
Rtos API example
GenericGattClient Class Reference
Generic implementation of the GattClient. More...
#include <GenericGattClient.h>
Inherits GattClient.
Public Types | |
enum | WriteOp_t { GATT_OP_WRITE_REQ = 0x01, GATT_OP_WRITE_CMD = 0x02 } |
GATT write operations. More... | |
typedef FunctionPointerWithContext < const GattReadCallbackParams * > | ReadCallback_t |
Attribute read event handler. | |
typedef CallChainOfFunctionPointersWithContext < const GattReadCallbackParams * > | ReadCallbackChain_t |
Callchain of attribute read event handlers. | |
typedef FunctionPointerWithContext < const GattWriteCallbackParams * > | WriteCallback_t |
Attribute write event handler. | |
typedef CallChainOfFunctionPointersWithContext < const GattWriteCallbackParams * > | WriteCallbackChain_t |
Callchain of attribute write event handlers. | |
typedef FunctionPointerWithContext < const GattHVXCallbackParams * > | HVXCallback_t |
Handle value notification/indication event handler. | |
typedef CallChainOfFunctionPointersWithContext < const GattHVXCallbackParams * > | HVXCallbackChain_t |
Callchain of handle value notification/indication event handlers. | |
typedef FunctionPointerWithContext < const GattClient * > | GattClientShutdownCallback_t |
Shutdown event handler. | |
typedef CallChainOfFunctionPointersWithContext < const GattClient * > | GattClientShutdownCallbackChain_t |
Callchain of shutdown event handlers. | |
Public Member Functions | |
GenericGattClient (pal::GattClient *pal_client) | |
Create a GenericGattClient from a pal::GattClient. | |
virtual ble_error_t | launchServiceDiscovery (Gap::Handle_t connection_handle, ServiceDiscovery::ServiceCallback_t service_callback, ServiceDiscovery::CharacteristicCallback_t characteristic_callback, const UUID &matching_service_uuid, const UUID &matching_characteristic_uuid) |
virtual bool | isServiceDiscoveryActive () const |
virtual void | terminateServiceDiscovery () |
virtual ble_error_t | read (Gap::Handle_t connection_handle, GattAttribute::Handle_t attribute_handle, uint16_t offset) const |
virtual ble_error_t | write (GattClient::WriteOp_t cmd, Gap::Handle_t connection_handle, GattAttribute::Handle_t attribute_handle, size_t length, const uint8_t *value) const |
virtual void | onServiceDiscoveryTermination (ServiceDiscovery::TerminationCallback_t callback) |
virtual ble_error_t | discoverCharacteristicDescriptors (const DiscoveredCharacteristic &characteristic, const CharacteristicDescriptorDiscovery::DiscoveryCallback_t &discoveryCallback, const CharacteristicDescriptorDiscovery::TerminationCallback_t &terminationCallback) |
virtual bool | isCharacteristicDescriptorDiscoveryActive (const DiscoveredCharacteristic &characteristic) const |
virtual void | terminateCharacteristicDescriptorDiscovery (const DiscoveredCharacteristic &characteristic) |
virtual ble_error_t | reset (void) |
virtual ble_error_t | discoverServices (Gap::Handle_t connectionHandle, ServiceDiscovery::ServiceCallback_t callback, const UUID &matchingServiceUUID=UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN)) |
Launch the service discovery procedure of a GATT server peer. | |
virtual ble_error_t | discoverServices (Gap::Handle_t connectionHandle, ServiceDiscovery::ServiceCallback_t callback, GattAttribute::Handle_t startHandle, GattAttribute::Handle_t endHandle) |
Launch the service discovery procedure of a GATT server peer. | |
void | onDataRead (ReadCallback_t callback) |
Register an attribute read event handler. | |
ReadCallbackChain_t & | onDataRead () |
Get the callchain of attribute read event handlers. | |
void | onDataWritten (WriteCallback_t callback) |
Register an attribute write event handler. | |
WriteCallbackChain_t & | onDataWritten () |
Get the callchain of attribute write event handlers. | |
MBED_DEPRECATED ("Use GattServer::onDataWritten()") void onDataWrite(WriteCallback_t callback) | |
Register an attribute write event handler. | |
void | onHVX (HVXCallback_t callback) |
Register an handler for Handle Value Notification/Indication events. | |
HVXCallbackChain_t & | onHVX () |
provide access to the callchain of HVX callbacks. | |
void | onShutdown (const GattClientShutdownCallback_t &callback) |
Register a shutdown event handler. | |
template<typename T > | |
void | onShutdown (T *objPtr, void(T::*memberPtr)(const GattClient *)) |
Register a shutdown event handler. | |
GattClientShutdownCallbackChain_t & | onShutdown () |
Get the callchain of shutdown event handlers. | |
void | processReadResponse (const GattReadCallbackParams *params) |
Forward an attribute read event to all registered handlers. | |
void | processWriteResponse (const GattWriteCallbackParams *params) |
Forward an attribute written event to all registered handlers. | |
void | processHVXEvent (const GattHVXCallbackParams *params) |
Forward a handle value notification or indication event to all registered handlers. | |
Protected Attributes | |
ReadCallbackChain_t | onDataReadCallbackChain |
Callchain containing all registered event handlers for data read events. | |
WriteCallbackChain_t | onDataWriteCallbackChain |
Callchain containing all registered event handlers for data write events. | |
HVXCallbackChain_t | onHVXCallbackChain |
Callchain containing all registered event handlers for update events. | |
GattClientShutdownCallbackChain_t | shutdownCallChain |
Callchain containing all registered event handlers for shutdown events. |
Detailed Description
Generic implementation of the GattClient.
