Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Constants.h
00001 // This file is part of Eigen, a lightweight C++ template library 00002 // for linear algebra. 00003 // 00004 // Copyright (C) 2008-2009 Gael Guennebaud <gael.guennebaud@inria.fr> 00005 // Copyright (C) 2007-2009 Benoit Jacob <jacob.benoit.1@gmail.com> 00006 // 00007 // This Source Code Form is subject to the terms of the Mozilla 00008 // Public License v. 2.0. If a copy of the MPL was not distributed 00009 // with this file, You can obtain one at http://mozilla.org/MPL/2.0/. 00010 00011 #ifndef EIGEN_CONSTANTS_H 00012 #define EIGEN_CONSTANTS_H 00013 00014 namespace Eigen { 00015 00016 /** This value means that a positive quantity (e.g., a size) is not known at compile-time, and that instead the value is 00017 * stored in some runtime variable. 00018 * 00019 * Changing the value of Dynamic breaks the ABI, as Dynamic is often used as a template parameter for Matrix. 00020 */ 00021 const int Dynamic = -1; 00022 00023 /** This value means that a signed quantity (e.g., a signed index) is not known at compile-time, and that instead its value 00024 * has to be specified at runtime. 00025 */ 00026 const int DynamicIndex = 0xffffff; 00027 00028 /** This value means +Infinity; it is currently used only as the p parameter to MatrixBase::lpNorm<int>(). 00029 * The value Infinity there means the L-infinity norm. 00030 */ 00031 const int Infinity = -1; 00032 00033 /** \defgroup flags Flags 00034 * \ingroup Core_Module 00035 * 00036 * These are the possible bits which can be OR'ed to constitute the flags of a matrix or 00037 * expression. 00038 * 00039 * It is important to note that these flags are a purely compile-time notion. They are a compile-time property of 00040 * an expression type, implemented as enum's. They are not stored in memory at runtime, and they do not incur any 00041 * runtime overhead. 00042 * 00043 * \sa MatrixBase::Flags 00044 */ 00045 00046 /** \ingroup flags 00047 * 00048 * for a matrix, this means that the storage order is row-major. 00049 * If this bit is not set, the storage order is column-major. 00050 * For an expression, this determines the storage order of 00051 * the matrix created by evaluation of that expression. 00052 * \sa \ref TopicStorageOrders */ 00053 const unsigned int RowMajorBit = 0x1; 00054 00055 /** \ingroup flags 00056 * 00057 * means the expression should be evaluated by the calling expression */ 00058 const unsigned int EvalBeforeNestingBit = 0x2; 00059 00060 /** \ingroup flags 00061 * 00062 * means the expression should be evaluated before any assignment */ 00063 const unsigned int EvalBeforeAssigningBit = 0x4; 00064 00065 /** \ingroup flags 00066 * 00067 * Short version: means the expression might be vectorized 00068 * 00069 * Long version: means that the coefficients can be handled by packets 00070 * and start at a memory location whose alignment meets the requirements 00071 * of the present CPU architecture for optimized packet access. In the fixed-size 00072 * case, there is the additional condition that it be possible to access all the 00073 * coefficients by packets (this implies the requirement that the size be a multiple of 16 bytes, 00074 * and that any nontrivial strides don't break the alignment). In the dynamic-size case, 00075 * there is no such condition on the total size and strides, so it might not be possible to access 00076 * all coeffs by packets. 00077 * 00078 * \note This bit can be set regardless of whether vectorization is actually enabled. 00079 * To check for actual vectorizability, see \a ActualPacketAccessBit. 00080 */ 00081 const unsigned int PacketAccessBit = 0x8; 00082 00083 #ifdef EIGEN_VECTORIZE 00084 /** \ingroup flags 00085 * 00086 * If vectorization is enabled (EIGEN_VECTORIZE is defined) this constant 00087 * is set to the value \a PacketAccessBit. 