
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
GattClient.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_GATT_CLIENT_H__ 00018 #define MBED_GATT_CLIENT_H__ 00019 00020 #include "Gap.h" 00021 #include "GattAttribute.h" 00022 #include "ServiceDiscovery.h" 00023 #include "CharacteristicDescriptorDiscovery.h" 00024 00025 #include "GattCallbackParamTypes.h" 00026 00027 #include "CallChainOfFunctionPointersWithContext.h" 00028 00029 /** 00030 * @addtogroup ble 00031 * @{ 00032 * @addtogroup gatt 00033 * @{ 00034 * @addtogroup client 00035 * @{ 00036 */ 00037 00038 /** 00039 * Define procedures required for interacting with a distant GATT server. 00040 * 00041 * @par Discovery procedures 00042 * 00043 * A GATT server hosts a fixed set of services. These services are a logical 00044 * composition of characteristics that may be discovered, read, written or also 00045 * broadcast their state to a connected client. These characteristics may also 00046 * contain metainformation named characteristic descriptors. A characteristic 00047 * descriptor may be used to indicate the unit used for a characteristic value, 00048 * describe in a textual form the characterisic purpose or allow a client to 00049 * register for notification of updates of the characteristic value. 00050 * 00051 * Prior to any interaction with server characteristic, a GATT client 00052 * discovers the layout of the services and characteristics present on the 00053 * server. 00054 * 00055 * The layout of the descriptors of a characteristic may also be issued to 00056 * as an extra discovery step. 00057 * 00058 * @par Attribute manipulation 00059 * 00060 * As a result of the discovery process, the client can start interacting with 00061 * the characteristic discovered. Depending on the characteristic properties 00062 * (acquired during discovery), a client can read or write the value of a given 00063 * characteristic. 00064 * 00065 * Mbed BLE abstracts most read and write operations to offer a single API that 00066 * can be used to read or write characteristics values. Application code does not 00067 * have to handle the fragmentation/reassembly process necessary if the attribute 00068 * value to transported cannot fit in a single data packet. 00069 * 00070 * @par Server Initiated events 00071 * 00072 * If a characteristic has to notify or indicate a property set; then, a client may 00073 * register to a notification or indication from the characteristic. When the 00074 * server updates the characteristic value, the server can forward the 00075 * new value to the registered clients. The notification/indication mechanism 00076 * prevents polling from the client and therefore minimize the transactions 00077 * involved between a client and a server. 00078 * 00079 * Registration is made by writing the Client Characteristic Configuration 00080 * Descriptor, which is present in the characteristic if the notify or 00081 * indicate properties are set. The client discovers that descriptor 00082 * if it intends to register to server initiated events. 00083 */ 00084 class GattClient { 00085 public: 00086 /** 00087 * Attribute read event handler. 00088 * 00089 * @see GattClient::onDataRead(). 00090 */ 00091 typedef FunctionPointerWithContext<const GattReadCallbackParams*> 00092 ReadCallback_t; 00093 00094 /** 00095 * Callchain of attribute read event handlers. 00096 */ 00097 typedef CallChainOfFunctionPointersWithContext<const GattReadCallbackParams*> 00098 ReadCallbackChain_t; 00099 00100 /** 00101 * GATT write operations. 00102 */ 00103 enum WriteOp_t { 00104 /** 00105 * Write request. 00106 * 00107 * It is used to request the server to write the value of an attribute 00108 * and acknowledge that this has been achieved in a Write Response. 00109 */ 00110 GATT_OP_WRITE_REQ = 0x01, 00111 00112 /** 00113 * Write command. 00114 * 00115 * It is used to request the server to write the value of an attribute. 00116 * The server does not acknowledge the status of the operation. 00117 */ 00118 GATT_OP_WRITE_CMD = 0x02, 00119 }; 00120 00121 /** 00122 * Attribute write event handler. 00123 * 00124 * @see GattClient::onDataWrite(). 00125 */ 00126 typedef FunctionPointerWithContext<const GattWriteCallbackParams*> 00127 WriteCallback_t; 00128 00129 /** 00130 * Callchain of attribute write event handlers. 