Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
CAN.h
00001 /* mbed Microcontroller Library - can 00002 * Copyright (c) 2009-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_CAN_H 00006 #define MBED_CAN_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_CAN 00011 00012 #include "Base.h" 00013 #include "platform.h" 00014 #include "PinNames.h" 00015 #include "PeripheralNames.h" 00016 00017 #include "can_helper.h" 00018 #include "FunctionPointer.h" 00019 00020 #include <string.h> 00021 00022 namespace mbed { 00023 00024 /** CANMessage class 00025 */ 00026 class CANMessage : public CAN_Message { 00027 00028 public: 00029 00030 /** Creates empty CAN message. 00031 */ 00032 CANMessage() { 00033 len = 8; 00034 type = CANData; 00035 format = CANStandard; 00036 id = 0; 00037 memset(data, 0, 8); 00038 } 00039 00040 /** Creates CAN message with specific content. 00041 */ 00042 CANMessage(int _id, const char *_data, char _len = 8, CANType _type = CANData, CANFormat _format = CANStandard) { 00043 len = _len & 0xF; 00044 type = _type; 00045 format = _format; 00046 id = _id; 00047 memcpy(data, _data, _len); 00048 } 00049 00050 /** Creates CAN remote message. 00051 */ 00052 CANMessage(int _id, CANFormat _format = CANStandard) { 00053 len = 0; 00054 type = CANRemote; 00055 format = _format; 00056 id = _id; 00057 memset(data, 0, 8); 00058 } 00059 #if 0 // Inhereted from CAN_Message, for documentation only 00060 00061 /** The message id. 00062 * 00063 * - If format is CANStandard it must be an 11 bit long id. 00064 * - If format is CANExtended it must be an 29 bit long id. 00065 */ 00066 unsigned int id; 00067 00068 /** Space for 8 byte payload. 00069 * 00070 * If type is CANData data can store up to 8 byte data. 00071 */ 00072 unsigned char data[8]; 00073 00074 /** Length of data in bytes. 00075 * 00076 * If type is CANData data can store up to 8 byte data. 00077 */ 00078 unsigned char len; 00079 00080 /** Defines if the message has standard or extended format. 00081 * 00082 * Defines the type of message id: 00083 * Default is CANStandard which implies 11 bit id. 00084 * CANExtended means 29 bit message id. 00085 */ 00086 CANFormat format; 00087 00088 /** Defines the type of a message. 00089 * 00090 * The message type can rather be CANData for a message with data (default). 00091 * Or CANRemote for a request of a specific CAN message. 00092 */ 00093 CANType type; // 0 - DATA FRAME, 1 - REMOTE FRAME 00094 #endif 00095 }; 00096 00097 /** A can bus client, used for communicating with can devices 00098 */ 00099 class CAN : public Base { 00100 00101 public: 00102 00103 /** Creates an CAN interface connected to specific pins. 00104 * 00105 * @param rd read from transmitter 00106 * @param td transmit to transmitter 00107 * 00108 * Example: 00109 * @code 00110 * #include "mbed.h" 00111 * 00112 * Ticker ticker; 00113 * DigitalOut led1(LED1); 00114 * DigitalOut led2(LED2); 00115 * CAN can1(p9, p10); 00116 * CAN can2(p30, p29); 00117 * 00118 * char counter = 0; 00119 * 00120 * void send() { 00121 * if(can1.write(CANMessage(1337, &counter, 1))) { 00122 * printf("Message sent: %d\n", counter); 00123 * counter++; 00124 * } 00125 * led1 = !led1; 00126 * } 00127 * 00128 * int main() { 00129 * ticker.attach(&send, 1); 00130 * CANMessage msg; 00131 * while(1) { 00132 * if(can2.read(msg)) { 00133 * printf("Message received: %d\n\n", msg.data[0]); 00134 * led2 = !led2; 00135 * } 00136 * wait(0.2); 00137 * } 00138 * } 00139 * @endcode 00140 */ 00141 CAN(PinName rd, PinName td); 00142 virtual ~CAN(); 00143 00144 /** Set the frequency of the CAN interface 00145 * 00146 * @param hz The bus frequency in hertz 00147 * 00148 * @returns 00149 * 1 if successful, 00150 * 0 otherwise 00151 */ 00152 int frequency(int hz); 00153 00154 /** Write a CANMessage to the bus. 00155 * 00156 * @param msg The CANMessage to write. 00157 * 00158 * @returns 00159 * 0 if write failed, 00160 * 1 if write was successful 00161 */ 00162 int write(CANMessage msg); 00163 00164 /** Read a CANMessage from the bus. 00165 * 00166 * @param msg A CANMessage to read to. 00167 * 00168 * @returns 00169 * 0 if no message arrived, 00170 * 1 if message arrived 00171 */ 00172 int read(CANMessage &msg); 00173 00174 /** Reset CAN interface. 00175 * 00176 * To use after error overflow. 00177 */ 00178 void reset(); 00179 00180 /** Puts or removes the CAN interface into silent monitoring mode 00181 * 00182 * @param silent boolean indicating whether to go into silent mode or not 00183 */ 00184 void monitor(bool silent); 00185 00186 /** Returns number of read errors to detect read overflow errors. 00187 */ 00188 unsigned char rderror(); 00189 00190 /** Returns number of write errors to detect write overflow errors. 00191 */ 00192 unsigned char tderror(); 00193 00194 /** Attach a function to call whenever a CAN frame received interrupt is 00195 * generated. 00196 * 00197 * @param fptr A pointer to a void function, or 0 to set as none 00198 */ 00199 void attach(void (*fptr)(void)); 00200 00201 /** Attach a member function to call whenever a CAN frame received interrupt 00202 * is generated. 00203 * 00204 * @param tptr pointer to the object to call the member function on 00205 * @param mptr pointer to the member function to be called 00206 */ 00207 template<typename T> 00208 void attach(T* tptr, void (T::*mptr)(void)) { 00209 if((mptr != NULL) && (tptr != NULL)) { 00210 _rxirq.attach(tptr, mptr); 00211 setup_interrupt(); 00212 } else { 00213 remove_interrupt(); 00214 } 00215 } 00216 00217 private: 00218 00219 CANName _id; 00220 FunctionPointer _rxirq; 00221 00222 void setup_interrupt(void); 00223 void remove_interrupt(void); 00224 }; 00225 00226 } // namespace mbed 00227 00228 #endif // MBED_CAN_H 00229 00230 #endif
Generated on Tue Jul 12 2022 11:27:26 by
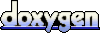