00088 * 00089 * If vectorization is not enabled (EIGEN_VECTORIZE is not defined) this constant 00090 * is set to the value 0. 00091 */ 00092 const unsigned int ActualPacketAccessBit = PacketAccessBit; 00093 #else 00094 const unsigned int ActualPacketAccessBit = 0x0; 00095 #endif 00096 00097 /** \ingroup flags 00098 * 00099 * Short version: means the expression can be seen as 1D vector. 00100 * 00101 * Long version: means that one can access the coefficients 00102 * of this expression by coeff(int), and coeffRef(int) in the case of a lvalue expression. These 00103 * index-based access methods are guaranteed 00104 * to not have to do any runtime computation of a (row, col)-pair from the index, so that it 00105 * is guaranteed that whenever it is available, index-based access is at least as fast as 00106 * (row,col)-based access. Expressions for which that isn't possible don't have the LinearAccessBit. 00107 * 00108 * If both PacketAccessBit and LinearAccessBit are set, then the 00109 * packets of this expression can be accessed by packet(int), and writePacket(int) in the case of a 00110 * lvalue expression. 00111 * 00112 * Typically, all vector expressions have the LinearAccessBit, but there is one exception: 00113 * Product expressions don't have it, because it would be troublesome for vectorization, even when the 00114 * Product is a vector expression. Thus, vector Product expressions allow index-based coefficient access but 00115 * not index-based packet access, so they don't have the LinearAccessBit. 00116 */ 00117 const unsigned int LinearAccessBit = 0x10; 00118 00119 /** \ingroup flags 00120 * 00121 * Means the expression has a coeffRef() method, i.e. is writable as its individual coefficients are directly addressable. 00122 * This rules out read-only expressions. 00123 * 00124 * Note that DirectAccessBit and LvalueBit are mutually orthogonal, as there are examples of expression having one but note 00125 * the other: 00126 * \li writable expressions that don't have a very simple memory layout as a strided array, have LvalueBit but not DirectAccessBit 00127 * \li Map-to-const expressions, for example Map<const Matrix>, have DirectAccessBit but not LvalueBit 00128 * 00129 * Expressions having LvalueBit also have their coeff() method returning a const reference instead of returning a new value. 00130 */ 00131 const unsigned int LvalueBit = 0x20; 00132 00133 /** \ingroup flags 00134 * 00135 * Means that the underlying array of coefficients can be directly accessed as a plain strided array. The memory layout 00136 * of the array of coefficients must be exactly the natural one suggested by rows(), cols(), 00137 * outerStride(), innerStride(), and the RowMajorBit. This rules out expressions such as Diagonal, whose coefficients, 00138 * though referencable, do not have such a regular memory layout. 00139 * 00140 * See the comment on LvalueBit for an explanation of how LvalueBit and DirectAccessBit are mutually orthogonal. 00141 */ 00142 const unsigned int DirectAccessBit = 0x40; 00143 00144 /** \ingroup flags 00145 * 00146 * means the first coefficient packet is guaranteed to be aligned */ 00147 const unsigned int AlignedBit = 0x80; 00148 00149 const unsigned int NestByRefBit = 0x100; 00150 00151 // list of flags that are inherited by default 00152 const unsigned int HereditaryBits = RowMajorBit 00153 | EvalBeforeNestingBit 00154 | EvalBeforeAssigningBit; 00155 00156 /** \defgroup enums Enumerations 00157 * \ingroup Core_Module 00158 * 00159 * Various enumerations used in %Eigen. Many of these are used as template parameters. 