CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
RealFFT
[Transform Functions]
Functions | |
void | arm_rfft_f32 (const arm_rfft_instance_f32 *S, float32_t *pSrc, float32_t *pDst) |
Processing function for the floating-point RFFT/RIFFT. | |
void | arm_rfft_fast_f32 (arm_rfft_fast_instance_f32 *S, float32_t *p, float32_t *pOut, uint8_t ifftFlag) |
Processing function for the floating-point real FFT. | |
arm_status | arm_rfft_fast_init_f32 (arm_rfft_fast_instance_f32 *S, uint16_t fftLen) |
Initialization function for the floating-point real FFT. | |
arm_status | arm_rfft_init_f32 (arm_rfft_instance_f32 *S, arm_cfft_radix4_instance_f32 *S_CFFT, uint32_t fftLenReal, uint32_t ifftFlagR, uint32_t bitReverseFlag) |
Initialization function for the floating-point RFFT/RIFFT. | |
arm_status | arm_rfft_init_q15 (arm_rfft_instance_q15 *S, uint32_t fftLenReal, uint32_t ifftFlagR, uint32_t bitReverseFlag) |
Initialization function for the Q15 RFFT/RIFFT. | |
arm_status | arm_rfft_init_q31 (arm_rfft_instance_q31 *S, uint32_t fftLenReal, uint32_t ifftFlagR, uint32_t bitReverseFlag) |
Initialization function for the Q31 RFFT/RIFFT. | |
void | arm_rfft_q15 (const arm_rfft_instance_q15 *S, q15_t *pSrc, q15_t *pDst) |
Processing function for the Q15 RFFT/RIFFT. | |
void | arm_rfft_q31 (const arm_rfft_instance_q31 *S, q31_t *pSrc, q31_t *pDst) |
Processing function for the Q31 RFFT/RIFFT. | |
Variables | |
static const float32_t | realCoefA [8192] |
static const float32_t | realCoefB [8192] |
static const q15_t ALIGN4 | realCoefAQ15 [8192] |
static const q15_t ALIGN4 | realCoefBQ15 [8192] |
static const q31_t | realCoefAQ31 [8192] |
static const q31_t | realCoefBQ31 [8192] |
Function Documentation
void arm_rfft_f32 | ( | const arm_rfft_instance_f32 * | S, |
float32_t * | pSrc, | ||
float32_t * | pDst | ||
) |
Processing function for the floating-point RFFT/RIFFT.
- Parameters:
-
[in] *S points to an instance of the floating-point RFFT/RIFFT structure. [in] *pSrc points to the input buffer. [out] *pDst points to the output buffer.
- Returns:
- none.
Definition at line 100 of file arm_rfft_f32.c.
void arm_rfft_fast_f32 | ( | arm_rfft_fast_instance_f32 * | S, |
float32_t * | p, | ||
float32_t * | pOut, | ||
uint8_t | ifftFlag | ||
) |
Processing function for the floating-point real FFT.
- Parameters:
-
[in] *S points to an arm_rfft_fast_instance_f32 structure. [in] *p points to the input buffer. [in] *pOut points to the output buffer. [in] ifftFlag RFFT if flag is 0, RIFFT if flag is 1
- Returns:
- none.
Definition at line 324 of file arm_rfft_fast_f32.c.
arm_status arm_rfft_fast_init_f32 | ( | arm_rfft_fast_instance_f32 * | S, |
uint16_t | fftLen | ||
) |
Initialization function for the floating-point real FFT.
- Parameters:
-
[in,out] *S points to an arm_rfft_fast_instance_f32 structure. [in] fftLen length of the Real Sequence.
- Returns:
- The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if
fftLen
is not a supported value.
- Description:
- The parameter
fftLen
Specifies length of RFFT/CIFFT process. Supported FFT Lengths are 32, 64, 128, 256, 512, 1024, 2048, 4096.
- This Function also initializes Twiddle factor table pointer and Bit reversal table pointer.
Definition at line 65 of file arm_rfft_fast_init_f32.c.
arm_status arm_rfft_init_f32 | ( | arm_rfft_instance_f32 * | S, |
arm_cfft_radix4_instance_f32 * | S_CFFT, | ||
uint32_t | fftLenReal, | ||
uint32_t | ifftFlagR, | ||
uint32_t | bitReverseFlag | ||
) |
Initialization function for the floating-point RFFT/RIFFT.
