Driver for the Digole Serial universal LCD display adapter
Embed:
(wiki syntax)
Show/hide line numbers
DigoleSerialDisp.h
00001 /** Digole Serial Display library, I2C 00002 * 00003 * @Author: Digole Digital Solutions : www.digole.com ported from Arduino to mbed by Michael Shimniok www.bot-thoughts.com 00004 */ 00005 #ifndef DigoleSerialDisp_h 00006 #define DigoleSerialDisp_h 00007 00008 #include "mbed.h" 00009 #include <inttypes.h> 00010 00011 #define DEC 10 00012 #define HEX 16 00013 #define OCT 8 00014 #define BIN 2 00015 00016 #define delay(x) wait_ms(x) 00017 00018 // Communication set up command 00019 // Text function command 00020 // Graph function command 00021 00022 #define Serial_UART 0; 00023 #define Serial_I2C 1; 00024 #define Serial_SPI 2; 00025 #define _TEXT_ 0 00026 #define _GRAPH_ 1 00027 00028 /** Digole Serial LCD/OLED Library 00029 * www.digole.com/index.php?productID=535 00030 * 00031 * Includes Arduino Print class member functions 00032 */ 00033 class DigoleSerialDisp { 00034 public: 00035 00036 /** Create a new Digole Serial Display interface 00037 * 00038 * @param sda is the pin for I2C SDA 00039 * @param scl is the pin for I2C SCL 00040 * @param address is the 7-bit address (default is 0x27 for the device) 00041 */ 00042 DigoleSerialDisp(PinName sda, PinName scl, uint8_t address=0x27); 00043 00044 00045 /** Carryover from Arduino library, not needed 00046 */ 00047 void begin(void) { } // nothing to do here 00048 00049 00050 /** Write out a raw character 00051 * @param x is the character to write 00052 * @returns 1 00053 */ 00054 size_t write(const char x); 00055 00056 00057 /** Write out raw data from a buffer 00058 * @param buffer is the char array to write 00059 * @param size is the the number of bytes to write 00060 * @returns size 00061 */ 00062 size_t write(const char *buffer, size_t size); 00063 00064 00065 /** Write out raw string 00066 * @param str is the string to write 00067 * @returns number of bytes written 00068 */ 00069 size_t write(const char *str); 00070 00071 00072 /** Prints a char to the display in a single I2C transmission using "TTb\0" 00073 * 00074 * @param c is the character to print 00075 * @returns 1 00076 */ 00077 size_t print(const char c); 00078 00079 00080 /** Prints a string of data to the display in a single I2C transmission using "TTbbb...\0" 00081 * 00082 * @param s is the null-terminated char array to print 00083 * @returns length of s 00084 */ 00085 size_t print(const char s[]); 00086 00087 00088 /** Print out an unsigned char as a number 00089 * @param u is the integer to print 00090 * @param base is the base to print, either DEC (default), HEX, BIN 00091 * @returns number of chars written 00092 */ 00093 size_t print(unsigned char u, int base = DEC); 00094 00095 00096 /** Print out an integer 00097 * @param i is the integer to print 00098 * @param base is the base to print, either DEC (default), HEX, BIN 00099 * @returns number of chars written 00100 */ 00101 size_t print(int i, int base = DEC); 00102 00103 00104 /** Print out an unsigned integer 00105 * @param u is the integer to print 00106 * @param base is the base to print, either DEC (default), HEX, BIN 00107 * @returns number of chars written 00108 */ 00109 size_t print(unsigned int u, int base = DEC); 00110 00111 00112 /** Print out a long as a number 00113 * @param l is the integer to print 00114 * @param base is the base to print, either DEC (default), HEX, BIN 00115 * @returns number of chars written 00116 */ 00117 size_t print(long l, int base = DEC); 00118 00119 00120 /** Print out an unsigned long 00121 * @param l is the integer to print 00122 * @param base is the base to print, either DEC (default), HEX, BIN 00123 * @returns number of chars written 00124 */ 00125 size_t print(unsigned long l, int base = DEC); 00126 00127 00128 /** Print out a double 00129 * @param f is the integer to print 00130 * @param digits is the number of digits after the decimal 00131 */ 00132 size_t print(double f, int digits = 2); 00133 00134 00135 /** Prints a string of data to the display in a single I2C transmission using "TTbbb...