00160 */ 00161 00162 /** \ingroup enums 00163 * Enum containing possible values for the \p Mode parameter of 00164 * MatrixBase::selfadjointView() and MatrixBase::triangularView(). */ 00165 enum { 00166 /** View matrix as a lower triangular matrix. */ 00167 Lower=0x1, 00168 /** View matrix as an upper triangular matrix. */ 00169 Upper=0x2, 00170 /** %Matrix has ones on the diagonal; to be used in combination with #Lower or #Upper. */ 00171 UnitDiag=0x4, 00172 /** %Matrix has zeros on the diagonal; to be used in combination with #Lower or #Upper. */ 00173 ZeroDiag=0x8, 00174 /** View matrix as a lower triangular matrix with ones on the diagonal. */ 00175 UnitLower=UnitDiag|Lower, 00176 /** View matrix as an upper triangular matrix with ones on the diagonal. */ 00177 UnitUpper=UnitDiag|Upper, 00178 /** View matrix as a lower triangular matrix with zeros on the diagonal. */ 00179 StrictlyLower=ZeroDiag|Lower, 00180 /** View matrix as an upper triangular matrix with zeros on the diagonal. */ 00181 StrictlyUpper=ZeroDiag|Upper, 00182 /** Used in BandMatrix and SelfAdjointView to indicate that the matrix is self-adjoint. */ 00183 SelfAdjoint=0x10, 00184 /** Used to support symmetric, non-selfadjoint, complex matrices. */ 00185 Symmetric=0x20 00186 }; 00187 00188 /** \ingroup enums 00189 * Enum for indicating whether an object is aligned or not. */ 00190 enum { 00191 /** Object is not correctly aligned for vectorization. */ 00192 Unaligned=0, 00193 /** Object is aligned for vectorization. */ 00194 Aligned=1 00195 }; 00196 00197 /** \ingroup enums 00198 * Enum used by DenseBase::corner() in Eigen2 compatibility mode. */ 00199 // FIXME after the corner() API change, this was not needed anymore, except by AlignedBox 00200 // TODO: find out what to do with that. Adapt the AlignedBox API ? 00201 enum CornerType { TopLeft, TopRight, BottomLeft, BottomRight }; 00202 00203 /** \ingroup enums 00204 * Enum containing possible values for the \p Direction parameter of 00205 * Reverse, PartialReduxExpr and VectorwiseOp. */ 00206 enum DirectionType { 00207 /** For Reverse, all columns are reversed; 00208 * for PartialReduxExpr and VectorwiseOp, act on columns. */ 00209 Vertical, 00210 /** For Reverse, all rows are reversed; 00211 * for PartialReduxExpr and VectorwiseOp, act on rows. */ 00212 Horizontal, 00213 /** For Reverse, both rows and columns are reversed; 00214 * not used for PartialReduxExpr and VectorwiseOp. */ 00215 BothDirections 00216 }; 00217 00218 /** \internal \ingroup enums 00219 * Enum to specify how to traverse the entries of a matrix. */ 00220 enum { 00221 /** \internal Default traversal, no vectorization, no index-based access */ 00222 DefaultTraversal, 00223 /** \internal No vectorization, use index-based access to have only one for loop instead of 2 nested loops */ 00224 LinearTraversal, 00225 /** \internal Equivalent to a slice vectorization for fixed-size matrices having good alignment 00226 * and good size */ 00227 InnerVectorizedTraversal, 00228 /** \internal Vectorization path using a single loop plus scalar loops for the 00229 * unaligned boundaries */ 00230 LinearVectorizedTraversal, 00231 /** \internal Generic vectorization path using one vectorized loop per row/column with some 00232 * scalar loops to handle the unaligned boundaries */ 00233 SliceVectorizedTraversal, 00234 /** \internal Special case to properly handle incompatible scalar types or other defecting cases*/ 00235 InvalidTraversal, 00236 /** \internal Evaluate all entries at once */ 00237 AllAtOnceTraversal 00238 }; 00239 00240 /** \internal \ingroup enums 00241 * Enum to specify whether to unroll loops when traversing over the entries of a matrix. */ 00242 enum { 00243 /** \internal Do not unroll loops. */ 00244 NoUnrolling, 00245 /** \internal Unroll only the inner loop, but not the outer loop. */ 00246 InnerUnrolling, 00247 /** \internal Unroll both the inner and the outer loop. If there is only one loop, 00248 * because linear traversal is used, then unroll that loop. */ 00249 CompleteUnrolling 00250 }; 00251 00252 /** \internal \ingroup enums 00253 * Enum to specify whether to use the default (built-in) implementation or the specialization. */ 00254 enum { 00255 Specialized, 00256 BuiltIn 00257 }; 00258 00259 /** \ingroup enums 00260 * Enum containing possible values for the \p _Options template parameter of 00261 * Matrix, Array and BandMatrix. */ 00262 enum { 00263 /** Storage order is column major (see \ref TopicStorageOrders). */ 00264 ColMajor = 0, 00265 /** Storage order is row major (see \ref TopicStorageOrders). */ 00266 RowMajor = 0x1, // it is only a coincidence that this is equal to RowMajorBit -- don't rely on that 00267 /** Align the matrix itself if it is vectorizable fixed-size */ 00268 AutoAlign = 0, 00269 /** Don't require alignment for the matrix itself (the array of coefficients, if dynamically allocated, may still be requested to be aligned) */ // FIXME --- clarify the situation 00270 DontAlign = 0x2 00271 }; 00272 00273 /** \ingroup enums 00274 * Enum for specifying whether to apply or solve on the left or right. */ 00275 enum { 00276 /** Apply transformation on the left. */ 00277 OnTheLeft = 1, 00278 /** Apply transformation on the right. */ 00279 OnTheRight = 2 00280 }; 00281 00282 /* the following used to be written as: 00283 * 00284 * struct NoChange_t {}; 00285 * namespace { 00286 * EIGEN_UNUSED NoChange_t NoChange; 00287 * } 00288 * 00289 * on the ground that it feels dangerous to disambiguate overloaded functions on enum/integer types. 00290 * However, this leads to "variable declared but never referenced" warnings on Intel Composer XE, 00291 * and we do not know how to get rid of them (bug 450). 00292 */ 00293 00294 enum NoChange_t { NoChange }; 00295 enum Sequential_t { Sequential }; 00296 enum Default_t { Default }; 00297 00298 /** \internal \ingroup enums 00299 * Used in AmbiVector. */ 00300 enum { 00301 IsDense = 0, 00302 IsSparse 00303 }; 00304 00305 /** \ingroup enums 00306 * Used as template parameter in DenseCoeffBase and MapBase to indicate 00307 * which accessors should be provided. */ 00308 enum AccessorLevels { 00309 /** Read-only access via a member function. */ 00310 ReadOnlyAccessors, 00311 /** Read/write access via member functions. */ 00312 WriteAccessors, 00313 /** Direct read-only access to the coefficients. */ 00314 DirectAccessors, 00315 /** Direct read/write access to the coefficients. */ 00316 DirectWriteAccessors 00317 }; 00318 00319 /** \ingroup enums 00320 * Enum with options to give to various decompositions. */ 00321 enum DecompositionOptions { 00322 /** \internal Not used (meant for LDLT?). */ 00323 Pivoting = 0x01, 00324 /** \internal Not used (meant for LDLT?). */ 00325 NoPivoting = 0x02, 00326 /** Used in JacobiSVD to indicate that the square matrix U is to be computed. */ 00327 ComputeFullU = 0x04, 00328 /** Used in JacobiSVD to indicate that the thin matrix U is to be computed. */ 00329 ComputeThinU = 0x08, 00330 /** Used in JacobiSVD to indicate that the square matrix V is to be computed. */ 00331 ComputeFullV = 0x10, 00332 /** Used in JacobiSVD to indicate that the thin matrix V is to be computed. */ 00333 ComputeThinV = 0x20, 00334 /** Used in SelfAdjointEigenSolver and GeneralizedSelfAdjointEigenSolver to specify 00335 * that only the eigenvalues are to be computed and not the eigenvectors. */ 00336 EigenvaluesOnly = 0x40, 00337 /** Used in SelfAdjointEigenSolver and GeneralizedSelfAdjointEigenSolver to specify 00338 * that both the eigenvalues and the eigenvectors are to be computed. */ 00339 ComputeEigenvectors = 0x80, 00340 /** \internal */ 00341 EigVecMask = EigenvaluesOnly | ComputeEigenvectors, 00342 /** Used in GeneralizedSelfAdjointEigenSolver to indicate that it should 00343 * solve the generalized eigenproblem \f$ Ax = \lambda B x \f$. */ 00344 Ax_lBx = 0x100, 00345 /** Used in