
Rtos API example
Gap Class Reference
[Gap]
Define device discovery, connection and link management procedures. More...
#include <Gap.h>
Data Structures | |
struct | AdvertisementCallbackParams_t |
Representation of a scanned advertising packet. More... | |
struct | ConnectionCallbackParams_t |
Connection events. More... | |
struct | ConnectionParams_t |
Parameters of a BLE connection. More... | |
struct | DisconnectionCallbackParams_t |
Disconnection event. More... | |
struct | GapState_t |
Description of the states of the device. More... | |
struct | Whitelist_t |
Representation of a whitelist of addresses. More... | |
Public Types | |
enum | DeprecatedAddressType_t |
Address-type for BLEProtocol addresses. More... | |
enum | TimeoutSource_t { TIMEOUT_SRC_ADVERTISING = 0x00, TIMEOUT_SRC_SECURITY_REQUEST = 0x01, TIMEOUT_SRC_SCAN = 0x02, TIMEOUT_SRC_CONN = 0x03 } |
Enumeration of possible timeout sources. More... | |
enum | DisconnectionReason_t { CONNECTION_TIMEOUT = 0x08, REMOTE_USER_TERMINATED_CONNECTION = 0x13, REMOTE_DEV_TERMINATION_DUE_TO_LOW_RESOURCES = 0x14, REMOTE_DEV_TERMINATION_DUE_TO_POWER_OFF = 0x15, LOCAL_HOST_TERMINATED_CONNECTION = 0x16, CONN_INTERVAL_UNACCEPTABLE = 0x3B } |
Enumeration of disconnection reasons. More... | |
enum | AdvertisingPolicyMode_t { ADV_POLICY_IGNORE_WHITELIST = 0, ADV_POLICY_FILTER_SCAN_REQS = 1, ADV_POLICY_FILTER_CONN_REQS = 2, ADV_POLICY_FILTER_ALL_REQS = 3 } |
Advertising policy filter modes. More... | |
enum | ScanningPolicyMode_t { SCAN_POLICY_IGNORE_WHITELIST = 0, SCAN_POLICY_FILTER_ALL_ADV = 1 } |
Scanning policy filter mode. More... | |
enum | InitiatorPolicyMode_t { INIT_POLICY_IGNORE_WHITELIST = 0, INIT_POLICY_FILTER_ALL_ADV = 1 } |
Connection initiation policy filter mode. More... | |
enum | Role_t { PERIPHERAL = 0x1, CENTRAL = 0x2 } |
Enumeration of GAP roles. More... | |
typedef BLEProtocol::AddressType_t | AddressType_t |
Address-type for BLEProtocol addresses. | |
typedef BLEProtocol::AddressType_t | addr_type_t |
Address-type for BLEProtocol addresses. | |
typedef BLEProtocol::AddressBytes_t | Address_t |
48-bit address, LSB format. | |
typedef BLEProtocol::AddressBytes_t | address_t |
48-bit address, LSB format. | |
typedef ble::connection_handle_t | Handle_t |
Opaque value type representing a connection handle. | |
typedef FunctionPointerWithContext < const AdvertisementCallbackParams_t * > | AdvertisementReportCallback_t |
Type of the callback handling scanned advertisement packets. | |
typedef FunctionPointerWithContext < TimeoutSource_t > | TimeoutEventCallback_t |
Timeout event handler. | |
typedef CallChainOfFunctionPointersWithContext < TimeoutSource_t > | TimeoutEventCallbackChain_t |
Callchain of timeout event handlers. | |
typedef FunctionPointerWithContext < const ConnectionCallbackParams_t * > | ConnectionEventCallback_t |
Connection event handler. | |
typedef CallChainOfFunctionPointersWithContext < const ConnectionCallbackParams_t * > | ConnectionEventCallbackChain_t |
Callchain of connection event handlers. | |
typedef FunctionPointerWithContext < const DisconnectionCallbackParams_t * > | DisconnectionEventCallback_t |
Disconnection event handler. | |
typedef CallChainOfFunctionPointersWithContext < const DisconnectionCallbackParams_t * > | DisconnectionEventCallbackChain_t |
Callchain of disconnection event handlers. | |
typedef FunctionPointerWithContext < bool > | RadioNotificationEventCallback_t |
Radio notification event handler. | |
typedef FunctionPointerWithContext < const Gap * > | GapShutdownCallback_t |
Gap shutdown event handler. | |
typedef CallChainOfFunctionPointersWithContext < const Gap * > | GapShutdownCallbackChain_t |
Callchain of gap shutdown event handler. | |
Public Member Functions | |
virtual ble_error_t | setAddress (BLEProtocol::AddressType_t type, const BLEProtocol::AddressBytes_t address) |
Set the device MAC address and type. | |
virtual ble_error_t | getAddress (BLEProtocol::AddressType_t *typeP, BLEProtocol::AddressBytes_t address) |
Fetch the current address and its type. | |
virtual uint16_t | getMinAdvertisingInterval (void) const |
Get the minimum advertising interval in milliseconds, which can be used for connectable advertising types. | |
virtual uint16_t | getMinNonConnectableAdvertisingInterval (void) const |
Get the minimum advertising interval in milliseconds, which can be used for nonconnectable advertising type. | |
virtual uint16_t | getMaxAdvertisingInterval (void) const |
Get the maximum advertising interval in milliseconds. | |
virtual ble_error_t | stopAdvertising (void) |
Stop the ongoing advertising procedure. | |
virtual ble_error_t | stopScan () |
Stop the ongoing scanning procedure. | |
virtual ble_error_t | connect (const BLEProtocol::AddressBytes_t peerAddr, BLEProtocol::AddressType_t peerAddrType, const ConnectionParams_t *connectionParams, const GapScanningParams *scanParams) |
Initiate a connection to a peer. | |
MBED_DEPRECATED ("Gap::DeprecatedAddressType_t is deprecated, use BLEProtocol::AddressType_t instead") ble_error_t connect(const BLEProtocol | |
Initiate a connection to a peer. | |
virtual ble_error_t | disconnect (Handle_t connectionHandle, DisconnectionReason_t reason) |
Initiate a disconnection procedure. | |
MBED_DEPRECATED ("Use disconnect(Handle_t, DisconnectionReason_t) instead.") virtual ble_error_t disconnect(DisconnectionReason_t reason) | |
Initiate a disconnection procedure. | |
virtual ble_error_t | getPreferredConnectionParams (ConnectionParams_t *params) |
Returned the preferred connection parameters exposed in the GATT Generic Access Service. | |
virtual ble_error_t | setPreferredConnectionParams (const ConnectionParams_t *params) |
Set the value of the preferred connection parameters exposed in the GATT Generic Access Service. | |
virtual ble_error_t | updateConnectionParams (Handle_t handle, const ConnectionParams_t *params) |
Update connection parameters of an existing connection. | |
virtual ble_error_t | setDeviceName (const uint8_t *deviceName) |
Set the value of the device name characteristic in the Generic Access Service. | |
virtual ble_error_t | getDeviceName (uint8_t *deviceName, unsigned *lengthP) |
