Driver for the Digole Serial universal LCD display adapter
DigoleSerialDisp Class Reference
Digole Serial LCD/OLED Library www.digole.com/index.php?productID=535. More...
#include <DigoleSerialDisp.h>
Public Member Functions | |
DigoleSerialDisp (PinName sda, PinName scl, uint8_t address=0x27) | |
Create a new Digole Serial Display interface. | |
void | begin (void) |
Carryover from Arduino library, not needed. | |
size_t | write (const char x) |
Write out a raw character. | |
size_t | write (const char *buffer, size_t size) |
Write out raw data from a buffer. | |
size_t | write (const char *str) |
Write out raw string. | |
size_t | print (const char c) |
Prints a char to the display in a single I2C transmission using "TTb\0". | |
size_t | print (const char s[]) |
Prints a string of data to the display in a single I2C transmission using "TTbbb...\0". | |
size_t | print (unsigned char u, int base=DEC) |
Print out an unsigned char as a number. | |
size_t | print (int i, int base=DEC) |
Print out an integer. | |
size_t | print (unsigned int u, int base=DEC) |
Print out an unsigned integer. | |
size_t | print (long l, int base=DEC) |
Print out a long as a number. | |
size_t | print (unsigned long l, int base=DEC) |
Print out an unsigned long. | |
size_t | print (double f, int digits=2) |
Print out a double. | |
size_t | println (const char s[]) |
Prints a string of data to the display in a single I2C transmission using "TTbbb...\0". | |
size_t | println (char c) |
Prints a char the display in a single I2C transmission using "TTb\0". | |
size_t | println (unsigned char u, int base=DEC) |
Prints an unsigned char as a number. | |
size_t | println (int i, int base=DEC) |
Print out an integer. | |
size_t | println (unsigned int u, int base=DEC) |
Print out an unsigned char as a number. | |
size_t | println (long l, int base=DEC) |
Print out a long as a number. | |
size_t | println (unsigned long l, int base=DEC) |
Print out an unsigned long. | |
size_t | println (double f, int digits=2) |
Print out a double. | |
size_t | println (void) |
prints, well, nothing in this case, but pretend we printed a newline | |
void | disableCursor (void) |
Turns off the cursor. | |
void | enableCursor (void) |
Turns on the cursor. | |
void | drawStr (uint8_t x, uint8_t y, const char *s) |
Displays a string at specified coordinates. | |
void | setPrintPos (uint8_t x, uint8_t y, uint8_t graph=_TEXT_) |
Sets the print position for graphics or text. | |
void | clearScreen (void) |
Clears the display screen. | |
void | setLCDColRow (uint8_t col, uint8_t row) |
Configure your LCD if other than 1602 and the chip is other than KS0066U/F / HD44780. | |
void | setI2CAddress (uint8_t add) |
Sets a new I2C address for the display (default is 0x27), the adapter will store the new address in memory. | |
void | displayConfig (uint8_t v) |
Display Config on/off, the factory default set is on, so, when the module is powered up, it will display current communication mode on LCD, after you design finished, you can turn it off. | |
void | preprint (void) |
Holdover from Arduino library; not needed. | |
void | setFont (uint8_t font) |
Sets the font. | |
void | nextTextLine (void) |
go to next text line, depending on the font size | |
void | setColor (uint8_t) |
set color for graphic function | |
void | backLightOn (void) |
Turn on back light. | |
void | backLightOff (void) |
Turn off back light. | |
void | directCommand (uint8_t d) |
send command to LCD drectly | |
void | directData (uint8_t d) |
send data to LCD drectly | |
void | moveArea (uint8_t x0, uint8_t y0, uint8_t x1, uint8_t y1, char xoffset, char yoffset) |
Move rectangle area on screen to another place. | |
void | displayStartScreen (uint8_t m) |
Display startup screen. | |
void | setMode (uint8_t m) |
Set display mode. | |
void | setTextPosBack (void) |
set text position back to previous, only one back allowed | |
void | setLCDChip (uint8_t chip) |
Only for universal serial adapter. | |
void | uploadStartScreen (int lon, const unsigned char *data) |
Set Start Screen, 1st B is the lower byte of data length. | |
void | uploadUserFont (int lon, const unsigned char *data, uint8_t sect) |
Upload a user font. | |
void | digitalOutput (uint8_t x) |
Send a Byte to output head on board. |
Detailed Description
Digole Serial LCD/OLED Library www.digole.com/index.php?productID=535.
