A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
Clickable.cpp
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "Clickable.h" 00018 #include "mbed.h" 00019 #include "DMBoard.h" 00020 00021 #include "lpc_swim_font.h" 00022 00023 Clickable::Clickable(COLOR_T* fb, uint16_t x, uint16_t y, uint16_t width, uint16_t height) 00024 { 00025 _enabled = true; 00026 _pressed = false; 00027 _func = NULL; 00028 00029 Display* disp = DMBoard::instance().display(); 00030 00031 swim_window_open_noclear( 00032 &_win, 00033 disp->width(), disp->height(), // full size 00034 fb, 00035 x, y, x+width-1, y+height-1, // window position and size 00036 0, // border 00037 BLACK, BLACK, BLACK); // colors: pen, backgr, forgr 00038 } 00039 00040 bool Clickable::handle(uint16_t x, uint16_t y, bool pressed) 00041 { 00042 bool needsRepaint = false; 00043 if (_enabled) { 00044 if (!pressed && _pressed) { 00045 // user released => click 00046 needsRepaint = true; 00047 _pressed = false; 00048 if (_func != NULL) { 00049 _func(_funcArg); 00050 } 00051 } 00052 else if ((x >= _win.xpmin) && (y >= _win.ypmin) && (x <= _win.xpmax) && (y <= _win.ypmax)) { 00053 if (pressed && !_pressed) { 00054 // user pressing inside area 00055 needsRepaint = true; 00056 _pressed = true; 00057 } 00058 } 00059 else if (_pressed) { 00060 // pressed but moved outside of the button area 00061 needsRepaint = true; 00062 _pressed = false; 00063 } 00064 } 00065 return needsRepaint; 00066 } 00067
Generated on Tue Jul 12 2022 21:27:03 by
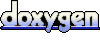