Get the value of the device name characteristic in the Generic Access Service. | |
virtual ble_error_t | setAppearance (GapAdvertisingData::Appearance appearance) |
Set the value of the appearance characteristic in the GAP service. | |
virtual ble_error_t | getAppearance (GapAdvertisingData::Appearance *appearanceP) |
Get the value of the appearance characteristic in the GAP service. | |
virtual ble_error_t | setTxPower (int8_t txPower) |
Set the radio's transmit power. | |
virtual void | getPermittedTxPowerValues (const int8_t **valueArrayPP, size_t *countP) |
Query the underlying stack for allowed Tx power values. | |
virtual uint8_t | getMaxWhitelistSize (void) const |
Get the maximum size of the whitelist. | |
virtual ble_error_t | getWhitelist (Whitelist_t &whitelist) const |
Get the Link Layer to use the internal whitelist when scanning, advertising or initiating a connection depending on the filter policies. | |
virtual ble_error_t | setWhitelist (const Whitelist_t &whitelist) |
Set the value of the whitelist to be used during GAP procedures. | |
virtual ble_error_t | setAdvertisingPolicyMode (AdvertisingPolicyMode_t mode) |
Set the advertising policy filter mode to be used during the next advertising procedure. | |
virtual ble_error_t | setScanningPolicyMode (ScanningPolicyMode_t mode) |
Set the scan policy filter mode to be used during the next scan procedure. | |
virtual ble_error_t | setInitiatorPolicyMode (InitiatorPolicyMode_t mode) |
Set the initiator policy filter mode to be used during the next connection initiation. | |
virtual AdvertisingPolicyMode_t | getAdvertisingPolicyMode (void) const |
Get the current advertising policy filter mode. | |
virtual ScanningPolicyMode_t | getScanningPolicyMode (void) const |
Get the current scan policy filter mode. | |
virtual InitiatorPolicyMode_t | getInitiatorPolicyMode (void) const |
Get the current initiator policy filter mode. | |
GapState_t | getState (void) const |
Get the current advertising and connection states of the device. | |
void | setAdvertisingType (GapAdvertisingParams::AdvertisingType_t advType) |
Set the advertising type to use during the advertising procedure. | |
void | setAdvertisingInterval (uint16_t interval) |
Set the advertising interval. | |
void | setAdvertisingTimeout (uint16_t timeout) |
Set the advertising duration. | |
ble_error_t | startAdvertising (void) |
Start the advertising procedure. | |
void | clearAdvertisingPayload (void) |
Reset the value of the advertising payload advertised. | |
ble_error_t | accumulateAdvertisingPayload (uint8_t flags) |
Set gap flags in the advertising payload. | |
ble_error_t | accumulateAdvertisingPayload (GapAdvertisingData::Appearance app) |
Set the appearance field in the advertising payload. | |
ble_error_t | accumulateAdvertisingPayloadTxPower (int8_t power) |
Set the Tx Power field in the advertising payload. | |
ble_error_t | accumulateAdvertisingPayload (GapAdvertisingData::DataType type, const uint8_t *data, uint8_t len) |
Add a new field in the advertising payload. | |
ble_error_t | updateAdvertisingPayload (GapAdvertisingData::DataType type, const uint8_t *data, uint8_t len) |
Update a particular field in the advertising payload. | |
ble_error_t | setAdvertisingPayload (const GapAdvertisingData &payload) |
Set the value of the payload advertised. | |
const GapAdvertisingData & | getAdvertisingPayload (void) const |
Get a reference to the current advertising payload. | |
ble_error_t | accumulateScanResponse (GapAdvertisingData::DataType type, const uint8_t *data, uint8_t len) |
Add a new field in the advertising payload. | |
void | clearScanResponse (void) |
Reset the content of the scan response. | |
ble_error_t | setScanParams (uint16_t interval=GapScanningParams::SCAN_INTERVAL_MAX, uint16_t window=GapScanningParams::SCAN_WINDOW_MAX, uint16_t timeout=0, bool activeScanning=false) |
Set the parameters used during a scan procedure. | |
ble_error_t | setScanInterval (uint16_t interval) |
Set the interval parameter used during scanning procedures. | |
ble_error_t | setScanWindow (uint16_t window) |
Set the window parameter used during scanning procedures. | |
ble_error_t | setScanTimeout (uint16_t timeout) |
Set the timeout parameter used during scanning procedures. | |
ble_error_t | setActiveScanning (bool activeScanning) |
Enable or disable active scanning. | |
ble_error_t | startScan (void(*callback)(const AdvertisementCallbackParams_t *params)) |
Start the scanning procedure. | |
template<typename T > | |
ble_error_t | startScan (T *object, void(T::*callbackMember)(const AdvertisementCallbackParams_t *params)) |
Start the scanning procedure. | |
virtual ble_error_t | initRadioNotification (void) |
Enable radio-notification events. | |
GapAdvertisingParams & | getAdvertisingParams (void) |
Get the current advertising parameters. | |
const GapAdvertisingParams & | getAdvertisingParams (void) const |
Const alternative to Gap::getAdvertisingParams(). | |
void | setAdvertisingParams (const GapAdvertisingParams &newParams) |
Set the advertising parameters. | |
void | onTimeout (TimeoutEventCallback_t callback) |
Register a callback handling timeout events. | |
TimeoutEventCallbackChain_t & | onTimeout () |
Get the callchain of registered timeout event handlers. | |
void | onConnection (ConnectionEventCallback_t callback) |
Register a callback handling connection events. | |
template<typename T > | |
void | onConnection (T *tptr, void(T::*mptr)(const ConnectionCallbackParams_t *)) |
Register a callback handling connection events. | |
ConnectionEventCallbackChain_t & | onConnection () |
Get the callchain of registered connection event handlers. | |
void | onDisconnection (DisconnectionEventCallback_t callback) |
Register a callback handling disconnection events. | |
template<typename T > | |
void | onDisconnection (T *tptr, void(T::*mptr)(const DisconnectionCallbackParams_t *)) |
Register a callback handling disconnection events. | |
DisconnectionEventCallbackChain_t & | onDisconnection () |
Get the callchain of registered disconnection event handlers. | |
void | onRadioNotification (void(*callback)(bool param)) |
Set the radio-notification events handler. | |
template<typename T > | |
