Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
Serial Class Reference
A serial port (UART) for communication with other serial devices. More...
#include <Serial.h>
Inherits mbed::Stream.
Inherited by SerialHalfDuplex.
Public Member Functions | |
Serial (PinName tx, PinName rx, const char *name=NULL) | |
Create a Serial port, connected to the specified transmit and receive pins. | |
void | baud (int baudrate) |
Set the baud rate of the serial port. | |
void | format (int bits=8, Parity parity=Serial::None, int stop_bits=1) |
Set the transmission format used by the Serial port. | |
int | putc (int c) |
Write a character. | |
int | getc () |
Reads a character from the serial port. | |
int | printf (const char *format,...) |
Write a formated string. | |
int | scanf (const char *format,...) |
Read a formated string. | |
int | readable () |
Determine if there is a character available to read. | |
int | writeable () |
Determine if there is space available to write a character. | |
void | attach (void(*fptr)(void), IrqType type=RxIrq) |
Attach a function to call whenever a serial interrupt is generated. | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(void), IrqType type=RxIrq) |
Attach a member function to call whenever a serial interrupt is generated. | |
virtual struct rpc_method * | get_rpc_methods () |
Returns a pointer to an array describing the rpc methods supported by this object, terminated by either RPC_METHOD_END or RPC_METHOD_SUPER(Superclass). | |
void | register_object (const char *name) |
Registers this object with the given name, so that it can be looked up with lookup. | |
const char * | name () |
Returns the name of the object. | |
virtual bool | rpc (const char *method, const char *arguments, char *result) |
Call the given method with the given arguments, and write the result into the string pointed to by result. | |
Static Public Member Functions | |
static bool | rpc (const char *name, const char *method, const char *arguments, char *result) |
Use the lookup function to lookup an object and, if successful, call its rpc method. | |
static Base * | lookup (const char *name, unsigned int len) |
Lookup and return the object that has the given name. | |
template<class C > | |
static void | add_rpc_class () |
Add the class to the list of classes which can have static methods called via rpc (the static methods which can be called are defined by that class' get_rpc_class() static method). |
Detailed Description
A serial port (UART) for communication with other serial devices.
Can be used for Full Duplex communication, or Simplex by specifying one pin as NC (Not Connected)
Example:
// Print "Hello World" to the PC #include "mbed.h" Serial pc(USBTX, USBRX); int main() { pc.printf("Hello World\n"); }
Definition at line 38 of file Serial.h.
Constructor & Destructor Documentation
Serial | ( | PinName | tx, |
PinName | rx, | ||
const char * | name = NULL |
||
) |
Create a Serial port, connected to the specified transmit and receive pins.
- Parameters:
-
tx Transmit pin rx Receive pin
- Note:
- Either tx or rx may be specified as NC if unused
Member Function Documentation
static void add_rpc_class | ( | ) | [static, inherited] |
void attach | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
IrqType | type = RxIrq |
||
) |
Attach a member function to call whenever a serial interrupt is generated.
- Parameters:
-
tptr pointer to the object to call the member function on mptr pointer to the member function to be called type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
void attach | ( | void(*)(void) | fptr, |
IrqType | type = RxIrq |
||
) |
Attach a function to call whenever a serial interrupt is generated.
- Parameters:
-
fptr A pointer to a void function, or 0 to set as none type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
void baud | ( | int | baudrate ) |
Set the baud rate of the serial port.
- Parameters:
-
baudrate The baudrate of the serial port (default = 9600).
Reimplemented in SerialHalfDuplex.
void format | ( | int | bits = 8 , |
Parity | parity = Serial::None , |
||
int | stop_bits = 1 |
||
) |
Set the transmission format used by the Serial port.
- Parameters:
-
bits The number of bits in a word (5-8; default = 8) parity The parity used (Serial::None, Serial::Odd, Serial::Even, Serial::Forced1, Serial::Forced0; default = Serial::None) stop The number of stop bits (1 or 2; default = 1)
Reimplemented in SerialHalfDuplex.
virtual struct rpc_method* get_rpc_methods | ( | ) | [read, virtual] |
Returns a pointer to an array describing the rpc methods supported by this object, terminated by either RPC_METHOD_END or RPC_METHOD_SUPER(Superclass).
Example
class Example : public Base { int foo(int a, int b) { return a + b; } virtual const struct rpc_method *get_rpc_methods() { static const rpc_method rpc_methods[] = { { "foo", generic_caller<int, Example, int, int, &Example::foo> }, RPC_METHOD_SUPER(Base) }; return rpc_methods; } };
Reimplemented from Base.
int getc | ( | ) |
Reads a character from the serial port.
This will block until a character is available. To see if a character is available, see readable()
- Returns:
- The character read from the serial port
Reimplemented in SerialHalfDuplex.
static Base* lookup | ( | const char * | name, |
unsigned int | len | ||
) | [static, inherited] |
Lookup and return the object that has the given name.
- Parameters:
-
name the name to lookup. len the length of name.
const char* name | ( | ) | [inherited] |
Returns the name of the object.
- Returns:
- The name of the object, or NULL if it has no name.
int printf | ( | const char * | format, |
... | |||
) |
Write a formated string.
- Parameters:
-
format A printf-style format string, followed by the variables to use in formating the string.
Reimplemented in SerialHalfDuplex.
int putc | ( | int | c ) |
Write a character.
- Parameters:
-
c The character to write to the serial port
Reimplemented in SerialHalfDuplex.
int readable | ( | ) |
Determine if there is a character available to read.
- Returns:
- 1 if there is a character available to read, 0 otherwise
Reimplemented in SerialHalfDuplex.
void register_object | ( | const char * | name ) | [inherited] |
Registers this object with the given name, so that it can be looked up with lookup.
If this object has already been registered, then this just changes the name.
- Parameters:
-
name The name to give the object. If NULL we do nothing.
virtual bool rpc | ( | const char * | method, |
const char * | arguments, | ||
char * | result | ||
) | [virtual, inherited] |
Call the given method with the given arguments, and write the result into the string pointed to by result.
The default implementation calls rpc_methods to determine the supported methods.
- Parameters:
-
method The name of the method to call. arguments A list of arguments separated by spaces. result A pointer to a string to write the result into. May be NULL, in which case nothing is written.
- Returns:
- true if method corresponds to a valid rpc method, or false otherwise.
static bool rpc | ( | const char * | name, |
const char * | method, | ||
const char * | arguments, | ||
char * | result | ||
) | [static, inherited] |
Use the lookup function to lookup an object and, if successful, call its rpc method.
- Returns:
- false if name does not correspond to an object, otherwise the return value of the call to the object's rpc method.
int scanf | ( | const char * | format, |
... | |||
) |
Read a formated string.
- Parameters:
-
format A scanf-style format string, followed by the pointers to variables to store the results.
Reimplemented in SerialHalfDuplex.
int writeable | ( | ) |
Determine if there is space available to write a character.
- Returns:
- 1 if there is space to write a character, 0 otherwise
Reimplemented in SerialHalfDuplex.
Generated on Tue Jul 12 2022 11:27:31 by
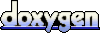