A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
Image Class Reference
#include <Image.h>
Static Public Member Functions | |
static int | decode (const unsigned char *pDataIn, unsigned int sizeIn, Resolution res, ImageData_t *pDataOut) |
Decodes the specified image data. | |
static int | decode (const char *filename, Resolution res, ImageData_t *pDataOut, Mutex *pLock=NULL) |
Reads the specified file and decodes the image data. | |
static int | decode (Resource *res, Resolution resolution, ImageData_t *pDataOut, Mutex *pLock=NULL) |
Decodes the specified image resource. |
Detailed Description
Image example.
#include "mbed.h" #include "Image.h" const unsigned char cube_image1[] = { 137,80,78,71, ... }; int cube_image1_sz = sizeof(cube_image1); int main(void) { // initialize the display ... // decode an image from an array Image::ImageData_t img; if (Image::decode(cube_image1, cube_image1_sz, &img) == 0) { // draw on display using img.pixels, img.width and img.height ... free(img.pixels); } // decode an image from a file if (Image::decode("/ram/image.png", &img) == 0) { // draw on display using img.pixels, img.width and img.height ... free(img.pixels); } }
Definition at line 56 of file Image.h.
Member Function Documentation
int decode | ( | const unsigned char * | pDataIn, |
unsigned int | sizeIn, | ||
Resolution | res, | ||
ImageData_t * | pDataOut | ||
) | [static] |
Decodes the specified image data.
Note that if this function returns a zero, indicating success, the pixels member of the pDataOut structure must be deallocated using free() when no longer needed.
- Parameters:
-
pDataIn the image data sizeIn the number of bytes in the pDataIn array res the format of the display pDataOut the decoded image (only valid if 0 is returned)
- Returns:
- 0 on success 1 on failure
int decode | ( | Resource * | res, |
Resolution | resolution, | ||
ImageData_t * | pDataOut, | ||
Mutex * | pLock = NULL |
||
) | [static] |
Decodes the specified image resource.
Note that if this function returns a zero, indicating success, the pixels member of the pDataOut structure must be deallocated using free() when no longer needed.
The decoded image is cached in the Resource to prevent decoding the same resource over and over.
- Parameters:
-
res the resource to decode resolution the format of the display pDataOut the decoded image (only valid if 0 is returned)
- Returns:
- 0 on success 1 on failure
int decode | ( | const char * | filename, |
Resolution | res, | ||
ImageData_t * | pDataOut, | ||
Mutex * | pLock = NULL |
||
) | [static] |
Reads the specified file and decodes the image data.
Note that if this function returns a zero, indicating success, the pixels member of the pDataOut structure must be deallocated using free() when no longer needed.
- Parameters:
-
filename the file name and path res the format of the display pDataOut the decoded image (only valid if 0 is returned) pLock an optional mutex to prevent multiple access to file operations
- Returns:
- 0 on success 1 on failure
Generated on Tue Jul 12 2022 21:27:04 by
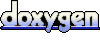