void | onRadioNotification (T *tptr, void(T::*mptr)(bool)) |
Set the radio-notification events handler. | |
void | onShutdown (const GapShutdownCallback_t &callback) |
Register a Gap shutdown event handler. | |
template<typename T > | |
void | onShutdown (T *objPtr, void(T::*memberPtr)(const Gap *)) |
Register a Gap shutdown event handler. | |
GapShutdownCallbackChain_t & | onShutdown () |
Access the callchain of shutdown event handler. | |
virtual ble_error_t | reset (void) |
Reset the Gap instance. | |
void | processConnectionEvent (Handle_t handle, Role_t role, BLEProtocol::AddressType_t peerAddrType, const BLEProtocol::AddressBytes_t peerAddr, BLEProtocol::AddressType_t ownAddrType, const BLEProtocol::AddressBytes_t ownAddr, const ConnectionParams_t *connectionParams) |
Notify all registered connection event handlers of a connection event. | |
void | processDisconnectionEvent (Handle_t handle, DisconnectionReason_t reason) |
Notify all registered disconnection event handlers of a disconnection event. | |
void | processAdvertisementReport (const BLEProtocol::AddressBytes_t peerAddr, int8_t rssi, bool isScanResponse, GapAdvertisingParams::AdvertisingType_t type, uint8_t advertisingDataLen, const uint8_t *advertisingData) |
Forward a received advertising packet to all registered event handlers listening for scanned packet events. | |
void | processTimeoutEvent (TimeoutSource_t source) |
Notify the occurrence of a timeout event to all registered timeout events handler. | |
Static Public Member Functions | |
static uint16_t | MSEC_TO_GAP_DURATION_UNITS (uint32_t durationInMillis) |
Convert milliseconds into 1.25ms units. | |
Static Public Attributes | |
static const unsigned | ADDR_LEN = BLEProtocol::ADDR_LEN |
Length (in octets) of the BLE MAC address. | |
static const uint16_t | UNIT_1_25_MS = 1250 |
Number of microseconds in 1.25 milliseconds. | |
Protected Member Functions | |
virtual ble_error_t | startRadioScan (const GapScanningParams &scanningParams) |
Start scanning procedure in the underlying BLE stack. | |
Gap () | |
Construct a Gap instance. | |
Protected Attributes | |
GapAdvertisingParams | _advParams |
Current advertising parameters. | |
GapAdvertisingData | _advPayload |
Current advertising data. | |
GapScanningParams | _scanningParams |
Current scanning parameters. | |
GapAdvertisingData | _scanResponse |
Current scan response. | |
uint8_t | connectionCount |
Number of open connections. | |
GapState_t | state |
Current GAP state. | |
bool | scanningActive |
Active scanning flag. | |
TimeoutEventCallbackChain_t | timeoutCallbackChain |
Callchain containing all registered callback handlers for timeout events. | |
RadioNotificationEventCallback_t | radioNotificationCallback |
The registered callback handler for radio notification events. | |
AdvertisementReportCallback_t | onAdvertisementReport |
The registered callback handler for scanned advertisement packet notifications. | |
ConnectionEventCallbackChain_t | connectionCallChain |
Callchain containing all registered callback handlers for connection events. | |
DisconnectionEventCallbackChain_t | disconnectionCallChain |
Callchain containing all registered callback handlers for disconnection events. |
Detailed Description
Define device discovery, connection and link management procedures.
- Device discovery: A device can advertise nearby peers of its existence, identity and capabilities. Similarly, a device can scan its environment to find advertising peers. The information acquired during the scan helps to identify peers and understand their use. A scanner may acquire more information about an advertising peer by sending a scan request. If the peer accepts scan requests, it may reply with additional information about its state.
- Connection: A bluetooth device can establish a connection to a connectable advertising peer. Once the connection is established, both devices can communicate using the GATT protocol. The GATT protocol allows connected devices to expose a set of states that the other peer can discover, read and write.
- Link Management: Connected devices may drop the connection and may adjust connection parameters according to the power envelop needed for their application.
- Accessing gap
Instance of a Gap class for a given BLE device should be accessed using BLE::gap(). The reference returned remains valid until the BLE instance shut down (see BLE::shutdown()).
- Advertising
Advertising consists of broadcasting at a regular interval a small amount of data containing valuable informations about the device. These packets may be scanned by peer devices listening on BLE advertising channels.
Scanners may also request additional information from a device advertising by sending a scan request. If the broadcaster accepts scan requests, it can reply with a scan response packet containing additional information.
// assuming gap has been initialized Gap& gap; // construct the packet to advertise GapAdvertisingData advertising_data; // Add advertiser flags advertising_data.addFlags( GapAdvertisingData::LE_GENERAL_DISCOVERABLE | GapAdvertisingData::BREDR_NOT_SUPPORTED ); // Add the name of the device to the advertising data static const uint8_t device_name[] = "HRM"; advertising_data.addData( GapAdvertisingData::COMPLETE_LOCAL_NAME, device_name, sizeof(device_name) ); // set the advertising data in the gap instance, they will be used when // advertising starts. gap.setAdvertisingPayload(advertising_data); // Configure the advertising procedure GapAdvertisingParams advertising_params( GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED, // type of advertising GapAdvertisingParams::MSEC_TO_ADVERTISEMENT_DURATION_UNITS(1000), // interval 0 // The advertising procedure will not timeout ); gap.setAdvertisingParams(advertising_params); // start the advertising procedure, the device will advertise its flag and the // name "HRM". Other peers will also be allowed to connect to it. gap.startAdvertising();
- Scanning
Scanning consist of listening for peer advertising packets. From a scan, a device can identify devices available in its environment.
If the device scans actively, then it will send scan request to scannable advertisers and collect their scan response.
