Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
SPI Class Reference
A SPI Master, used for communicating with SPI slave devices. More...
#include <SPI.h>
Inherits mbed::Base.
Inherited by SPIHalfDuplex.
Public Member Functions | |
SPI (PinName mosi, PinName miso, PinName sclk, const char *name=NULL) | |
Create a SPI master connected to the specified pins. | |
void | format (int bits, int mode=0) |
Configure the data transmission format. | |
void | frequency (int hz=1000000) |
Set the spi bus clock frequency. | |
virtual int | write (int value) |
Write to the SPI Slave and return the response. | |
virtual struct rpc_method * | get_rpc_methods () |
Returns a pointer to an array describing the rpc methods supported by this object, terminated by either RPC_METHOD_END or RPC_METHOD_SUPER(Superclass). | |
void | register_object (const char *name) |
Registers this object with the given name, so that it can be looked up with lookup. | |
const char * | name () |
Returns the name of the object. | |
virtual bool | rpc (const char *method, const char *arguments, char *result) |
Call the given method with the given arguments, and write the result into the string pointed to by result. | |
Static Public Member Functions | |
static bool | rpc (const char *name, const char *method, const char *arguments, char *result) |
Use the lookup function to lookup an object and, if successful, call its rpc method. | |
static Base * | lookup (const char *name, unsigned int len) |
Lookup and return the object that has the given name. | |
template<class C > | |
static void | add_rpc_class () |
Add the class to the list of classes which can have static methods called via rpc (the static methods which can be called are defined by that class' get_rpc_class() static method). |
Detailed Description
A SPI Master, used for communicating with SPI slave devices.
The default format is set to 8-bits, mode 0, and a clock frequency of 1MHz
Most SPI devices will also require Chip Select and Reset signals. These can be controlled using <DigitalOut> pins
Example:
// Send a byte to a SPI slave, and record the response #include "mbed.h" SPI device(p5, p6, p7); // mosi, miso, sclk int main() { int response = device.write(0xFF); }
Definition at line 39 of file SPI.h.
Constructor & Destructor Documentation
SPI | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
const char * | name = NULL |
||
) |
Member Function Documentation
static void add_rpc_class | ( | ) | [static, inherited] |
void format | ( | int | bits, |
int | mode = 0 |
||
) |
Configure the data transmission format.
- Parameters:
-
bits Number of bits per SPI frame (4 - 16) mode Clock polarity and phase mode (0 - 3)
mode | POL PHA -----+-------- 0 | 0 0 1 | 0 1 2 | 1 0 3 | 1 1
Reimplemented in SPIHalfDuplex.
void frequency | ( | int | hz = 1000000 ) |
Set the spi bus clock frequency.
- Parameters:
-
hz SCLK frequency in hz (default = 1MHz)
Reimplemented in SPIHalfDuplex.
virtual struct rpc_method* get_rpc_methods | ( | ) | [read, virtual] |
Returns a pointer to an array describing the rpc methods supported by this object, terminated by either RPC_METHOD_END or RPC_METHOD_SUPER(Superclass).
Example
class Example : public Base { int foo(int a, int b) { return a + b; } virtual const struct rpc_method *get_rpc_methods() { static const rpc_method rpc_methods[] = { { "foo", generic_caller<int, Example, int, int, &Example::foo> }, RPC_METHOD_SUPER(Base) }; return rpc_methods; } };
Reimplemented from Base.
static Base* lookup | ( | const char * | name, |
unsigned int | len | ||
) | [static, inherited] |
Lookup and return the object that has the given name.
- Parameters:
-
name the name to lookup. len the length of name.
const char* name | ( | ) | [inherited] |
Returns the name of the object.
- Returns:
- The name of the object, or NULL if it has no name.
void register_object | ( | const char * | name ) | [inherited] |
Registers this object with the given name, so that it can be looked up with lookup.
If this object has already been registered, then this just changes the name.
- Parameters:
-
name The name to give the object. If NULL we do nothing.
static bool rpc | ( | const char * | name, |
const char * | method, | ||
const char * | arguments, | ||
char * | result | ||
) | [static, inherited] |
Use the lookup function to lookup an object and, if successful, call its rpc method.
- Returns:
- false if name does not correspond to an object, otherwise the return value of the call to the object's rpc method.
virtual bool rpc | ( | const char * | method, |
const char * | arguments, | ||
char * | result | ||
) | [virtual, inherited] |
Call the given method with the given arguments, and write the result into the string pointed to by result.
The default implementation calls rpc_methods to determine the supported methods.
- Parameters:
-
method The name of the method to call. arguments A list of arguments separated by spaces. result A pointer to a string to write the result into. May be NULL, in which case nothing is written.
- Returns:
- true if method corresponds to a valid rpc method, or false otherwise.
virtual int write | ( | int | value ) | [virtual] |
Write to the SPI Slave and return the response.
- Parameters:
-
value Data to be sent to the SPI slave
- Returns:
- Response from the SPI slave
Reimplemented in SPIHalfDuplex.
Generated on Tue Jul 12 2022 11:27:31 by
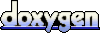