It requires a pal::GattClient injected at construction site. : Not part of the public interface of BLE API.
Definition at line 41 of file GenericGattClient.h.
Member Typedef Documentation
typedef FunctionPointerWithContext<const GattClient *> GattClientShutdownCallback_t [inherited] |
Shutdown event handler.
- See also:
- GattClient::onShutdown().
Definition at line 159 of file GattClient.h.
typedef CallChainOfFunctionPointersWithContext<const GattClient *> GattClientShutdownCallbackChain_t [inherited] |
Callchain of shutdown event handlers.
- See also:
- to GattClient::onShutown().
Definition at line 168 of file GattClient.h.
typedef FunctionPointerWithContext<const GattHVXCallbackParams*> HVXCallback_t [inherited] |
Handle value notification/indication event handler.
- See also:
- to GattClient::onHVX().
Definition at line 143 of file GattClient.h.
typedef CallChainOfFunctionPointersWithContext<const GattHVXCallbackParams*> HVXCallbackChain_t [inherited] |
Callchain of handle value notification/indication event handlers.
- See also:
- GattClient::onHVX().
Definition at line 151 of file GattClient.h.
typedef FunctionPointerWithContext<const GattReadCallbackParams*> ReadCallback_t [inherited] |
Attribute read event handler.
- See also:
- GattClient::onDataRead().
Definition at line 92 of file GattClient.h.
typedef CallChainOfFunctionPointersWithContext<const GattReadCallbackParams*> ReadCallbackChain_t [inherited] |
Callchain of attribute read event handlers.
Definition at line 98 of file GattClient.h.
typedef FunctionPointerWithContext<const GattWriteCallbackParams*> WriteCallback_t [inherited] |
Attribute write event handler.
- See also:
- GattClient::onDataWrite().
Definition at line 127 of file GattClient.h.
typedef CallChainOfFunctionPointersWithContext<const GattWriteCallbackParams*> WriteCallbackChain_t [inherited] |
Callchain of attribute write event handlers.
- See also:
- GattClient::onDataWrite().
Definition at line 135 of file GattClient.h.
Member Enumeration Documentation
enum WriteOp_t [inherited] |
GATT write operations.
- Enumerator:
Definition at line 103 of file GattClient.h.
Constructor & Destructor Documentation
GenericGattClient | ( | pal::GattClient * | pal_client ) |
Create a GenericGattClient from a pal::GattClient.
Definition at line 892 of file GenericGattClient.cpp.
Member Function Documentation
ble_error_t discoverCharacteristicDescriptors | ( | const DiscoveredCharacteristic & | characteristic, |
const CharacteristicDescriptorDiscovery::DiscoveryCallback_t & | discoveryCallback, | ||
const CharacteristicDescriptorDiscovery::TerminationCallback_t & | terminationCallback | ||
) | [virtual] |
Reimplemented from GattClient.
Definition at line 1108 of file GenericGattClient.cpp.
virtual ble_error_t discoverServices | ( | Gap::Handle_t | connectionHandle, |
ServiceDiscovery::ServiceCallback_t | callback, | ||
const UUID & | matchingServiceUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN) |
||
) | [virtual, inherited] |
Launch the service discovery procedure of a GATT server peer.
The procedure invokes the application callback for matching services. The process ends after all the services present on the distant GATT server have been discovered. Termination callbacks registered with onServiceDiscoveryTermination() are invoked to notify the application of the termination of the procedure.
Application code can track the status of the procedure by invoking the function isServiceDiscoveryActive(), which returns true if the procedure is ongoing.
At any point, application code can prematurely terminate the discovery procedure by calling terminateServiceDiscovery().
- Parameters:
-
[in] connectionHandle Handle of the connection with the peer GATT server. [in] callback Service discovered event handler invoked when a matching service has been discovered. This parameter may be NULL. [in] matchingServiceUUID UUID of the service the caller is interested in. If a service discovered matches this filter, then sc
is invoked with it. The special value BLE_UUID_UNKNOWN act is a wildcard, which can be used to discover all services present on the peer GATT server.
