A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
Image.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef IMAGE_H 00018 #define IMAGE_H 00019 00020 #include "mbed.h" 00021 #include "rtos.h" 00022 00023 class Resource; 00024 00025 /** 00026 * Image example 00027 * 00028 * @code 00029 * #include "mbed.h" 00030 * #include "Image.h" 00031 * 00032 * const unsigned char cube_image1[] = { 137,80,78,71, ... }; 00033 * int cube_image1_sz = sizeof(cube_image1); 00034 * 00035 * int main(void) { 00036 * // initialize the display 00037 * ... 00038 * 00039 * // decode an image from an array 00040 * Image::ImageData_t img; 00041 * if (Image::decode(cube_image1, cube_image1_sz, &img) == 0) { 00042 * // draw on display using img.pixels, img.width and img.height 00043 * ... 00044 * free(img.pixels); 00045 * } 00046 * 00047 * // decode an image from a file 00048 * if (Image::decode("/ram/image.png", &img) == 0) { 00049 * // draw on display using img.pixels, img.width and img.height 00050 * ... 00051 * free(img.pixels); 00052 * } 00053 * } 00054 * @endcode 00055 */ 00056 class Image { 00057 public: 00058 00059 enum Type { 00060 BMP = 0, 00061 PNG, 00062 RAW, /* Image prepared with the img_conv.jar tool */ 00063 UNKNOWN 00064 }; 00065 00066 enum Resolution { 00067 RES_16BIT, 00068 RES_24BIT 00069 }; 00070 00071 typedef struct { 00072 uint16_t* pixels; 00073 uint32_t width; 00074 uint32_t height; 00075 Resolution res; 00076 void* pointerToFree; 00077 } ImageData_t; 00078 00079 /** Decodes the specified image data 00080 * 00081 * Note that if this function returns a zero, indicating success, 00082 * the pixels member of the pDataOut structure must be 00083 * deallocated using free() when no longer needed. 00084 * 00085 * @param pDataIn the image data 00086 * @param sizeIn the number of bytes in the pDataIn array 00087 * @param res the format of the display 00088 * @param pDataOut the decoded image (only valid if 0 is returned) 00089 * 00090 * @returns 00091 * 0 on success 00092 * 1 on failure 00093 */ 00094 static int decode(const unsigned char* pDataIn, unsigned int sizeIn, Resolution res, ImageData_t* pDataOut); 00095 00096 /** Reads the specified file and decodes the image data 00097 * 00098 * Note that if this function returns a zero, indicating success, 00099 * the pixels member of the pDataOut structure must be 00100 * deallocated using free() when no longer needed. 00101 * 00102 * @param filename the file name and path 00103 * @param res the format of the display 00104 * @param pDataOut the decoded image (only valid if 0 is returned) 00105 * @param pLock an optional mutex to prevent multiple access to file operations 00106 * 00107 * @returns 00108 * 0 on success 00109 * 1 on failure 00110 */ 00111 static int decode(const char* filename, Resolution res, ImageData_t* pDataOut, Mutex* pLock=NULL); 00112 00113 /** Decodes the specified image resource 00114 * 00115 * Note that if this function returns a zero, indicating success, 00116 * the pixels member of the pDataOut structure must be 00117 * deallocated using free() when no longer needed. 00118 * 00119 * The decoded image is cached in the Resource to prevent decoding 00120 * the same resource over and over. 00121 * 00122 * @param res the resource to decode 00123 * @param resolution the format of the display 00124 * @param pDataOut the decoded image (only valid if 0 is returned) 00125 * 00126 * @returns 00127 * 0 on success 00128 * 1 on failure 00129 */ 00130 static int decode(Resource* res, Resolution resolution, ImageData_t* pDataOut, Mutex* pLock=NULL); 00131 00132 private: 00133 00134 /** No instance needed 00135 * 00136 */ 00137 Image(); 00138 00139 static Type imageType(const unsigned char* pDataIn, unsigned int sizeIn); 00140 00141 static uint32_t fileSize(FILE* f); 00142 }; 00143 00144 #endif
Generated on Tue Jul 12 2022 21:27:03 by
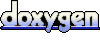