
An advanced robot that uses PID control on the motor speed, and an IMU for making accurate turns.
Dependencies: mbed Motor ITG3200 QEI ADXL345 IMUfilter PID
IMU Class Reference
IMU consisting of ADXL345 accelerometer and ITG-3200 gyroscope to calculate roll, pitch and yaw angles. More...
#include <IMU.h>
Public Member Functions | |
IMU (float imuRate, double gyroscopeMeasurementError, float accelerometerRate, float gyroscopeRate) | |
Constructor. | |
double | getRoll (void) |
Get the current roll angle. | |
double | getPitch (void) |
Get the current pitch angle. | |
double | getYaw (void) |
Get the current yaw angle. | |
void | sample (void) |
Sample the sensors, and if enough samples have been taken, take an average, and compute the new Euler angles. | |
void | reset (void) |
Recalibrate the sensors and reset the IMU filter. |
Detailed Description
IMU consisting of ADXL345 accelerometer and ITG-3200 gyroscope to calculate roll, pitch and yaw angles.
Definition at line 75 of file IMU.h.
Constructor & Destructor Documentation
IMU | ( | float | imuRate, |
double | gyroscopeMeasurementError, | ||
float | accelerometerRate, | ||
float | gyroscopeRate | ||
) |
Constructor.
Includes.
- Parameters:
-
imuRate Rate which IMUfilter update and Euler angle calculation occurs. gyroscopeMeasurementError IMUfilter tuning parameter. accelerometerRate Rate at which accelerometer data is sampled. gyroscopeRate Rate at which gyroscope data is sampled.
LICENSE
Copyright (c) 2010 ARM Limited
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
DESCRIPTION
IMU consisting of ADXL345 accelerometer and ITG-3200 gyroscope using orientation filter developed by Sebastian Madgwick.
Find more details about his paper here:
Member Function Documentation
double getPitch | ( | void | ) |
double getRoll | ( | void | ) |
double getYaw | ( | void | ) |
void reset | ( | void | ) |
Generated on Tue Jul 12 2022 14:43:10 by
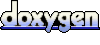