GeneralizedSelfAdjointEigenSolver to indicate that it should 00346 * solve the generalized eigenproblem \f$ ABx = \lambda x \f$. */ 00347 ABx_lx = 0x200, 00348 /** Used in GeneralizedSelfAdjointEigenSolver to indicate that it should 00349 * solve the generalized eigenproblem \f$ BAx = \lambda x \f$. */ 00350 BAx_lx = 0x400, 00351 /** \internal */ 00352 GenEigMask = Ax_lBx | ABx_lx | BAx_lx 00353 }; 00354 00355 /** \ingroup enums 00356 * Possible values for the \p QRPreconditioner template parameter of JacobiSVD. */ 00357 enum QRPreconditioners { 00358 /** Do not specify what is to be done if the SVD of a non-square matrix is asked for. */ 00359 NoQRPreconditioner, 00360 /** Use a QR decomposition without pivoting as the first step. */ 00361 HouseholderQRPreconditioner, 00362 /** Use a QR decomposition with column pivoting as the first step. */ 00363 ColPivHouseholderQRPreconditioner, 00364 /** Use a QR decomposition with full pivoting as the first step. */ 00365 FullPivHouseholderQRPreconditioner 00366 }; 00367 00368 #ifdef Success 00369 #error The preprocessor symbol 'Success' is defined, possibly by the X11 header file X.h 00370 #endif 00371 00372 /** \ingroup enums 00373 * Enum for reporting the status of a computation. */ 00374 enum ComputationInfo { 00375 /** Computation was successful. */ 00376 Success = 0, 00377 /** The provided data did not satisfy the prerequisites. */ 00378 NumericalIssue = 1, 00379 /** Iterative procedure did not converge. */ 00380 NoConvergence = 2, 00381 /** The inputs are invalid, or the algorithm has been improperly called. 00382 * When assertions are enabled, such errors trigger an assert. */ 00383 InvalidInput = 3 00384 }; 00385 00386 /** \ingroup enums 00387 * Enum used to specify how a particular transformation is stored in a matrix. 00388 * \sa Transform, Hyperplane::transform(). */ 00389 enum TransformTraits { 00390 /** Transformation is an isometry. */ 00391 Isometry = 0x1, 00392 /** Transformation is an affine transformation stored as a (Dim+1)^2 matrix whose last row is 00393 * assumed to be [0 ... 0 1]. */ 00394 Affine = 0x2, 00395 /** Transformation is an affine transformation stored as a (Dim) x (Dim+1) matrix. */ 00396 AffineCompact = 0x10 | Affine, 00397 /** Transformation is a general projective transformation stored as a (Dim+1)^2 matrix. */ 00398 Projective = 0x20 00399 }; 00400 00401 /** \internal \ingroup enums 00402 * Enum used to choose between implementation depending on the computer architecture. */ 00403 namespace Architecture 00404 { 00405 enum Type { 00406 Generic = 0x0, 00407 SSE = 0x1, 00408 AltiVec = 0x2, 00409 #if defined EIGEN_VECTORIZE_SSE 00410 Target = SSE 00411 #elif defined EIGEN_VECTORIZE_ALTIVEC 00412 Target = AltiVec 00413 #else 00414 Target = Generic 00415 #endif 00416 }; 00417 } 00418 00419 /** \internal \ingroup enums 00420 * Enum used as template parameter in GeneralProduct. */ 00421 enum { CoeffBasedProductMode, LazyCoeffBasedProductMode, OuterProduct, InnerProduct, GemvProduct, GemmProduct }; 00422 00423 /** \internal \ingroup enums 00424 * Enum used in experimental parallel implementation. */ 00425 enum Action {GetAction, SetAction}; 00426 00427 /** The type used to identify a dense storage. */ 00428 struct Dense {}; 00429 00430 /** The type used to identify a matrix expression */ 00431 struct MatrixXpr {}; 00432 00433 /** The type used to identify an array expression */ 00434 struct ArrayXpr {}; 00435 00436 namespace internal { 00437 /** \internal 00438 * Constants for comparison functors 00439 */ 00440 enum ComparisonName { 00441 cmp_EQ = 0, 00442 cmp_LT = 1, 00443 cmp_LE = 2, 00444 cmp_UNORD = 3, 00445 cmp_NEQ = 4 00446 }; 00447 } 00448 00449 } // end namespace Eigen 00450 00451 #endif // EIGEN_CONSTANTS_H
Generated on Tue Jul 12 2022 17:46:51 by
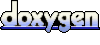