TinyCHR6dm is a simplified interface for the CH Robotics CHR-6dm AHRS
TinyCHR6dm Class Reference
TinyCHR6dm is a simplified interface for the CH Robotics CHR-6dm AHRS http://www.chrobotics.com/index.php?main_page=product_info&cPath=1&products_id=2. More...
#include <TinyCHR6dm.h>
Public Member Functions | |
TinyCHR6dm () | |
Creates an protocol parser for CHR-6dm device. | |
void | parse (char c) |
Parse a character of data from CHR-6dm. | |
float | readYaw (void) |
Get the current yaw (course) | |
bool | dataReady (void) |
Determine if yaw data is ready. | |
void | resetReady (void) |
Reset the data ready flag. | |
void | send_packet (Serial *serial, uint8 pt, uint8 n, char data[]) |
Create a packet using specified PT, N, and data and adding 'snp' header and 2-byte checksum. | |
int | status (void) |
Return latest status packet type sent by the AHRS, resets statusReady() to false. | |
bool | statusReady (void) |
Indicates whether a status message is ready to be read with status() | |
char * | statusString (int status) |
Converts status to readable text string. |
Detailed Description
TinyCHR6dm is a simplified interface for the CH Robotics CHR-6dm AHRS http://www.chrobotics.com/index.php?main_page=product_info&cPath=1&products_id=2.
Written by Michael Shimniok http://www.bot-thoughts.com/
In the style of TinyGPS, this library parses a subset of the CHR-6dm packets to provide a minimal set of data and status messages to the caller. Also provides utility to send commands to the AHRS. This is an early release and a work in progress, so the interface may change significantly over the next couple of versions. Also, the current version only supports reading yaw data. More may be supported in the future.
#include "mbed.h" #include "TinyCHR6dm.h" Serial pc(USBTX, USBRX); Serial ahrs(p26, p25); TinyCHR6dm ahrsParser; DigitalOut myled(LED1); Timer t; void recv() { while (ahrs.readable()) ahrsParser.parse(ahrs.getc()); } int main() { char data[128]; int status; t.reset(); t.start(); pc.baud(115200); ahrs.baud(115200); ahrs.attach(recv, Serial::RxIrq); wait(0.5); // Configure AHRS data[0] = Accel_EN; ahrsParser.send_packet(&ahrs, SET_EKF_CONFIG, 1, data); wait(0.5); status = ahrsParser.status(); pc.printf("Status: %02x %s\n", status, ahrsParser.statusString(status)); ahrsParser.send_packet(&ahrs, ZERO_RATE_GYROS, 0, 0); wait(0.5); status = ahrsParser.status(); pc.printf("Status: %02x %s\n", status, ahrsParser.statusString(status)); ahrsParser.send_packet(&ahrs, EKF_RESET, 0, 0); wait(0.5); status = ahrsParser.status(); pc.printf("Status: %02x %s\n", status, ahrsParser.statusString(status)); while(1) { int millis = t.read_ms(); if ((millis % 500) == 0) { while (!ahrsParser.dataReady()); pc.printf("yaw: %.2f\n", ahrsParser.readYaw()); } } }
Definition at line 166 of file TinyCHR6dm.h.
Constructor & Destructor Documentation
TinyCHR6dm | ( | ) |
Creates an protocol parser for CHR-6dm device.
Definition at line 20 of file TinyCHR6dm.cpp.
Member Function Documentation
bool dataReady | ( | void | ) |
Determine if yaw data is ready.
- Parameters:
-
return true right after parsing SENSOR_DATA from AHRS
Definition at line 80 of file TinyCHR6dm.cpp.
void parse | ( | char | c ) |
Parse a character of data from CHR-6dm.
- Parameters:
-
c is the character to be parsed
Definition at line 26 of file TinyCHR6dm.cpp.
float readYaw | ( | void | ) |
Get the current yaw (course)
- Returns:
- the current inertia-frame yaw aka course
Definition at line 76 of file TinyCHR6dm.cpp.
void resetReady | ( | void | ) |
Reset the data ready flag.
Definition at line 84 of file TinyCHR6dm.cpp.
void send_packet | ( | Serial * | serial, |
uint8 | pt, | ||
uint8 | n, | ||
char | data[] | ||
) |
Create a packet using specified PT, N, and data and adding 'snp' header and 2-byte checksum.
Checksum Datasheet: After the CHR6-dm receives a full acket, it checks to ensure that the checksum given in the last two bytes matches the sum of all preceding bytes in the packet.
Definition at line 90 of file TinyCHR6dm.cpp.
int status | ( | void | ) |
Return latest status packet type sent by the AHRS, resets statusReady() to false.
- Parameters:
-
returns the status as an integer corresponding to the TX packet type
Definition at line 126 of file TinyCHR6dm.cpp.
bool statusReady | ( | void | ) |
Indicates whether a status message is ready to be read with status()
- Parameters:
-
returns true if a status message has been received since the last call to status()
Definition at line 131 of file TinyCHR6dm.cpp.
char * statusString | ( | int | status ) |
Converts status to readable text string.
- Parameters:
-
returns pointer to static string containing status message text
Definition at line 135 of file TinyCHR6dm.cpp.
Generated on Tue Jul 19 2022 04:53:45 by
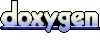