XBee-mbed library http://mbed.org/users/okini3939/notebook/xbee-mbed/
Dependents: device_server_udp led_sender_post XBee_API_ex1 XBee_API_ex2 ... more
XBee.h
00001 /** 00002 * XBee-mbed library 00003 * Modified for mbed, 2011 Suga. 00004 * 00005 * 00006 * Copyright (c) 2009 Andrew Rapp. All rights reserved. 00007 * 00008 * This file is part of XBee-Arduino. 00009 * 00010 * XBee-Arduino is free software: you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation, either version 3 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * XBee-Arduino is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License 00021 * along with XBee-Arduino. If not, see <http://www.gnu.org/licenses/>. 00022 */ 00023 00024 /** @file 00025 * @brief XBee library for mbed 00026 */ 00027 00028 #include "XBee_conf.h" 00029 00030 #ifndef XBee_h 00031 #define XBee_h 00032 00033 #include "mbed.h" 00034 #include <inttypes.h> 00035 00036 #define SERIES_1 00037 #define SERIES_2 00038 #define WIFI 00039 00040 // set to ATAP value of XBee. AP=2 is recommended 00041 #define ATAP 2 00042 00043 #define START_BYTE 0x7e 00044 #define ESCAPE 0x7d 00045 #define XON 0x11 00046 #define XOFF 0x13 00047 00048 // This value determines the size of the byte array for receiving RX packets 00049 // Most users won't be dealing with packets this large so you can adjust this 00050 // value to reduce memory consumption. But, remember that 00051 // if a RX packet exceeds this size, it cannot be parsed! 00052 00053 // This value is determined by the largest packet size (100 byte payload + 64-bit address + option byte and rssi byte) of a series 1 radio 00054 //#define MAX_FRAME_DATA_SIZE 110 00055 #define MAX_FRAME_DATA_SIZE 1520 00056 #define MAX_RXBUF_SIZE 32 00057 00058 #define BROADCAST_ADDRESS 0xffff 00059 #define ZB_BROADCAST_ADDRESS 0xfffe 00060 00061 // the non-variable length of the frame data (not including frame id or api id or variable data size (e.g. payload, at command set value) 00062 #define ZB_TX_API_LENGTH 12 00063 #define TX_16_API_LENGTH 3 00064 #define TX_64_API_LENGTH 9 00065 #define AT_COMMAND_API_LENGTH 2 00066 #define REMOTE_AT_COMMAND_API_LENGTH 13 00067 // start/length(2)/api/frameid/checksum bytes 00068 #define PACKET_OVERHEAD_LENGTH 6 00069 // api is always the third byte in packet 00070 #define API_ID_INDEX 3 00071 00072 // frame position of rssi byte 00073 #define RX_16_RSSI_OFFSET 2 00074 #define RX_64_RSSI_OFFSET 8 00075 00076 #define DEFAULT_FRAME_ID 1 00077 #define NO_RESPONSE_FRAME_ID 0 00078 00079 // TODO put in tx16 class 00080 #define ACK_OPTION 0 00081 #define DISABLE_ACK_OPTION 1 00082 #define BROADCAST_OPTION 4 00083 00084 // RX options 00085 #define ZB_PACKET_ACKNOWLEDGED 0x01 00086 #define ZB_BROADCAST_PACKET 0x02 00087 00088 // not everything is implemented! 00089 /** 00090 * Api Id constants 00091 */ 00092 #define TX_64_REQUEST 0x0 00093 #define TX_16_REQUEST 0x1 00094 #define AT_COMMAND_REQUEST 0x08 00095 #define AT_COMMAND_QUEUE_REQUEST 0x09 00096 #define REMOTE_AT_REQUEST 0x17 00097 #define ZB_TX_REQUEST 0x10 00098 #define ZB_EXPLICIT_TX_REQUEST 0x11 00099 #define RX_64_RESPONSE 0x80 00100 #define RX_16_RESPONSE 0x81 00101 #define RX_64_IO_RESPONSE 0x82 00102 #define RX_16_IO_RESPONSE 0x83 00103 #define AT_RESPONSE 0x88 00104 #define TX_STATUS_RESPONSE 0x89 00105 #define MODEM_STATUS_RESPONSE 0x8a 00106 #define ZB_RX_RESPONSE 0x90 00107 #define ZB_EXPLICIT_RX_RESPONSE 0x91 00108 #define ZB_TX_STATUS_RESPONSE 0x8b 00109 #define ZB_IO_SAMPLE_RESPONSE 0x92 00110 #define ZB_IO_NODE_IDENTIFIER_RESPONSE 0x95 00111 #define AT_COMMAND_RESPONSE 0x88 00112 #define REMOTE_AT_COMMAND_RESPONSE 0x97 00113 00114 00115 /** 00116 * TX STATUS constants 00117 */ 00118 #define SUCCESS 0x0 00119 #define CCA_FAILURE 0x2 00120 #define INVALID_DESTINATION_ENDPOINT_SUCCESS 0x15 00121 #define NETWORK_ACK_FAILURE 0x21 00122 #define NOT_JOINED_TO_NETWORK 0x22 00123 #define SELF_ADDRESSED 0x23 00124 #define ADDRESS_NOT_FOUND 0x24 00125 #define ROUTE_NOT_FOUND 0x25 00126 #define PAYLOAD_TOO_LARGE 0x74 00127 00128 // modem status 00129 #define HARDWARE_RESET 0 00130 #define