Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
LDLT< _MatrixType, _UpLo > Class Template Reference
[Cholesky module]
Robust Cholesky decomposition of a matrix with pivoting. More...
#include <LDLT.h>
Public Member Functions | |
LDLT () | |
Default Constructor. | |
LDLT (Index size) | |
Default Constructor with memory preallocation. | |
LDLT (const MatrixType &matrix) | |
Constructor with decomposition. | |
void | setZero () |
Clear any existing decomposition. | |
Traits::MatrixU | matrixU () const |
Traits::MatrixL | matrixL () const |
const TranspositionType & | transpositionsP () const |
Diagonal< const MatrixType > | vectorD () const |
bool | isPositive () const |
bool | isNegative (void) const |
template<typename Rhs > | |
const internal::solve_retval < LDLT, Rhs > | solve (const MatrixBase< Rhs > &b) const |
LDLT & | compute (const MatrixType &matrix) |
Compute / recompute the LDLT decomposition A = L D L^* = U^* D U of matrix. | |
const MatrixType & | matrixLDLT () const |
MatrixType | reconstructedMatrix () const |
ComputationInfo | info () const |
Reports whether previous computation was successful. | |
template<typename Derived > | |
LDLT< MatrixType, _UpLo > & | rankUpdate (const MatrixBase< Derived > &w, const typename LDLT< MatrixType, _UpLo >::RealScalar &sigma) |
Update the LDLT decomposition: given A = L D L^T, efficiently compute the decomposition of A + sigma w w^T. |
Detailed Description
template<typename _MatrixType, int _UpLo>
class Eigen::LDLT< _MatrixType, _UpLo >
Robust Cholesky decomposition of a matrix with pivoting.
- Parameters:
-
MatrixType the type of the matrix of which to compute the LDL^T Cholesky decomposition UpLo the triangular part that will be used for the decompositon: Lower (default) or Upper. The other triangular part won't be read.
Perform a robust Cholesky decomposition of a positive semidefinite or negative semidefinite matrix such that
, where P is a permutation matrix, L is lower triangular with a unit diagonal and D is a diagonal matrix.
The decomposition uses pivoting to ensure stability, so that L will have zeros in the bottom right rank(A) - n submatrix. Avoiding the square root on D also stabilizes the computation.
Remember that Cholesky decompositions are not rank-revealing. Also, do not use a Cholesky decomposition to determine whether a system of equations has a solution.
- See also:
- MatrixBase::ldlt(), class LLT
Definition at line 48 of file LDLT.h.
Constructor & Destructor Documentation
LDLT | ( | ) |
Default Constructor.
The default constructor is useful in cases in which the user intends to perform decompositions via LDLT::compute(const MatrixType&).
LDLT | ( | Index | size ) |
LDLT | ( | const MatrixType & | matrix ) |
Constructor with decomposition.
This calculates the decomposition for the input matrix.
- See also:
- LDLT(Index size)
Member Function Documentation
LDLT< MatrixType, _UpLo > & compute | ( | const MatrixType & | matrix ) |
ComputationInfo info | ( | ) | const |
bool isNegative | ( | void | ) | const |
bool isPositive | ( | ) | const |
Traits::MatrixL matrixL | ( | ) | const |
const MatrixType& matrixLDLT | ( | ) | const |
Traits::MatrixU matrixU | ( | ) | const |
LDLT<MatrixType,_UpLo>& rankUpdate | ( | const MatrixBase< Derived > & | w, |
const typename LDLT< MatrixType, _UpLo >::RealScalar & | sigma | ||
) |
Update the LDLT decomposition: given A = L D L^T, efficiently compute the decomposition of A + sigma w w^T.
- Parameters:
-
w a vector to be incorporated into the decomposition. sigma a scalar, +1 for updates and -1 for "downdates," which correspond to removing previously-added column vectors. Optional; default value is +1.
- See also:
- setZero()
MatrixType reconstructedMatrix | ( | ) | const |
void setZero | ( | ) |
const internal::solve_retval<LDLT, Rhs> solve | ( | const MatrixBase< Rhs > & | b ) | const |
- Returns:
- a solution x of
using the current decomposition of A.
This function also supports in-place solves using the syntax x = decompositionObject.solve(x)
.
More precisely, this method solves using the decomposition
by solving the systems
,
,
,
and
in succession. If the matrix
is singular, then
will also be singular (all the other matrices are invertible). In that case, the least-square solution of
is computed. This does not mean that this function computes the least-square solution of
is
is singular.
- See also:
- MatrixBase::ldlt()
const TranspositionType& transpositionsP | ( | ) | const |
Generated on Tue Jul 12 2022 17:47:03 by
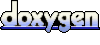