CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
Complex FFT Tables
[Transform Functions]
Variables | |
const uint16_t | armBitRevTable [1024] |
const float32_t | twiddleCoef_16 [32] |
const float32_t | twiddleCoef_32 [64] |
const float32_t | twiddleCoef_64 [128] |
const float32_t | twiddleCoef_128 [256] |
const float32_t | twiddleCoef_256 [512] |
const float32_t | twiddleCoef_512 [1024] |
const float32_t | twiddleCoef_1024 [2048] |
const float32_t | twiddleCoef_2048 [4096] |
const float32_t | twiddleCoef_4096 [8192] |
const q31_t | twiddleCoef_16_q31 [24] |
const q31_t | twiddleCoef_32_q31 [48] |
const q31_t | twiddleCoef_64_q31 [96] |
const q31_t | twiddleCoef_128_q31 [192] |
const q31_t | twiddleCoef_256_q31 [384] |
const q31_t | twiddleCoef_512_q31 [768] |
const q31_t | twiddleCoef_1024_q31 [1536] |
const q31_t | twiddleCoef_2048_q31 [3072] |
const q31_t | twiddleCoef_4096_q31 [6144] |
const q15_t | twiddleCoef_16_q15 [24] |
const q15_t | twiddleCoef_32_q15 [48] |
const q15_t | twiddleCoef_64_q15 [96] |
const q15_t | twiddleCoef_128_q15 [192] |
const q15_t | twiddleCoef_256_q15 [384] |
const q15_t | twiddleCoef_512_q15 [768] |
const q15_t | twiddleCoef_1024_q15 [1536] |
const q15_t | twiddleCoef_2048_q15 [3072] |
const q15_t | twiddleCoef_4096_q15 [6144] |
Variable Documentation
const uint16_t armBitRevTable[1024] |
- Pseudo code for Generation of Bit reversal Table is
for(l=1;l <= N/4;l++) { for(i=0;i<logN2;i++) { a[i]=l&(1<<i); } for(j=0; j<logN2; j++) { if (a[j]!=0) y[l]+=(1<<((logN2-1)-j)); } y[l] = y[l] >> 1; }
- where N = 4096 logN2 = 12
- N is the maximum FFT Size supported
Definition at line 80 of file arm_common_tables.c.
const float32_t twiddleCoef_1024[2048] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 1024 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 1322 of file arm_common_tables.c.
const q15_t twiddleCoef_1024_q15[1536] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 1024 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 15763 of file arm_common_tables.c.
const q31_t twiddleCoef_1024_q31[1536] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 1024 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 9434 of file arm_common_tables.c.
const float32_t twiddleCoef_128[256] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 128 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 373 of file arm_common_tables.c.
const q15_t twiddleCoef_128_q15[192] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 128 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 15028 of file arm_common_tables.c.
const q31_t twiddleCoef_128_q31[192] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 128 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8699 of file arm_common_tables.c.
const float32_t twiddleCoef_16[32] |
{ 1.000000000f, 0.000000000f, 0.923879533f, 0.382683432f, 0.707106781f, 0.707106781f, 0.382683432f, 0.923879533f, 0.000000000f, 1.000000000f, -0.382683432f, 0.923879533f, -0.707106781f, 0.707106781f, -0.923879533f, 0.382683432f, -1.000000000f, 0.000000000f, -0.923879533f, -0.382683432f, -0.707106781f, -0.707106781f, -0.382683432f, -0.923879533f, -0.000000000f, -1.000000000f, 0.382683432f, -0.923879533f, 0.707106781f, -0.707106781f, 0.923879533f, -0.382683432f }
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 16 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 206 of file arm_common_tables.c.
const q15_t twiddleCoef_16_q15[24] |
{ 0x7FFF, 0x0000, 0x7641, 0x30FB, 0x5A82, 0x5A82, 0x30FB, 0x7641, 0x0000, 0x7FFF, 0xCF04, 0x7641, 0xA57D, 0x5A82, 0x89BE, 0x30FB, 0x8000, 0x0000, 0x89BE, 0xCF04, 0xA57D, 0xA57D, 0xCF04, 0x89BE }
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 16 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 14881 of file arm_common_tables.c.
const q31_t twiddleCoef_16_q31[24] |
{ 0x7FFFFFFF, 0x00000000, 0x7641AF3C, 0x30FBC54D, 0x5A82799A, 0x5A82799A, 0x30FBC54D, 0x7641AF3C, 0x00000000, 0x7FFFFFFF, 0xCF043AB2, 0x7641AF3C, 0xA57D8666, 0x5A82799A, 0x89BE50C3, 0x30FBC54D, 0x80000000, 0x00000000, 0x89BE50C3, 0xCF043AB2, 0xA57D8666, 0xA57D8666, 0xCF043AB2, 0x89BE50C3 }
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 16 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8552 of file arm_common_tables.c.