00131 * 00132 * @see GattClient::onDataWrite(). 00133 */ 00134 typedef CallChainOfFunctionPointersWithContext<const GattWriteCallbackParams*> 00135 WriteCallbackChain_t; 00136 00137 /** 00138 * Handle value notification/indication event handler. 00139 * 00140 * @see to GattClient::onHVX(). 00141 */ 00142 typedef FunctionPointerWithContext<const GattHVXCallbackParams*> 00143 HVXCallback_t; 00144 00145 /** 00146 * Callchain of handle value notification/indication event handlers. 00147 * 00148 * @see GattClient::onHVX(). 00149 */ 00150 typedef CallChainOfFunctionPointersWithContext<const GattHVXCallbackParams*> 00151 HVXCallbackChain_t; 00152 00153 /** 00154 * Shutdown event handler. 00155 * 00156 * @see GattClient::onShutdown(). 00157 */ 00158 typedef FunctionPointerWithContext<const GattClient *> 00159 GattClientShutdownCallback_t; 00160 00161 00162 /** 00163 * Callchain of shutdown event handlers. 00164 * 00165 * @see to GattClient::onShutown(). 00166 */ 00167 typedef CallChainOfFunctionPointersWithContext<const GattClient *> 00168 GattClientShutdownCallbackChain_t; 00169 00170 /* 00171 * The following functions are meant to be overridden in the platform 00172 * specific subclass. 00173 */ 00174 public: 00175 00176 virtual ~GattClient() { } 00177 00178 /** 00179 * Launch the service and characteristic discovery procedure of a GATT server 00180 * peer. 00181 * 00182 * The procedure invokes application callbacks for matching services or 00183 * characteristics. The process ends after all the services and 00184 * characteristics present on the distant GATT server have been discovered. 00185 * Termination callbacks registered with onServiceDiscoveryTermination() are 00186 * invoked to notify the application of the termination of the procedure. 00187 * 00188 * Application code can track the status of the procedure by invoking the 00189 * function isServiceDiscoveryActive(), which returns true if the 00190 * procedure is ongoing. 00191 * 00192 * At any point, application code can prematurely terminate the discovery 00193 * procedure by calling terminateServiceDiscovery(). 00194 * 00195 * @param[in] connectionHandle Handle of the connection with the peer GATT 00196 * server. 00197 * @param[in] sc Service discovered event handler invoked when a matching 00198 * service has been discovered. This parameter may be NULL. 00199 * @param[in] cc Characteristic discovered event handler invoked when a 00200 * matching characteristic has been found. This parameter may be NULL. 00201 * @param[in] matchingServiceUUID UUID of the service the caller is 00202 * interested in. If a service discovered matches this filter, then @p sc is 00203 * invoked with it. The special value BLE_UUID_UNKNOWN acts as a wildcard, 00204 * which can be used to discover all services present on the peer GATT 00205 * server. 00206 * @param[in] matchingCharacteristicUUIDIn UUID of the characteristic the 00207 * caller is interested in. If a characteristic discovered matches this 00208 * filter, then @p cc is invoked with it. The special value BLE_UUID_UNKNOWN 00209 * acts as a wildcard, which can be used to discover all services present on 00210 * the peer GATT server. 00211 * 00212 * @par Discovery procedure implementation detail 00213 * 00214 * It is recommended to implement several strategies based on the 00215 * combination of callbacks and filters passed in input to efficiently 00216 * realize the discovery procedure: 00217 * - If @p sc and @p cc are NULL, then it is not necessay to initiate any 00218 * discovery, and the termination handlers can be invoked immediately. 00219 * - If @p matchingServiceUUID is set, then the GATT discover services by 00220 * service UUID procedure should be used; otherwise, the GATT discover primary 00221 * services procedure should be used. 00222 * - If @p cc is NULL, then the discovery process should end after the discovery 00223 * of the services. 00224 * 00225 * @return BLE_ERROR_NONE if the discovery procedure has been successfully 00226 * started and an appropriate error otherwise. 