A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
Button.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BUTTON_H 00018 #define BUTTON_H 00019 00020 #include "Clickable.h" 00021 00022 /** 00023 * A button with a caption 00024 * 00025 * @code 00026 * #include "mbed.h" 00027 * #include "Button.h" 00028 * 00029 * SWIM_WINDOW_T win; 00030 * 00031 * static void buttonClicked(uint32_t x) 00032 * { 00033 * bool* done = (bool*)x; 00034 * *done = true; 00035 * } 00036 * 00037 * int main(void) { 00038 * // initialize the display and touch 00039 * DMBoard::instance().init(); 00040 * 00041 * // setup the SWIM window to use 00042 * swim_window_open(&win, ...); 00043 * 00044 * // create a 60x30 pixels button labeled "Done" at 100,100 00045 * Button btn("Done", win.fb, 100, 100, 60, 30); 00046 * btn.draw(); 00047 * 00048 * // register a callback for when the button is pressed and pass the 00049 * // done flag for the callback to modify 00050 * bool done = false; 00051 * btn.setAction(buttonClicked, (uint32_t)&done); 00052 * 00053 * // keep processing touch events until the button is clicked 00054 * TouchPanel* touch = DMBoard::instance().touchPanel(); 00055 * TouchPanel::touchCoordinate_t coord; 00056 * while(!done) { 00057 * if (touch->read(coord) == TouchPanel::TouchError_Ok) { 00058 * if (btn.handle(coord.x, coord.y, coord.z > 0)) { 00059 * btn.draw(); 00060 * } 00061 * } 00062 * } 00063 * } 00064 * @endcode 00065 */ 00066 class Button : public Clickable { 00067 public: 00068 00069 /** Creates a new button 00070 * 00071 * This button will use a SWIM window to draw on. That window will use 00072 * part of the full size frame buffer to draw on. 00073 * 00074 * @param caption the button text 00075 * @param fb the frame buffer 00076 * @param x the upper left corner of the button 00077 * @param y the upper left corner of the button 00078 * @param width the width of the button 00079 * @param height the height of the button 00080 */ 00081 Button(const char* caption, COLOR_T* fb, uint16_t x, uint16_t y, uint16_t width, uint16_t height); 00082 virtual ~Button() {}; 00083 00084 /** Changes the caption 00085 * 00086 * @param caption the new text on the button 00087 */ 00088 void setCaption(const char* caption); 00089 00090 /** Changes the colors 00091 * 00092 * @param bg background color when not pressed 00093 * @param fg text color when pressed 00094 * @param bgPressed background color when pressed 00095 * @param fgPressed text color when pressed 00096 */ 00097 void setColors(COLOR_T bg, COLOR_T fg, COLOR_T bgPressed, COLOR_T fgPressed); 00098 00099 /** Draws the button (on a new framebuffer if one is specified) 00100 * @param fb the frame buffer 00101 */ 00102 virtual void draw(COLOR_T* fb = 0); 00103 00104 private: 00105 const char* _caption; 00106 int _capx, _capy; 00107 COLOR_T _bgCol, _fgCol, _bgColPressed, _fgColPressed; 00108 }; 00109 00110 #endif /* BUTTON_H */
Generated on Tue Jul 12 2022 21:27:03 by
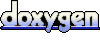