WATCHDOG_TIMER_RESET 1 00131 #define ASSOCIATED 2 00132 #define DISASSOCIATED 3 00133 #define SYNCHRONIZATION_LOST 4 00134 #define COORDINATOR_REALIGNMENT 5 00135 #define COORDINATOR_STARTED 6 00136 00137 #define ZB_BROADCAST_RADIUS_MAX_HOPS 0 00138 00139 #define ZB_TX_UNICAST 0 00140 #define ZB_TX_BROADCAST 8 00141 00142 #define AT_OK 0 00143 #define AT_ERROR 1 00144 #define AT_INVALID_COMMAND 2 00145 #define AT_INVALID_PARAMETER 3 00146 #define AT_NO_RESPONSE 4 00147 00148 #define NO_ERROR 0 00149 #define CHECKSUM_FAILURE 1 00150 #define PACKET_EXCEEDS_BYTE_ARRAY_LENGTH 2 00151 #define UNEXPECTED_START_BYTE 3 00152 00153 /** 00154 * The super class of all XBee responses (RX packets) 00155 * Users should never attempt to create an instance of this class; instead 00156 * create an instance of a subclass 00157 * It is recommend to reuse subclasses to conserve memory 00158 */ 00159 class XBeeResponse { 00160 public: 00161 //static const int MODEM_STATUS = 0x8a; 00162 /** 00163 * Default constructor 00164 */ 00165 XBeeResponse(); 00166 /** 00167 * Returns Api Id of the response 00168 */ 00169 uint8_t getApiId(); 00170 void setApiId(uint8_t apiId); 00171 /** 00172 * Returns the MSB length of the packet 00173 */ 00174 uint8_t getMsbLength(); 00175 void setMsbLength(uint8_t msbLength); 00176 /** 00177 * Returns the LSB length of the packet 00178 */ 00179 uint8_t getLsbLength(); 00180 void setLsbLength(uint8_t lsbLength); 00181 /** 00182 * Returns the packet checksum 00183 */ 00184 uint8_t getChecksum(); 00185 void setChecksum(uint8_t checksum); 00186 /** 00187 * Returns the length of the frame data: all bytes after the api id, and prior to the checksum 00188 * Note up to release 0.1.2, this was incorrectly including the checksum in the length. 00189 */ 00190 uint16_t getFrameDataLength(); 00191 void setFrameData(uint8_t* frameDataPtr); 00192 /** 00193 * Returns the buffer that contains the response. 00194 * Starts with byte that follows API ID and includes all bytes prior to the checksum 00195 * Length is specified by getFrameDataLength() 00196 * Note: Unlike Digi's definition of the frame data, this does not start with the API ID.. 00197 * The reason for this is all responses include an API ID, whereas my frame data 00198 * includes only the API specific data. 00199 */ 00200 uint8_t* getFrameData(); 00201 00202 void setFrameLength(uint16_t frameLength); 00203 // to support future 65535 byte packets I guess 00204 /** 00205 * Returns the length of the packet 00206 */ 00207 uint16_t getPacketLength(); 00208 /** 00209 * Resets the response to default values 00210 */ 00211 void reset(); 00212 /** 00213 * Initializes the response 00214 */ 00215 void init(); 00216 #ifdef SERIES_2 00217 /** 00218 * Call with instance of ZBTxStatusResponse class only if getApiId() == ZB_TX_STATUS_RESPONSE 00219 * to populate response 00220 */ 00221 void getZBTxStatusResponse(XBeeResponse &response); 00222 /** 00223 * Call with instance of ZBRxResponse class only if getApiId() == ZB_RX_RESPONSE 00224 * to populate response 00225 */ 00226 void getZBRxResponse(XBeeResponse &response); 00227 /** 00228 * Call with instance of ZBRxIoSampleResponse class only if getApiId() == ZB_IO_SAMPLE_RESPONSE 00229 * to populate response 00230 */ 00231 void getZBRxIoSampleResponse(XBeeResponse &response); 00232 #endif 00233 #ifdef SERIES_1 00234 /** 00235 * Call with instance of TxStatusResponse only if getApiId() == TX_STATUS_RESPONSE 00236 */ 00237 void getTxStatusResponse(XBeeResponse &response); 00238 /** 00239 * Call with instance of Rx16Response only if getApiId() == RX_16_RESPONSE 00240 */ 00241 void getRx16Response(XBeeResponse &response); 00242 /** 00243 * Call with instance of Rx64Response only if getApiId() == RX_64_RESPONSE 00244 */ 00245 void getRx64Response(XBeeResponse &response); 00246 /** 00247 * Call with instance of Rx16IoSampleResponse only if getApiId() == RX_16_IO_RESPONSE 00248 */ 00249 void getRx16IoSampleResponse(XBeeResponse &response); 00250 /** 00251 * Call with instance of Rx64IoSampleResponse only if getApiId() == RX_64_IO_RESPONSE 00252 */ 00253 void getRx64IoSampleResponse(XBeeResponse &response); 00254 #endif 00255 /** 00256 * Call with instance of AtCommandResponse only if getApiId() == AT_COMMAND_RESPONSE 00257 */ 00258 void getAtCommandResponse(XBeeResponse &responses); 00259 /** 00260 * Call with instance of RemoteAtCommandResponse only if getApiId() == REMOTE_AT_COMMAND_RESPONSE 00261 */ 00262 void getRemoteAtCommandResponse(XBeeResponse &response); 00263 /** 00264 * Call with instance of ModemStatusResponse only if getApiId() == MODEM_STATUS_RESPONSE 00265 */ 00266 void getModemStatusResponse(XBeeResponse &response); 00267 /** 00268 * Returns true if the response has been successfully parsed and is complete and ready for use 00269 */ 00270 bool isAvailable(); 00271 void setAvailable(bool complete); 00272 /** 00273 * Returns true if the response contains errors 00274 */ 00275 bool isError(); 00276 /** 00277 * Returns an error code, or zero, if successful. 00278 * Error codes include: CHECKSUM_FAILURE, PACKET_EXCEEDS_BYTE_ARRAY_LENGTH, UNEXPECTED_START_BYTE 00279 */ 00280 uint8_t getErrorCode(); 00281 void setErrorCode(uint8_t errorCode); 00282 protected: 00283 // pointer to frameData 00284 uint8_t* _frameDataPtr; 00285 private: 00286 void setCommon(XBeeResponse &target); 00287 uint8_t _apiId; 00288 uint8_t _msbLength; 00289 uint8_t _lsbLength; 00290 uint8_t _checksum; 00291 uint16_t _frameLength; 00292 bool _complete; 00293 uint8_t _errorCode; 00294 }; 00295 00296 class XBeeAddress { 00297 public: 00298 XBeeAddress(); 00299 }; 00300 00301 /** 00302 * Represents a 64-bit XBee Address 00303 */ 00304 class XBeeAddress64 : public XBeeAddress { 00305 public: 00306 XBeeAddress64(uint32_t msb, uint32_t lsb); 00307 XBeeAddress64(); 00308 uint32_t getMsb(); 00309 uint32_t getLsb(); 00310 void setMsb(uint32_t msb); 00311 void setLsb(uint32_t lsb); 00312 private: 00313 uint32_t _msb; 00314 uint32_t _lsb; 00315 }; 00316 00317 //class XBeeAddress16 : public XBeeAddress { 00318 //public: 00319 // XBeeAddress16(uint16_t addr); 00320 // XBeeAddress16(); 00321 // uint16_t getAddress(); 00322 // void setAddress(uint16_t addr); 00323 //private: 00324 // uint16_t _addr; 00325 //}; 00326 00327 /** 00328 * This class is extended by all Responses that include a frame id 00329 */ 00330 class FrameIdResponse : public XBeeResponse { 00331 public: 00332 FrameIdResponse(); 00333 uint8_t getFrameId(); 00334 private: 00335 uint8_t _frameId; 00336 }; 00337 00338 /** 00339 * Common functionality for both Series 1 and 2 data RX data packets 00340 */ 00341 class RxDataResponse : public XBeeResponse { 00342 public: 00343 RxDataResponse(); 00344 /** 00345 * Returns the specified index of the payload. The index may be 0 to getDataLength() - 1 00346 * This method is deprecated; use uint8_t* getData() 00347 */ 00348 uint8_t getData(int index); 00349 /** 00350 * Returns the payload array. This may be accessed from index 0 to getDataLength() - 1 00351 */ 00352 uint8_t* getData(); 00353 /** 00354 * Returns the length of the payload 00355 */ 00356 virtual uint16_t getDataLength() = 0; 00357 /** 00358 * Returns the position in the frame data where the data begins 00359 */ 00360 virtual uint8_t getDataOffset() = 0; 00361 }; 00362 00363 // getResponse to return the proper subclass: 00364 // we maintain a pointer to each type of response, when a response is parsed, it is allocated only if NULL 00365 // can we allocate an object in a function? 00366 00367 #ifdef SERIES_2 00368 /** 00369 * Represents a Series 2 TX status packet 00370 */ 00371 class ZBTxStatusResponse : public FrameIdResponse { 00372 public: 00373 ZBTxStatusResponse(); 00374 uint16_t getRemoteAddress(); 00375 uint8_t getTxRetryCount(); 00376 uint8_t getDeliveryStatus(); 00377 uint8_t getDiscoveryStatus(); 00378 bool isSuccess(); 00379 }; 00380 00381 /** 00382 * Represents a Series 2 RX packet 00383 */ 00384 class ZBRxResponse : public RxDataResponse { 00385 public: 00386 ZBRxResponse(); 00387 XBeeAddress64& getRemoteAddress64(); 00388 uint16_t getRemoteAddress16(); 00389 uint8_t getOption(); 00390 virtual uint16_t getDataLength(); 00391 // frame position where data starts 00392 virtual uint8_t