00227 */ 00228 virtual ble_error_t launchServiceDiscovery( 00229 Gap::Handle_t connectionHandle, 00230 ServiceDiscovery::ServiceCallback_t sc = NULL, 00231 ServiceDiscovery::CharacteristicCallback_t cc = NULL, 00232 const UUID &matchingServiceUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN), 00233 const UUID &matchingCharacteristicUUIDIn = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN) 00234 ) { 00235 /* Avoid compiler warnings about unused variables. */ 00236 (void)connectionHandle; 00237 (void)sc; 00238 (void)cc; 00239 (void)matchingServiceUUID; 00240 (void)matchingCharacteristicUUIDIn; 00241 00242 /* Requesting action from porters: override this API if this capability 00243 is supported. */ 00244 return BLE_ERROR_NOT_IMPLEMENTED; 00245 } 00246 00247 /** 00248 * Launch the service discovery procedure of a GATT server peer. 00249 * 00250 * The procedure invokes the application callback for matching services. 00251 * The process ends after all the services present on the distant GATT 00252 * server have been discovered. 00253 * Termination callbacks registered with onServiceDiscoveryTermination() are 00254 * invoked to notify the application of the termination of the procedure. 00255 * 00256 * Application code can track the status of the procedure by invoking the 00257 * function isServiceDiscoveryActive(), which returns true if the 00258 * procedure is ongoing. 00259 * 00260 * At any point, application code can prematurely terminate the discovery 00261 * procedure by calling terminateServiceDiscovery(). 00262 * 00263 * @param[in] connectionHandle Handle of the connection with the peer GATT 00264 * server. 00265 * @param[in] callback Service discovered event handler invoked when a 00266 * matching service has been discovered. This parameter may be NULL. 00267 * @param[in] matchingServiceUUID UUID of the service the caller is 00268 * interested in. If a service discovered matches this filter, then @p sc is 00269 * invoked with it. The special value BLE_UUID_UNKNOWN act is a wildcard, 00270 * which can be used to discover all services present on the peer GATT 00271 * server. 00272 * 00273 * @return BLE_ERROR_NONE if the discovery procedure has been successfully 00274 * started and an appropriate error otherwise. 00275 */ 00276 virtual ble_error_t discoverServices( 00277 Gap::Handle_t connectionHandle, 00278 ServiceDiscovery::ServiceCallback_t callback, 00279 const UUID &matchingServiceUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN) 00280 ) { 00281 /* We take advantage of the property 00282 * that providing NULL for the characteristic callback results in 00283 * characteristic discovery being skipped for each matching 00284 * service. This allows for an inexpensive method to discover only 00285 * services. Porters are free to override this. */ 00286 return launchServiceDiscovery( 00287 connectionHandle, callback, NULL, matchingServiceUUID 00288 ); 00289 } 00290 00291 /** 00292 * Launch the service discovery procedure of a GATT server peer. 00293 * 00294 * The process ends after all the services present in the attribute range @p 00295 * startHandle to @p endHandle have been discovered. 00296 * 00297 * Termination callbacks registered with onServiceDiscoveryTermination() are 00298 * invoked to notify the application of the termination of the procedure. 00299 * 00300 * Application code can track the status of the procedure by invoking the 00301 * function isServiceDiscoveryActive(), which returns true if the 00302 * procedure is ongoing. 00303 * 00304 * At any point, application code can prematurely terminate the discovery 00305 * procedure by calling terminateServiceDiscovery(). 00306 * 00307 * @param[in] connectionHandle Handle of the connection with the peer GATT 00308 * server. 00309 * @param[in] callback Service discovered event handler invoked when a 00310 * matching service has been discovered. This parameter may be NULL. 00311 * @param[in] startHandle First attribute handle of the discovery range. 00312 * @param[in] endHandle end Lasr attribute handle of the discovery range. 00313 * 00314 * @return BLE_ERROR_NONE if the discovery procedure has been successfully 00315 * started and an appropriate error otherwise. 00316 */ 00317 virtual ble_error_t discoverServices( 00318 Gap::Handle_t connectionHandle, 00319 ServiceDiscovery::ServiceCallback_t callback, 00320 GattAttribute::Handle_t startHandle, 00321 GattAttribute::Handle_t endHandle 00322 ) { 00323 /* Avoid compiler warnings about unused variables. */ 00324 (void)connectionHandle; 00325 (void)callback; 00326 (void)startHandle; 00327 (void)endHandle; 00328 00329 /* Requesting action from porters: override this API if this capability 00330 is supported. */ 00331 return BLE_ERROR_NOT_IMPLEMENTED; 00332 } 00333 00334 /** 00335 * Check if the service discovery procedure is currently active. 