// assuming gap has been initialized Gap& gap; // Handle advertising packet by dumping their content void handle_advertising_packet(const AdvertisementCallbackParams_t* packet) { printf("Packet received: \r\n"); printf(" - peer address: %02X:%02X:%02X:%02X:%02X:%02X\r\n", packet->peerAddr[5], packet->peerAddr[4], packet->peerAddr[3], packet->peerAddr[2], packet->peerAddr[1], packet->peerAddr[0]); printf(" - rssi: %d", packet->rssi); printf(" - scan response: %s\r\n", packet->isScanresponse ? "true" : "false"); printf(" - advertising type: %d\r\n", packet->type); printf(" - advertising type: %d\r\n", packet->type); printf(" - Advertising data: \r\n"); // parse advertising data, it is a succession of AD structures where // the first byte is the size of the AD structure, the second byte the // type of the data and remaining bytes are the value. for (size_t i = 0; i < packet->advertisingDataLen; i += packet->advertisingData[i]) { printf(" - type: 0X%02X, data: ", packet->advertisingData[i + 1]); for (size_t j = 0; j < packet->advertisingData[i] - 2; ++j) { printf("0X%02X ", packet->advertisingData[i + 2 + j]); } printf("\r\n"); } } // set the scan parameters gap.setScanParams( 100, // interval between two scan window in ms 50, // scan window: period during which the device listen for advertising packets. 0, // the scan process never ends true // the device sends scan request to scannable peers. ); // start the scan procedure gap.startScan(handle_advertising_packet);
- Connection event handling
A peer may connect device advertising connectable packets. The advertising procedure ends as soon as the device is connected.
A device accepting a connection request from a peer is named a peripheral, and the device initiating the connection is named a central.
Peripheral and central receive a connection event when the connection is effective.
Gap& gap; // handle connection event void when_connected(const ConnectionCallbackParams_t *connection_event) { // If this callback is entered, then the connection to a peer is effective. } // register connection event handler, which will be invoked whether the device // acts as a central or a peripheral gap.onConnection(when_connected);
- Connection initiation
Connection is initiated central devices.
// assuming gap has been initialized Gap& gap; // Handle the connection event void handle_connection(const ConnectionCallbackParams_t* connection_event) { // event handling } // Handle advertising packet: connect to the first connectable device void handle_advertising_packet(const AdvertisementCallbackParams_t* packet) { if (packet->type != GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED) { return; } // register connection event handler gap.onConnection(handle_connection); Gap::ConnectionParams_t connection_parameters = { 50, // min connection interval 100, // max connection interval 0, // slave latency 600 // connection supervision timeout }; // scan parameter used to find the device to connect to GapScanningParams scanning_params( 100, // interval 100, // window 0, // timeout false // active ); // Initiate the connection procedure gap.connect( packet->peerAddr, BLEProtocol::RANDOM_STATIC, &connection_parameters, &scanning_params ); } // set the scan parameters gap.setScanParams( 100, // interval between two scan window in ms 50, // scan window: period during which the device listen for advertising packets. 0, // the scan process never ends true // the device sends scan request to scannable peers. ); // start the scan procedure gap.startScan(handle_advertising_packet);
- disconnection
The application code initiates a disconnection when it calls the disconnect(Handle_t, DisconnectionReason_t) function.
Disconnection may also be initiated by the remote peer or the local controller/stack. To catch all disconnection events, application code may set up an handler taking care of disconnection events by calling onDisconnection().
Definition at line 268 of file Gap.h.
Member Typedef Documentation
Address-type for BLEProtocol addresses.
typedef BLEProtocol::AddressBytes_t address_t |
typedef BLEProtocol::AddressBytes_t Address_t |
Address-type for BLEProtocol addresses.
typedef FunctionPointerWithContext<const AdvertisementCallbackParams_t *> AdvertisementReportCallback_t |
Type of the callback handling scanned advertisement packets.
- See also:
- Gap::startScan().
typedef CallChainOfFunctionPointersWithContext<const ConnectionCallbackParams_t *> ConnectionEventCallbackChain_t |
Callchain of connection event handlers.
- See also:
- Gap::onConnection().
typedef CallChainOfFunctionPointersWithContext<const DisconnectionCallbackParams_t*> DisconnectionEventCallbackChain_t |
Callchain of disconnection event handlers.
- See also:
- Gap::onDisconnection().
typedef FunctionPointerWithContext<const Gap *> GapShutdownCallback_t |
typedef CallChainOfFunctionPointersWithContext<const Gap *> GapShutdownCallbackChain_t |
Callchain of gap shutdown event handler.
- See also:
- Gap::onShutdown().
typedef ble::connection_handle_t Handle_t |
Opaque value type representing a connection handle.
It is used to identify to refer to a specific connection across Gap, GattClient and GattEvent API.
- Note:
- instances are generated by in the connection callback.
typedef FunctionPointerWithContext<bool> RadioNotificationEventCallback_t |
Radio notification event handler.
- See also:
- Gap::onRadioNotification().
Callchain of timeout event handlers.
- See also:
- Gap::onTimeout().
Member Enumeration Documentation
Advertising policy filter modes.
- See also:
- Bluetooth Core Specification 4.2 (Vol. 6), Part B, Section 4.3.2.
- Enumerator:
Address-type for BLEProtocol addresses.
Enumeration of disconnection reasons.
There might be a mismatch between the disconnection reason passed to disconnect() and the disconnection event generated locally because the disconnection reason passed to disconnect() is the disconnection reason to be transmitted to the peer.
- Enumerator:
CONNECTION_TIMEOUT The connection timed out.
shall not be used as a reason in disconnect().
REMOTE_USER_TERMINATED_CONNECTION Connection terminated by the user.
REMOTE_DEV_TERMINATION_DUE_TO_LOW_RESOURCES Remote device terminated connection due to low resources.
REMOTE_DEV_TERMINATION_DUE_TO_POWER_OFF Remote device terminated connection due to power off.
LOCAL_HOST_TERMINATED_CONNECTION Indicate that the local user or the internal Bluetooth subsystem terminated the connection.
shall not be used as a reason in disconnect().
CONN_INTERVAL_UNACCEPTABLE Connection parameters were unacceptable.
Connection initiation policy filter mode.
- See also:
- Bluetooth Core Specification 4.2 (vol. 6), Part B, Section 4.4.4.
enum Role_t |
Enumeration of GAP roles.