getDataOffset(); 00393 private: 00394 XBeeAddress64 _remoteAddress64; 00395 }; 00396 00397 /** 00398 * Represents a Series 2 RX I/O Sample packet 00399 */ 00400 class ZBRxIoSampleResponse : public ZBRxResponse { 00401 public: 00402 ZBRxIoSampleResponse(); 00403 bool containsAnalog(); 00404 bool containsDigital(); 00405 /** 00406 * Returns true if the pin is enabled 00407 */ 00408 bool isAnalogEnabled(uint8_t pin); 00409 /** 00410 * Returns true if the pin is enabled 00411 */ 00412 bool isDigitalEnabled(uint8_t pin); 00413 /** 00414 * Returns the 10-bit analog reading of the specified pin. 00415 * Valid pins include ADC:xxx. 00416 */ 00417 uint16_t getAnalog(uint8_t pin); 00418 /** 00419 * Returns true if the specified pin is high/on. 00420 * Valid pins include DIO:xxx. 00421 */ 00422 bool isDigitalOn(uint8_t pin); 00423 uint8_t getDigitalMaskMsb(); 00424 uint8_t getDigitalMaskLsb(); 00425 uint8_t getAnalogMask(); 00426 }; 00427 00428 #endif 00429 00430 #ifdef SERIES_1 00431 /** 00432 * Represents a Series 1 TX Status packet 00433 */ 00434 class TxStatusResponse : public FrameIdResponse { 00435 public: 00436 TxStatusResponse(); 00437 uint8_t getStatus(); 00438 bool isSuccess(); 00439 }; 00440 00441 /** 00442 * Represents a Series 1 RX packet 00443 */ 00444 class RxResponse : public RxDataResponse { 00445 public: 00446 RxResponse(); 00447 // remember rssi is negative but this is unsigned byte so it's up to you to convert 00448 uint8_t getRssi(); 00449 uint8_t getOption(); 00450 bool isAddressBroadcast(); 00451 bool isPanBroadcast(); 00452 virtual uint16_t getDataLength(); 00453 virtual uint8_t getDataOffset(); 00454 virtual uint8_t getRssiOffset() = 0; 00455 }; 00456 00457 /** 00458 * Represents a Series 1 16-bit address RX packet 00459 */ 00460 class Rx16Response : public RxResponse { 00461 public: 00462 Rx16Response(); 00463 virtual uint8_t getRssiOffset(); 00464 uint16_t getRemoteAddress16(); 00465 protected: 00466 uint16_t _remoteAddress; 00467 }; 00468 00469 /** 00470 * Represents a Series 1 64-bit address RX packet 00471 */ 00472 class Rx64Response : public RxResponse { 00473 public: 00474 Rx64Response(); 00475 virtual uint8_t getRssiOffset(); 00476 XBeeAddress64& getRemoteAddress64(); 00477 private: 00478 XBeeAddress64 _remoteAddress; 00479 }; 00480 00481 /** 00482 * Represents a Series 1 RX I/O Sample packet 00483 */ 00484 class RxIoSampleBaseResponse : public RxResponse { 00485 public: 00486 RxIoSampleBaseResponse(); 00487 /** 00488 * Returns the number of samples in this packet 00489 */ 00490 uint8_t getSampleSize(); 00491 bool containsAnalog(); 00492 bool containsDigital(); 00493 /** 00494 * Returns true if the specified analog pin is enabled 00495 */ 00496 bool isAnalogEnabled(uint8_t pin); 00497 /** 00498 * Returns true if the specified digital pin is enabled 00499 */ 00500 bool isDigitalEnabled(uint8_t pin); 00501 /** 00502 * Returns the 10-bit analog reading of the specified pin. 00503 * Valid pins include ADC:0-5. Sample index starts at 0 00504 */ 00505 uint16_t getAnalog(uint8_t pin, uint8_t sample); 00506 /** 00507 * Returns true if the specified pin is high/on. 00508 * Valid pins include DIO:0-8. Sample index starts at 0 00509 */ 00510 bool isDigitalOn(uint8_t pin, uint8_t sample); 00511 uint8_t getSampleOffset(); 00512 private: 00513 }; 00514 00515 class Rx16IoSampleResponse : public RxIoSampleBaseResponse { 00516 public: 00517 Rx16IoSampleResponse(); 00518 uint16_t getRemoteAddress16(); 00519 virtual uint8_t getRssiOffset(); 00520 00521 }; 00522 00523 class Rx64IoSampleResponse : public RxIoSampleBaseResponse { 00524 public: 00525 Rx64IoSampleResponse(); 00526 XBeeAddress64& getRemoteAddress64(); 00527 virtual uint8_t getRssiOffset(); 00528 private: 00529 XBeeAddress64 _remoteAddress; 00530 }; 00531 00532 #endif 00533 00534 /** 00535 * Represents a Modem Status RX packet 00536 */ 00537 class ModemStatusResponse : public XBeeResponse { 00538 public: 00539 ModemStatusResponse(); 00540 uint8_t getStatus(); 00541 }; 00542 00543 /** 00544 * Represents an AT Command RX packet 00545 */ 00546 class AtCommandResponse : public FrameIdResponse { 00547 public: 00548 AtCommandResponse(); 00549 /** 00550 * Returns an array containing the two character command 00551 */ 00552 uint8_t* getCommand(); 00553 /** 00554 * Returns the command status code. 00555 * Zero represents a successful command 00556 */ 00557 uint8_t getStatus(); 00558 /** 00559 * Returns an array containing the command value. 