Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Core module
This is the main module of Eigen providing dense matrix and vector support (both fixed and dynamic size) with all the features corresponding to a BLAS library and much more... More...
Data Structures | |
class | DenseCoeffsBase< Derived, ReadOnlyAccessors > |
Base class providing read-only coefficient access to matrices and arrays. More... | |
class | DenseCoeffsBase< Derived, WriteAccessors > |
Base class providing read/write coefficient access to matrices and arrays. More... | |
class | DenseCoeffsBase< Derived, DirectAccessors > |
Base class providing direct read-only coefficient access to matrices and arrays. More... | |
class | DenseCoeffsBase< Derived, DirectWriteAccessors > |
Base class providing direct read/write coefficient access to matrices and arrays. More... | |
class | Array< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > |
General-purpose arrays with easy API for coefficient-wise operations. More... | |
class | ArrayBase< Derived > |
Base class for all 1D and 2D array, and related expressions. More... | |
class | ArrayWrapper< ExpressionType > |
Expression of a mathematical vector or matrix as an array object. More... | |
class | MatrixWrapper< ExpressionType > |
Expression of an array as a mathematical vector or matrix. More... | |
class | BandMatrix< _Scalar, Rows, Cols, Supers, Subs, Options > |
Represents a rectangular matrix with a banded storage. More... | |
class | TridiagonalMatrix< Scalar, Size, Options > |
Represents a tridiagonal matrix with a compact banded storage. More... | |
class | Block< XprType, BlockRows, BlockCols, InnerPanel > |
Expression of a fixed-size or dynamic-size block. More... | |
class | CommaInitializer< XprType > |
Helper class used by the comma initializer operator. More... | |
class | CwiseBinaryOp< BinaryOp, Lhs, Rhs > |
Generic expression where a coefficient-wise binary operator is applied to two expressions. More... | |
class | CwiseNullaryOp< NullaryOp, PlainObjectType > |
Generic expression of a matrix where all coefficients are defined by a functor. More... | |
class | CwiseUnaryOp< UnaryOp, XprType > |
Generic expression where a coefficient-wise unary operator is applied to an expression. More... | |
class | CwiseUnaryView< ViewOp, MatrixType > |
Generic lvalue expression of a coefficient-wise unary operator of a matrix or a vector. More... | |
class | DenseBase |
Base class for all dense matrices, vectors, and arrays. More... | |
class | Diagonal< MatrixType, _DiagIndex > |
Expression of a diagonal/subdiagonal/superdiagonal in a matrix. More... | |
class | DiagonalMatrix< _Scalar, SizeAtCompileTime, MaxSizeAtCompileTime > |
Represents a diagonal matrix with its storage. More... | |
class | DiagonalWrapper< _DiagonalVectorType > |
Expression of a diagonal matrix. More... | |
class | Flagged< ExpressionType, Added, Removed > |
Expression with modified flags. More... | |
class | ForceAlignedAccess< ExpressionType > |
Enforce aligned packet loads and stores regardless of what is requested. More... | |
class | GeneralProduct |
Expression of the product of two general matrices or vectors. More... | |
class | ProductReturnType< Lhs, Rhs, ProductType > |
Helper class to get the correct and optimized returned type of operator*. More... | |
class | IOFormat |
Stores a set of parameters controlling the way matrices are printed. More... | |
class | WithFormat< ExpressionType > |
Pseudo expression providing matrix output with given format. More... | |
class | Map< PlainObjectType, MapOptions, StrideType > |
A matrix or vector expression mapping an existing array of data. More... | |
class | MapBase |
Base class for Map and Block expression with direct access. More... | |
class | Matrix< _Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols > |
The matrix class, also used for vectors and row-vectors. More... | |
class | MatrixBase< Derived > |
Base class for all dense matrices, vectors, and expressions. More... | |
class | NestByValue< ExpressionType > |
Expression which must be nested by value. More... | |
class | NoAlias< ExpressionType, StorageBase > |
Pseudo expression providing an operator = assuming no aliasing. More... | |
class | NumTraits< T > |
Holds information about the various numeric (i.e. More... | |
class | PermutationBase< Derived > |
Base class for permutations. More... | |
class | PermutationMatrix< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType > |
Permutation matrix. More... | |
class | PermutationWrapper< _IndicesType > |
Class to view a vector of integers as a permutation matrix. More... | |
class | Ref< PlainObjectType, Options, StrideType > |
A matrix or vector expression mapping an existing expressions. More... | |
class | Replicate< MatrixType, RowFactor, ColFactor > |
Expression of the multiple replication of a matrix or vector. More... | |
class | Reverse< MatrixType, Direction > |
Expression of the reverse of a vector or matrix. More... | |
class | Select< ConditionMatrixType, ThenMatrixType, ElseMatrixType > |
Expression of a coefficient wise version of the C++ ternary operator ?: More... | |
class | SelfAdjointView< MatrixType, UpLo > |
Expression of a selfadjoint matrix from a triangular part of a dense matrix. More... | |
class | Stride< _OuterStrideAtCompileTime, _InnerStrideAtCompileTime > |
Holds strides information for Map. More... | |
class | Transpose< MatrixType > |
Expression of the transpose of a matrix. More... | |
class | Transpositions< SizeAtCompileTime, MaxSizeAtCompileTime, IndexType > |
Represents a sequence of transpositions (row/column interchange) More... | |
class | TriangularView< _MatrixType, _Mode > |
Base class for triangular part in a matrix. More... | |
class | aligned_allocator< T > |
STL compatible allocator to use with with 16 byte aligned types. More... | |
class | VectorBlock< VectorType, Size > |
Expression of a fixed-size or dynamic-size sub-vector. More... | |
class | PartialReduxExpr< MatrixType, MemberOp, Direction > |
Generic expression of a partially reduxed matrix. More... | |
class | VectorwiseOp< ExpressionType, Direction > |
Pseudo expression providing partial reduction operations. More... | |
Modules | |
Global array typedefs | |
Eigen defines several typedef shortcuts for most common 1D and 2D array types. | |
Global matrix typedefs | |
Eigen defines several typedef shortcuts for most common matrix and vector types. | |
Flags | |
These are the possible bits which can be OR'ed to constitute the flags of a matrix or expression. | |
Enumerations | |
Various enumerations used in Eigen. |
Detailed Description
This is the main module of Eigen providing dense matrix and vector support (both fixed and dynamic size) with all the features corresponding to a BLAS library and much more...
#include <Eigen/Core>
Generated on Tue Jul 12 2022 17:47:02 by
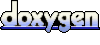