- Note:
- The BLE API does not express the broadcaster and scanner roles.
A device can fulfill different roles concurrently.
- Enumerator:
enum ScanningPolicyMode_t |
enum TimeoutSource_t |
Constructor & Destructor Documentation
Member Function Documentation
ble_error_t accumulateAdvertisingPayload | ( | uint8_t | flags ) |
Set gap flags in the advertising payload.
A call to this function is equivalent to:
Gap ⪆ GapAdvertisingData payload = gap.getAdvertisingPayload(); payload.addFlags(flags); gap.setAdvertisingPayload(payload);
- Parameters:
-
[in] flags The flags to be added.
- Returns:
- BLE_ERROR_NONE if the data was successfully added to the advertising payload.
ble_error_t accumulateAdvertisingPayload | ( | GapAdvertisingData::Appearance | app ) |
Set the appearance field in the advertising payload.
A call to this function is equivalent to:
Gap ⪆ GapAdvertisingData payload = gap.getAdvertisingPayload(); payload.addAppearance(app); gap.setAdvertisingPayload(payload);
- Parameters:
-
[in] app The appearance to advertise.
- Returns:
- BLE_ERROR_NONE if the data was successfully added to the advertising payload.
ble_error_t accumulateAdvertisingPayload | ( | GapAdvertisingData::DataType | type, |
const uint8_t * | data, | ||
uint8_t | len | ||
) |
Add a new field in the advertising payload.
A call to this function is equivalent to:
Gap ⪆ GapAdvertisingData payload = gap.getAdvertisingPayload(); payload.addData(type, data, len); gap.setAdvertisingPayload(payload);
- Parameters:
-
[in] type Identity of the field being added. [in] data Buffer containing the value of the field. [in] len Length of the data buffer.
- Returns:
- BLE_ERROR_NONE if the advertisement payload was updated based on matching AD type; otherwise, an appropriate error.
- Note:
- When the specified AD type is INCOMPLETE_LIST_16BIT_SERVICE_IDS, COMPLETE_LIST_16BIT_SERVICE_IDS, INCOMPLETE_LIST_32BIT_SERVICE_IDS, COMPLETE_LIST_32BIT_SERVICE_IDS, INCOMPLETE_LIST_128BIT_SERVICE_IDS, COMPLETE_LIST_128BIT_SERVICE_IDS or LIST_128BIT_SOLICITATION_IDS the supplied value is appended to the values previously added to the payload.
ble_error_t accumulateAdvertisingPayloadTxPower | ( | int8_t | power ) |
Set the Tx Power field in the advertising payload.
A call to this function is equivalent to:
Gap ⪆ GapAdvertisingData payload = gap.getAdvertisingPayload(); payload.addTxPower(power); gap.setAdvertisingPayload(payload);
- Parameters:
-
[in] power Transmit power in dBm used by the controller to advertise.
- Returns:
- BLE_ERROR_NONE if the data was successfully added to the advertising payload.
ble_error_t accumulateScanResponse | ( | GapAdvertisingData::DataType | type, |
const uint8_t * | data, | ||
uint8_t | len | ||
) |
void clearAdvertisingPayload | ( | void | ) |
void clearScanResponse | ( | void | ) |
Reset the content of the scan response.
- Note:
- This should be followed by a call to Gap::setAdvertisingPayload() or Gap::startAdvertising() before the update takes effect.
virtual ble_error_t connect | ( | const BLEProtocol::AddressBytes_t | peerAddr, |
BLEProtocol::AddressType_t | peerAddrType, | ||
const ConnectionParams_t * | connectionParams, | ||
const GapScanningParams * | scanParams | ||
) | [virtual] |
Initiate a connection to a peer.
Once the connection is established, a ConnectionCallbackParams_t event is emitted to handlers that have been registered with onConnection().
- Parameters:
-
[in] peerAddr MAC address of the peer. It must be in LSB format. [in] peerAddrType Address type of the peer. [in] connectionParams Connection parameters to use. [in] scanParams Scan parameters used to find the peer.
- Returns:
- BLE_ERROR_NONE if connection establishment procedure is started successfully. The connectionCallChain (if set) is invoked upon a connection event.
virtual ble_error_t disconnect | ( | Handle_t | connectionHandle, |
DisconnectionReason_t | reason | ||
) | [virtual] |
Initiate a disconnection procedure.
Once the disconnection procedure has completed a DisconnectionCallbackParams_t, the event is emitted to handlers that have been registered with onDisconnection().
- Parameters:
-
[in] reason Reason of the disconnection transmitted to the peer. [in] connectionHandle Handle of the connection to end.
- Returns:
- BLE_ERROR_NONE if the disconnection procedure successfully started.
virtual ble_error_t getAddress | ( | BLEProtocol::AddressType_t * | typeP, |
BLEProtocol::AddressBytes_t | address | ||
) | [virtual] |
GapAdvertisingParams& getAdvertisingParams | ( | void | ) |
const GapAdvertisingParams& getAdvertisingParams | ( | void | ) | const |
Const alternative to Gap::getAdvertisingParams().
- Returns:
- A const reference to the current advertising parameters.
const GapAdvertisingData& getAdvertisingPayload | ( | void | ) | const |
virtual AdvertisingPolicyMode_t getAdvertisingPolicyMode | ( | void | ) | const [virtual] |
virtual ble_error_t getAppearance | ( | GapAdvertisingData::Appearance * | appearanceP ) | [virtual] |
virtual ble_error_t getDeviceName | ( | uint8_t * | deviceName, |
unsigned * | lengthP | ||
) | [virtual] |
Get the value of the device name characteristic in the Generic Access Service.
To obtain the length of the deviceName value, this function is invoked with the deviceName
parameter set to NULL.
- Parameters:
-
[out] deviceName Pointer to an empty buffer where the UTF-8 non NULL-terminated string is placed. [in,out] lengthP Length of the deviceName
buffer. If the device name is successfully copied, then the length of the device name string (excluding the null terminator) replaces this value.
- Returns:
- BLE_ERROR_NONE if the device name was fetched correctly from the underlying BLE stack.
