Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Array< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > Class Template Reference
[Core module]
General-purpose arrays with easy API for coefficient-wise operations. More...
#include <Array.h>
Inherits PlainObjectBase< Array< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > >.
Public Member Functions | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE Array & | operator= (const EigenBase< OtherDerived > &other) |
The usage of using Base::operator=; fails on MSVC. | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE Array & | operator= (const ArrayBase< OtherDerived > &other) |
Copies the value of the expression other into *this with automatic resizing. | |
EIGEN_STRONG_INLINE Array & | operator= (const Array &other) |
This is a special case of the templated operator=. | |
EIGEN_STRONG_INLINE | Array () |
Default constructor. | |
EIGEN_STRONG_INLINE | Array (Index dim) |
Constructs a vector or row-vector with given dimension. | |
Array (Index rows, Index cols) | |
constructs an uninitialized matrix with rows rows and cols columns. | |
Array (const Scalar &val0, const Scalar &val1) | |
constructs an initialized 2D vector with given coefficients | |
EIGEN_STRONG_INLINE | Array (const Scalar &val0, const Scalar &val1, const Scalar &val2) |
constructs an initialized 3D vector with given coefficients | |
EIGEN_STRONG_INLINE | Array (const Scalar &val0, const Scalar &val1, const Scalar &val2, const Scalar &val3) |
constructs an initialized 4D vector with given coefficients | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE | Array (const ArrayBase< OtherDerived > &other) |
Constructor copying the value of the expression other. | |
EIGEN_STRONG_INLINE | Array (const Array &other) |
Copy constructor. | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE | Array (const ReturnByValue< OtherDerived > &other) |
Copy constructor with in-place evaluation. | |
template<typename OtherDerived > | |
EIGEN_STRONG_INLINE | Array (const EigenBase< OtherDerived > &other) |
template<typename OtherDerived > | |
void | swap (ArrayBase< OtherDerived > const &other) |
Override MatrixBase::swap() since for dynamic-sized matrices of same type it is enough to swap the data pointers. | |
EIGEN_STRONG_INLINE const Scalar * | data () const |
EIGEN_STRONG_INLINE Scalar * | data () |
EIGEN_STRONG_INLINE void | resize (Index nbRows, Index nbCols) |
Resizes *this to a rows x cols matrix. | |
void | resize (Index size) |
Resizes *this to a vector of length size. | |
void | resize (NoChange_t, Index nbCols) |
Resizes the matrix, changing only the number of columns. | |
void | resize (Index nbRows, NoChange_t) |
Resizes the matrix, changing only the number of rows. | |
EIGEN_STRONG_INLINE void | resizeLike (const EigenBase< OtherDerived > &_other) |
Resizes *this to have the same dimensions as other. | |
EIGEN_STRONG_INLINE void | conservativeResize (Index nbRows, Index nbCols) |
Resizes the matrix to rows x cols while leaving old values untouched. | |
EIGEN_STRONG_INLINE void | conservativeResize (Index nbRows, NoChange_t) |
Resizes the matrix to rows x cols while leaving old values untouched. | |
EIGEN_STRONG_INLINE void | conservativeResize (NoChange_t, Index nbCols) |
Resizes the matrix to rows x cols while leaving old values untouched. | |
EIGEN_STRONG_INLINE void | conservativeResize (Index size) |
Resizes the vector to size while retaining old values. | |
EIGEN_STRONG_INLINE void | conservativeResizeLike (const DenseBase< OtherDerived > &other) |
Resizes the matrix to rows x cols of other , while leaving old values untouched. | |
EIGEN_STRONG_INLINE Array < _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > & | lazyAssign (const DenseBase< OtherDerived > &other) |
Static Public Member Functions | |
Map | |
static ConstMapType | Map (const Scalar *data) |
static MapType | Map (Scalar *data) |
static ConstMapType | Map (const Scalar *data, Index size) |
static MapType | Map (Scalar *data, Index size) |
static ConstMapType | Map (const Scalar *data, Index rows, Index cols) |
static MapType | Map (Scalar *data, Index rows, Index cols) |
