Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
Base.h
00001 /* mbed Microcontroller Library - Base 00002 * Copyright (c) 2006-2008 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_BASE_H 00006 #define MBED_BASE_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include <cstdlib> 00012 #include "DirHandle.h" 00013 00014 namespace mbed { 00015 00016 #ifdef MBED_RPC 00017 struct rpc_function { 00018 const char *name; 00019 void (*caller)(const char*, char*); 00020 }; 00021 00022 struct rpc_class { 00023 const char *name; 00024 const rpc_function *static_functions; 00025 struct rpc_class *next; 00026 }; 00027 #endif 00028 00029 /** The base class for most things 00030 */ 00031 class Base { 00032 00033 public: 00034 00035 Base(const char *name = NULL); 00036 00037 virtual ~Base(); 00038 00039 /** Registers this object with the given name, so that it can be 00040 * looked up with lookup. If this object has already been 00041 * registered, then this just changes the name. 00042 * 00043 * @param name The name to give the object. If NULL we do nothing. 00044 */ 00045 void register_object(const char *name); 00046 00047 /** Returns the name of the object 00048 * 00049 * @returns 00050 * The name of the object, or NULL if it has no name. 00051 */ 00052 const char *name(); 00053 00054 #ifdef MBED_RPC 00055 00056 /** Call the given method with the given arguments, and write the 00057 * result into the string pointed to by result. The default 00058 * implementation calls rpc_methods to determine the supported 00059 * methods. 00060 * 00061 * @param method The name of the method to call. 00062 * @param arguments A list of arguments separated by spaces. 00063 * @param result A pointer to a string to write the result into. May be NULL, in which case nothing is written. 00064 * 00065 * @returns 00066 * true if method corresponds to a valid rpc method, or 00067 * false otherwise. 00068 */ 00069 virtual bool rpc(const char *method, const char *arguments, char *result); 00070 00071 /** Returns a pointer to an array describing the rpc methods 00072 * supported by this object, terminated by either 00073 * RPC_METHOD_END or RPC_METHOD_SUPER(Superclass). 00074 * 00075 * Example 00076 * @code 00077 * class Example : public Base { 00078 * int foo(int a, int b) { return a + b; } 00079 * virtual const struct rpc_method *get_rpc_methods() { 00080 * static const rpc_method rpc_methods[] = { 00081 * { "foo", generic_caller<int, Example, int, int, &Example::foo> }, 00082 * RPC_METHOD_SUPER(Base) 00083 * }; 00084 * return rpc_methods; 00085 * } 00086 * }; 00087 * @endcode 00088 */ 00089 virtual const struct rpc_method *get_rpc_methods(); 00090 00091 /** Use the lookup function to lookup an object and, if 00092 * successful, call its rpc method 00093 * 00094 * @returns 00095 * false if name does not correspond to an object, 00096 * otherwise the return value of the call to the object's rpc 00097 * method. 00098 */ 00099 static bool rpc(const char *name, const char *method, const char *arguments, char *result); 00100 00101 #endif 00102 00103 /** Lookup and return the object that has the given name. 00104 * 00105 * @param name the name to lookup. 00106 * @param len the length of name. 00107 */ 00108 static Base *lookup(const char *name, unsigned int len); 00109 00110 static DirHandle *opendir(); 00111 friend class BaseDirHandle; 00112 00113 protected: 00114 00115 static Base *_head; 00116 Base *_next; 00117 const char *_name; 00118 bool _from_construct; 00119 00120 private: 00121 00122 #ifdef MBED_RPC 00123 static rpc_class *_classes; 00124 00125 static const rpc_function _base_funcs[]; 00126 static rpc_class _base_class; 00127 #endif 00128 00129 void delete_self(); 00130 static void list_objs(const char *arguments, char *result); 00131 static void clear(const char*,char*); 00132 00133 static char *new_name(Base *p); 00134 00135 public: 00136 00137 #ifdef MBED_RPC 00138 /** Add the class to the list of classes which can have static 00139 * methods called via rpc (the static methods which can be called 00140 * are defined by that class' get_rpc_class() static method). 00141 */ 00142 template<class C> 00143 static void add_rpc_class() { 00144 rpc_class *c = C::get_rpc_class(); 00145 c->next = _classes; 00146 _classes = c; 00147 } 00148 00149 template<class C> 00150 static const char *construct() { 00151 Base *p = new C(); 00152 p->_from_construct = true; 00153 if(p->_name==NULL) { 00154 p->register_object(new_name(p)); 00155 } 00156 return p->_name; 00157 } 00158 00159 template<class C, typename A1> 00160 static const char *construct(A1 arg1) { 00161 Base *p = new C(arg1); 00162 p->_from_construct = true; 00163 if(p->_name==NULL) { 00164 p->register_object(new_name(p)); 00165 } 00166 return p->_name; 00167 } 00168 00169 template<class C, typename A1, typename A2> 00170 static const char *construct(A1 arg1, A2 arg2) { 00171 Base *p = new C(arg1,arg2); 00172 p->_from_construct = true; 00173 if(p->_name==NULL) { 00174 p->register_object(new_name(p)); 00175 } 00176 return p->_name; 00177 } 00178 00179 template<class C, typename A1, typename A2, typename A3> 00180 static const char *construct(A1 arg1, A2 arg2, A3 arg3) { 00181 Base *p = new C(arg1,arg2,arg3); 00182 p->_from_construct = true; 00183 if(p->_name==NULL) { 00184 p->register_object(new_name(p)); 00185 } 00186 return p->_name; 00187 } 00188 00189 template<class C, typename A1, typename A2, typename A3, typename A4> 00190 static const char *construct(A1 arg1, A2 arg2, A3 arg3, A4 arg4) { 00191 Base *p = new C(arg1,arg2,arg3,arg4); 00192 p->_from_construct = true; 00193 if(p->_name==NULL) { 00194 p->register_object(new_name(p)); 00195 } 00196 return p->_name; 00197 } 00198 #endif 00199 00200 }; 00201 00202 /** The maximum size of object name (including terminating null byte) 00203 * that will be recognised when using fopen to open a FileLike 00204 * object, or when using the rpc function. 00205 */ 00206 #define MBED_OBJECT_NAME_MAX 32 00207 00208 /** The maximum size of rpc method name (including terminating null 00209 * byte) that will be recognised by the rpc function (in rpc.h). 00210 */ 00211 #define MBED_METHOD_NAME_MAX 32 00212 00213 } // namespace mbed 00214 00215 #endif 00216
Generated on Tue Jul 12 2022 11:27:25 by
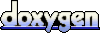