
Rtos API example
Common
[Ble]
Data Structures | |
class | CallChainOfFunctionPointersWithContext< ContextType > |
Function like object hosting a list of FunctionPointerWithContext. More... | |
class | FunctionPointerWithContext< ContextType > |
Function like object adapter over freestanding and member functions. More... | |
class | SafeBool< T > |
Safe conversion of objects in boolean context. More... | |
class | UUID |
Representation of a Universally Unique Identifier (UUID). More... | |
Namespaces | |
namespace | ble |
Entry namespace for all BLE API definitions. | |
namespace | BLEProtocol |
Common namespace for types and constants used everywhere in BLE API. | |
namespace | SafeBool_ |
Private namespace used to host details of the SafeBool implementation. | |
Files | |
file | ArrayView.h |
Enumerations | |
enum | { BLE_UUID_UNKNOWN = 0x0000, BLE_UUID_SERVICE_PRIMARY = 0x2800, BLE_UUID_SERVICE_SECONDARY = 0x2801, BLE_UUID_SERVICE_INCLUDE = 0x2802, BLE_UUID_CHARACTERISTIC = 0x2803, BLE_UUID_DESCRIPTOR_CHAR_EXT_PROP = 0x2900, BLE_UUID_DESCRIPTOR_CHAR_USER_DESC = 0x2901, BLE_UUID_DESCRIPTOR_CLIENT_CHAR_CONFIG = 0x2902, BLE_UUID_DESCRIPTOR_SERVER_CHAR_CONFIG = 0x2903, BLE_UUID_DESCRIPTOR_CHAR_PRESENTATION_FORMAT = 0x2904, BLE_UUID_DESCRIPTOR_CHAR_AGGREGATE_FORMAT = 0x2905, BLE_UUID_GATT = 0x1801, BLE_UUID_GATT_CHARACTERISTIC_SERVICE_CHANGED = 0x2A05, BLE_UUID_GAP = 0x1800, BLE_UUID_GAP_CHARACTERISTIC_DEVICE_NAME = 0x2A00, BLE_UUID_GAP_CHARACTERISTIC_APPEARANCE = 0x2A01, BLE_UUID_GAP_CHARACTERISTIC_PPF = 0x2A02, BLE_UUID_GAP_CHARACTERISTIC_RECONN_ADDR = 0x2A03, BLE_UUID_GAP_CHARACTERISTIC_PPCP = 0x2A04 } |
Assigned values for BLE UUIDs. More... | |
enum | ble_error_t { BLE_ERROR_NONE = 0, BLE_ERROR_BUFFER_OVERFLOW = 1, BLE_ERROR_NOT_IMPLEMENTED = 2, BLE_ERROR_PARAM_OUT_OF_RANGE = 3, BLE_ERROR_INVALID_PARAM = 4, BLE_STACK_BUSY = 5, BLE_ERROR_INVALID_STATE = 6, BLE_ERROR_NO_MEM = 7, BLE_ERROR_OPERATION_NOT_PERMITTED = 8, BLE_ERROR_INITIALIZATION_INCOMPLETE = 9, BLE_ERROR_ALREADY_INITIALIZED = 10, BLE_ERROR_UNSPECIFIED = 11, BLE_ERROR_INTERNAL_STACK_FAILURE = 12 } |
Error codes for the BLE API. More... | |
enum | HVXType_t { BLE_HVX_NOTIFICATION = 0x01, BLE_HVX_INDICATION = 0x02 } |
Handle Value Notification/Indication event. More... | |
Functions | |
template<typename T , typename ContextType > | |
FunctionPointerWithContext < ContextType > | makeFunctionPointer (T *object, void(T::*member)(ContextType context)) |
Factory of adapted member function pointers. | |
template<typename T , typename U > | |
void | operator== (const SafeBool< T > &lhs, const SafeBool< U > &rhs) |
Avoid conversion to bool between different classes. | |
template<typename T , typename U > | |
void | operator!= (const SafeBool< T > &lhs, const SafeBool< U > &rhs) |
Avoid conversion to bool between different classes. | |
static uint8_t | char2int (char c) |
Convert a character containing an hexadecimal digit into an unsigned integer. | |
Variables | |
static const unsigned | BLE_GATT_MTU_SIZE_DEFAULT = 23 |
Default MTU size. |
Enumeration Type Documentation
anonymous enum |
Assigned values for BLE UUIDs.
- Enumerator:
BLE_UUID_UNKNOWN Reserved UUID.
BLE_UUID_SERVICE_PRIMARY Primary Service.
BLE_UUID_SERVICE_SECONDARY Secondary Service.
BLE_UUID_SERVICE_INCLUDE Included service.
BLE_UUID_CHARACTERISTIC Characteristic.