static StridedConstMapType < Stride< Outer, Inner > >::type | Map (const Scalar *data, const Stride< Outer, Inner > &stride) |
static StridedMapType< Stride < Outer, Inner > >::type | Map (Scalar *data, const Stride< Outer, Inner > &stride) |
static StridedConstMapType < Stride< Outer, Inner > >::type | Map (const Scalar *data, Index size, const Stride< Outer, Inner > &stride) |
static StridedMapType< Stride < Outer, Inner > >::type | Map (Scalar *data, Index size, const Stride< Outer, Inner > &stride) |
static StridedConstMapType < Stride< Outer, Inner > >::type | Map (const Scalar *data, Index rows, Index cols, const Stride< Outer, Inner > &stride) |
static StridedMapType< Stride < Outer, Inner > >::type | Map (Scalar *data, Index rows, Index cols, const Stride< Outer, Inner > &stride) |
static ConstAlignedMapType | MapAligned (const Scalar *data) |
static AlignedMapType | MapAligned (Scalar *data) |
static ConstAlignedMapType | MapAligned (const Scalar *data, Index size) |
static AlignedMapType | MapAligned (Scalar *data, Index size) |
static ConstAlignedMapType | MapAligned (const Scalar *data, Index rows, Index cols) |
static AlignedMapType | MapAligned (Scalar *data, Index rows, Index cols) |
static StridedConstAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (const Scalar *data, const Stride< Outer, Inner > &stride) |
static StridedAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (Scalar *data, const Stride< Outer, Inner > &stride) |
static StridedConstAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (const Scalar *data, Index size, const Stride< Outer, Inner > &stride) |
static StridedAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (Scalar *data, Index size, const Stride< Outer, Inner > &stride) |
static StridedConstAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (const Scalar *data, Index rows, Index cols, const Stride< Outer, Inner > &stride) |
static StridedAlignedMapType < Stride< Outer, Inner > >::type | MapAligned (Scalar *data, Index rows, Index cols, const Stride< Outer, Inner > &stride) |
Protected Member Functions | |
EIGEN_STRONG_INLINE Array < _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > & | _set (const DenseBase< OtherDerived > &other) |
Copies the value of the expression other into *this with automatic resizing. | |
Friends | |
class | Eigen::Map |
class | Eigen::Map< Derived, Unaligned > |
class | Eigen::Map< const Derived, Unaligned > |
class | Eigen::Map< Derived, Aligned > |
class | Eigen::Map< const Derived, Aligned > |
Detailed Description
template<typename _Scalar, int _Rows, int _Cols, int _Options, int _MaxRows, int _MaxCols>
class Eigen::Array< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols >
General-purpose arrays with easy API for coefficient-wise operations.
The Array class is very similar to the Matrix class. It provides general-purpose one- and two-dimensional arrays. The difference between the Array and the Matrix class is primarily in the API: the API for the Array class provides easy access to coefficient-wise operations, while the API for the Matrix class provides easy access to linear-algebra operations.
This class can be extended with the help of the plugin mechanism described on the page TopicCustomizingEigen by defining the preprocessor symbol EIGEN_ARRAY_PLUGIN
.
- See also:
- TutorialArrayClass, TopicClassHierarchy
Definition at line 42 of file src/Core/Array.h.
Constructor & Destructor Documentation
EIGEN_STRONG_INLINE Array | ( | ) |
Default constructor.
For fixed-size matrices, does nothing.
For dynamic-size matrices, creates an empty matrix of size 0. Does not allocate any array. Such a matrix is called a null matrix. This constructor is the unique way to create null matrices: resizing a matrix to 0 is not supported.
- See also:
- resize(Index,Index)
Definition at line 110 of file src/Core/Array.h.
EIGEN_STRONG_INLINE Array | ( | Index | dim ) | [explicit] |
Constructs a vector or row-vector with given dimension.
Note that this is only useful for dynamic-size vectors. For fixed-size vectors, it is redundant to pass the dimension here, so it makes more sense to use the default constructor Matrix() instead.