00560 * This is only applicable to query commands. 00561 */ 00562 uint8_t* getValue(); 00563 /** 00564 * Returns the length of the command value array. 00565 */ 00566 uint16_t getValueLength(); 00567 /** 00568 * Returns true if status equals AT_OK 00569 */ 00570 bool isOk(); 00571 }; 00572 00573 /** 00574 * Represents a Remote AT Command RX packet 00575 */ 00576 class RemoteAtCommandResponse : public AtCommandResponse { 00577 public: 00578 RemoteAtCommandResponse(); 00579 /** 00580 * Returns an array containing the two character command 00581 */ 00582 uint8_t* getCommand(); 00583 /** 00584 * Returns the command status code. 00585 * Zero represents a successful command 00586 */ 00587 uint8_t getStatus(); 00588 /** 00589 * Returns an array containing the command value. 00590 * This is only applicable to query commands. 00591 */ 00592 uint8_t* getValue(); 00593 /** 00594 * Returns the length of the command value array. 00595 */ 00596 uint16_t getValueLength(); 00597 /** 00598 * Returns the 16-bit address of the remote radio 00599 */ 00600 uint16_t getRemoteAddress16(); 00601 /** 00602 * Returns the 64-bit address of the remote radio 00603 */ 00604 XBeeAddress64& getRemoteAddress64(); 00605 /** 00606 * Returns true if command was successful 00607 */ 00608 bool isOk(); 00609 private: 00610 XBeeAddress64 _remoteAddress64; 00611 }; 00612 00613 00614 /** 00615 * Super class of all XBee requests (TX packets) 00616 * Users should never create an instance of this class; instead use an subclass of this class 00617 * It is recommended to reuse Subclasses of the class to conserve memory 00618 * <p/> 00619 * This class allocates a buffer to 00620 */ 00621 class XBeeRequest { 00622 public: 00623 /** 00624 * Constructor 00625 * TODO make protected 00626 */ 00627 XBeeRequest(uint8_t apiId, uint8_t frameId); 00628 /** 00629 * Sets the frame id. Must be between 1 and 255 inclusive to get a TX status response. 00630 */ 00631 void setFrameId(uint8_t frameId); 00632 /** 00633 * Returns the frame id 00634 */ 00635 uint8_t getFrameId(); 00636 /** 00637 * Returns the API id 00638 */ 00639 uint8_t getApiId(); 00640 // setting = 0 makes this a pure virtual function, meaning the subclass must implement, like abstract in java 00641 /** 00642 * Starting after the frame id (pos = 0) and up to but not including the checksum 00643 * Note: Unlike Digi's definition of the frame data, this does not start with the API ID. 00644 * The reason for this is the API ID and Frame ID are common to all requests, whereas my definition of 00645 * frame data is only the API specific data. 00646 */ 00647 virtual uint8_t getFrameData(uint16_t pos) = 0; 00648 /** 00649 * Returns the size of the api frame (not including frame id or api id or checksum). 00650 */ 00651 virtual uint16_t getFrameDataLength() = 0; 00652 //void reset(); 00653 protected: 00654 void setApiId(uint8_t apiId); 00655 private: 00656 uint8_t _apiId; 00657 uint8_t _frameId; 00658 }; 00659 00660 // TODO add reset/clear method since responses are often reused 00661 /** 00662 * Primary interface for communicating with an XBee Radio. 00663 * This class provides methods for sending and receiving packets with an XBee radio via the serial port. 00664 * The XBee radio must be configured in API (packet) mode (AP=2) 00665 * in order to use this software. 00666 * <p/> 00667 * Since this code is designed to run on a microcontroller, with only one thread, you are responsible for reading the 00668 * data off the serial buffer in a timely manner. This involves a call to a variant of readPacket(...). 00669 * If your serial port is receiving data faster than you are reading, you can expect to lose packets. 00670 * Arduino only has a 128 byte serial buffer so it can easily overflow if two or more packets arrive 00671 * without a call to readPacket(...) 