My current Code
#include "mbed.h"
#define OUT_MAX 2104
#define OUT_MIN 1632
#define PRES_MAX 5
#define PRES_MIN 0
SPI spi(p5, p6, p7); // mosi - no connection, miso - connected to pin 3 of sensor, sclk connected to pin 4 of sensor
DigitalOut cs(p8); // chip Select line connected to pin 5 of sensor
Serial pc(p13,p14); // tx, rx
int main() {
// Setup the spi for 8 bit data with a 800KHz clock rate
spi.format(8,0);
spi.frequency(800000);
while(1)
{
// Select the device by seting chip select low
cs = 0;
wait(0.1);
// Send a dummy byte to receive the contents
int byte_1 = spi.write(0x00);
int byte_2 = spi.write(0x00);
int byte_3 = spi.write(0x00);
int byte_4 = spi.write(0x00);
float temp = byte_3<<3;
temp = ((temp/2047)*200)-50;
float psi = byte_1<<8|byte_2;
//psi = byte_1|byte_2;
psi = ((psi-OUT_MIN)*5)/(OUT_MAX-OUT_MIN);
pc.printf("Byte 1 = %X; Byte 2 = %X; Byte 3 = %X; Byte 4 = %X; PSI = %.4f; TEMP = %.2f\r",byte_1,byte_2,byte_3,byte_4,psi,temp);
// Deselect the device
cs = 1;
wait(0.5);
}
}
And my results;
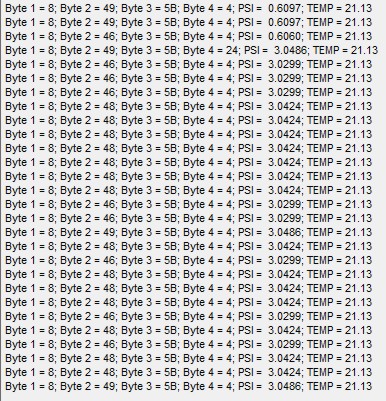
The TEMP is fairly close. it is of by 2 degrees (I'm only using byte 3), but I assume the inside of the chip is warmer than the outside air.
The PSI is somewhat close, but not right. (This is a 0 to 5 psi sensor BTW, http://sensing.honeywell.com/index.cfm/ci_id/156218/la_id/1/document/1/re_id/0 )
I'm fairly sure I don't have the math correct, but I don't know what the OUTPUTmin and OUTPUTmax are supposed to be. I tried using the 2^14 but thats way off. If I use the value at 0 psi for OUTPUTmin and then use the value at 5 psi for the OUTPUTmax I can get it close but still varies wildly (as in the current code) - so I don't think that is right as it is not very accurate to even call it a sensor.
So I am still missing something here.
Thanks for your help, much appreciated.
Hello,
I need to communicate with a Honeywell pressure sensor over the SPI bus, but I am not familiar with the SPI bus at all.
Can anyone tell me how to read the pressure, or at least explain what I need. This is a link the SPI information but it seems incomplete to me.
http://sensing.honeywell.com/index.cfm/ci_id/156989/la_id/1/document/1/re_id/0
Thanks