Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
HouseholderSequence< VectorsType, CoeffsType, Side > Class Template Reference
[Householder module]
#include <HouseholderSequence.h>
Inherits EigenBase< HouseholderSequence< VectorsType, CoeffsType, Side > >.
Public Member Functions | |
HouseholderSequence (const VectorsType &v, const CoeffsType &h) | |
Constructor. | |
HouseholderSequence (const HouseholderSequence &other) | |
Copy constructor. | |
Index | rows () const |
Number of rows of transformation viewed as a matrix. | |
Index | cols () const |
Number of columns of transformation viewed as a matrix. | |
const EssentialVectorType | essentialVector (Index k) const |
Essential part of a Householder vector. | |
HouseholderSequence | transpose () const |
Transpose of the Householder sequence. | |
ConjugateReturnType | conjugate () const |
Complex conjugate of the Householder sequence. | |
ConjugateReturnType | adjoint () const |
Adjoint (conjugate transpose) of the Householder sequence. | |
ConjugateReturnType | inverse () const |
Inverse of the Householder sequence (equals the adjoint). | |
template<typename OtherDerived > | |
internal::matrix_type_times_scalar_type < Scalar, OtherDerived >::Type | operator* (const MatrixBase< OtherDerived > &other) const |
Computes the product of a Householder sequence with a matrix. | |
HouseholderSequence & | setLength (Index length) |
Sets the length of the Householder sequence. | |
HouseholderSequence & | setShift (Index shift) |
Sets the shift of the Householder sequence. | |
Index | length () const |
Returns the length of the Householder sequence. | |
Index | shift () const |
Returns the shift of the Householder sequence. | |
HouseholderSequence < VectorsType, CoeffsType, Side > & | derived () |
const HouseholderSequence < VectorsType, CoeffsType, Side > & | derived () const |
Index | size () const |
Protected Member Functions | |
HouseholderSequence & | setTrans (bool trans) |
Sets the transpose flag. | |
bool | trans () const |
Returns the transpose flag. |
Detailed Description
template<typename VectorsType, typename CoeffsType, int Side>
class Eigen::HouseholderSequence< VectorsType, CoeffsType, Side >
Sequence of Householder reflections acting on subspaces with decreasing size
- Template Parameters:
-
VectorsType type of matrix containing the Householder vectors CoeffsType type of vector containing the Householder coefficients Side either OnTheLeft (the default) or OnTheRight
This class represents a product sequence of Householder reflections where the first Householder reflection acts on the whole space, the second Householder reflection leaves the one-dimensional subspace spanned by the first unit vector invariant, the third Householder reflection leaves the two-dimensional subspace spanned by the first two unit vectors invariant, and so on up to the last reflection which leaves all but one dimensions invariant and acts only on the last dimension. Such sequences of Householder reflections are used in several algorithms to zero out certain parts of a matrix. Indeed, the methods HessenbergDecomposition::matrixQ(), Tridiagonalization::matrixQ(), HouseholderQR::householderQ(), and ColPivHouseholderQR::householderQ() all return a HouseholderSequence.
More precisely, the class HouseholderSequence represents an matrix
of the form
where the i-th Householder reflection is
. The i-th Householder coefficient
is a scalar and the i-th Householder vector
is a vector of the form
The last entries of
are called the essential part of the Householder vector.
Typical usages are listed below, where H is a HouseholderSequence:
A.applyOnTheRight(H); // A = A * H A.applyOnTheLeft(H); // A = H * A A.applyOnTheRight(H.adjoint()); // A = A * H^* A.applyOnTheLeft(H.adjoint()); // A = H^* * A MatrixXd Q = H; // conversion to a dense matrix
In addition to the adjoint, you can also apply the inverse (=adjoint), the transpose, and the conjugate operators.
See the documentation for HouseholderSequence(const VectorsType&, const CoeffsType&) for an example.
Definition at line 112 of file HouseholderSequence.h.
Constructor & Destructor Documentation
HouseholderSequence | ( | const VectorsType & | v, |
const CoeffsType & | h | ||
) |
Constructor.
- Parameters:
-
[in] v Matrix containing the essential parts of the Householder vectors [in] h Vector containing the Householder coefficients
Constructs the Householder sequence with coefficients given by h
and vectors given by v
. The i-th Householder coefficient is given by
h(i)
and the essential part of the i-th Householder vector is given by
v(k,i)
with k
> i
(the subdiagonal part of the i-th column). If v
has fewer columns than rows, then the Householder sequence contains as many Householder reflections as there are columns.
- Note:
- The HouseholderSequence object stores
v
andh
by reference.
Example:
Output:
- See also:
- setLength(), setShift()
Definition at line 154 of file HouseholderSequence.h.
