A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
Button Class Reference
A button with a caption. More...
#include <Button.h>
Inherits Clickable.
Public Member Functions | |
Button (const char *caption, COLOR_T *fb, uint16_t x, uint16_t y, uint16_t width, uint16_t height) | |
Creates a new button. | |
void | setCaption (const char *caption) |
Changes the caption. | |
void | setColors (COLOR_T bg, COLOR_T fg, COLOR_T bgPressed, COLOR_T fgPressed) |
Changes the colors. | |
virtual void | draw (COLOR_T *fb=0) |
Draws the button (on a new framebuffer if one is specified) | |
void | setAction (void(*func)(uint32_t arg), uint32_t arg) |
Set the function to call when clicked. | |
bool | handle (uint16_t x, uint16_t y, bool pressed) |
Process the touch event. | |
bool | pressed () |
Test if the button is held down (usable for repeated presses) |
Detailed Description
A button with a caption.
#include "mbed.h" #include "Button.h" SWIM_WINDOW_T win; static void buttonClicked(uint32_t x) { bool* done = (bool*)x; *done = true; } int main(void) { // initialize the display and touch DMBoard::instance().init(); // setup the SWIM window to use swim_window_open(&win, ...); // create a 60x30 pixels button labeled "Done" at 100,100 Button btn("Done", win.fb, 100, 100, 60, 30); btn.draw(); // register a callback for when the button is pressed and pass the // done flag for the callback to modify bool done = false; btn.setAction(buttonClicked, (uint32_t)&done); // keep processing touch events until the button is clicked TouchPanel* touch = DMBoard::instance().touchPanel(); TouchPanel::touchCoordinate_t coord; while(!done) { if (touch->read(coord) == TouchPanel::TouchError_Ok) { if (btn.handle(coord.x, coord.y, coord.z > 0)) { btn.draw(); } } } }
Definition at line 66 of file Button.h.
Constructor & Destructor Documentation
Button | ( | const char * | caption, |
COLOR_T * | fb, | ||
uint16_t | x, | ||
uint16_t | y, | ||
uint16_t | width, | ||
uint16_t | height | ||
) |
Creates a new button.
This button will use a SWIM window to draw on. That window will use part of the full size frame buffer to draw on.
- Parameters:
-
caption the button text fb the frame buffer x the upper left corner of the button y the upper left corner of the button width the width of the button height the height of the button
Definition at line 23 of file Button.cpp.
Member Function Documentation
void draw | ( | COLOR_T * | fb = 0 ) |
[virtual] |
Draws the button (on a new framebuffer if one is specified)
- Parameters:
-
fb the frame buffer
Implements Clickable.
Definition at line 50 of file Button.cpp.
bool handle | ( | uint16_t | x, |
uint16_t | y, | ||
bool | pressed | ||
) | [inherited] |
Process the touch event.
This function will detect if and how the touch event affects it. If the event causes a click then the registered callback function is called before handle() returns.
The return value is to let the caller now if the button should be redrawn or not.
- Parameters:
-
x the touched x coordinate y the touched y coordinate pressed true if the user pressed the display
- Returns:
- true if the button should be redrawn false if the event did not affect the button
Definition at line 40 of file Clickable.cpp.
bool pressed | ( | ) | [inherited] |
Test if the button is held down (usable for repeated presses)
- Returns:
- true if the button is pressed false otherwise
Definition at line 77 of file Clickable.h.
void setAction | ( | void(*)(uint32_t arg) | func, |
uint32_t | arg | ||
) | [inherited] |
Set the function to call when clicked.
Note that this function can be called with NULL as func to unregister the callback function.
- Parameters:
-
func the function to call arc the argument to pass to the function when calling
Definition at line 50 of file Clickable.h.
void setCaption | ( | const char * | caption ) |
Changes the caption.
- Parameters:
-
caption the new text on the button
Definition at line 33 of file Button.cpp.
void setColors | ( | COLOR_T | bg, |
COLOR_T | fg, | ||
COLOR_T | bgPressed, | ||
COLOR_T | fgPressed | ||
) |
Changes the colors.
- Parameters:
-
bg background color when not pressed fg text color when pressed bgPressed background color when pressed fgPressed text color when pressed
Definition at line 42 of file Button.cpp.
Generated on Tue Jul 12 2022 21:27:04 by
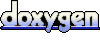