scalar y | ( | ) | const [inherited] |
- Returns:
- the
y
coefficient
Definition at line 62 of file Quaternion.h.
Scalar& z | ( | ) | [inherited] |
- Returns:
- a reference to the
z
coefficient
Definition at line 73 of file Quaternion.h.
Scalar z | ( | ) | const [inherited] |
- Returns:
- the
z
coefficient
Definition at line 64 of file Quaternion.h.
Friends And Related Function Documentation
RotationMatrixType operator* | ( | const EigenBase< OtherDerived > & | l, |
const Quaternion< _Scalar, _Options > & | r | ||
) | [friend, inherited] |
- Returns:
- the concatenation of a linear transformation l with the rotation r
Definition at line 76 of file RotationBase.h.
Transform<Scalar,Dim,Affine> operator* | ( | const DiagonalMatrix< Scalar, Dim > & | l, |
const Quaternion< _Scalar, _Options > & | r | ||
) | [friend, inherited] |
- Returns:
- the concatenation of a scaling l with the rotation r
Definition at line 80 of file RotationBase.h.
Generated on Tue Jul 12 2022 17:47:05 by
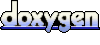