00672 * <p/> 00673 * In order to conserve resources, this class only supports storing one response packet in memory at a time. 00674 * This means that you must fully consume the packet prior to calling readPacket(...), because calling 00675 * readPacket(...) overwrites the previous response. 00676 * <p/> 00677 * This class creates an array of size MAX_FRAME_DATA_SIZE for storing the response packet. You may want 00678 * to adjust this value to conserve memory. 00679 * 00680 * \author Andrew Rapp 00681 */ 00682 class XBee { 00683 public: 00684 XBee(PinName p_tx, PinName p_rx); 00685 XBee(PinName p_tx, PinName p_rx, PinName p_cts, PinName p_rts); 00686 // for eclipse dev only 00687 // void setSerial(HardwareSerial serial); 00688 /** 00689 * Reads all available serial bytes until a packet is parsed, an error occurs, or the buffer is empty. 00690 * You may call <i>xbee</i>.getResponse().isAvailable() after calling this method to determine if 00691 * a packet is ready, or <i>xbee</i>.getResponse().isError() to determine if 00692 * a error occurred. 00693 * <p/> 00694 * This method should always return quickly since it does not wait for serial data to arrive. 00695 * You will want to use this method if you are doing other timely stuff in your loop, where 00696 * a delay would cause problems. 00697 * NOTE: calling this method resets the current response, so make sure you first consume the 00698 * current response 00699 */ 00700 void readPacket(); 00701 /** 00702 * Waits a maximum of <i>timeout</i> milliseconds for a response packet before timing out; returns true if packet is read. 00703 * Returns false if timeout or error occurs. 00704 */ 00705 bool readPacket(int timeout); 00706 /** 00707 * Reads until a packet is received or an error occurs. 00708 * Caution: use this carefully since if you don't get a response, your Arduino code will hang on this 00709 * call forever!! often it's better to use a timeout: readPacket(int) 00710 */ 00711 void readPacketUntilAvailable(); 00712 /** 00713 * Starts the serial connection at the supplied baud rate 00714 */ 00715 void begin(long baud); 00716 void getResponse(XBeeResponse &response); 00717 /** 00718 * Returns a reference to the current response 00719 * Note: once readPacket is called again this response will be overwritten! 00720 */ 00721 XBeeResponse& getResponse(); 00722 /** 00723 * Sends a XBeeRequest (TX packet) out the serial port 00724 */ 00725 void send(XBeeRequest &request); 00726 //uint8_t sendAndWaitForResponse(XBeeRequest &request, int timeout); 00727 /** 00728 * Returns a sequential frame id between 1 and 255 00729 */ 00730 uint8_t getNextFrameId(); 00731 00732 void isr_recv (); 00733 int getbuf (); 00734 int bufreadable (); 00735 private: 00736 void sendByte(uint8_t b, bool escape); 00737 void resetResponse(); 00738 XBeeResponse _response; 00739 bool _escape; 00740 // current packet position for response. just a state variable for packet parsing and has no relevance for the response otherwise 00741 uint16_t _pos, _epos; 00742 // last byte read 00743 uint8_t b; 00744 uint16_t _checksumTotal; 00745 uint8_t _nextFrameId; 00746 // buffer for incoming RX packets. holds only the api specific frame data, starting after the api id byte and prior to checksum 00747 uint8_t _responseFrameData[MAX_FRAME_DATA_SIZE]; 00748 Serial _xbee; 00749 char _rxbuf[MAX_RXBUF_SIZE]; 00750 int _rxaddr_w, _rxaddr_r; 00751 bool _rts; 00752 }; 00753 00754 /** 00755 * All TX packets that support payloads extend this class 00756 */ 00757 class PayloadRequest : public XBeeRequest { 00758 public: 00759 PayloadRequest(uint8_t apiId, uint8_t frameId, uint8_t *payload, uint16_t payloadLength); 00760 /** 00761 * Returns the payload of the packet, if not null 00762 */ 00763 uint8_t* getPayload(); 00764 /** 00765 * Sets the payload array 00766 */ 00767 void setPayload(uint8_t* payloadPtr); 00768 /** 00769 * Returns the length of the payload array, as specified by the user. 00770 */ 00771 uint16_t getPayloadLength(); 00772 /** 00773 * Sets the length of the payload to include in the request. For example if the payload array 00774 * is 50 bytes and you only want the first 10 to be included in the packet, set the length to 10. 