00336 * 00337 * @return true if service discovery procedure is active and false otherwise. 00338 */ 00339 virtual bool isServiceDiscoveryActive(void) const 00340 { 00341 /* Requesting action from porters: override this API if this capability 00342 is supported. */ 00343 return false; 00344 } 00345 00346 /** 00347 * Terminate all ongoing service discovery procedures. 00348 * 00349 * It results in an invocation of the service discovery termination handler 00350 * registered with onServiceDiscoveryTermination(). 00351 */ 00352 virtual void terminateServiceDiscovery(void) 00353 { 00354 /* Requesting action from porters: override this API if this capability 00355 is supported. */ 00356 } 00357 00358 /** 00359 * Initiate the read procedure of an attribute handle. 00360 * 00361 * Once the attribute value has been read in its entirety, the process issues 00362 * an attribute read event and passes it to all events handlers registered 00363 * by onDataRead. 00364 * 00365 * @param[in] connHandle Handle of the connection used to send the read 00366 * request. 00367 * @param[in] attributeHandle Handle of the attribute to read data from. 00368 * @param[in] offset The offset from the start of the attribute value to be 00369 * read. 00370 * 00371 * @return BLE_ERROR_NONE if read procedure successfully started. 00372 * 00373 * @par Implementation notes: 00374 * 00375 * Reading the attribute value in its entirety may involve sending several 00376 * GATT requests to the peer. The following algorithm may be used to 00377 * implement the process: 00378 * 00379 * If the offset is equal to 0, then send a read request; otherwise, send a 00380 * read blob request at the specified offset. 00381 * 00382 * While the attribute data in the response are MTU - 1 long: 00383 * - Concat the response to the value containing the previous responses. 00384 * - Increment the value of the offset by MTU - 1. 00385 * - Send a read blob request with the updated offset. 00386 * 00387 * Finally, concat the last response with the value containing all the 00388 * previous responses and forward that value to the event handlers. 00389 */ 00390 virtual ble_error_t read( 00391 Gap::Handle_t connHandle, 00392 GattAttribute::Handle_t attributeHandle, 00393 uint16_t offset 00394 ) const { 00395 /* Avoid compiler warnings about unused variables. */ 00396 (void)connHandle; 00397 (void)attributeHandle; 00398 (void)offset; 00399 00400 /* Requesting action from porters: override this API if this capability 00401 is supported. */ 00402 return BLE_ERROR_NOT_IMPLEMENTED; 00403 } 00404 00405 /** 00406 * Initiate a write procedure on an attribute value. 00407 * 00408 * If @p cmd is equal to GATT_OP_WRITE_REQ, then the status of the operation 00409 * is reported to the event handlers registered through onDataWritten(). 00410 * 00411 * @param[in] cmd Type of the write procedure used. If GATT_OP_WRITE_CMD 00412 * is set, then value length is not greater than the size of the mtu 00413 * of connHandle minus three. 00414 * @param[in] connHandle Handle of the connection used to send the write 00415 * request or command. 00416 * @param[in] attributeHandle Handle of the attribute value to write. 00417 * @param[in] length Number of bytes present in @p value. 00418 * @param[in] value Data buffer to write to attributeHandle. 00419 * 00420 * @return BLE_ERROR_NONE if the write procedure successfully started. 00421 * 00422 * @par Implementation notes: 00423 * 00424 * If the operation is a write command, then an implementation uses the 00425 * GATT write without response procedure and an error is returned if 00426 * the data buffer to write is larger than the size of the MTU - 3. 00427 * 00428 * If the operation is a write command and the size of the data buffer to 00429 * write is less than than the size of the MTU - 3, then the ATT write request 00430 * procedure is used, and the response is reported to the handlers 00431 * listening for write response. 