- Note:
- If the device name is longer than the size of the supplied buffer, length returns the complete device name length and not the number of bytes actually returned in deviceName. The application may use this information to retry with a suitable buffer size.
virtual InitiatorPolicyMode_t getInitiatorPolicyMode | ( | void | ) | const [virtual] |
virtual uint16_t getMaxAdvertisingInterval | ( | void | ) | const [virtual] |
virtual uint8_t getMaxWhitelistSize | ( | void | ) | const [virtual] |
virtual uint16_t getMinAdvertisingInterval | ( | void | ) | const [virtual] |
virtual uint16_t getMinNonConnectableAdvertisingInterval | ( | void | ) | const [virtual] |
virtual void getPermittedTxPowerValues | ( | const int8_t ** | valueArrayPP, |
size_t * | countP | ||
) | [virtual] |
virtual ble_error_t getPreferredConnectionParams | ( | ConnectionParams_t * | params ) | [virtual] |
virtual ScanningPolicyMode_t getScanningPolicyMode | ( | void | ) | const [virtual] |
GapState_t getState | ( | void | ) | const |
virtual ble_error_t getWhitelist | ( | Whitelist_t & | whitelist ) | const [virtual] |
Get the Link Layer to use the internal whitelist when scanning, advertising or initiating a connection depending on the filter policies.
- Parameters:
-
[in,out] whitelist Define the whitelist instance which is used to store the whitelist requested. In input, the caller provisions memory.
- Returns:
- BLE_ERROR_NONE if the implementation's whitelist was successfully copied into the supplied reference.
virtual ble_error_t initRadioNotification | ( | void | ) | [virtual] |
Enable radio-notification events.
Radio Notification is a feature that notifies the application when the radio is in use.
The ACTIVE signal is sent before the radio event starts. The nACTIVE signal is sent at the end of the radio event. The application programmer can use these signals to synchronize application logic with radio activity. For example, the ACTIVE signal can be used to shut off external devices, to manage peak current drawn during periods when the radio is on or to trigger sensor data collection for transmission in the Radio Event.
- Returns:
- BLE_ERROR_NONE on successful initialization, otherwise an error code.
MBED_DEPRECATED | ( | "Gap::DeprecatedAddressType_t is | deprecated, |
use BLEProtocol::AddressType_t instead" | |||
) | const |
MBED_DEPRECATED | ( | "Use disconnect(Handle_t, DisconnectionReason_t) instead." | ) |
static uint16_t MSEC_TO_GAP_DURATION_UNITS | ( | uint32_t | durationInMillis ) | [static] |
Convert milliseconds into 1.25ms units.
This function may be used to convert ms time of connection intervals into the format expected for connection parameters.
- Parameters:
-
[in] durationInMillis The duration in milliseconds.
- Returns:
- The duration in unit of 1.25ms.
void onConnection | ( | ConnectionEventCallback_t | callback ) |
Register a callback handling connection events.
- Parameters:
-
[in] callback Event handler being registered.
- Note:
- A callback may be unregistered using onConnection().detach(callback).
void onConnection | ( | T * | tptr, |
void(T::*)(const ConnectionCallbackParams_t *) | mptr | ||
) |
Register a callback handling connection events.
- Parameters:
-
[in] tptr Instance used to invoke mptr
.[in] mptr Event handler being registered.
- Note:
- A callback may be unregistered using onConnection().detach(callback).
ConnectionEventCallbackChain_t& onConnection | ( | ) |
Get the callchain of registered connection event handlers.
- Note:
- To register callbacks, use onConnection().add(callback).
- To unregister callbacks, use onConnection().detach(callback).
- Returns:
- A reference to the connection event callbacks chain.
void onDisconnection | ( | T * | tptr, |
void(T::*)(const DisconnectionCallbackParams_t *) | mptr | ||
) |
Register a callback handling disconnection events.
- Parameters:
-
[in] tptr Instance used to invoke mptr. [in] mptr Event handler being registered.
- Note:
- A callback may be unregistered using onDisconnection().detach(callback).
void onDisconnection | ( | DisconnectionEventCallback_t | callback ) |
Register a callback handling disconnection events.
- Parameters:
-
[in] callback Event handler being registered.
- Note:
- A callback may be unregistered using onDisconnection().detach(callback).
DisconnectionEventCallbackChain_t& onDisconnection | ( | ) |
Get the callchain of registered disconnection event handlers.
- Note:
- To register callbacks use onDisconnection().add(callback).
- To unregister callbacks use onDisconnection().detach(callback).
- Returns:
- A reference to the disconnection event callbacks chain.
void onRadioNotification | ( | void(*)(bool param) | callback ) |
Set the radio-notification events handler.
Radio Notification is a feature that enables ACTIVE and INACTIVE (nACTIVE) signals from the stack that notify the application when the radio is in use.
The ACTIVE signal is sent before the radio event starts. The nACTIVE signal is sent at the end of the radio event. The application programmer can use these signals to synchronize application logic with radio activity. For example, the ACTIVE signal can be used to shut off external devices, to manage peak current drawn during periods when the radio is on or to trigger sensor data collection for transmission in the Radio Event.
- Parameters:
-
[in] callback Application handler to be invoked in response to a radio ACTIVE/INACTIVE event.
void onRadioNotification | ( | T * | tptr, |
void(T::*)(bool) | mptr | ||
) |
void onShutdown | ( | const GapShutdownCallback_t & | callback ) |
Register a Gap shutdown event handler.
The handler is called when the Gap instance is about to shut down. It is usually issued after a call to BLE::shutdown().
- Parameters:
-
[in] callback Shutdown event handler to register.
- Note:
- To unregister a shutdown event handler, use onShutdown().detach(callback).
void onShutdown | ( | T * | objPtr, |
void(T::*)(const Gap *) | memberPtr | ||
) |
GapShutdownCallbackChain_t& onShutdown | ( | ) |
Access the callchain of shutdown event handler.
- Note:
- To register callbacks, use onShutdown().add(callback).
- To unregister callbacks, use onShutdown().detach(callback).
- Returns:
- A reference to the shutdown event callback chain.
void onTimeout | ( | TimeoutEventCallback_t | callback ) |
Register a callback handling timeout events.
- Parameters:
-
[in] callback Event handler being registered.
- Note:
- A callback may be unregistered using onTimeout().detach(callback).
- See also:
- TimeoutSource_t
TimeoutEventCallbackChain_t& onTimeout | ( | ) |
Get the callchain of registered timeout event handlers.
- Note:
- To register callbacks, use onTimeout().add(callback).
- To unregister callbacks, use onTimeout().detach(callback).