HouseholderSequence | ( | const HouseholderSequence< VectorsType, CoeffsType, Side > & | other ) |
Copy constructor.
Definition at line 161 of file HouseholderSequence.h.
Member Function Documentation
ConjugateReturnType adjoint | ( | ) | const |
Adjoint (conjugate transpose) of the Householder sequence.
Definition at line 218 of file HouseholderSequence.h.
Index cols | ( | void | ) | const |
Number of columns of transformation viewed as a matrix.
- Returns:
- Number of columns
This equals the dimension of the space that the transformation acts on.
Reimplemented from EigenBase< HouseholderSequence< VectorsType, CoeffsType, Side > >.
Definition at line 180 of file HouseholderSequence.h.
ConjugateReturnType conjugate | ( | ) | const |
Complex conjugate of the Householder sequence.
Definition at line 209 of file HouseholderSequence.h.
HouseholderSequence< VectorsType, CoeffsType, Side > & derived | ( | ) | [inherited] |
- Returns:
- a reference to the derived object
Definition at line 34 of file EigenBase.h.
const HouseholderSequence< VectorsType, CoeffsType, Side > & derived | ( | ) | const [inherited] |
- Returns:
- a const reference to the derived object
Definition at line 36 of file EigenBase.h.
const EssentialVectorType essentialVector | ( | Index | k ) | const |
Essential part of a Householder vector.
- Parameters:
-
[in] k Index of Householder reflection
- Returns:
- Vector containing non-trivial entries of k-th Householder vector
This function returns the essential part of the Householder vector . This is a vector of length
containing the last
entries of the vector
The index equals
k
+ shift(), corresponding to the k-th column of the matrix v
passed to the constructor.
- See also:
- setShift(), shift()
Definition at line 196 of file HouseholderSequence.h.
ConjugateReturnType inverse | ( | ) | const |
Inverse of the Householder sequence (equals the adjoint).
Definition at line 224 of file HouseholderSequence.h.
Index length | ( | ) | const |
Returns the length of the Householder sequence.
Definition at line 369 of file HouseholderSequence.h.
internal::matrix_type_times_scalar_type<Scalar, OtherDerived>::Type operator* | ( | const MatrixBase< OtherDerived > & | other ) | const |
Computes the product of a Householder sequence with a matrix.
- Parameters:
-
[in] other Matrix being multiplied.
- Returns:
- Expression object representing the product.
This function computes where
is the Householder sequence represented by
*this
and is the matrix
other
.
Definition at line 327 of file HouseholderSequence.h.
Index rows | ( | void | ) | const |
Number of rows of transformation viewed as a matrix.
- Returns:
- Number of rows
This equals the dimension of the space that the transformation acts on.
Reimplemented from EigenBase< HouseholderSequence< VectorsType, CoeffsType, Side > >.
Definition at line 174 of file HouseholderSequence.h.
HouseholderSequence& setLength | ( | Index | length ) |
Sets the length of the Householder sequence.
- Parameters:
-
[in] length New value for the length.
By default, the length of the Householder sequence
is set to the number of columns of the matrix
v
passed to the constructor, or the number of rows if that is smaller. After this function is called, the length equals length
.
- See also:
- length()
Definition at line 346 of file HouseholderSequence.h.
HouseholderSequence& setShift | ( | Index | shift ) |
Sets the shift of the Householder sequence.
- Parameters:
-
[in] shift New value for the shift.
By default, a HouseholderSequence object represents and the i-th column of the matrix
v
passed to the constructor corresponds to the i-th Householder reflection. After this function is called, the object represents and the i-th column of
v
corresponds to the (shift+i)-th Householder reflection.
- See also:
- shift()
Definition at line 363 of file HouseholderSequence.h.
HouseholderSequence& setTrans | ( | bool | trans ) | [protected] |
Sets the transpose flag.
- Parameters:
-
[in] trans New value of the transpose flag.
By default, the transpose flag is not set. If the transpose flag is set, then this object represents instead of
.
- See also:
- trans()
Definition at line 385 of file HouseholderSequence.h.
Index shift | ( | ) | const |
Returns the shift of the Householder sequence.
Definition at line 370 of file HouseholderSequence.h.
Index size | ( | ) | const [inherited] |
- Returns:
- the number of coefficients, which is rows()*cols().
- See also:
- rows(), cols(), SizeAtCompileTime.
Definition at line 49 of file EigenBase.h.
bool trans | ( | ) | const [protected] |
Returns the transpose flag.
Definition at line 391 of file HouseholderSequence.h.
HouseholderSequence transpose | ( | ) | const |
Transpose of the Householder sequence.
Definition at line 203 of file HouseholderSequence.h.
Generated on Tue Jul 12 2022 17:47:05 by
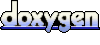