XBee-mbed library http://mbed.org/users/okini3939/notebook/xbee-mbed/
Dependents: device_server_udp led_sender_post XBee_API_ex1 XBee_API_ex2 ... more
Rx64Response Class Reference
Represents a Series 1 64-bit address RX packet. More...
#include <XBee.h>
Inherits RxResponse.
Public Member Functions | |
virtual uint16_t | getDataLength () |
Returns the length of the payload. | |
virtual uint8_t | getDataOffset () |
Returns the position in the frame data where the data begins. | |
uint8_t | getData (int index) |
Returns the specified index of the payload. | |
uint8_t * | getData () |
Returns the payload array. | |
uint8_t | getApiId () |
Returns Api Id of the response. | |
uint8_t | getMsbLength () |
Returns the MSB length of the packet. | |
uint8_t | getLsbLength () |
Returns the LSB length of the packet. | |
uint8_t | getChecksum () |
Returns the packet checksum. | |
uint16_t | getFrameDataLength () |
Returns the length of the frame data: all bytes after the api id, and prior to the checksum Note up to release 0.1.2, this was incorrectly including the checksum in the length. | |
uint8_t * | getFrameData () |
Returns the buffer that contains the response. | |
uint16_t | getPacketLength () |
Returns the length of the packet. | |
void | reset () |
Resets the response to default values. | |
void | init () |
Initializes the response. | |
void | getZBTxStatusResponse (XBeeResponse &response) |
Call with instance of ZBTxStatusResponse class only if getApiId() == ZB_TX_STATUS_RESPONSE to populate response. | |
void | getZBRxResponse (XBeeResponse &response) |
Call with instance of ZBRxResponse class only if getApiId() == ZB_RX_RESPONSE to populate response. | |
void | getZBRxIoSampleResponse (XBeeResponse &response) |
Call with instance of ZBRxIoSampleResponse class only if getApiId() == ZB_IO_SAMPLE_RESPONSE to populate response. | |
void | getTxStatusResponse (XBeeResponse &response) |
Call with instance of TxStatusResponse only if getApiId() == TX_STATUS_RESPONSE. | |
void | getRx16Response (XBeeResponse &response) |
Call with instance of Rx16Response only if getApiId() == RX_16_RESPONSE. | |
void | getRx64Response (XBeeResponse &response) |
Call with instance of Rx64Response only if getApiId() == RX_64_RESPONSE. | |
void | getRx16IoSampleResponse (XBeeResponse &response) |
Call with instance of Rx16IoSampleResponse only if getApiId() == RX_16_IO_RESPONSE. | |
void | getRx64IoSampleResponse (XBeeResponse &response) |
Call with instance of Rx64IoSampleResponse only if getApiId() == RX_64_IO_RESPONSE. | |
void | getAtCommandResponse (XBeeResponse &responses) |
Call with instance of AtCommandResponse only if getApiId() == AT_COMMAND_RESPONSE. | |
void | getRemoteAtCommandResponse (XBeeResponse &response) |
Call with instance of RemoteAtCommandResponse only if getApiId() == REMOTE_AT_COMMAND_RESPONSE. | |
void | getModemStatusResponse (XBeeResponse &response) |
Call with instance of ModemStatusResponse only if getApiId() == MODEM_STATUS_RESPONSE. | |
bool | isAvailable () |
Returns true if the response has been successfully parsed and is complete and ready for use. | |
bool | isError () |
Returns true if the response contains errors. | |
uint8_t | getErrorCode () |
Returns an error code, or zero, if successful. |
Detailed Description
Represents a Series 1 64-bit address RX packet.
Definition at line 472 of file XBee.h.
Member Function Documentation
uint8_t getApiId | ( | ) | [inherited] |
void getAtCommandResponse | ( | XBeeResponse & | responses ) | [inherited] |
Call with instance of AtCommandResponse only if getApiId() == AT_COMMAND_RESPONSE.
uint8_t getChecksum | ( | ) | [inherited] |
uint8_t getData | ( | int | index ) | [inherited] |
Returns the specified index of the payload.
The index may be 0 to getDataLength() - 1 This method is deprecated; use uint8_t* getData()
uint8_t * getData | ( | ) | [inherited] |
Returns the payload array.
This may be accessed from index 0 to getDataLength() - 1
uint16_t getDataLength | ( | ) | [virtual, inherited] |
Returns the length of the payload.
Implements RxDataResponse.
uint8_t getDataOffset | ( | ) | [virtual, inherited] |
Returns the position in the frame data where the data begins.
Implements RxDataResponse.
uint8_t getErrorCode | ( | ) | [inherited] |
uint8_t * getFrameData | ( | ) | [inherited] |
Returns the buffer that contains the response.
Starts with byte that follows API ID and includes all bytes prior to the checksum Length is specified by getFrameDataLength() Note: Unlike Digi's definition of the frame data, this does not start with the API ID.. The reason for this is all responses include an API ID, whereas my frame data includes only the API specific data.
uint16_t getFrameDataLength | ( | ) | [inherited] |
uint8_t getLsbLength | ( | ) | [inherited] |
void getModemStatusResponse | ( | XBeeResponse & | response ) | [inherited] |
Call with instance of ModemStatusResponse only if getApiId() == MODEM_STATUS_RESPONSE.
uint8_t getMsbLength | ( | ) | [inherited] |
uint16_t getPacketLength | ( | ) | [inherited] |
void getRemoteAtCommandResponse | ( | XBeeResponse & | response ) | [inherited] |
Call with instance of RemoteAtCommandResponse only if getApiId() == REMOTE_AT_COMMAND_RESPONSE.
void getRx16IoSampleResponse | ( | XBeeResponse & | response ) | [inherited] |
Call with instance of Rx16IoSampleResponse only if getApiId() == RX_16_IO_RESPONSE.
void getRx16Response | ( | XBeeResponse & | response ) | [inherited] |
Call with instance of Rx16Response only if getApiId() == RX_16_RESPONSE.
void getRx64IoSampleResponse | ( | XBeeResponse & | response ) | [inherited] |
Call with instance of Rx64IoSampleResponse only if getApiId() == RX_64_IO_RESPONSE.
void getRx64Response | ( | XBeeResponse & | response ) | [inherited] |
Call with instance of Rx64Response only if getApiId() == RX_64_RESPONSE.
void getTxStatusResponse | ( | XBeeResponse & | response ) | [inherited] |
Call with instance of TxStatusResponse only if getApiId() == TX_STATUS_RESPONSE.
void getZBRxIoSampleResponse | ( | XBeeResponse & | response ) | [inherited] |
Call with instance of ZBRxIoSampleResponse class only if getApiId() == ZB_IO_SAMPLE_RESPONSE to populate response.
void getZBRxResponse | ( | XBeeResponse & | response ) | [inherited] |
Call with instance of ZBRxResponse class only if getApiId() == ZB_RX_RESPONSE to populate response.
void getZBTxStatusResponse | ( | XBeeResponse & | response ) | [inherited] |
Call with instance of ZBTxStatusResponse class only if getApiId() == ZB_TX_STATUS_RESPONSE to populate response.
bool isAvailable | ( | ) | [inherited] |
bool isError | ( | ) | [inherited] |
Generated on Tue Jul 12 2022 18:07:39 by
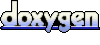