Includes Arduino Print class member functions
Definition at line 33 of file DigoleSerialDisp.h.
Constructor & Destructor Documentation
DigoleSerialDisp | ( | PinName | sda, |
PinName | scl, | ||
uint8_t | address = 0x27 |
||
) |
Create a new Digole Serial Display interface.
- Parameters:
-
sda is the pin for I2C SDA scl is the pin for I2C SCL address is the 7-bit address (default is 0x27 for the device)
Definition at line 21 of file DigoleSerialDisp.cpp.
Member Function Documentation
void backLightOff | ( | void | ) |
Turn off back light.
Definition at line 448 of file DigoleSerialDisp.cpp.
void backLightOn | ( | void | ) |
Turn on back light.
Definition at line 443 of file DigoleSerialDisp.cpp.
void begin | ( | void | ) |
Carryover from Arduino library, not needed.
Definition at line 47 of file DigoleSerialDisp.h.
void clearScreen | ( | void | ) |
Clears the display screen.
Definition at line 297 of file DigoleSerialDisp.cpp.
void digitalOutput | ( | uint8_t | x ) |
Send a Byte to output head on board.
- Parameters:
-
x is the byte to output
Definition at line 549 of file DigoleSerialDisp.cpp.
void directCommand | ( | uint8_t | d ) |
send command to LCD drectly
- Parameters:
-
d - command
Definition at line 453 of file DigoleSerialDisp.cpp.
void directData | ( | uint8_t | d ) |
send data to LCD drectly
- Parameters:
-
d is the data
Definition at line 458 of file DigoleSerialDisp.cpp.
void disableCursor | ( | void | ) |
Turns off the cursor.
Definition at line 262 of file DigoleSerialDisp.cpp.
void displayConfig | ( | uint8_t | v ) |
Display Config on/off, the factory default set is on, so, when the module is powered up, it will display current communication mode on LCD, after you design finished, you can turn it off.
- Parameters:
-
v is the 1 is on, 0 is off
Definition at line 318 of file DigoleSerialDisp.cpp.
void displayStartScreen | ( | uint8_t | m ) |
Display startup screen.
Definition at line 474 of file DigoleSerialDisp.cpp.
void drawStr | ( | uint8_t | x, |
uint8_t | y, | ||
const char * | s | ||
) |
Displays a string at specified coordinates.
- Parameters:
-
x is the x coordinate to display the string y is the y coordinate to display the string s is the string to display
Definition at line 274 of file DigoleSerialDisp.cpp.
void enableCursor | ( | void | ) |
Turns on the cursor.
Definition at line 268 of file DigoleSerialDisp.cpp.
void moveArea | ( | uint8_t | x0, |
uint8_t | y0, | ||
uint8_t | x1, | ||
uint8_t | y1, | ||
char | xoffset, | ||
char | yoffset | ||
) |
Move rectangle area on screen to another place.
- Parameters:
-
x0,y1 is the top left of the area to move x1,y1 is the bottom right of the area to move xoffset,yoffset is the the distance to move
Definition at line 463 of file DigoleSerialDisp.cpp.
void nextTextLine | ( | void | ) |
go to next text line, depending on the font size
Definition at line 428 of file DigoleSerialDisp.cpp.
void preprint | ( | void | ) |
Holdover from Arduino library; not needed.