00432 * 00433 * Otherwise, the data buffer to write is divided in chunks with a 00434 * maximum size of MTU - 5. Those chunks are sent sequentially to the 00435 * peer in ATT prepare write requests. If an error response is received 00436 * during the process, the procedure ends immediately, the prepared 00437 * write is discarded and an error is reported to the application handlers. 00438 * Once all the chunks have been sent, the transaction is completed 00439 * by sending an execute write request to the peer. The peer response is 00440 * forwarded to the application handlers. 00441 */ 00442 virtual ble_error_t write( 00443 GattClient::WriteOp_t cmd, 00444 Gap::Handle_t connHandle, 00445 GattAttribute::Handle_t attributeHandle, 00446 size_t length, 00447 const uint8_t *value 00448 ) const { 00449 /* Avoid compiler warnings about unused variables. */ 00450 (void)cmd; 00451 (void)connHandle; 00452 (void)attributeHandle; 00453 (void)length; 00454 (void)value; 00455 00456 /* Requesting action from porters: override this API if this capability 00457 is supported. */ 00458 return BLE_ERROR_NOT_IMPLEMENTED; 00459 } 00460 00461 /* Event callback handlers. */ 00462 public: 00463 00464 /** 00465 * Register an attribute read event handler. 00466 * 00467 * @note It is possible to unregister a callback using 00468 * onDataRead().detach(callbackToRemove). 00469 * 00470 * @param[in] callback Event handler being registered. 00471 */ 00472 void onDataRead(ReadCallback_t callback) 00473 { 00474 onDataReadCallbackChain.add(callback); 00475 } 00476 00477 /** 00478 * Get the callchain of attribute read event handlers. 00479 * 00480 * @return A reference to the read event callback chain. 00481 * 00482 * @note It is possible to register new handlers using 00483 * onDataRead().add(callback). 00484 * 00485 * @note It is possible to unregister an handler by using 00486 * onDataRead().detach(callback). 00487 */ 00488 ReadCallbackChain_t & onDataRead() 00489 { 00490 return onDataReadCallbackChain; 00491 } 00492 00493 /** 00494 * Register an attribute write event handler. 00495 * 00496 * @param[in] callback Event handler being registered. 00497 * 00498 * @note It is possible to remove registered handlers using 00499 * onDataWritten().detach(callbackToRemove). 00500 * 00501 * @note Write commands (issued using writeWoResponse) don't generate a 00502 * response. 00503 */ 00504 void onDataWritten(WriteCallback_t callback) 00505 { 00506 onDataWriteCallbackChain.add(callback); 00507 } 00508 00509 /** 00510 * Get the callchain of attribute write event handlers. 00511 * 00512 * @return A reference to the data written callbacks chain. 00513 * 00514 * @note It is possible to register new handlers by using 00515 * onDataWritten().add(callback). 00516 * 00517 * @note It is possible to unregister an handler by using 00518 * onDataWritten().detach(callback). 00519 */ 00520 WriteCallbackChain_t & onDataWritten() 00521 { 00522 return onDataWriteCallbackChain; 00523 } 00524 00525 /** 00526 * Register an attribute write event handler. 00527 * 00528 * @param[in] callback Event handler being registered. 00529 * 00530 * @note It is possible to remove registered handlers using 00531 * onDataWritten().detach(callbackToRemove). 00532 * 00533 * @note Write commands (issued using writeWoResponse) don't generate a 00534 * response. 00535 * 00536 * @deprecated Use GattServer::onDataWritten(). 00537 */ 00538 MBED_DEPRECATED("Use GattServer::onDataWritten()") 00539 void onDataWrite(WriteCallback_t callback) 00540 { 00541 onDataWritten(callback); 00542 } 00543 00544 /** 00545 * Register a service discovery termination event handler. 00546 * 00547 * @param[in] callback Event handler being registered. 00548 */ 00549 virtual void onServiceDiscoveryTermination( 00550 ServiceDiscovery::TerminationCallback_t callback 00551 ) { 00552 (void)callback; /* Avoid compiler warnings about ununsed variables. */ 00553 00554 /* Requesting action from porters: override this API if this capability 00555 is supported. */ 00556 } 00557 00558 /** 00559 * Initiate the descriptor discovery procedure for a given characteristic. 00560 * 00561 * When a descriptor is discovered the discovered descriptor is forwarded 00562 * to @p discoveryCallback. After the discovery of all the descriptors, the 00563 * procedure ends and send a descriptor discovery termination event to @p 00564 * termination callback. 