- Returns:
- BLE_ERROR_NONE if the discovery procedure has been successfully started and an appropriate error otherwise.
Definition at line 276 of file GattClient.h.
virtual ble_error_t discoverServices | ( | Gap::Handle_t | connectionHandle, |
ServiceDiscovery::ServiceCallback_t | callback, | ||
GattAttribute::Handle_t | startHandle, | ||
GattAttribute::Handle_t | endHandle | ||
) | [virtual, inherited] |
Launch the service discovery procedure of a GATT server peer.
The process ends after all the services present in the attribute range startHandle
to endHandle
have been discovered.
Termination callbacks registered with onServiceDiscoveryTermination() are invoked to notify the application of the termination of the procedure.
Application code can track the status of the procedure by invoking the function isServiceDiscoveryActive(), which returns true if the procedure is ongoing.
At any point, application code can prematurely terminate the discovery procedure by calling terminateServiceDiscovery().
- Parameters:
-
[in] connectionHandle Handle of the connection with the peer GATT server. [in] callback Service discovered event handler invoked when a matching service has been discovered. This parameter may be NULL. [in] startHandle First attribute handle of the discovery range. [in] endHandle end Lasr attribute handle of the discovery range.
- Returns:
- BLE_ERROR_NONE if the discovery procedure has been successfully started and an appropriate error otherwise.
Definition at line 317 of file GattClient.h.
bool isCharacteristicDescriptorDiscoveryActive | ( | const DiscoveredCharacteristic & | characteristic ) | const [virtual] |
Reimplemented from GattClient.
Definition at line 1152 of file GenericGattClient.cpp.
bool isServiceDiscoveryActive | ( | void | ) | const [virtual] |
- See also:
- GattClient::isServiceDiscoveryActive
Reimplemented from GattClient.
Definition at line 961 of file GenericGattClient.cpp.
ble_error_t launchServiceDiscovery | ( | Gap::Handle_t | connection_handle, |
ServiceDiscovery::ServiceCallback_t | service_callback, | ||
ServiceDiscovery::CharacteristicCallback_t | characteristic_callback, | ||
const UUID & | matching_service_uuid, | ||
const UUID & | matching_characteristic_uuid | ||
) | [virtual] |
- See also:
- GattClient::launchServiceDiscovery
Reimplemented from GattClient.
Definition at line 904 of file GenericGattClient.cpp.
MBED_DEPRECATED | ( | "Use GattServer::onDataWritten()" | ) | [inherited] |
Register an attribute write event handler.
- Parameters:
-
[in] callback Event handler being registered.
- Note:
- It is possible to remove registered handlers using onDataWritten().detach(callbackToRemove).
- Write commands (issued using writeWoResponse) don't generate a response.
Definition at line 538 of file GattClient.h.
void onDataRead | ( | ReadCallback_t | callback ) | [inherited] |
Register an attribute read event handler.
- Note:
- It is possible to unregister a callback using onDataRead().detach(callbackToRemove).
- Parameters:
-
[in] callback Event handler being registered.
Definition at line 472 of file GattClient.h.
ReadCallbackChain_t& onDataRead | ( | ) | [inherited] |
Get the callchain of attribute read event handlers.
- Returns:
- A reference to the read event callback chain.
- Note:
- It is possible to register new handlers using onDataRead().add(callback).
- It is possible to unregister an handler by using onDataRead().detach(callback).
Definition at line 488 of file GattClient.h.
WriteCallbackChain_t& onDataWritten | ( | ) | [inherited] |
Get the callchain of attribute write event handlers.
- Returns:
- A reference to the data written callbacks chain.
- Note:
- It is possible to register new handlers by using onDataWritten().add(callback).
- It is possible to unregister an handler by using onDataWritten().detach(callback).
Definition at line 520 of file GattClient.h.
void onDataWritten | ( | WriteCallback_t | callback ) | [inherited] |
Register an attribute write event handler.
- Parameters:
-
[in] callback Event handler being registered.
- Note:
- It is possible to remove registered handlers using onDataWritten().detach(callbackToRemove).
- Write commands (issued using writeWoResponse) don't generate a response.
Definition at line 504 of file GattClient.h.
void onHVX | ( | HVXCallback_t | callback ) | [inherited] |
Register an handler for Handle Value Notification/Indication events.
- Parameters:
-
callback Event handler to register.
- Note:
- It is possible to unregister a callback by using onHVX().detach(callbackToRemove).
Definition at line 637 of file GattClient.h.
HVXCallbackChain_t& onHVX | ( | ) | [inherited] |
provide access to the callchain of HVX callbacks.