- Returns:
- A reference to the timeout event callbacks chain.
void processAdvertisementReport | ( | const BLEProtocol::AddressBytes_t | peerAddr, |
int8_t | rssi, | ||
bool | isScanResponse, | ||
GapAdvertisingParams::AdvertisingType_t | type, | ||
uint8_t | advertisingDataLen, | ||
const uint8_t * | advertisingData | ||
) |
Forward a received advertising packet to all registered event handlers listening for scanned packet events.
This function is meant to be called from the BLE stack specific implementation when a disconnection event occurs.
- Parameters:
-
[in] peerAddr Address of the peer that has emitted the packet. [in] rssi Value of the RSSI measured for the received packet. [in] isScanReponse If true, then the packet is a response to a scan request. [in] type Advertising type of the packet. [in] advertisingDataLen Length of the advertisement data received. [in] advertisingData Pointer to the advertisement packet's data.
void processConnectionEvent | ( | Handle_t | handle, |
Role_t | role, | ||
BLEProtocol::AddressType_t | peerAddrType, | ||
const BLEProtocol::AddressBytes_t | peerAddr, | ||
BLEProtocol::AddressType_t | ownAddrType, | ||
const BLEProtocol::AddressBytes_t | ownAddr, | ||
const ConnectionParams_t * | connectionParams | ||
) |
Notify all registered connection event handlers of a connection event.
This function is meant to be called from the BLE stack specific implementation when a connection event occurs.
- Parameters:
-
[in] handle Handle of the new connection. [in] role Role of this BLE device in the connection. [in] peerAddrType Address type of the connected peer. [in] peerAddr Address of the connected peer. [in] ownAddrType Address type this device uses for this connection. [in] ownAddr Address this device uses for this connection. [in] connectionParams Parameters of the connection.
void processDisconnectionEvent | ( | Handle_t | handle, |
DisconnectionReason_t | reason | ||
) |
Notify all registered disconnection event handlers of a disconnection event.
This function is meant to be called from the BLE stack specific implementation when a disconnection event occurs.
- Parameters:
-
[in] handle Handle of the terminated connection. [in] reason Reason of the disconnection.
void processTimeoutEvent | ( | TimeoutSource_t | source ) |
virtual ble_error_t reset | ( | void | ) | [virtual] |
Reset the Gap instance.
Reset process starts by notifying all registered shutdown event handlers that the Gap instance is about to be shut down. Then, it clears all Gap state of the associated object and then cleans the state present in the vendor implementation.
This function is meant to be overridden in the platform-specific subclass. Nevertheless, the subclass only resets its state and not the data held in Gap members. This is achieved by a call to Gap::reset() from the subclass' reset() implementation.
- Returns:
- BLE_ERROR_NONE on success.
- Note:
- Currently, a call to reset() does not reset the advertising and scan parameters to default values.
ble_error_t setActiveScanning | ( | bool | activeScanning ) |
Enable or disable active scanning.
- Parameters:
-
[in] activeScanning If set to true, then the scanner sends scan requests to a scannable or connectable advertiser. If set to false then the scanner does not send any request during the scan procedure.
- Returns:
- BLE_ERROR_NONE if active scanning was successfully set.
- Note:
- If scanning is already in progress, then active scanning is enabled for the underlying BLE stack.
virtual ble_error_t setAddress | ( | BLEProtocol::AddressType_t | type, |
const BLEProtocol::AddressBytes_t | address | ||
) | [virtual] |
Set the device MAC address and type.
The address set is used in subsequent GAP operations: scanning, advertising and connection initiation.
- Parameters:
-
[in] type Type of the address to set. [in] address Value of the address to set. It is ordered in little endian. This parameter is not considered if the address type is RANDOM_PRIVATE_RESOLVABLE or RANDOM_PRIVATE_NON_RESOLVABLE. For those types of address, the BLE API itself generates the address.
- Note:
- Some implementation may refuse to set a new PUBLIC address.
- Random static address set does not change.
- Returns:
- BLE_ERROR_NONE on success.
void setAdvertisingInterval | ( | uint16_t | interval ) |
Set the advertising interval.
- Parameters:
-
[in] interval Advertising interval in units of milliseconds. Advertising is disabled if interval is 0. If interval is smaller than the minimum supported value, then the minimum supported value is used instead. This minimum value can be discovered using getMinAdvertisingInterval().
This field must be set to 0 if connectionMode is equal to ADV_CONNECTABLE_DIRECTED.
- Note:
- Decreasing this value allows central devices to detect a peripheral faster, at the expense of the radio using more power due to the higher data transmit rate.
void setAdvertisingParams | ( | const GapAdvertisingParams & | newParams ) |
ble_error_t setAdvertisingPayload | ( | const GapAdvertisingData & | payload ) |
virtual ble_error_t setAdvertisingPolicyMode | ( | AdvertisingPolicyMode_t | mode ) | [virtual] |
void setAdvertisingTimeout | ( | uint16_t | timeout ) |
void setAdvertisingType | ( | GapAdvertisingParams::AdvertisingType_t | advType ) |
virtual ble_error_t setAppearance | ( | GapAdvertisingData::Appearance | appearance ) | [virtual] |
virtual ble_error_t setDeviceName | ( | const uint8_t * | deviceName ) | [virtual] |
virtual ble_error_t setInitiatorPolicyMode | ( | InitiatorPolicyMode_t | mode ) | [virtual] |
virtual ble_error_t setPreferredConnectionParams | ( | const ConnectionParams_t * | params ) | [virtual] |
Set the value of the preferred connection parameters exposed in the GATT Generic Access Service.
A connected peer may read the characteristic exposing these parameters and request an update of the connection parameters to accomodate the local device.
- Parameters:
-
[in] params Value of the preferred connection parameters.
- Returns:
- BLE_ERROR_NONE if the preferred connection params were set correctly.
ble_error_t setScanInterval | ( | uint16_t | interval ) |
Set the interval parameter used during scanning procedures.
- Parameters:
-
[in] interval Interval in ms between the start of two consecutive scan windows. That value is greater or equal to the scan window value. The maximum allowed value is 10.24ms.
- Returns:
- BLE_ERROR_NONE if the scan interval was correctly set.
virtual ble_error_t setScanningPolicyMode | ( | ScanningPolicyMode_t | mode ) | [virtual] |
ble_error_t setScanParams | ( | uint16_t | interval = GapScanningParams::SCAN_INTERVAL_MAX , |
uint16_t | window = GapScanningParams::SCAN_WINDOW_MAX , |
||
uint16_t | timeout = 0 , |
||
bool | activeScanning = false |
||
) |
Set the parameters used during a scan procedure.