Definition at line 148 of file src/Core/Array.h.
Array | ( | Index | rows, |
Index | cols | ||
) |
constructs an uninitialized matrix with rows rows and cols columns.
This is useful for dynamic-size matrices. For fixed-size matrices, it is redundant to pass these parameters, so one should use the default constructor Matrix() instead.
Array | ( | const Scalar & | val0, |
const Scalar & | val1 | ||
) |
constructs an initialized 2D vector with given coefficients
EIGEN_STRONG_INLINE Array | ( | const Scalar & | val0, |
const Scalar & | val1, | ||
const Scalar & | val2 | ||
) |
constructs an initialized 3D vector with given coefficients
Definition at line 177 of file src/Core/Array.h.
EIGEN_STRONG_INLINE Array | ( | const Scalar & | val0, |
const Scalar & | val1, | ||
const Scalar & | val2, | ||
const Scalar & | val3 | ||
) |
constructs an initialized 4D vector with given coefficients
Definition at line 186 of file src/Core/Array.h.
Constructor copying the value of the expression other.
Definition at line 200 of file src/Core/Array.h.
EIGEN_STRONG_INLINE Array | ( | const Array< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > & | other ) |
Copy constructor.
Definition at line 207 of file src/Core/Array.h.
EIGEN_STRONG_INLINE Array | ( | const ReturnByValue< OtherDerived > & | other ) |
Copy constructor with in-place evaluation.
Definition at line 215 of file src/Core/Array.h.
- See also:
- MatrixBase::operator=(const EigenBase<OtherDerived>&)
Definition at line 224 of file src/Core/Array.h.
Member Function Documentation
EIGEN_STRONG_INLINE Array< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > & _set | ( | const DenseBase< OtherDerived > & | other ) | [protected, inherited] |
Copies the value of the expression other into *this
with automatic resizing.
*this might be resized to match the dimensions of other. If *this was a null matrix (not already initialized), it will be initialized.
Note that copying a row-vector into a vector (and conversely) is allowed. The resizing, if any, is then done in the appropriate way so that row-vectors remain row-vectors and vectors remain vectors.
- See also:
- operator=(const MatrixBase<OtherDerived>&), _set_noalias()
Definition at line 628 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void conservativeResize | ( | Index | size ) | [inherited] |
Resizes the vector to size while retaining old values.
. This method does not work for partially dynamic matrices when the static dimension is anything other than 1. For example it will not work with Matrix<double, 2, Dynamic>.
When values are appended, they will be uninitialized.
Definition at line 381 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void conservativeResize | ( | NoChange_t | , |
Index | nbCols | ||
) | [inherited] |
Resizes the matrix to rows x cols while leaving old values untouched.
As opposed to conservativeResize(Index rows, Index cols), this version leaves the number of rows unchanged.
In case the matrix is growing, new columns will be uninitialized.
Definition at line 367 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void conservativeResize | ( | Index | nbRows, |
Index | nbCols | ||
) | [inherited] |
Resizes the matrix to rows x cols while leaving old values untouched.
The method is intended for matrices of dynamic size. If you only want to change the number of rows and/or of columns, you can use conservativeResize(NoChange_t, Index) or conservativeResize(Index, NoChange_t).
Matrices are resized relative to the top-left element. In case values need to be appended to the matrix they will be uninitialized.
Definition at line 342 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void conservativeResize | ( | Index | nbRows, |
NoChange_t | |||
) | [inherited] |
Resizes the matrix to rows x cols while leaving old values untouched.
As opposed to conservativeResize(Index rows, Index cols), this version leaves the number of columns unchanged.
In case the matrix is growing, new rows will be uninitialized.
Definition at line 354 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void conservativeResizeLike | ( | const DenseBase< OtherDerived > & | other ) | [inherited] |
Resizes the matrix to rows x cols of other
, while leaving old values untouched.
The method is intended for matrices of dynamic size. If you only want to change the number of rows and/or of columns, you can use conservativeResize(NoChange_t, Index) or conservativeResize(Index, NoChange_t).