00565 * 00566 * Application code may monitor the discovery process by querying its status 00567 * with isCharacteristicDescriptorDiscoveryActive(). It can also end the 00568 * discovery process by calling terminateCharacteristicDescriptorDiscovery(). 00569 * 00570 * @param[in] characteristic The characteristic owning the descriptors to 00571 * discover. 00572 * @param[in] discoveryCallback Handle descriptor discovered events for the 00573 * duration of the procedure. 00574 * @param[in] terminationCallback Handle descriptor discovery termination 00575 * event of the procedure. 00576 * 00577 * @return BLE_ERROR_NONE if the characteristic descriptor discovery 00578 * procedure has been launched successfully otherwise an appropriate error. 00579 */ 00580 virtual ble_error_t discoverCharacteristicDescriptors( 00581 const DiscoveredCharacteristic& characteristic, 00582 const CharacteristicDescriptorDiscovery::DiscoveryCallback_t & discoveryCallback, 00583 const CharacteristicDescriptorDiscovery::TerminationCallback_t & terminationCallback 00584 ) { 00585 (void) characteristic; 00586 (void) discoveryCallback; 00587 (void) terminationCallback; 00588 /* Requesting action from porter(s): override this API if this 00589 capability is supported. */ 00590 return BLE_ERROR_NOT_IMPLEMENTED; 00591 } 00592 00593 /** 00594 * Query status of the descriptor discovery procedure for a given 00595 * characteristic. 00596 * 00597 * @param[in] characteristic The characteristic concerned by the descriptors 00598 * discovery. 00599 * 00600 * @return true if a descriptors discovery is active for the characteristic 00601 * in input otherwise false. 00602 */ 00603 virtual bool isCharacteristicDescriptorDiscoveryActive( 00604 const DiscoveredCharacteristic& characteristic 00605 ) const { 00606 (void) characteristic; 00607 /* Requesting action from porter(s): override this API if this 00608 capability is supported. */ 00609 return false; 00610 } 00611 00612 /** 00613 * @brief Terminate an ongoing characteristic descriptor discovery procedure. 00614 * 00615 * If the procedure is active, then it ends, and the termination handler 00616 * associated with the procedure is called. 00617 * 00618 * @param[in] characteristic The characteristic containing the descriptors 00619 * being discovered. 00620 */ 00621 virtual void terminateCharacteristicDescriptorDiscovery( 00622 const DiscoveredCharacteristic& characteristic 00623 ) { 00624 /* Requesting action from porter(s): override this API if this 00625 capability is supported. */ 00626 (void) characteristic; 00627 } 00628 00629 /** 00630 * Register an handler for Handle Value Notification/Indication events. 00631 * 00632 * @param callback Event handler to register. 00633 * 00634 * @note It is possible to unregister a callback by using 00635 * onHVX().detach(callbackToRemove). 00636 */ 00637 void onHVX(HVXCallback_t callback) 00638 { 00639 onHVXCallbackChain.add(callback); 00640 } 00641 00642 /** 00643 * Register a shutdown event handler. 00644 * 00645 * The registered handler is invoked when the GattClient instance is 00646 * about to be shut down. 00647 * 00648 * @param[in] callback Event handler to invoke when a shutdown event is 00649 * available. 00650 * 00651 * @note onShutdown().detach(callback) may be used to unregister a given 00652 * callback. 00653 * 00654 * @see BLE::shutdown() 00655 */ 00656 void onShutdown(const GattClientShutdownCallback_t & callback) 00657 { 00658 shutdownCallChain.add(callback); 00659 } 00660 00661 /** 00662 * Register a shutdown event handler. 00663 * 00664 * The registered handler is invoked when the GattClient instance is 00665 * about to be shut down. 00666 * 00667 * @param[in] objPtr Instance that will be used to invoke @p memberPtr. 00668 * @param[in] memberPtr Event handler to invoke when a shutdown event is 00669 * available. 00670 */ 00671 template <typename T> 00672 void onShutdown(T *objPtr, void (T::*memberPtr)(const GattClient *)) 00673 { 00674 shutdownCallChain.add(objPtr, memberPtr); 00675 } 00676 00677 /** 00678 * Get the callchain of shutdown event handlers. 00679 * 00680 * @return A reference to the shutdown event callbacks chain. 00681 * 00682 * @note onShutdown().add(callback) may be used to register new handlers. 