Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
DiagonalMatrix< _Scalar, SizeAtCompileTime, MaxSizeAtCompileTime > Class Template Reference
[Core module]
Represents a diagonal matrix with its storage. More...
#include <DiagonalMatrix.h>
Inherits Eigen::DiagonalBase< DiagonalMatrix< _Scalar, SizeAtCompileTime, MaxSizeAtCompileTime > >.
Public Member Functions | |
const DiagonalVectorType & | diagonal () const |
const version of diagonal(). | |
DiagonalVectorType & | diagonal () |
DiagonalMatrix () | |
Default constructor without initialization. | |
DiagonalMatrix (Index dim) | |
Constructs a diagonal matrix with given dimension. | |
DiagonalMatrix (const Scalar &x, const Scalar &y) | |
2D constructor. | |
DiagonalMatrix (const Scalar &x, const Scalar &y, const Scalar &z) | |
3D constructor. | |
template<typename OtherDerived > | |
DiagonalMatrix (const DiagonalBase< OtherDerived > &other) | |
Copy constructor. | |
DiagonalMatrix (const DiagonalMatrix &other) | |
copy constructor. | |
template<typename OtherDerived > | |
DiagonalMatrix (const MatrixBase< OtherDerived > &other) | |
generic constructor from expression of the diagonal coefficients | |
template<typename OtherDerived > | |
DiagonalMatrix & | operator= (const DiagonalBase< OtherDerived > &other) |
Copy operator. | |
DiagonalMatrix & | operator= (const DiagonalMatrix &other) |
This is a special case of the templated operator=. | |
void | resize (Index size) |
Resizes to given size. | |
void | setZero () |
Sets all coefficients to zero. | |
void | setZero (Index size) |
Resizes and sets all coefficients to zero. | |
void | setIdentity () |
Sets this matrix to be the identity matrix of the current size. | |
void | setIdentity (Index size) |
Sets this matrix to be the identity matrix of the given size. | |
template<typename MatrixDerived > | |
const DiagonalProduct < MatrixDerived, Derived, OnTheLeft > | operator* (const MatrixBase< MatrixDerived > &matrix) const |
Index | size () const |
Detailed Description
template<typename _Scalar, int SizeAtCompileTime, int MaxSizeAtCompileTime>
class Eigen::DiagonalMatrix< _Scalar, SizeAtCompileTime, MaxSizeAtCompileTime >
Represents a diagonal matrix with its storage.
- Parameters:
-
_Scalar the type of coefficients SizeAtCompileTime the dimension of the matrix, or Dynamic MaxSizeAtCompileTime the dimension of the matrix, or Dynamic. This parameter is optional and defaults to SizeAtCompileTime. Most of the time, you do not need to specify it.
- See also:
- class DiagonalWrapper
Definition at line 135 of file DiagonalMatrix.h.
Constructor & Destructor Documentation
DiagonalMatrix | ( | ) |
Default constructor without initialization.
Definition at line 159 of file DiagonalMatrix.h.
DiagonalMatrix | ( | Index | dim ) |
Constructs a diagonal matrix with given dimension.
Definition at line 162 of file DiagonalMatrix.h.
DiagonalMatrix | ( | const Scalar & | x, |
const Scalar & | y | ||
) |
2D constructor.
Definition at line 165 of file DiagonalMatrix.h.
DiagonalMatrix | ( | const Scalar & | x, |
const Scalar & | y, | ||
const Scalar & | z | ||
) |
3D constructor.
Definition at line 168 of file DiagonalMatrix.h.
DiagonalMatrix | ( | const DiagonalBase< OtherDerived > & | other ) |
Copy constructor.
Definition at line 172 of file DiagonalMatrix.h.
DiagonalMatrix | ( | const DiagonalMatrix< _Scalar, SizeAtCompileTime, MaxSizeAtCompileTime > & | other ) |
copy constructor.
prevent a default copy constructor from hiding the other templated constructor
Definition at line 176 of file DiagonalMatrix.h.
DiagonalMatrix | ( | const MatrixBase< OtherDerived > & | other ) | [explicit] |
generic constructor from expression of the diagonal coefficients
Definition at line 181 of file DiagonalMatrix.h.
Member Function Documentation
const DiagonalVectorType& diagonal | ( | ) | const |
const version of diagonal().
Definition at line 154 of file DiagonalMatrix.h.
DiagonalVectorType& diagonal | ( | ) |
- Returns:
- a reference to the stored vector of diagonal coefficients.
Definition at line 156 of file DiagonalMatrix.h.
const DiagonalProduct<MatrixDerived, Derived, OnTheLeft> operator* | ( | const MatrixBase< MatrixDerived > & | matrix ) | const [inherited] |
- Returns:
- the diagonal matrix product of
*this
by the matrix matrix.
Definition at line 63 of file DiagonalMatrix.h.
DiagonalMatrix& operator= | ( | const DiagonalMatrix< _Scalar, SizeAtCompileTime, MaxSizeAtCompileTime > & | other ) |
This is a special case of the templated operator=.
Its purpose is to prevent a default operator= from hiding the templated operator=.
Definition at line 196 of file DiagonalMatrix.h.
DiagonalMatrix& operator= | ( | const DiagonalBase< OtherDerived > & | other ) |
Copy operator.
Definition at line 186 of file DiagonalMatrix.h.
void resize | ( | Index | size ) |
Resizes to given size.
Definition at line 204 of file DiagonalMatrix.h.
void setIdentity | ( | ) |
Sets this matrix to be the identity matrix of the current size.
Definition at line 210 of file DiagonalMatrix.h.
void setIdentity | ( | Index | size ) |
Sets this matrix to be the identity matrix of the given size.
Definition at line 212 of file DiagonalMatrix.h.
void setZero | ( | ) |
Sets all coefficients to zero.
Definition at line 206 of file DiagonalMatrix.h.
void setZero | ( | Index | size ) |
Resizes and sets all coefficients to zero.
Definition at line 208 of file DiagonalMatrix.h.
Index size | ( | ) | const [inherited] |
- Returns:
- the number of coefficients, which is rows()*cols().
Reimplemented in PermutationBase< Derived >, PermutationBase< PermutationWrapper< _IndicesType > >, PermutationBase< Map< PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType >, _PacketAccess > >, and PermutationBase< PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType > >.
Definition at line 49 of file EigenBase.h.
Generated on Tue Jul 12 2022 17:47:04 by
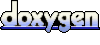