- Parameters:
-
[in,out] *S points to an instance of the floating-point RFFT/RIFFT structure. [in,out] *S_CFFT points to an instance of the floating-point CFFT/CIFFT structure. [in] fftLenReal length of the FFT. [in] ifftFlagR flag that selects forward (ifftFlagR=0) or inverse (ifftFlagR=1) transform. [in] bitReverseFlag flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output.
- Returns:
- The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if
fftLenReal
is not a supported value.
- Description:
- The parameter
fftLenReal
Specifies length of RFFT/RIFFT Process. Supported FFT Lengths are 128, 512, 2048.
- The parameter
ifftFlagR
controls whether a forward or inverse transform is computed. Set(=1) ifftFlagR to calculate RIFFT, otherwise RFFT is calculated.
- The parameter
bitReverseFlag
controls whether output is in normal order or bit reversed order. Set(=1) bitReverseFlag for output to be in normal order otherwise output is in bit reversed order.
- This function also initializes Twiddle factor table.
Definition at line 8304 of file arm_rfft_init_f32.c.
arm_status arm_rfft_init_q15 | ( | arm_rfft_instance_q15 * | S, |
uint32_t | fftLenReal, | ||
uint32_t | ifftFlagR, | ||
uint32_t | bitReverseFlag | ||
) |
Initialization function for the Q15 RFFT/RIFFT.
- Parameters:
-
[in,out] *S points to an instance of the Q15 RFFT/RIFFT structure. [in] fftLenReal length of the FFT. [in] ifftFlagR flag that selects forward (ifftFlagR=0) or inverse (ifftFlagR=1) transform. [in] bitReverseFlag flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output.
- Returns:
- The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if
fftLenReal
is not a supported value.
- Description:
- The parameter
fftLenReal
Specifies length of RFFT/RIFFT Process. Supported FFT Lengths are 32, 64, 128, 256, 512, 1024, 2048, 4096, 8192.
- The parameter
ifftFlagR
controls whether a forward or inverse transform is computed. Set(=1) ifftFlagR to calculate RIFFT, otherwise RFFT is calculated.
- The parameter
bitReverseFlag
controls whether output is in normal order or bit reversed order. Set(=1) bitReverseFlag for output to be in normal order otherwise output is in bit reversed order.
- This function also initializes Twiddle factor table.
Definition at line 2160 of file arm_rfft_init_q15.c.
arm_status arm_rfft_init_q31 | ( | arm_rfft_instance_q31 * | S, |
uint32_t | fftLenReal, | ||
uint32_t | ifftFlagR, | ||
uint32_t | bitReverseFlag | ||
) |
Initialization function for the Q31 RFFT/RIFFT.
- Parameters:
-
[in,out] *S points to an instance of the Q31 RFFT/RIFFT structure. [in] fftLenReal length of the FFT. [in] ifftFlagR flag that selects forward (ifftFlagR=0) or inverse (ifftFlagR=1) transform. [in] bitReverseFlag flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output.
- Returns:
- The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if
fftLenReal
is not a supported value.
- Description:
- The parameter
fftLenReal
Specifies length of RFFT/RIFFT Process. Supported FFT Lengths are 32, 64, 128, 256, 512, 1024, 2048, 4096, 8192.
- The parameter
ifftFlagR
controls whether a forward or inverse transform is computed. Set(=1) ifftFlagR to calculate RIFFT, otherwise RFFT is calculated.
- The parameter
bitReverseFlag
controls whether output is in normal order or bit reversed order. Set(=1) bitReverseFlag for output to be in normal order otherwise output is in bit reversed order.
- 7
- This function also initializes Twiddle factor table.
Definition at line 4210 of file arm_rfft_init_q31.c.
void arm_rfft_q15 | ( | const arm_rfft_instance_q15 * | S, |
q15_t * | pSrc, | ||
q15_t * | pDst | ||
) |
Processing function for the Q15 RFFT/RIFFT.
- Parameters:
-
[in] *S points to an instance of the Q15 RFFT/RIFFT structure. [in] *pSrc points to the input buffer. [out] *pDst points to the output buffer.
- Returns:
- none.