\0" 00136 * 00137 * @param s is the null-terminated char array to print 00138 * @returns length of s 00139 */ 00140 size_t println(const char s[]); 00141 00142 00143 /** Prints a char the display in a single I2C transmission using "TTb\0" 00144 * 00145 * @param c is the character to print 00146 * @returns 1 00147 */ 00148 size_t println(char c); 00149 00150 00151 /** Prints an unsigned char as a number 00152 * 00153 * @param u is the unsigned char number 00154 * @returns 1 00155 */ 00156 size_t println(unsigned char u, int base = DEC); 00157 00158 00159 /** Print out an integer 00160 * @param i is the integer to print 00161 * @param base is the base to print, either DEC (default), HEX, BIN 00162 * @returns number of chars written 00163 */ 00164 size_t println(int i, int base = DEC); 00165 00166 00167 /** Print out an unsigned char as a number 00168 * @param u is the integer to print 00169 * @param base is the base to print, either DEC (default), HEX, BIN 00170 * @returns number of chars written 00171 */ 00172 size_t println(unsigned int u, int base = DEC); 00173 00174 00175 /** Print out a long as a number 00176 * @param l is the integer to print 00177 * @param base is the base to print, either DEC (default), HEX, BIN 00178 * @returns number of chars written 00179 */ 00180 size_t println(long l, int base = DEC); 00181 00182 00183 /** Print out an unsigned long 00184 * @param l is the integer to print 00185 * @param base is the base to print, either DEC (default), HEX, BIN 00186 * @returns number of chars written 00187 */ 00188 size_t println(unsigned long l, int base = DEC); 00189 00190 00191 /** Print out a double 00192 * @param f is the integer to print 00193 * @param digits is the number of digits after the decimal 00194 * @returns number of chars written 00195 */ 00196 size_t println(double f, int digits = 2); 00197 00198 00199 /** prints, well, nothing in this case, but pretend we printed a newline 00200 * @returns 1 00201 */ 00202 size_t println(void); 00203 00204 00205 /*---------functions for Text and Graphic LCD adapters---------*/ 00206 00207 /** Turns off the cursor */ 00208 void disableCursor(void); 00209 00210 /** Turns on the cursor */ 00211 void enableCursor(void); 00212 00213 /** Displays a string at specified coordinates 00214 * @param x is the x coordinate to display the string 00215 * @param y is the y coordinate to display the string 00216 * @param s is the string to display 00217 */ 00218 void drawStr(uint8_t x, uint8_t y, const char *s); 00219 00220 /** Sets the print position for graphics or text 00221 * @param x is the x coordinate to display the string 00222 * @param y is the y coordinate to display the string 00223 * @param graph if set to _TEXT_ affects subsequent text position, otherwise, affects graphics position 00224 */ 00225 void setPrintPos(uint8_t x, uint8_t y, uint8_t graph = _TEXT_); 00226 00227 /** Clears the display screen */ 00228 void clearScreen(void); 00229 00230 /** Configure your LCD if other than 1602 and the chip is other than KS0066U/F / HD44780 00231 * @param col is the number of columns 00232 * @param row is the number of rows 00233 */ 00234 void setLCDColRow(uint8_t col, uint8_t row); 00235 00236 /** Sets a new I2C address for the display (default is 0x27), the adapter will store the new address in memory 00237 * @param address is the the new address 00238 */ 00239 void setI2CAddress(uint8_t add); 00240 00241 /** Display Config on/off, the factory default set is on, 00242 * so, when the module is powered up, it will display 00243 * current communication mode on LCD, after you 00244 * design finished, you can turn it off 00245 * @param v is the 1 is on, 0 is off 00246 */ 