00775 * Length must be <= to the array length. 00776 */ 00777 void setPayloadLength(uint16_t payloadLength); 00778 private: 00779 uint8_t* _payloadPtr; 00780 uint16_t _payloadLength; 00781 }; 00782 00783 #ifdef SERIES_1 00784 00785 /** 00786 * Represents a Series 1 TX packet that corresponds to Api Id: TX_16_REQUEST 00787 * <p/> 00788 * Be careful not to send a data array larger than the max packet size of your radio. 00789 * This class does not perform any validation of packet size and there will be no indication 00790 * if the packet is too large, other than you will not get a TX Status response. 00791 * The datasheet says 100 bytes is the maximum, although that could change in future firmware. 00792 */ 00793 class Tx16Request : public PayloadRequest { 00794 public: 00795 Tx16Request(uint16_t addr16, uint8_t option, uint8_t *payload, uint16_t payloadLength, uint8_t frameId); 00796 /** 00797 * Creates a Unicast Tx16Request with the ACK option and DEFAULT_FRAME_ID 00798 */ 00799 Tx16Request(uint16_t addr16, uint8_t *payload, uint16_t payloadLength); 00800 /** 00801 * Creates a default instance of this class. At a minimum you must specify 00802 * a payload, payload length and a destination address before sending this request. 00803 */ 00804 Tx16Request(); 00805 uint16_t getAddress16(); 00806 void setAddress16(uint16_t addr16); 00807 uint8_t getOption(); 00808 void setOption(uint8_t option); 00809 virtual uint8_t getFrameData(uint16_t pos); 00810 virtual uint16_t getFrameDataLength(); 00811 protected: 00812 private: 00813 uint16_t _addr16; 00814 uint8_t _option; 00815 }; 00816 00817 /** 00818 * Represents a Series 1 TX packet that corresponds to Api Id: TX_64_REQUEST 00819 * 00820 * Be careful not to send a data array larger than the max packet size of your radio. 00821 * This class does not perform any validation of packet size and there will be no indication 00822 * if the packet is too large, other than you will not get a TX Status response. 00823 * The datasheet says 100 bytes is the maximum, although that could change in future firmware. 00824 */ 00825 class Tx64Request : public PayloadRequest { 00826 public: 00827 Tx64Request(XBeeAddress64 &addr64, uint8_t option, uint8_t *payload, uint16_t payloadLength, uint8_t frameId); 00828 /** 00829 * Creates a unicast Tx64Request with the ACK option and DEFAULT_FRAME_ID 00830 */ 00831 Tx64Request(XBeeAddress64 &addr64, uint8_t *payload, uint16_t payloadLength); 00832 /** 00833 * Creates a default instance of this class. At a minimum you must specify 00834 * a payload, payload length and a destination address before sending this request. 00835 */ 00836 Tx64Request(); 00837 XBeeAddress64& getAddress64(); 00838 void setAddress64(XBeeAddress64& addr64); 00839 // TODO move option to superclass 00840 uint8_t getOption(); 00841 void setOption(uint8_t option); 00842 virtual uint8_t getFrameData(uint16_t pos); 00843 virtual uint16_t getFrameDataLength(); 00844 private: 00845 XBeeAddress64 _addr64; 00846 uint8_t _option; 00847 }; 00848 00849 #endif 00850 00851 00852 #ifdef SERIES_2 00853 00854 /** 00855 * Represents a Series 2 TX packet that corresponds to Api Id: ZB_TX_REQUEST 00856 * 00857 * Be careful not to send a data array larger than the max packet size of your radio. 00858 * This class does not perform any validation of packet size and there will be no indication 00859 * if the packet is too large, other than you will not get a TX Status response. 00860 * The datasheet says 72 bytes is the maximum for ZNet firmware and ZB Pro firmware provides 00861 * the ATNP command to get the max supported payload size. This command is useful since the 00862 * maximum payload size varies according to certain settings, such as encryption. 00863 * ZB Pro firmware provides a PAYLOAD_TOO_LARGE that is returned if payload size 00864 * exceeds the maximum. 