00683 * 00684 * @note onShutdown().detach(callback) may be used to unregister an handler. 00685 */ 00686 GattClientShutdownCallbackChain_t & onShutdown() 00687 { 00688 return shutdownCallChain; 00689 } 00690 00691 /** 00692 * @brief provide access to the callchain of HVX callbacks. 00693 * 00694 * @return A reference to the HVX callbacks chain. 00695 * 00696 * @note It is possible to register callbacks using onHVX().add(callback). 00697 * 00698 * @note It is possible to unregister callbacks using onHVX().detach(callback). 00699 */ 00700 HVXCallbackChain_t & onHVX() { 00701 return onHVXCallbackChain; 00702 } 00703 00704 public: 00705 /** 00706 * Reset the state of the GattClient instance. 00707 * 00708 * Prior to any state modification, shutdown event handlers are notified 00709 * that the GattClient instance is about to be shut down. Then, running 00710 * procedures end. Finally, the state of the instance is reset. 00711 * 00712 * @par implementation note 00713 * 00714 * This function is meant to be overridden in the platform-specific 00715 * subclass. Nevertheless, the subclass only resets its 00716 * state and not the data held in GattClient members. This is achieved 00717 * by a call to GattClient::reset() from the subclass' reset() 00718 * implementation. 00719 * 00720 * @return BLE_ERROR_NONE on success. 00721 */ 00722 virtual ble_error_t reset(void) 00723 { 00724 /* Notify that the instance is about to shut down. */ 00725 shutdownCallChain.call(this); 00726 shutdownCallChain.clear(); 00727 00728 onDataReadCallbackChain.clear(); 00729 onDataWriteCallbackChain.clear(); 00730 onHVXCallbackChain.clear(); 00731 00732 return BLE_ERROR_NONE; 00733 } 00734 00735 protected: 00736 GattClient() 00737 { 00738 /* Empty */ 00739 } 00740 00741 /* Entry points for the underlying stack to report events back to the user. */ 00742 public: 00743 /** 00744 * Forward an attribute read event to all registered handlers. 00745 * 00746 * @important This function is meant to be called from the vendor 00747 * implementation when an attribute read event occurs. 00748 * 00749 * @param[in] params Attribute read event to pass to the registered handlers. 00750 */ 00751 void processReadResponse(const GattReadCallbackParams *params) 00752 { 00753 onDataReadCallbackChain(params); 00754 } 00755 00756 /** 00757 * Forward an attribute written event to all registered handlers. 00758 * 00759 * @important This function is meant to be called from the vendor 00760 * implementation when an attribute written event occurs. 00761 * 00762 * @param[in] params Attribute written event to pass to the registered 00763 * handlers. 00764 */ 00765 void processWriteResponse(const GattWriteCallbackParams *params) 00766 { 00767 onDataWriteCallbackChain(params); 00768 } 00769 00770 /** 00771 * Forward a handle value notification or indication event to all registered 00772 * handlers. 00773 * 00774 * @important This function is meant to be called from the vendor 00775 * implementation when a notification or indication event is available. 00776 * 00777 * @param[in] params Notification or Indication event to pass to the 00778 * registered handlers. 00779 */ 00780 void processHVXEvent(const GattHVXCallbackParams *params) 00781 { 00782 if (onHVXCallbackChain) { 00783 onHVXCallbackChain(params); 00784 } 00785 } 00786 00787 protected: 00788 /** 00789 * Callchain containing all registered event handlers for data read 00790 * events. 00791 */ 00792 ReadCallbackChain_t onDataReadCallbackChain; 00793 00794 /** 00795 * Callchain containing all registered event handlers for data write 00796 * events. 00797 */ 00798 WriteCallbackChain_t onDataWriteCallbackChain; 00799 00800 /** 00801 * Callchain containing all registered event handlers for update 00802 * events. 00803 */ 00804 HVXCallbackChain_t onHVXCallbackChain; 00805 00806 /** 00807 * Callchain containing all registered event handlers for shutdown 00808 * events. 00809 */ 00810 GattClientShutdownCallbackChain_t shutdownCallChain; 00811 00812 private: 00813 /* Disallow copy and assignment. */ 00814 GattClient(const GattClient &); 00815 GattClient& operator=(const GattClient &); 00816 }; 00817 00818 /** 00819 * @} 00820 * @} 00821 * @} 00822 */ 00823 00824 #endif /* ifndef MBED_GATT_CLIENT_H__ */
Generated on Sun Jul 17 2022 08:25:23 by
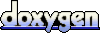