A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
SlideShow Class Reference
#include <SlideShow.h>
Public Types | |
typedef SlideShowError(* | calloutFunc )(int calloutId, SlideShow *ss, int identifier) |
A function that the script can call during execution. | |
Public Member Functions | |
SlideShow (Renderer *r, const char *pathPrefix=NULL, int xoff=0, int yoff=0, int layer=0, Mutex *fileMutex=NULL) | |
Create a new slideshow. | |
SlideShowError | prepare (const char *scriptFile) |
Parses the script file and prepares internal structures. | |
SlideShowError | run () |
Run the slideshow. | |
void | setCalloutHandler (calloutFunc func, int calloutId) |
Register a function that the script can call. | |
void | releaseScreen (void) |
Decouples this SlideShow from the Renderer. | |
void | stop () |
Stops the SlideShow. |
Detailed Description
SlideShow engine.
For information on how to use the SlideShow and some examples see https://developer.mbed.org/teams/Embedded-Artists/wiki/LPC4088DM-Using-the-SlideShow-Engine
#include "mbed.h" #include "SlideShow.h" #include "Renderer.h" static void tRender(void const *args) { Renderer* s = (Renderer*)args; s->render(); } int main(void) { // initialize the display ... // create the renderer that will handle the display Renderer r; // create the slideshow SlideShow s(&r); // parse and validate the script SlideShow::SlideShowError err = s.prepare("/mci/myscript.txt"); if (err != SlideShow::Ok) { // handle error here } // Create the thread to handle the display Thread tr(tRender, &r, osPriorityHigh); // Run the slideshow s.run(); }
Definition at line 66 of file SlideShow.h.
Member Typedef Documentation
typedef SlideShowError(* calloutFunc)(int calloutId, SlideShow *ss, int identifier) |
A function that the script can call during execution.
- Parameters:
-
calloutId identifier given in setCalloutHandler() ss the slideshow instance identifier parameter to the "callout" tag in the script
- Returns:
- Ok on success One of the SlideShowError error codes on failure
Definition at line 89 of file SlideShow.h.
Constructor & Destructor Documentation
SlideShow | ( | Renderer * | r, |
const char * | pathPrefix = NULL , |
||
int | xoff = 0 , |
||
int | yoff = 0 , |
||
int | layer = 0 , |
||
Mutex * | fileMutex = NULL |
||
) |
Create a new slideshow.
- Parameters:
-
r the renderer pathPrefix optional path to prepend to all paths in the script file xoff optional x coordinate to draw the slideshow at, default is 0 yoff optional y coordinate to draw the slideshow at, default is 0 layer optional z-order, higher layers are drawn on top of lower layers fileMutex optional mutex to prevent simultaneous access of the file system
Definition at line 816 of file SlideShow.cpp.
Member Function Documentation
SlideShow::SlideShowError prepare | ( | const char * | scriptFile ) |
Parses the script file and prepares internal structures.
- Parameters:
-
scriptFile the script with the slideshow
- Returns:
- Ok on success One of the SlideShowError error codes on failure
Definition at line 871 of file SlideShow.cpp.
void releaseScreen | ( | void | ) |
Decouples this SlideShow from the Renderer.
Definition at line 928 of file SlideShow.cpp.
SlideShow::SlideShowError run | ( | ) |
Run the slideshow.
This function will run until the script ends. If the script has an eternal loop then this function will never return.
- Returns:
- Ok on success One of the SlideShowError error codes on failure
Definition at line 915 of file SlideShow.cpp.
void setCalloutHandler | ( | calloutFunc | func, |
int | calloutId | ||
) |
Register a function that the script can call.
A callout function allows the use of the "callout" tag in a slideshow script to e.g. notify the system or to trigger something.
There can only be one callout function.
Definition at line 922 of file SlideShow.cpp.
void stop | ( | ) |
Stops the SlideShow.
The SlideShow will be stopped at the next suitable time, typically before processing the next step in the script.
This function is typically called upon an external trigger like the user pressing a button to end the slideshow.
Definition at line 146 of file SlideShow.h.
Generated on Tue Jul 12 2022 21:27:04 by
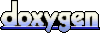