Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
ParametrizedLine< _Scalar, _AmbientDim, _Options > Class Template Reference
[Geometry module]
#include <ParametrizedLine.h>
Public Member Functions | |
ParametrizedLine () | |
Default constructor without initialization. | |
ParametrizedLine (Index _dim) | |
Constructs a dynamic-size line with _dim the dimension of the ambient space. | |
ParametrizedLine (const VectorType &origin, const VectorType &direction) | |
Initializes a parametrized line of direction direction and origin origin. | |
template<int OtherOptions> | |
ParametrizedLine (const Hyperplane< _Scalar, _AmbientDim, OtherOptions > &hyperplane) | |
Constructs a parametrized line from a 2D hyperplane. | |
Index | dim () const |
RealScalar | squaredDistance (const VectorType &p) const |
RealScalar | distance (const VectorType &p) const |
VectorType | projection (const VectorType &p) const |
template<int OtherOptions> | |
Scalar | intersectionParameter (const Hyperplane< _Scalar, _AmbientDim, OtherOptions > &hyperplane) const |
template<int OtherOptions> | |
Scalar | intersection (const Hyperplane< _Scalar, _AmbientDim, OtherOptions > &hyperplane) const |
template<int OtherOptions> | |
VectorType | intersectionPoint (const Hyperplane< _Scalar, _AmbientDim, OtherOptions > &hyperplane) const |
template<typename NewScalarType > | |
internal::cast_return_type < ParametrizedLine, ParametrizedLine < NewScalarType, AmbientDimAtCompileTime, Options > >::type | cast () const |
template<typename OtherScalarType , int OtherOptions> | |
ParametrizedLine (const ParametrizedLine< OtherScalarType, AmbientDimAtCompileTime, OtherOptions > &other) | |
Copy constructor with scalar type conversion. | |
bool | isApprox (const ParametrizedLine &other, const typename NumTraits< Scalar >::Real &prec=NumTraits< Scalar >::dummy_precision()) const |
Static Public Member Functions | |
static ParametrizedLine | Through (const VectorType &p0, const VectorType &p1) |
Constructs a parametrized line going from p0 to p1. |
Detailed Description
template<typename _Scalar, int _AmbientDim, int _Options>
class Eigen::ParametrizedLine< _Scalar, _AmbientDim, _Options >
A parametrized line
A parametrized line is defined by an origin point and a unit direction vector
such that the line corresponds to the set
,
.
- Parameters:
-
_Scalar the scalar type, i.e., the type of the coefficients _AmbientDim the dimension of the ambient space, can be a compile time value or Dynamic.
Definition at line 30 of file ParametrizedLine.h.
Constructor & Destructor Documentation
ParametrizedLine | ( | ) |
Default constructor without initialization.
Definition at line 44 of file ParametrizedLine.h.
ParametrizedLine | ( | Index | _dim ) | [explicit] |
Constructs a dynamic-size line with _dim the dimension of the ambient space.
Definition at line 53 of file ParametrizedLine.h.
ParametrizedLine | ( | const VectorType & | origin, |
const VectorType & | direction | ||
) |
Initializes a parametrized line of direction direction and origin origin.
- Warning:
- the vector direction is assumed to be normalized.
Definition at line 58 of file ParametrizedLine.h.
ParametrizedLine | ( | const Hyperplane< _Scalar, _AmbientDim, OtherOptions > & | hyperplane ) | [explicit] |
Constructs a parametrized line from a 2D hyperplane.
- Warning:
- the ambient space must have dimension 2 such that the hyperplane actually describes a line
Definition at line 146 of file ParametrizedLine.h.
ParametrizedLine | ( | const ParametrizedLine< OtherScalarType, AmbientDimAtCompileTime, OtherOptions > & | other ) | [explicit] |
Copy constructor with scalar type conversion.
Definition at line 122 of file ParametrizedLine.h.
Member Function Documentation
internal::cast_return_type<ParametrizedLine, ParametrizedLine<NewScalarType,AmbientDimAtCompileTime,Options> >::type cast | ( | ) | const |
- Returns:
*this
with scalar type casted to NewScalarType
Note that if NewScalarType is equal to the current scalar type of *this
then this function smartly returns a const reference to *this
.
Definition at line 114 of file ParametrizedLine.h.
Index dim | ( | ) | const |
- Returns:
- the dimension in which the line holds
Definition at line 71 of file ParametrizedLine.h.
RealScalar distance | ( | const VectorType & | p ) | const |
- Returns:
- the distance of a point p to its projection onto the line
*this
.
- See also:
- squaredDistance()
Definition at line 90 of file ParametrizedLine.h.
_Scalar intersection | ( | const Hyperplane< _Scalar, _AmbientDim, OtherOptions > & | hyperplane ) | const |
- Returns:
- the parameter value of the intersection between
*this
and the given hyperplane
Definition at line 178 of file ParametrizedLine.h.
_Scalar intersectionParameter | ( | const Hyperplane< _Scalar, _AmbientDim, OtherOptions > & | hyperplane ) | const |
- Returns:
- the parameter value of the intersection between
*this
and the given hyperplane
Definition at line 166 of file ParametrizedLine.h.
ParametrizedLine< _Scalar, _AmbientDim, _Options >::VectorType intersectionPoint | ( | const Hyperplane< _Scalar, _AmbientDim, OtherOptions > & | hyperplane ) | const |
- Returns:
- the point of the intersection between
*this
and the given hyperplane
Definition at line 188 of file ParametrizedLine.h.
bool isApprox | ( | const ParametrizedLine< _Scalar, _AmbientDim, _Options > & | other, |
const typename NumTraits< Scalar >::Real & | prec = NumTraits<Scalar>::dummy_precision() |
||
) | const |
- Returns:
true
if*this
is approximately equal to other, within the precision determined by prec.
- See also:
- MatrixBase::isApprox()
Definition at line 132 of file ParametrizedLine.h.
VectorType projection | ( | const VectorType & | p ) | const |
- Returns:
- the projection of a point p onto the line
*this
.
Definition at line 93 of file ParametrizedLine.h.
RealScalar squaredDistance | ( | const VectorType & | p ) | const |
- Returns:
- the squared distance of a point p to its projection onto the line
*this
.
- See also:
- distance()
Definition at line 82 of file ParametrizedLine.h.
static ParametrizedLine Through | ( | const VectorType & | p0, |
const VectorType & | p1 | ||
) | [static] |
Constructs a parametrized line going from p0 to p1.
Definition at line 65 of file ParametrizedLine.h.
Generated on Tue Jul 12 2022 17:47:05 by
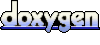