Definition at line 324 of file DigoleSerialDisp.cpp.
size_t print | ( | int | i, |
int | base = DEC |
||
) |
Print out an integer.
- Parameters:
-
i is the integer to print base is the base to print, either DEC (default), HEX, BIN
- Returns:
- number of chars written
Definition at line 111 of file DigoleSerialDisp.cpp.
size_t print | ( | unsigned int | u, |
int | base = DEC |
||
) |
Print out an unsigned integer.
- Parameters:
-
u is the integer to print base is the base to print, either DEC (default), HEX, BIN
- Returns:
- number of chars written
Definition at line 116 of file DigoleSerialDisp.cpp.
size_t print | ( | unsigned long | l, |
int | base = DEC |
||
) |
Print out an unsigned long.
- Parameters:
-
l is the integer to print base is the base to print, either DEC (default), HEX, BIN
- Returns:
- number of chars written
Definition at line 137 of file DigoleSerialDisp.cpp.
size_t print | ( | const char | c ) |
Prints a char to the display in a single I2C transmission using "TTb\0".
- Parameters:
-
c is the character to print
- Returns:
- 1
Definition at line 54 of file DigoleSerialDisp.cpp.
size_t print | ( | long | l, |
int | base = DEC |
||
) |
Print out a long as a number.
- Parameters:
-
l is the integer to print base is the base to print, either DEC (default), HEX, BIN
- Returns:
- number of chars written
Definition at line 121 of file DigoleSerialDisp.cpp.
size_t print | ( | double | f, |
int | digits = 2 |
||
) |
Print out a double.
- Parameters:
-
f is the integer to print digits is the number of digits after the decimal
Definition at line 143 of file DigoleSerialDisp.cpp.
size_t print | ( | const char | s[] ) |
Prints a string of data to the display in a single I2C transmission using "TTbbb...\0".
- Parameters:
-
s is the null-terminated char array to print
- Returns:
- length of s
Definition at line 65 of file DigoleSerialDisp.cpp.
size_t print | ( | unsigned char | u, |
int | base = DEC |
||
) |
Print out an unsigned char as a number.
- Parameters:
-
u is the integer to print base is the base to print, either DEC (default), HEX, BIN
- Returns:
- number of chars written
Definition at line 106 of file DigoleSerialDisp.cpp.
size_t println | ( | int | i, |
int | base = DEC |
||
) |
Print out an integer.
- Parameters:
-
i is the integer to print base is the base to print, either DEC (default), HEX, BIN
- Returns:
- number of chars written
Definition at line 155 of file DigoleSerialDisp.cpp.
size_t println | ( | unsigned int | u, |
int | base = DEC |
||
) |
Print out an unsigned char as a number.
- Parameters:
-
u is the integer to print base is the base to print, either DEC (default), HEX, BIN
- Returns:
- number of chars written
Definition at line 162 of file DigoleSerialDisp.cpp.
size_t println | ( | long | l, |
int | base = DEC |
||
) |
Print out a long as a number.
- Parameters:
-
l is the integer to print base is the base to print, either DEC (default), HEX, BIN
- Returns:
- number of chars written
Definition at line 169 of file DigoleSerialDisp.cpp.
size_t println | ( | unsigned long | l, |
int | base = DEC |
||
) |
Print out an unsigned long.
- Parameters:
-
l is the integer to print base is the base to print, either DEC (default), HEX, BIN
- Returns:
- number of chars written
Definition at line 176 of file DigoleSerialDisp.cpp.
size_t println | ( | double | f, |
int | digits = 2 |
||
) |
Print out a double.
- Parameters:
-
f is the integer to print digits is the number of digits after the decimal
- Returns:
- number of chars written
Definition at line 183 of file DigoleSerialDisp.cpp.
size_t println | ( | void | ) |
prints, well, nothing in this case, but pretend we printed a newline
- Returns:
- 1
Definition at line 190 of file DigoleSerialDisp.cpp.
size_t println | ( | const char | s[] ) |
Prints a string of data to the display in a single I2C transmission using "TTbbb...\0".