Matrices are resized relative to the top-left element. In case values need to be appended to the matrix they will copied from other
.
Definition at line 396 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE const Scalar* data | ( | ) | const [inherited] |
- Returns:
- a const pointer to the data array of this matrix
Definition at line 212 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE Scalar* data | ( | ) | [inherited] |
- Returns:
- a pointer to the data array of this matrix
Definition at line 216 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE Array< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > & lazyAssign | ( | const DenseBase< OtherDerived > & | other ) | [inherited] |
- See also:
- MatrixBase::lazyAssign()
Definition at line 411 of file PlainObjectBase.h.
The usage of using Base::operator=; fails on MSVC.
Since the code below is working with GCC and MSVC, we skipped the usage of 'using'. This should be done only for operator=.
Reimplemented from PlainObjectBase< Array< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > >.
Definition at line 72 of file src/Core/Array.h.
EIGEN_STRONG_INLINE Array& operator= | ( | const Array< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > & | other ) |
This is a special case of the templated operator=.
Its purpose is to prevent a default operator= from hiding the templated operator=.
Definition at line 95 of file src/Core/Array.h.
Copies the value of the expression other into *this
with automatic resizing.
*this might be resized to match the dimensions of other. If *this was a null matrix (not already initialized), it will be initialized.
Note that copying a row-vector into a vector (and conversely) is allowed. The resizing, if any, is then done in the appropriate way so that row-vectors remain row-vectors and vectors remain vectors.
Definition at line 87 of file src/Core/Array.h.
void resize | ( | Index | nbRows, |
NoChange_t | |||
) | [inherited] |
Resizes the matrix, changing only the number of rows.
For the parameter of type NoChange_t, just pass the special value NoChange
as in the example below.
Example:
Output:
- See also:
- resize(Index,Index)
Definition at line 302 of file PlainObjectBase.h.
void resize | ( | Index | size ) | [inherited] |
Resizes *this
to a vector of length size.
. This method does not work for partially dynamic matrices when the static dimension is anything other than 1. For example it will not work with Matrix<double, 2, Dynamic>.
Example:
Output:
- See also:
- resize(Index,Index), resize(NoChange_t, Index), resize(Index, NoChange_t)
Definition at line 265 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void resize | ( | Index | nbRows, |
Index | nbCols | ||
) | [inherited] |
Resizes *this
to a rows x cols matrix.
This method is intended for dynamic-size matrices, although it is legal to call it on any matrix as long as fixed dimensions are left unchanged. If you only want to change the number of rows and/or of columns, you can use resize(NoChange_t, Index), resize(Index, NoChange_t).
If the current number of coefficients of *this
exactly matches the product rows * cols, then no memory allocation is performed and the current values are left unchanged. In all other cases, including shrinking, the data is reallocated and all previous values are lost.
Example:
Output:
- See also:
- resize(Index) for vectors, resize(NoChange_t, Index), resize(Index, NoChange_t)
Definition at line 235 of file PlainObjectBase.h.
void resize | ( | NoChange_t | , |
Index | nbCols | ||
) | [inherited] |
Resizes the matrix, changing only the number of columns.
For the parameter of type NoChange_t, just pass the special value NoChange
as in the example below.
Example:
Output:
- See also:
- resize(Index,Index)
Definition at line 289 of file PlainObjectBase.h.
EIGEN_STRONG_INLINE void resizeLike | ( | const EigenBase< OtherDerived > & | _other ) | [inherited] |
Resizes *this
to have the same dimensions as other.
Takes care of doing all the checking that's needed.
Note that copying a row-vector into a vector (and conversely) is allowed. The resizing, if any, is then done in the appropriate way so that row-vectors remain row-vectors and vectors remain vectors.
Definition at line 315 of file PlainObjectBase.h.
void swap | ( | ArrayBase< OtherDerived > const & | other ) |
Override MatrixBase::swap() since for dynamic-sized matrices of same type it is enough to swap the data pointers.
Definition at line 236 of file src/Core/Array.h.
Generated on Tue Jul 12 2022 17:47:03 by
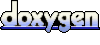