A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
Resource.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef RESOURCE_H 00018 #define RESOURCE_H 00019 00020 #include "mbed.h" 00021 #include "Image.h" 00022 00023 /** 00024 * A resource container to pass around instead of using hardcoded data. 00025 * 00026 * Having a Resource class makes it possible to hide the source from the 00027 * user so that e.g. AppColorPicker doesn't need to know if the OK button 00028 * is loaded from an array or from a file system. The AppColorPicker only 00029 * need the resource. The Image class takes care of the loading. 00030 * 00031 * @code 00032 * #include "mbed.h" 00033 * #include "Resource.h" 00034 * #include "Image.h" 00035 * 00036 * const unsigned char ok_image1[] = { 137,80,78,71, ... }; 00037 * int ok_image1_sz = sizeof(ok_image1); 00038 * 00039 * int main(void) { 00040 * // initialize the display 00041 * ... 00042 * 00043 * // create the resources 00044 * Resource resOk(ok_image1, ok_image1_sz, 40, 40); 00045 * Resource resCancel("/qspi/cancel.png", 40, 40); 00046 * 00047 * // add resource to application 00048 * AppNetwork app ...; 00049 * app.setResource(AppNetwork::Resource_Ok_Button, &resOk); 00050 * app.setResource(AppNetwork::Resource_Cancel_Button, &resCancel); 00051 * 00052 * // run application 00053 * app.setup(); 00054 * app.runToCompletion(); 00055 * 00056 * ... 00057 * } 00058 * @endcode 00059 */ 00060 class Resource { 00061 public: 00062 00063 /** Create a new file resource. 00064 * 00065 * The width and height are only guidelines and are often not used. 00066 * The purpose is to allow e.g. the AppColorPicker to know the size 00067 * of the image before it is loaded from the file system. It can also 00068 * be used as a guide by the AppLauncher. 00069 * 00070 * @param filename the resource location 00071 * @param width the width of the image 00072 * @param height the height of the image 00073 */ 00074 Resource(const char* filename, int width, int height); 00075 00076 /** Create a new resource from a data array. 00077 * 00078 * The width and height are only guidelines and are often not used. 00079 * The purpose is to allow e.g. the AppColorPicker to know the size 00080 * of the image before it is loaded from the file system. It can also 00081 * be used as a guide by the AppLauncher. 00082 * 00083 * @param data the resource 00084 * @param dataSize number of bytes in data 00085 * @param width the width of the image 00086 * @param height the height of the image 00087 */ 00088 Resource(const unsigned char* data, const unsigned int dataSize, int width, int height); 00089 ~Resource(); 00090 00091 /** Returns the width of the resource. 00092 * 00093 * The width and height are only guidelines and are often not used. 00094 * The purpose is to allow e.g. the AppColorPicker to know the size 00095 * of the image before it is loaded from the file system. It can also 00096 * be used as a guide by the AppLauncher. 00097 * 00098 * @return the width 00099 */ 00100 int width() { return _width; } 00101 00102 /** Returns the height of the resource. 00103 * 00104 * The width and height are only guidelines and are often not used. 00105 * The purpose is to allow e.g. the AppColorPicker to know the size 00106 * of the image before it is loaded from the file system. It can also 00107 * be used as a guide by the AppLauncher. 00108 * 00109 * @return the height 00110 */ 00111 int height() { return _height; } 00112 00113 private: 00114 friend class Image; 00115 00116 int _width; 00117 int _height; 00118 bool _isFile; 00119 char* _filename; 00120 const unsigned char* _data; 00121 unsigned int _dataSize; 00122 00123 Image::ImageData_t _img; 00124 }; 00125 00126 #endif
Generated on Tue Jul 12 2022 21:27:04 by
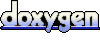