BLE_UUID_DESCRIPTOR_CHAR_EXT_PROP Characteristic Extended Properties Descriptor.
BLE_UUID_DESCRIPTOR_CHAR_USER_DESC Characteristic User Description Descriptor.
BLE_UUID_DESCRIPTOR_CLIENT_CHAR_CONFIG Client Characteristic Configuration Descriptor.
BLE_UUID_DESCRIPTOR_SERVER_CHAR_CONFIG Server Characteristic Configuration Descriptor.
BLE_UUID_DESCRIPTOR_CHAR_PRESENTATION_FORMAT Characteristic Presentation Format Descriptor.
BLE_UUID_DESCRIPTOR_CHAR_AGGREGATE_FORMAT Characteristic Aggregate Format Descriptor.
BLE_UUID_GATT Generic Attribute Profile.
BLE_UUID_GATT_CHARACTERISTIC_SERVICE_CHANGED Service Changed Characteristic.
BLE_UUID_GAP Generic Access Profile.
BLE_UUID_GAP_CHARACTERISTIC_DEVICE_NAME Device Name Characteristic.
BLE_UUID_GAP_CHARACTERISTIC_APPEARANCE Appearance Characteristic.
BLE_UUID_GAP_CHARACTERISTIC_PPF Peripheral Privacy Flag Characteristic.
BLE_UUID_GAP_CHARACTERISTIC_RECONN_ADDR Reconnection Address Characteristic.
BLE_UUID_GAP_CHARACTERISTIC_PPCP Peripheral Preferred Connection Parameters Characteristic.
Definition at line 34 of file blecommon.h.
enum ble_error_t |
Error codes for the BLE API.
The value 0 means that no error was reported; therefore, it allows an API user to cleanly test for errors.
ble_error_t error = some_ble_api_function(); if (error) { // handle the error }
- Enumerator:
BLE_ERROR_NONE No error.
BLE_ERROR_BUFFER_OVERFLOW The requested action would cause a buffer overflow and has been aborted.
BLE_ERROR_NOT_IMPLEMENTED Requested a feature that isn't yet implemented or isn't supported by the target HW.
BLE_ERROR_PARAM_OUT_OF_RANGE One of the supplied parameters is outside the valid range.
BLE_ERROR_INVALID_PARAM One of the supplied parameters is invalid.
BLE_STACK_BUSY The stack is busy.
BLE_ERROR_INVALID_STATE Invalid state.
BLE_ERROR_NO_MEM Out of memory.
BLE_ERROR_OPERATION_NOT_PERMITTED The operation requested is not permitted.
BLE_ERROR_INITIALIZATION_INCOMPLETE The BLE subsystem has not completed its initialization.
BLE_ERROR_ALREADY_INITIALIZED The BLE system has already been initialized.
BLE_ERROR_UNSPECIFIED Unknown error.
BLE_ERROR_INTERNAL_STACK_FAILURE The platform-specific stack failed.
Definition at line 147 of file blecommon.h.
enum HVXType_t |
Handle Value Notification/Indication event.
Emmitted when a notification or indication has been received from a GATT server.
- Enumerator:
BLE_HVX_NOTIFICATION Handle Value Notification.
BLE_HVX_INDICATION Handle Value Indication.
Definition at line 226 of file blecommon.h.
Function Documentation
static uint8_t char2int | ( | char | c ) | [static] |
FunctionPointerWithContext<ContextType> makeFunctionPointer | ( | T * | object, |
void(T::*)(ContextType context) | member | ||
) |
Factory of adapted member function pointers.
This factory eliminates the need to invoke the qualified constructor of FunctionPointerWithContext by using automatic type deduction of function templates.
struct ReadHandler { void on_data_read(const GattReadCallbackParams*); }; ReadHandler read_handler; GattClient& client; client.onDataRead( makeFunctionPointer(&read_handler, &ReadHandler::on_data_read) ); // instead of client.onDataRead( FunctionPointerWithContext<const GattReadCallbackParams*> ( &read_handler, &ReadHandler::on_data_read ) );
- Parameters:
-
[in] object Instance to bound with member
.member The member being adapted.
- Returns:
- Adaptation of the parameters in a FunctionPointerWithContext instance.
Definition at line 350 of file FunctionPointerWithContext.h.
Avoid conversion to bool between different classes.
Will generate a compile time error if instantiated.
Definition at line 142 of file SafeBool.h.
Avoid conversion to bool between different classes.
Will generate a compile time error if instantiated.
Definition at line 130 of file SafeBool.h.
Variable Documentation
const unsigned BLE_GATT_MTU_SIZE_DEFAULT = 23 [static] |
Default MTU size.
Definition at line 218 of file blecommon.h.
Generated on Sun Jul 17 2022 08:25:38 by
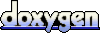