- Parameters:
-
s is the null-terminated char array to print
- Returns:
- length of s
Definition at line 80 of file DigoleSerialDisp.cpp.
size_t println | ( | char | c ) |
Prints a char the display in a single I2C transmission using "TTb\0".
- Parameters:
-
c is the character to print
- Returns:
- 1
size_t println | ( | unsigned char | u, |
int | base = DEC |
||
) |
Prints an unsigned char as a number.
- Parameters:
-
u is the unsigned char number
- Returns:
- 1
Definition at line 148 of file DigoleSerialDisp.cpp.
void setColor | ( | uint8_t | color ) |
set color for graphic function
Definition at line 438 of file DigoleSerialDisp.cpp.
void setFont | ( | uint8_t | font ) |
Sets the font.
font - available fonts: 6,10,18,51,120,123, user font 200-203
Definition at line 433 of file DigoleSerialDisp.cpp.
void setI2CAddress | ( | uint8_t | add ) |
Sets a new I2C address for the display (default is 0x27), the adapter will store the new address in memory.
- Parameters:
-
address is the the new address
Definition at line 311 of file DigoleSerialDisp.cpp.
void setLCDChip | ( | uint8_t | chip ) |
Only for universal serial adapter.
Definition at line 511 of file DigoleSerialDisp.cpp.
void setLCDColRow | ( | uint8_t | col, |
uint8_t | row | ||
) |
Configure your LCD if other than 1602 and the chip is other than KS0066U/F / HD44780.
- Parameters:
-
col is the number of columns row is the number of rows
Definition at line 303 of file DigoleSerialDisp.cpp.
void setMode | ( | uint8_t | m ) |
Set display mode.
Definition at line 480 of file DigoleSerialDisp.cpp.
void setPrintPos | ( | uint8_t | x, |
uint8_t | y, | ||
uint8_t | graph = _TEXT_ |
||
) |
Sets the print position for graphics or text.
- Parameters:
-
x is the x coordinate to display the string y is the y coordinate to display the string graph if set to _TEXT_ affects subsequent text position, otherwise, affects graphics position
Definition at line 284 of file DigoleSerialDisp.cpp.
void setTextPosBack | ( | void | ) |
set text position back to previous, only one back allowed
Definition at line 486 of file DigoleSerialDisp.cpp.
void uploadStartScreen | ( | int | lon, |
const unsigned char * | data | ||
) |
Set Start Screen, 1st B is the lower byte of data length.
Convert images to C array here: http://www.digole.com/tools/PicturetoC_Hex_converter.php
- Parameters:
-
lon is the length of data data is the binary data
Definition at line 517 of file DigoleSerialDisp.cpp.
void uploadUserFont | ( | int | lon, |
const unsigned char * | data, | ||
uint8_t | sect | ||
) |
Upload a user font.
- Parameters:
-
lon is the length of data data is the user font data sect is the section of memory you want to upload to
Definition at line 534 of file DigoleSerialDisp.cpp.
size_t write | ( | const char | x ) |
Write out a raw character.
- Parameters:
-
x is the character to write
- Returns:
- 1
Definition at line 29 of file DigoleSerialDisp.cpp.
size_t write | ( | const char * | buffer, |
size_t | size | ||
) |
Write out raw data from a buffer.
- Parameters:
-
buffer is the char array to write size is the the number of bytes to write
- Returns:
- size
Definition at line 42 of file DigoleSerialDisp.cpp.
size_t write | ( | const char * | str ) |
Write out raw string.
- Parameters:
-
str is the string to write
- Returns:
- number of bytes written
Definition at line 36 of file DigoleSerialDisp.cpp.
Generated on Tue Jul 12 2022 20:40:53 by
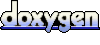