A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
DigitButton Class Reference
The DigitButton is used in the same way as the Button so see it for an example. More...
#include <DigitButton.h>
Inherits Clickable.
Public Member Functions | |
DigitButton (COLOR_T *fb, uint16_t x, uint16_t y, uint16_t width, uint16_t height) | |
Creates a new button. | |
bool | loadImages (const char *imgUp, const char *imgDown=0) |
Loads the mandatory "normal" state image and the optional "pressed" state image. | |
bool | loadImages (const Image::ImageData_t *imgUp, const Image::ImageData_t *imgDown=0) |
Loads the mandatory "normal" state image and the optional "pressed" state image. | |
bool | loadImages (const unsigned char *imgUp, unsigned int imgUpSize, const unsigned char *imgDown=0, unsigned int imgDownSize=0) |
Loads the mandatory "normal" state image and the optional "pressed" state image. | |
virtual void | draw (COLOR_T *fb=0) |
Draws the button (on a new framebuffer if one is specified) | |
void | setAction (void(*func)(uint32_t arg), uint32_t arg) |
Set the function to call when clicked. | |
bool | handle (uint16_t x, uint16_t y, bool pressed) |
Process the touch event. | |
bool | pressed () |
Test if the button is held down (usable for repeated presses) |
Detailed Description
The DigitButton is used in the same way as the Button so see it for an example.
Definition at line 26 of file DigitButton.h.
Constructor & Destructor Documentation
DigitButton | ( | COLOR_T * | fb, |
uint16_t | x, | ||
uint16_t | y, | ||
uint16_t | width, | ||
uint16_t | height | ||
) |
Creates a new button.
This button will use a SWIM window to draw on. That window will use part of the full size frame buffer to draw on.
- Parameters:
-
fb the frame buffer x the upper left corner of the button y the upper left corner of the button width the width of the button height the height of the button
Definition at line 23 of file DigitButton.cpp.
Member Function Documentation
void draw | ( | COLOR_T * | fb = 0 ) |
[virtual] |
Draws the button (on a new framebuffer if one is specified)
- Parameters:
-
fb the frame buffer
Implements Clickable.
Definition at line 135 of file DigitButton.cpp.
bool handle | ( | uint16_t | x, |
uint16_t | y, | ||
bool | pressed | ||
) | [inherited] |
Process the touch event.
This function will detect if and how the touch event affects it. If the event causes a click then the registered callback function is called before handle() returns.
The return value is to let the caller now if the button should be redrawn or not.
- Parameters:
-
x the touched x coordinate y the touched y coordinate pressed true if the user pressed the display
- Returns:
- true if the button should be redrawn false if the event did not affect the button
Definition at line 40 of file Clickable.cpp.
bool loadImages | ( | const Image::ImageData_t * | imgUp, |
const Image::ImageData_t * | imgDown = 0 |
||
) |
Loads the mandatory "normal" state image and the optional "pressed" state image.
- Parameters:
-
imgUp the decoded image for the normal state imgDown the decoded image for the pressed state (or NULL to use the same)
- Returns:
- true on success false on failure
Definition at line 74 of file DigitButton.cpp.
bool loadImages | ( | const char * | imgUp, |
const char * | imgDown = 0 |
||
) |
Loads the mandatory "normal" state image and the optional "pressed" state image.
- Parameters:
-
imgUp the file with the image for the normal state imgDown the file with the image for the pressed state (or NULL to use the same)
- Returns:
- true on success false on failure
Definition at line 47 of file DigitButton.cpp.
bool loadImages | ( | const unsigned char * | imgUp, |
unsigned int | imgUpSize, | ||
const unsigned char * | imgDown = 0 , |
||
unsigned int | imgDownSize = 0 |
||
) |
Loads the mandatory "normal" state image and the optional "pressed" state image.
- Parameters:
-
imgUp the image for the normal state imgDown the image for the pressed state (or NULL to use the same)
- Returns:
- true on success false on failure
Definition at line 95 of file DigitButton.cpp.
bool pressed | ( | ) | [inherited] |
Test if the button is held down (usable for repeated presses)
- Returns:
- true if the button is pressed false otherwise
Definition at line 77 of file Clickable.h.
void setAction | ( | void(*)(uint32_t arg) | func, |
uint32_t | arg | ||
) | [inherited] |
Set the function to call when clicked.
Note that this function can be called with NULL as func to unregister the callback function.
- Parameters:
-
func the function to call arc the argument to pass to the function when calling
Definition at line 50 of file Clickable.h.
Generated on Tue Jul 12 2022 21:27:04 by
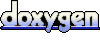