- Returns:
- A reference to the HVX callbacks chain.
- Note:
- It is possible to register callbacks using onHVX().add(callback).
- It is possible to unregister callbacks using onHVX().detach(callback).
Definition at line 700 of file GattClient.h.
void onServiceDiscoveryTermination | ( | ServiceDiscovery::TerminationCallback_t | callback ) | [virtual] |
Reimplemented from GattClient.
Definition at line 1102 of file GenericGattClient.cpp.
void onShutdown | ( | const GattClientShutdownCallback_t & | callback ) | [inherited] |
Register a shutdown event handler.
The registered handler is invoked when the GattClient instance is about to be shut down.
- Parameters:
-
[in] callback Event handler to invoke when a shutdown event is available.
- Note:
- onShutdown().detach(callback) may be used to unregister a given callback.
- See also:
- BLE::shutdown()
Definition at line 656 of file GattClient.h.
void onShutdown | ( | T * | objPtr, |
void(T::*)(const GattClient *) | memberPtr | ||
) | [inherited] |
Register a shutdown event handler.
The registered handler is invoked when the GattClient instance is about to be shut down.
- Parameters:
-
[in] objPtr Instance that will be used to invoke memberPtr
.[in] memberPtr Event handler to invoke when a shutdown event is available.
Definition at line 672 of file GattClient.h.
GattClientShutdownCallbackChain_t& onShutdown | ( | ) | [inherited] |
Get the callchain of shutdown event handlers.
- Returns:
- A reference to the shutdown event callbacks chain.
- Note:
- onShutdown().add(callback) may be used to register new handlers.
- onShutdown().detach(callback) may be used to unregister an handler.
Definition at line 686 of file GattClient.h.
void processHVXEvent | ( | const GattHVXCallbackParams * | params ) | [inherited] |
Forward a handle value notification or indication event to all registered handlers.
This function is meant to be called from the vendor implementation when a notification or indication event is available.
- Parameters:
-
[in] params Notification or Indication event to pass to the registered handlers.
Definition at line 780 of file GattClient.h.
void processReadResponse | ( | const GattReadCallbackParams * | params ) | [inherited] |
Forward an attribute read event to all registered handlers.
This function is meant to be called from the vendor implementation when an attribute read event occurs.
- Parameters:
-
[in] params Attribute read event to pass to the registered handlers.
Definition at line 751 of file GattClient.h.
void processWriteResponse | ( | const GattWriteCallbackParams * | params ) | [inherited] |
Forward an attribute written event to all registered handlers.
This function is meant to be called from the vendor implementation when an attribute written event occurs.
- Parameters:
-
[in] params Attribute written event to pass to the registered handlers.
Definition at line 765 of file GattClient.h.
ble_error_t read | ( | Gap::Handle_t | connection_handle, |
GattAttribute::Handle_t | attribute_handle, | ||
uint16_t | offset | ||
) | const [virtual] |
- See also:
- GattClient::read
Reimplemented from GattClient.
Definition at line 985 of file GenericGattClient.cpp.
ble_error_t reset | ( | void | ) | [virtual] |
- See also:
- GattClient::reset
Reimplemented from GattClient.
Definition at line 1188 of file GenericGattClient.cpp.
void terminateCharacteristicDescriptorDiscovery | ( | const DiscoveredCharacteristic & | characteristic ) | [virtual] |
Reimplemented from GattClient.
Definition at line 1168 of file GenericGattClient.cpp.
void terminateServiceDiscovery | ( | void | ) | [virtual] |
Reimplemented from GattClient.
Definition at line 974 of file GenericGattClient.cpp.
ble_error_t write | ( | GattClient::WriteOp_t | cmd, |
Gap::Handle_t | connection_handle, | ||
GattAttribute::Handle_t | attribute_handle, | ||
size_t | length, | ||
const uint8_t * | value | ||
) | const [virtual] |
- See also:
- GattClient::write
Reimplemented from GattClient.
Definition at line 1027 of file GenericGattClient.cpp.
Field Documentation
ReadCallbackChain_t onDataReadCallbackChain [protected, inherited] |
Callchain containing all registered event handlers for data read events.
Definition at line 792 of file GattClient.h.
WriteCallbackChain_t onDataWriteCallbackChain [protected, inherited] |
Callchain containing all registered event handlers for data write events.
Definition at line 798 of file GattClient.h.
HVXCallbackChain_t onHVXCallbackChain [protected, inherited] |
Callchain containing all registered event handlers for update events.
Definition at line 804 of file GattClient.h.
GattClientShutdownCallbackChain_t shutdownCallChain [protected, inherited] |
Callchain containing all registered event handlers for shutdown events.
Definition at line 810 of file GattClient.h.
Generated on Sun Jul 17 2022 08:25:42 by
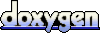