- Input an output formats:
- Internally input is downscaled by 2 for every stage to avoid saturations inside CFFT/CIFFT process. Hence the output format is different for different RFFT sizes. The input and output formats for different RFFT sizes and number of bits to upscale are mentioned in the tables below for RFFT and RIFFT:
Input and Output Formats for Q15 RFFT
Input and Output Formats for Q15 RIFFT
Definition at line 87 of file arm_rfft_q15.c.
void arm_rfft_q31 | ( | const arm_rfft_instance_q31 * | S, |
q31_t * | pSrc, | ||
q31_t * | pDst | ||
) |
Processing function for the Q31 RFFT/RIFFT.
- Parameters:
-
[in] *S points to an instance of the Q31 RFFT/RIFFT structure. [in] *pSrc points to the input buffer. [out] *pDst points to the output buffer.
- Returns:
- none.
- Input an output formats:
- Internally input is downscaled by 2 for every stage to avoid saturations inside CFFT/CIFFT process. Hence the output format is different for different RFFT sizes. The input and output formats for different RFFT sizes and number of bits to upscale are mentioned in the tables below for RFFT and RIFFT:
Input and Output Formats for Q31 RFFT
Input and Output Formats for Q31 RIFFT
Definition at line 87 of file arm_rfft_q31.c.
Variable Documentation
const float32_t realCoefA[8192] [static] |
- Generation of realCoefA array:
- n = 4096
for (i = 0; i < n; i++) { pATable[2 * i] = 0.5 * (1.0 - sin (2 * PI / (double) (2 * n) * (double) i)); pATable[2 * i + 1] = 0.5 * (-1.0 * cos (2 * PI / (double) (2 * n) * (double) i)); }
Definition at line 67 of file arm_rfft_init_f32.c.
const q15_t ALIGN4 realCoefAQ15[8192] [static] |
- Generation fixed-point realCoefAQ15 array in Q15 format:
- n = 4096
for (i = 0; i < n; i++) { pATable[2 * i] = 0.5 * (1.0 - sin (2 * PI / (double) (2 * n) * (double) i)); pATable[2 * i + 1] = 0.5 * (-1.0 * cos (2 * PI / (double) (2 * n) * (double) i)); }
- Convert to fixed point Q15 format round(pATable[i] * pow(2, 15))
Definition at line 71 of file arm_rfft_init_q15.c.
const q31_t realCoefAQ31[8192] [static] |
- Generation fixed-point realCoefAQ31 array in Q31 format:
- n = 4096
for (i = 0; i < n; i++) { pATable[2 * i] = 0.5 * (1.0 - sin (2 * PI / (double) (2 * n) * (double) i)); pATable[2 * i + 1] = 0.5 * (-1.0 * cos (2 * PI / (double) (2 * n) * (double) i)); }
- Convert to fixed point Q31 format round(pATable[i] * pow(2, 31))
Definition at line 70 of file arm_rfft_init_q31.c.
const float32_t realCoefB[8192] [static] |
- Generation of realCoefB array:
- n = 4096
for (i = 0; i < n; i++) { pBTable[2 * i] = 0.5 * (1.0 + sin (2 * PI / (double) (2 * n) * (double) i)); pBTable[2 * i + 1] = 0.5 * (1.0 * cos (2 * PI / (double) (2 * n) * (double) i)); }
Definition at line 4179 of file arm_rfft_init_f32.c.
const q15_t ALIGN4 realCoefBQ15[8192] [static] |
- Generation of real_CoefB array:
- n = 4096
for (i = 0; i < n; i++) { pBTable[2 * i] = 0.5 * (1.0 + sin (2 * PI / (double) (2 * n) * (double) i)); pBTable[2 * i + 1] = 0.5 * (1.0 * cos (2 * PI / (double) (2 * n) * (double) i)); }
- Convert to fixed point Q15 format round(pBTable[i] * pow(2, 15))
Definition at line 1113 of file arm_rfft_init_q15.c.
const q31_t realCoefBQ31[8192] [static] |
- Generation of realCoefBQ31 array:
- n = 4096
for (i = 0; i < n; i++) { pBTable[2 * i] = 0.5 * (1.0 + sin (2 * PI / (double) (2 * n) * (double) i)); pBTable[2 * i + 1] = 0.5 * (1.0 * cos (2 * PI / (double) (2 * n) * (double) i)); }
- Convert to fixed point Q31 format round(pBTable[i] * pow(2, 31))
Definition at line 2138 of file arm_rfft_init_q31.c.
Generated on Tue Jul 12 2022 11:59:20 by
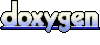