00247 void displayConfig(uint8_t v); 00248 00249 /** Holdover from Arduino library; not needed */ 00250 void preprint(void); 00251 00252 /*----------Functions for Graphic LCD/OLED adapters only---------*/ 00253 //the functions in this section compatible with u8glib 00254 void drawBitmap(uint8_t x, uint8_t y, uint8_t w, uint8_t h, const uint8_t *bitmap); 00255 void setRot90(void); 00256 void setRot180(void); 00257 void setRot270(void); 00258 void undoRotation(void); 00259 void setRotation(uint8_t); 00260 void setContrast(uint8_t); 00261 void drawBox(uint8_t x, uint8_t y, uint8_t w, uint8_t h); 00262 void drawCircle(uint8_t x, uint8_t y, uint8_t r, uint8_t = 0); 00263 void drawDisc(uint8_t x, uint8_t y, uint8_t r); 00264 void drawFrame(uint8_t x, uint8_t y, uint8_t w, uint8_t h); 00265 void drawPixel(uint8_t x, uint8_t y, uint8_t = 1); 00266 void drawLine(uint8_t x, uint8_t y, uint8_t x1, uint8_t y1); 00267 void drawLineTo(uint8_t x, uint8_t y); 00268 void drawHLine(uint8_t x, uint8_t y, uint8_t w); 00269 void drawVLine(uint8_t x, uint8_t y, uint8_t h); 00270 //------------------------------- 00271 //special functions for our adapters 00272 00273 /** Sets the font 00274 * 00275 * @parameter font - available fonts: 6,10,18,51,120,123, user font 200-203 00276 */ 00277 void setFont(uint8_t font); 00278 00279 /** go to next text line, depending on the font size */ 00280 void nextTextLine(void); 00281 00282 /** set color for graphic function */ 00283 void setColor(uint8_t); 00284 00285 /** Turn on back light */ 00286 void backLightOn(void); 00287 00288 /** Turn off back light */ 00289 void backLightOff(void); 00290 00291 /** send command to LCD drectly 00292 * @param d - command 00293 */ 00294 void directCommand(uint8_t d); 00295 00296 /** send data to LCD drectly 00297 * @param d is the data 00298 */ 00299 void directData(uint8_t d); 00300 00301 /** Move rectangle area on screen to another place 00302 * @param x0, y1 is the top left of the area to move 00303 * @param x1, y1 is the bottom right of the area to move 00304 * @param xoffset, yoffset is the the distance to move 00305 */ 00306 void moveArea(uint8_t x0, uint8_t y0, uint8_t x1, uint8_t y1, char xoffset, char yoffset); 00307 00308 /** Display startup screen */ 00309 void displayStartScreen(uint8_t m); 00310 00311 /** Set display mode */ 00312 void setMode(uint8_t m); 00313 00314 /** set text position back to previous, only one back allowed */ 00315 void setTextPosBack(void); 00316 00317 void setTextPosOffset(char xoffset, char yoffset); 00318 void setTextPosAbs(uint8_t x, uint8_t y); 00319 void setLinePattern(uint8_t pattern); 00320 /** Only for universal serial adapter */ 00321 void setLCDChip(uint8_t chip); 00322 00323 00324 /** Set Start Screen, 1st B is the lower byte of data length. 00325 * Convert images to C array here: <a href="http://www.digole.com/tools/PicturetoC_Hex_converter.php">http://www.digole.com/tools/PicturetoC_Hex_converter.php</a> 00326 * @param lon is the length of data 00327 * @param data is the binary data 00328 */ 00329 void uploadStartScreen(int lon, const unsigned char *data); //upload start screen 00330 00331 /** Upload a user font 00332 * @param lon is the length of data 00333 * @param data is the user font data 00334 * @param sect is the section of memory you want to upload to 00335 */ 00336 void uploadUserFont(int lon, const unsigned char *data, uint8_t sect); //upload user font 00337 00338 /** Send a Byte to output head on board 00339 * @param x is the byte to output 00340 */ 00341 void digitalOutput(uint8_t x); 00342 00343 private: 00344 I2C _device; 00345 uint8_t _address; 00346 uint8_t _Comdelay; 00347 char buf[128]; 00348 00349 size_t printNumber(unsigned long n, uint8_t base); 00350 size_t printFloat(double number, uint8_t digits); 00351 }; 00352 00353 #endif
Generated on Tue Jul 12 2022 20:40:53 by
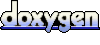