A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
Resource Class Reference
A resource container to pass around instead of using hardcoded data. More...
#include <Resource.h>
Public Member Functions | |
Resource (const char *filename, int width, int height) | |
Create a new file resource. | |
Resource (const unsigned char *data, const unsigned int dataSize, int width, int height) | |
Create a new resource from a data array. | |
int | width () |
Returns the width of the resource. | |
int | height () |
Returns the height of the resource. | |
Friends | |
class | Image |
Detailed Description
A resource container to pass around instead of using hardcoded data.
Having a Resource class makes it possible to hide the source from the user so that e.g. AppColorPicker doesn't need to know if the OK button is loaded from an array or from a file system. The AppColorPicker only need the resource. The Image class takes care of the loading.
#include "mbed.h" #include "Resource.h" #include "Image.h" const unsigned char ok_image1[] = { 137,80,78,71, ... }; int ok_image1_sz = sizeof(ok_image1); int main(void) { // initialize the display ... // create the resources Resource resOk(ok_image1, ok_image1_sz, 40, 40); Resource resCancel("/qspi/cancel.png", 40, 40); // add resource to application AppNetwork app ...; app.setResource(AppNetwork::Resource_Ok_Button, &resOk); app.setResource(AppNetwork::Resource_Cancel_Button, &resCancel); // run application app.setup(); app.runToCompletion(); ... }
Definition at line 60 of file Resource.h.
Constructor & Destructor Documentation
Resource | ( | const char * | filename, |
int | width, | ||
int | height | ||
) |
Create a new file resource.
The width and height are only guidelines and are often not used. The purpose is to allow e.g. the AppColorPicker to know the size of the image before it is loaded from the file system. It can also be used as a guide by the AppLauncher.
- Parameters:
-
filename the resource location width the width of the image height the height of the image
Definition at line 20 of file Resource.cpp.
Resource | ( | const unsigned char * | data, |
const unsigned int | dataSize, | ||
int | width, | ||
int | height | ||
) |
Create a new resource from a data array.
The width and height are only guidelines and are often not used. The purpose is to allow e.g. the AppColorPicker to know the size of the image before it is loaded from the file system. It can also be used as a guide by the AppLauncher.
- Parameters:
-
data the resource dataSize number of bytes in data width the width of the image height the height of the image
Definition at line 30 of file Resource.cpp.
Member Function Documentation
int height | ( | ) |
Returns the height of the resource.
The width and height are only guidelines and are often not used. The purpose is to allow e.g. the AppColorPicker to know the size of the image before it is loaded from the file system. It can also be used as a guide by the AppLauncher.
- Returns:
- the height
Definition at line 111 of file Resource.h.
int width | ( | ) |
Returns the width of the resource.
The width and height are only guidelines and are often not used. The purpose is to allow e.g. the AppColorPicker to know the size of the image before it is loaded from the file system. It can also be used as a guide by the AppLauncher.
- Returns:
- the width
Definition at line 100 of file Resource.h.
Generated on Tue Jul 12 2022 21:27:04 by
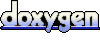