A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
Button.cpp
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "Button.h" 00018 #include "mbed.h" 00019 #include "DMBoard.h" 00020 00021 #include "lpc_swim_font.h" 00022 00023 Button::Button(const char* caption, COLOR_T* fb, uint16_t x, uint16_t y, uint16_t width, uint16_t height) : 00024 Clickable(fb, x, y, width, height), 00025 _caption(caption), _capx(0), _capy(0), 00026 _bgCol(GREEN), _fgCol(BLACK), 00027 _bgColPressed(BLACK), _fgColPressed(GREEN) 00028 { 00029 swim_set_font_transparency(&_win, 1); 00030 setCaption(caption); 00031 } 00032 00033 void Button::setCaption(const char* caption) 00034 { 00035 int w, h; 00036 _caption = caption; 00037 swim_get_string_bounds(&_win, _caption, &w, &h); 00038 _capx = (_win.xpmax-_win.xpmin-w)/2; 00039 _capy = (_win.ypmax-_win.ypmin-h)/2; 00040 } 00041 00042 void Button::setColors(COLOR_T bg, COLOR_T fg, COLOR_T bgPressed, COLOR_T fgPressed) 00043 { 00044 _bgCol = bg; 00045 _fgCol = fg; 00046 _bgColPressed = bgPressed; 00047 _fgColPressed = fgPressed; 00048 } 00049 00050 void Button::draw(COLOR_T* fb) 00051 { 00052 if (fb != NULL) { 00053 _win.fb = fb; 00054 } 00055 if (_pressed) { 00056 swim_set_pen_color(&_win, _fgColPressed); 00057 swim_set_bkg_color(&_win, _bgColPressed); 00058 swim_set_fill_color(&_win, _fgColPressed); 00059 swim_clear_screen(&_win, _bgColPressed); 00060 } else { 00061 swim_set_pen_color(&_win, _fgCol); 00062 swim_set_bkg_color(&_win, _bgCol); 00063 swim_set_fill_color(&_win, _fgCol); 00064 swim_clear_screen(&_win, _bgCol); 00065 } 00066 swim_put_text_xy(&_win, _caption, _capx, _capy); 00067 }
Generated on Tue Jul 12 2022 21:27:03 by
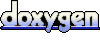