Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
CAN Class Reference
A can bus client, used for communicating with can devices. More...
#include <CAN.h>
Inherits mbed::Base.
Public Member Functions | |
CAN (PinName rd, PinName td) | |
Creates an CAN interface connected to specific pins. | |
int | frequency (int hz) |
Set the frequency of the CAN interface. | |
int | write (CANMessage msg) |
Write a CANMessage to the bus. | |
int | read (CANMessage &msg) |
Read a CANMessage from the bus. | |
void | reset () |
Reset CAN interface. | |
void | monitor (bool silent) |
Puts or removes the CAN interface into silent monitoring mode. | |
unsigned char | rderror () |
Returns number of read errors to detect read overflow errors. | |
unsigned char | tderror () |
Returns number of write errors to detect write overflow errors. | |
void | attach (void(*fptr)(void)) |
Attach a function to call whenever a CAN frame received interrupt is generated. | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(void)) |
Attach a member function to call whenever a CAN frame received interrupt is generated. | |
void | register_object (const char *name) |
Registers this object with the given name, so that it can be looked up with lookup. | |
const char * | name () |
Returns the name of the object. | |
virtual bool | rpc (const char *method, const char *arguments, char *result) |
Call the given method with the given arguments, and write the result into the string pointed to by result. | |
virtual struct rpc_method * | get_rpc_methods () |
Returns a pointer to an array describing the rpc methods supported by this object, terminated by either RPC_METHOD_END or RPC_METHOD_SUPER(Superclass). | |
Static Public Member Functions | |
static bool | rpc (const char *name, const char *method, const char *arguments, char *result) |
Use the lookup function to lookup an object and, if successful, call its rpc method. | |
static Base * | lookup (const char *name, unsigned int len) |
Lookup and return the object that has the given name. | |
template<class C > | |
static void | add_rpc_class () |
Add the class to the list of classes which can have static methods called via rpc (the static methods which can be called are defined by that class' get_rpc_class() static method). |
Detailed Description
A can bus client, used for communicating with can devices.
Definition at line 99 of file CAN.h.
Constructor & Destructor Documentation
CAN | ( | PinName | rd, |
PinName | td | ||
) |
Creates an CAN interface connected to specific pins.
- Parameters:
-
rd read from transmitter td transmit to transmitter
Example:
#include "mbed.h" Ticker ticker; DigitalOut led1(LED1); DigitalOut led2(LED2); CAN can1(p9, p10); CAN can2(p30, p29); char counter = 0; void send() { if(can1.write(CANMessage(1337, &counter, 1))) { printf("Message sent: %d\n", counter); counter++; } led1 = !led1; } int main() { ticker.attach(&send, 1); CANMessage msg; while(1) { if(can2.read(msg)) { printf("Message received: %d\n\n", msg.data[0]); led2 = !led2; } wait(0.2); } }
Member Function Documentation
static void add_rpc_class | ( | ) | [static, inherited] |
void attach | ( | void(*)(void) | fptr ) |
Attach a function to call whenever a CAN frame received interrupt is generated.
- Parameters:
-
fptr A pointer to a void function, or 0 to set as none
void attach | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) |
int frequency | ( | int | hz ) |
Set the frequency of the CAN interface.
- Parameters:
-
hz The bus frequency in hertz
- Returns:
- 1 if successful, 0 otherwise
virtual struct rpc_method* get_rpc_methods | ( | ) | [read, virtual, inherited] |
Returns a pointer to an array describing the rpc methods supported by this object, terminated by either RPC_METHOD_END or RPC_METHOD_SUPER(Superclass).
Example
class Example : public Base { int foo(int a, int b) { return a + b; } virtual const struct rpc_method *get_rpc_methods() { static const rpc_method rpc_methods[] = { { "foo", generic_caller<int, Example, int, int, &Example::foo> }, RPC_METHOD_SUPER(Base) }; return rpc_methods; } };
Reimplemented in AnalogIn, AnalogOut, BusIn, BusInOut, BusOut, DigitalIn, DigitalInOut, DigitalOut, PwmOut, Serial, SPI, and Timer.
static Base* lookup | ( | const char * | name, |
unsigned int | len | ||
) | [static, inherited] |
Lookup and return the object that has the given name.
- Parameters:
-
name the name to lookup. len the length of name.
void monitor | ( | bool | silent ) |
Puts or removes the CAN interface into silent monitoring mode.
- Parameters:
-
silent boolean indicating whether to go into silent mode or not
const char* name | ( | ) | [inherited] |
Returns the name of the object.
- Returns:
- The name of the object, or NULL if it has no name.
unsigned char rderror | ( | ) |
Returns number of read errors to detect read overflow errors.
int read | ( | CANMessage & | msg ) |
Read a CANMessage from the bus.
- Parameters:
-
msg A CANMessage to read to.
- Returns:
- 0 if no message arrived, 1 if message arrived
void register_object | ( | const char * | name ) | [inherited] |
Registers this object with the given name, so that it can be looked up with lookup.
If this object has already been registered, then this just changes the name.
- Parameters:
-
name The name to give the object. If NULL we do nothing.
void reset | ( | ) |
Reset CAN interface.
To use after error overflow.
static bool rpc | ( | const char * | name, |
const char * | method, | ||
const char * | arguments, | ||
char * | result | ||
) | [static, inherited] |
Use the lookup function to lookup an object and, if successful, call its rpc method.
- Returns:
- false if name does not correspond to an object, otherwise the return value of the call to the object's rpc method.
virtual bool rpc | ( | const char * | method, |
const char * | arguments, | ||
char * | result | ||
) | [virtual, inherited] |
Call the given method with the given arguments, and write the result into the string pointed to by result.
The default implementation calls rpc_methods to determine the supported methods.
- Parameters:
-
method The name of the method to call. arguments A list of arguments separated by spaces. result A pointer to a string to write the result into. May be NULL, in which case nothing is written.
- Returns:
- true if method corresponds to a valid rpc method, or false otherwise.
unsigned char tderror | ( | ) |
Returns number of write errors to detect write overflow errors.
int write | ( | CANMessage | msg ) |
Write a CANMessage to the bus.
- Parameters:
-
msg The CANMessage to write.
- Returns:
- 0 if write failed, 1 if write was successful
Generated on Tue Jul 12 2022 11:27:31 by
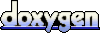