00865 */ 00866 class ZBTxRequest : public PayloadRequest { 00867 public: 00868 /** 00869 * Creates a unicast ZBTxRequest with the ACK option and DEFAULT_FRAME_ID 00870 */ 00871 ZBTxRequest(XBeeAddress64 &addr64, uint8_t *payload, uint16_t payloadLength); 00872 ZBTxRequest(XBeeAddress64 &addr64, uint16_t addr16, uint8_t broadcastRadius, uint8_t option, uint8_t *payload, uint16_t payloadLength, uint8_t frameId); 00873 /** 00874 * Creates a default instance of this class. At a minimum you must specify 00875 * a payload, payload length and a destination address before sending this request. 00876 */ 00877 ZBTxRequest(); 00878 XBeeAddress64& getAddress64(); 00879 uint16_t getAddress16(); 00880 uint8_t getBroadcastRadius(); 00881 uint8_t getOption(); 00882 void setAddress64(XBeeAddress64& addr64); 00883 void setAddress16(uint16_t addr16); 00884 void setBroadcastRadius(uint8_t broadcastRadius); 00885 void setOption(uint8_t option); 00886 protected: 00887 // declare virtual functions 00888 virtual uint8_t getFrameData(uint16_t pos); 00889 virtual uint16_t getFrameDataLength(); 00890 private: 00891 XBeeAddress64 _addr64; 00892 uint16_t _addr16; 00893 uint8_t _broadcastRadius; 00894 uint8_t _option; 00895 }; 00896 00897 #endif 00898 00899 /** 00900 * Represents an AT Command TX packet 00901 * The command is used to configure the serially connected XBee radio 00902 */ 00903 class AtCommandRequest : public XBeeRequest { 00904 public: 00905 AtCommandRequest(); 00906 AtCommandRequest(uint8_t *command); 00907 AtCommandRequest(uint8_t *command, uint8_t *commandValue, uint8_t commandValueLength); 00908 virtual uint8_t getFrameData(uint16_t pos); 00909 virtual uint16_t getFrameDataLength(); 00910 uint8_t* getCommand(); 00911 void setCommand(uint8_t* command); 00912 uint8_t* getCommandValue(); 00913 void setCommandValue(uint8_t* command); 00914 uint8_t getCommandValueLength(); 00915 void setCommandValueLength(uint8_t length); 00916 /** 00917 * Clears the optional commandValue and commandValueLength so that a query may be sent 00918 */ 00919 void clearCommandValue(); 00920 //void reset(); 00921 private: 00922 uint8_t *_command; 00923 uint8_t *_commandValue; 00924 uint8_t _commandValueLength; 00925 }; 00926 00927 /** 00928 * Represents an Remote AT Command TX packet 00929 * The command is used to configure a remote XBee radio 00930 */ 00931 class RemoteAtCommandRequest : public AtCommandRequest { 00932 public: 00933 RemoteAtCommandRequest(); 00934 /** 00935 * Creates a RemoteAtCommandRequest with 16-bit address to set a command. 00936 * 64-bit address defaults to broadcast and applyChanges is true. 00937 */ 00938 RemoteAtCommandRequest(uint16_t remoteAddress16, uint8_t *command, uint8_t *commandValue, uint8_t commandValueLength); 00939 /** 00940 * Creates a RemoteAtCommandRequest with 16-bit address to query a command. 00941 * 64-bit address defaults to broadcast and applyChanges is true. 00942 */ 00943 RemoteAtCommandRequest(uint16_t remoteAddress16, uint8_t *command); 00944 /** 00945 * Creates a RemoteAtCommandRequest with 64-bit address to set a command. 00946 * 16-bit address defaults to broadcast and applyChanges is true. 00947 */ 00948 RemoteAtCommandRequest(XBeeAddress64 &remoteAddress64, uint8_t *command, uint8_t *commandValue, uint8_t commandValueLength); 00949 /** 00950 * Creates a RemoteAtCommandRequest with 16-bit address to query a command. 00951 * 16-bit address defaults to broadcast and applyChanges is true. 00952 */ 00953 RemoteAtCommandRequest(XBeeAddress64 &remoteAddress64, uint8_t *command); 00954 uint16_t getRemoteAddress16(); 00955 void setRemoteAddress16(uint16_t remoteAddress16); 00956 XBeeAddress64& getRemoteAddress64(); 00957 void setRemoteAddress64(XBeeAddress64 &remoteAddress64); 00958 bool getApplyChanges(); 00959 void setApplyChanges(bool applyChanges); 00960 virtual uint8_t getFrameData(uint16_t pos); 00961 virtual uint16_t getFrameDataLength(); 00962 static XBeeAddress64 broadcastAddress64; 00963 // static uint16_t broadcast16Address; 00964 private: 00965 XBeeAddress64 _remoteAddress64; 00966 uint16_t _remoteAddress16; 00967 bool _applyChanges; 00968 }; 00969 00970 00971 00972 #endif //XBee_h
Generated on Tue Jul 12 2022 18:07:39 by
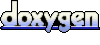