CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_rfft_fast_init_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_cfft_init_f32.c 00009 * 00010 * Description: Split Radix Decimation in Frequency CFFT Floating point processing function 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 #include "arm_common_tables.h" 00043 00044 /** 00045 * @ingroup groupTransforms 00046 */ 00047 00048 /** 00049 * @addtogroup RealFFT 00050 * @{ 00051 */ 00052 00053 /** 00054 * @brief Initialization function for the floating-point real FFT. 00055 * @param[in,out] *S points to an arm_rfft_fast_instance_f32 structure. 00056 * @param[in] fftLen length of the Real Sequence. 00057 * @return The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if <code>fftLen</code> is not a supported value. 00058 * 00059 * \par Description: 00060 * \par 00061 * The parameter <code>fftLen</code> Specifies length of RFFT/CIFFT process. Supported FFT Lengths are 32, 64, 128, 256, 512, 1024, 2048, 4096. 00062 * \par 00063 * This Function also initializes Twiddle factor table pointer and Bit reversal table pointer. 00064 */ 00065 arm_status arm_rfft_fast_init_f32( 00066 arm_rfft_fast_instance_f32 * S, 00067 uint16_t fftLen) 00068 { 00069 arm_cfft_instance_f32 * Sint; 00070 /* Initialise the default arm status */ 00071 arm_status status = ARM_MATH_SUCCESS; 00072 /* Initialise the FFT length */ 00073 Sint = &(S->Sint); 00074 Sint->fftLen = fftLen/2; 00075 S->fftLenRFFT = fftLen; 00076 00077 /* Initializations of structure parameters depending on the FFT length */ 00078 switch (Sint->fftLen) 00079 { 00080 case 2048u: 00081 /* Initializations of structure parameters for 2048 point FFT */ 00082 /* Initialise the bit reversal table length */ 00083 Sint->bitRevLength = ARMBITREVINDEXTABLE2048_TABLE_LENGTH; 00084 /* Initialise the bit reversal table pointer */ 00085 Sint->pBitRevTable = (uint16_t *)armBitRevIndexTable2048; 00086 /* Initialise the Twiddle coefficient pointers */ 00087 Sint->pTwiddle = (float32_t *) twiddleCoef_2048 ; 00088 S->pTwiddleRFFT = (float32_t *) twiddleCoef_rfft_4096; 00089 break; 00090 case 1024u: 00091 Sint->bitRevLength = ARMBITREVINDEXTABLE1024_TABLE_LENGTH; 00092 Sint->pBitRevTable = (uint16_t *)armBitRevIndexTable1024; 00093 Sint->pTwiddle = (float32_t *) twiddleCoef_1024 ; 00094 S->pTwiddleRFFT = (float32_t *) twiddleCoef_rfft_2048; 00095 break; 00096 case 512u: 00097 Sint->bitRevLength = ARMBITREVINDEXTABLE_512_TABLE_LENGTH; 00098 Sint->pBitRevTable = (uint16_t *)armBitRevIndexTable512; 00099 Sint->pTwiddle = (float32_t *) twiddleCoef_512 ; 00100 S->pTwiddleRFFT = (float32_t *) twiddleCoef_rfft_1024; 00101 break; 00102 case 256u: 00103 Sint->bitRevLength = ARMBITREVINDEXTABLE_256_TABLE_LENGTH; 00104 Sint->pBitRevTable = (uint16_t *)armBitRevIndexTable256; 00105 Sint->pTwiddle = (float32_t *) twiddleCoef_256 ; 00106 S->pTwiddleRFFT = (float32_t *) twiddleCoef_rfft_512; 00107 break; 00108 case 128u: 00109 Sint->bitRevLength = ARMBITREVINDEXTABLE_128_TABLE_LENGTH; 00110 Sint->pBitRevTable = (uint16_t *)armBitRevIndexTable128; 00111 Sint->pTwiddle = (float32_t *) twiddleCoef_128 ; 00112 S->pTwiddleRFFT = (float32_t *) twiddleCoef_rfft_256; 00113 break; 00114 case 64u: 00115 Sint->bitRevLength = ARMBITREVINDEXTABLE__64_TABLE_LENGTH; 00116 Sint->pBitRevTable = (uint16_t *)armBitRevIndexTable64; 00117 Sint->pTwiddle = (float32_t *) twiddleCoef_64 ; 00118 S->pTwiddleRFFT = (float32_t *) twiddleCoef_rfft_128; 00119 break; 00120 case 32u: 00121 Sint->bitRevLength = ARMBITREVINDEXTABLE__32_TABLE_LENGTH; 00122 Sint->pBitRevTable = (uint16_t *)armBitRevIndexTable32; 00123 Sint->pTwiddle = (float32_t *) twiddleCoef_32 ; 00124 S->pTwiddleRFFT = (float32_t *) twiddleCoef_rfft_64; 00125 break; 00126 case 16u: 00127 Sint->bitRevLength = ARMBITREVINDEXTABLE__16_TABLE_LENGTH; 00128 Sint->pBitRevTable = (uint16_t *)armBitRevIndexTable16; 00129 Sint->pTwiddle = (float32_t *) twiddleCoef_16 ; 00130 S->pTwiddleRFFT = (float32_t *) twiddleCoef_rfft_32; 00131 break; 00132 default: 00133 /* Reporting argument error if fftSize is not valid value */ 00134 status = ARM_MATH_ARGUMENT_ERROR; 00135 break; 00136 } 00137 00138 return (status); 00139 } 00140 00141 /** 00142 * @} end of RealFFT group 00143 */
Generated on Tue Jul 12 2022 11:59:18 by
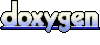