Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Transpositions< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType > Class Template Reference
[Core module]
Represents a sequence of transpositions (row/column interchange) More...
#include <Transpositions.h>
Inherits Eigen::TranspositionsBase< Transpositions< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType > >.
Public Member Functions | |
template<typename OtherDerived > | |
Transpositions (const TranspositionsBase< OtherDerived > &other) | |
Copy constructor. | |
Transpositions (const Transpositions &other) | |
Standard copy constructor. | |
template<typename Other > | |
Transpositions (const MatrixBase< Other > &a_indices) | |
Generic constructor from expression of the transposition indices. | |
template<typename OtherDerived > | |
Transpositions & | operator= (const TranspositionsBase< OtherDerived > &other) |
Copies the other transpositions into *this . | |
Transpositions & | operator= (const Transpositions &other) |
This is a special case of the templated operator=. | |
Transpositions (Index size) | |
Constructs an uninitialized permutation matrix of given size. | |
const IndicesType & | indices () const |
const version of indices(). | |
IndicesType & | indices () |
Index | size () const |
const Index & | coeff (Index i) const |
Direct access to the underlying index vector. | |
Index & | coeffRef (Index i) |
Direct access to the underlying index vector. | |
const Index & | operator() (Index i) const |
Direct access to the underlying index vector. | |
Index & | operator() (Index i) |
Direct access to the underlying index vector. | |
const Index & | operator[] (Index i) const |
Direct access to the underlying index vector. | |
Index & | operator[] (Index i) |
Direct access to the underlying index vector. | |
void | resize (int newSize) |
Resizes to given size. | |
void | setIdentity () |
Sets *this to represents an identity transformation. | |
Transpose< TranspositionsBase > | inverse () const |
Transpose< TranspositionsBase > | transpose () const |
Detailed Description
template<int SizeAtCompileTime, int MaxSizeAtCompileTime, typename IndexType>
class Eigen::Transpositions< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType >
Represents a sequence of transpositions (row/column interchange)
- Parameters:
-
SizeAtCompileTime the number of transpositions, or Dynamic MaxSizeAtCompileTime the maximum number of transpositions, or Dynamic. This optional parameter defaults to SizeAtCompileTime. Most of the time, you should not have to specify it.
This class represents a permutation transformation as a sequence of n transpositions . It is internally stored as a vector of integers
indices
. Each transposition applied on the left of a matrix (
) interchanges the rows
i
and indices
[i] of the matrix M
. A transposition applied on the right (e.g., ) yields a column interchange.
Compared to the class PermutationMatrix, such a sequence of transpositions is what is computed during a decomposition with pivoting, and it is faster when applying the permutation in-place.
To apply a sequence of transpositions to a matrix, simply use the operator * as in the following example:
Transpositions tr; MatrixXf mat; mat = tr * mat;
In this example, we detect that the matrix appears on both side, and so the transpositions are applied in-place without any temporary or extra copy.
- See also:
- class PermutationMatrix
Definition at line 156 of file Transpositions.h.
Constructor & Destructor Documentation
Transpositions | ( | const TranspositionsBase< OtherDerived > & | other ) |
Copy constructor.
Definition at line 169 of file Transpositions.h.
Transpositions | ( | const Transpositions< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType > & | other ) |
Standard copy constructor.
Defined only to prevent a default copy constructor from hiding the other templated constructor
Definition at line 175 of file Transpositions.h.
Transpositions | ( | const MatrixBase< Other > & | a_indices ) | [explicit] |
Generic constructor from expression of the transposition indices.
Definition at line 180 of file Transpositions.h.
Transpositions | ( | Index | size ) |
Constructs an uninitialized permutation matrix of given size.
Definition at line 203 of file Transpositions.h.
Member Function Documentation
const Index& coeff | ( | Index | i ) | const [inherited] |
Direct access to the underlying index vector.
Definition at line 84 of file Transpositions.h.
Index& coeffRef | ( | Index | i ) | [inherited] |
Direct access to the underlying index vector.
Definition at line 86 of file Transpositions.h.
const IndicesType& indices | ( | ) | const |
const version of indices().
Definition at line 207 of file Transpositions.h.
IndicesType& indices | ( | ) |
- Returns:
- a reference to the stored array representing the transpositions.
Definition at line 209 of file Transpositions.h.
Transpose<TranspositionsBase> inverse | ( | ) | const [inherited] |
- Returns:
- the inverse transformation
Definition at line 136 of file Transpositions.h.
const Index& operator() | ( | Index | i ) | const [inherited] |
Direct access to the underlying index vector.
Definition at line 88 of file Transpositions.h.
Index& operator() | ( | Index | i ) | [inherited] |
Direct access to the underlying index vector.
Definition at line 90 of file Transpositions.h.
Transpositions& operator= | ( | const Transpositions< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType > & | other ) |
This is a special case of the templated operator=.
Its purpose is to prevent a default operator= from hiding the templated operator=.
Definition at line 194 of file Transpositions.h.
Transpositions& operator= | ( | const TranspositionsBase< OtherDerived > & | other ) |
Copies the other transpositions into *this
.
Definition at line 185 of file Transpositions.h.
Index& operator[] | ( | Index | i ) | [inherited] |
Direct access to the underlying index vector.
Definition at line 94 of file Transpositions.h.
const Index& operator[] | ( | Index | i ) | const [inherited] |
Direct access to the underlying index vector.
Definition at line 92 of file Transpositions.h.
void resize | ( | int | newSize ) | [inherited] |
Resizes to given size.
Definition at line 102 of file Transpositions.h.
void setIdentity | ( | ) | [inherited] |
Sets *this
to represents an identity transformation.
Definition at line 108 of file Transpositions.h.
Index size | ( | ) | const [inherited] |
- Returns:
- the number of transpositions
Definition at line 81 of file Transpositions.h.
Transpose<TranspositionsBase> transpose | ( | ) | const [inherited] |
- Returns:
- the tranpose transformation
Definition at line 140 of file Transpositions.h.
Generated on Tue Jul 12 2022 17:47:04 by
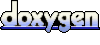