- Parameters:
-
[in] interval in ms between the start of two consecutive scan windows. That value is greater or equal to the scan window value. The maximum allowed value is 10.24ms. [in] window Period in ms during which the scanner listens to advertising channels. That value is in the range 2.5ms to 10.24s. [in] timeout Duration in seconds of the scan procedure if any. The special value 0 disable specific duration of the scan procedure. [in] activeScanning If set to true, then the scanner sends scan requests to a scannable or connectable advertiser. If set to false, then the scanner does not send any request during the scan procedure.
- Returns:
- BLE_ERROR_NONE if the scan parameters were correctly set.
- Note:
- The scanning window divided by the interval determines the duty cycle for scanning. For example, if the interval is 100ms and the window is 10ms, then the controller scans for 10 percent of the time.
- If the interval and the window are set to the same value, then the device scans continuously during the scan procedure. The scanning frequency changes at every interval.
- Once the scanning parameters have been configured, scanning can be enabled by using startScan().
- The scan interval and window are recommendations to the BLE stack.
ble_error_t setScanTimeout | ( | uint16_t | timeout ) |
Set the timeout parameter used during scanning procedures.
- Parameters:
-
[in] timeout Duration in seconds of the scan procedure if any. The special value 0 disables specific duration of the scan procedure.
- Returns:
- BLE_ERROR_NONE if the scan timeout was correctly set.
- Note:
- If scanning is already active, the updated value of scanTimeout is propagated to the underlying BLE stack.
ble_error_t setScanWindow | ( | uint16_t | window ) |
Set the window parameter used during scanning procedures.
- Parameters:
-
[in] window Period in ms during which the scanner listens to advertising channels. That value is in the range 2.5ms to 10.24s.
- Returns:
- BLE_ERROR_NONE if the scan window was correctly set.
- Note:
- If scanning is already active, the updated value of scanWindow is propagated to the underlying BLE stack.
virtual ble_error_t setTxPower | ( | int8_t | txPower ) | [virtual] |
virtual ble_error_t setWhitelist | ( | const Whitelist_t & | whitelist ) | [virtual] |
Set the value of the whitelist to be used during GAP procedures.
- Parameters:
-
[in] whitelist A reference to a whitelist containing the addresses to be copied to the internal whitelist.
- Returns:
- BLE_ERROR_NONE if the implementation's whitelist was successfully populated with the addresses in the given whitelist.
- Note:
- The whitelist must not contain addresses of type BLEProtocol::AddressType_t::RANDOM_PRIVATE_NON_RESOLVABLE. This results in a BLE_ERROR_INVALID_PARAM because the remote peer might change its private address at any time, and it is not possible to resolve it.
- If the input whitelist is larger than getMaxWhitelistSize(), then BLE_ERROR_PARAM_OUT_OF_RANGE is returned.
ble_error_t startAdvertising | ( | void | ) |
virtual ble_error_t startRadioScan | ( | const GapScanningParams & | scanningParams ) | [protected, virtual] |
ble_error_t startScan | ( | void(*)(const AdvertisementCallbackParams_t *params) | callback ) |
Start the scanning procedure.
Packets received during the scan procedure are forwarded to the scan packet handler passed as argument to this function.
- Parameters:
-
[in] callback Advertisement packet event handler. Upon reception of an advertising packet, the packet is forwarded to callback
.
- Returns:
- BLE_ERROR_NONE if the device successfully started the scan procedure.
- Note:
- The parameters used by the procedure are defined by setScanParams().
ble_error_t startScan | ( | T * | object, |
void(T::*)(const AdvertisementCallbackParams_t *params) | callbackMember | ||
) |
Start the scanning procedure.
Packets received during the scan procedure are forwarded to the scan packet handler passed as argument to this function.
- Parameters:
-
[in] object Instance used to invoke callbackMember
.[in] callbackMember Advertisement packet event handler. Upon reception of an advertising packet, the packet is forwarded to callback
invoked fromobject
.
- Returns:
- BLE_ERROR_NONE if the device successfully started the scan procedure.
- Note:
- The parameters used by the procedure are defined by setScanParams().
virtual ble_error_t stopAdvertising | ( | void | ) | [virtual] |
virtual ble_error_t stopScan | ( | ) | [virtual] |
ble_error_t updateAdvertisingPayload | ( | GapAdvertisingData::DataType | type, |
const uint8_t * | data, | ||
uint8_t | len | ||
) |
Update a particular field in the advertising payload.
A call to this function is equivalent to:
Gap ⪆ GapAdvertisingData payload = gap.getAdvertisingPayload(); payload.updateData(type, data, len); gap.setAdvertisingPayload(payload);
- Parameters:
-
[in] type Id of the field to update. [in] data data buffer containing the new value of the field. [in] len Length of the data buffer.
- Note:
- If advertisements are enabled, then the update takes effect immediately.
- Returns:
- BLE_ERROR_NONE if the advertisement payload was updated based on matching AD type; otherwise, an appropriate error.
virtual ble_error_t updateConnectionParams | ( | Handle_t | handle, |
const ConnectionParams_t * | params | ||
) | [virtual] |
Update connection parameters of an existing connection.
In the central role, this initiates a Link Layer connection parameter update procedure. In the peripheral role, this sends the corresponding L2CAP request and waits for the central to perform the procedure.
- Parameters:
-
[in] handle Connection Handle. [in] params Pointer to desired connection parameters.
- Returns:
- BLE_ERROR_NONE if the connection parameters were updated correctly.
Field Documentation
GapAdvertisingParams _advParams [protected] |
GapAdvertisingData _advPayload [protected] |
GapScanningParams _scanningParams [protected] |
GapAdvertisingData _scanResponse [protected] |
const unsigned ADDR_LEN = BLEProtocol::ADDR_LEN [static] |
ConnectionEventCallbackChain_t connectionCallChain [protected] |
uint8_t connectionCount [protected] |
AdvertisementReportCallback_t onAdvertisementReport [protected] |
bool scanningActive [protected] |
GapState_t state [protected] |
TimeoutEventCallbackChain_t timeoutCallbackChain [protected] |
const uint16_t UNIT_1_25_MS = 1250 [static] |
Generated on Sun Jul 17 2022 08:25:40 by
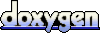