const float32_t twiddleCoef_2048[4096] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 2048 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 2364 of file arm_common_tables.c.
const q15_t twiddleCoef_2048_q15[3072] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 2048 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 16552 of file arm_common_tables.c.
const q31_t twiddleCoef_2048_q31[3072] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 2048 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 10223 of file arm_common_tables.c.
const float32_t twiddleCoef_256[512] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 256 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 519 of file arm_common_tables.c.
const q15_t twiddleCoef_256_q15[384] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 256 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 15145 of file arm_common_tables.c.
const q31_t twiddleCoef_256_q31[384] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 256 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8816 of file arm_common_tables.c.
const float32_t twiddleCoef_32[64] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 32 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 240 of file arm_common_tables.c.
const q15_t twiddleCoef_32_q15[48] |
{ 0x7FFF, 0x0000, 0x7D8A, 0x18F8, 0x7641, 0x30FB, 0x6A6D, 0x471C, 0x5A82, 0x5A82, 0x471C, 0x6A6D, 0x30FB, 0x7641, 0x18F8, 0x7D8A, 0x0000, 0x7FFF, 0xE707, 0x7D8A, 0xCF04, 0x7641, 0xB8E3, 0x6A6D, 0xA57D, 0x5A82, 0x9592, 0x471C, 0x89BE, 0x30FB, 0x8275, 0x18F8, 0x8000, 0x0000, 0x8275, 0xE707, 0x89BE, 0xCF04, 0x9592, 0xB8E3, 0xA57D, 0xA57D, 0xB8E3, 0x9592, 0xCF04, 0x89BE, 0xE707, 0x8275 }
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 32 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 14914 of file arm_common_tables.c.
const q31_t twiddleCoef_32_q31[48] |
{ 0x7FFFFFFF, 0x00000000, 0x7D8A5F3F, 0x18F8B83C, 0x7641AF3C, 0x30FBC54D, 0x6A6D98A4, 0x471CECE6, 0x5A82799A, 0x5A82799A, 0x471CECE6, 0x6A6D98A4, 0x30FBC54D, 0x7641AF3C, 0x18F8B83C, 0x7D8A5F3F, 0x00000000, 0x7FFFFFFF, 0xE70747C3, 0x7D8A5F3F, 0xCF043AB2, 0x7641AF3C, 0xB8E31319, 0x6A6D98A4, 0xA57D8666, 0x5A82799A, 0x9592675B, 0x471CECE6, 0x89BE50C3, 0x30FBC54D, 0x8275A0C0, 0x18F8B83C, 0x80000000, 0x00000000, 0x8275A0C0, 0xE70747C3, 0x89BE50C3, 0xCF043AB2, 0x9592675B, 0xB8E31319, 0xA57D8666, 0xA57D8666, 0xB8E31319, 0x9592675B, 0xCF043AB2, 0x89BE50C3, 0xE70747C3, 0x8275A0C0 }
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 32 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8585 of file arm_common_tables.c.
const float32_t twiddleCoef_4096[8192] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 4096 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 4430 of file arm_common_tables.c.
const q15_t twiddleCoef_4096_q15[6144] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 4096 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 18109 of file arm_common_tables.c.
const q31_t twiddleCoef_4096_q31[6144] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 4096 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 11780 of file arm_common_tables.c.
const float32_t twiddleCoef_512[1024] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 512 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 793 of file arm_common_tables.c.
const q15_t twiddleCoef_512_q15[768] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 512 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 15358 of file arm_common_tables.c.
const q31_t twiddleCoef_512_q31[768] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 512 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 9029 of file arm_common_tables.c.
const float32_t twiddleCoef_64[128] |
- Example code for Floating-point Twiddle factors Generation:
for(i = 0; i< N/; i++) { twiddleCoef[2*i]= cos(i * 2*PI/(float)N); twiddleCoef[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 64 and PI = 3.14159265358979
- Cos and Sin values are in interleaved fashion
Definition at line 290 of file arm_common_tables.c.
const q15_t twiddleCoef_64_q15[96] |
- Example code for q15 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefq15[2*i]= cos(i * 2*PI/(float)N); twiddleCoefq15[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 64 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to q15(Fixed point 1.15): round(twiddleCoefq15(i) * pow(2, 15))
Definition at line 14959 of file arm_common_tables.c.
const q31_t twiddleCoef_64_q31[96] |
- Example code for Q31 Twiddle factors Generation::
for(i = 0; i< 3N/4; i++) { twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); }
- where N = 64 and PI = 3.14159265358979
- Cos and Sin values are interleaved fashion
- Convert Floating point to Q31(Fixed point 1.31): round(twiddleCoefQ31(i) * pow(2, 31))
Definition at line 8630 of file arm_common_tables.c.
Generated on Tue Jul 12 2022 11:59:20 by
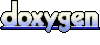