
Rtos API example
PPPCellularInterface Class Reference
PPPCellularInterface class. More...
#include <PPPCellularInterface.h>
Inherits CellularBase.
Inherited by UARTCellularInterface.
Public Member Functions | |
PPPCellularInterface (FileHandle *fh, bool debug=false) | |
Constructor for a generic modem, using a single FileHandle for commands and PPP data. | |
virtual void | set_credentials (const char *apn, const char *uname=0, const char *pwd=0) |
Set the Cellular network credentials. | |
virtual void | set_sim_pin (const char *sim_pin) |
Set the pin code for SIM card. | |
virtual nsapi_error_t | connect (const char *sim_pin, const char *apn=0, const char *uname=0, const char *pwd=0) |
Start the interface. | |
virtual nsapi_error_t | connect () |
Attempt to connect to the Cellular network. | |
virtual nsapi_error_t | disconnect () |
Attempt to disconnect from the network. | |
void | set_sim_pin_check (bool set) |
Adds or removes a SIM facility lock. | |
void | set_new_sim_pin (const char *new_pin) |
Change the pin for the SIM card. | |
virtual bool | is_connected () |
Check if the connection is currently established or not. | |
virtual const char * | get_ip_address () |
Get the local IP address. | |
virtual const char * | get_netmask () |
Get the local network mask. | |
virtual const char * | get_gateway () |
Get the local gateways. | |
void | connection_status_cb (Callback< void(nsapi_error_t)> cb) |
Get notified if the connection gets lost. | |
void | modem_debug_on (bool on) |
Turn modem debug traces on. | |
virtual const char * | get_mac_address () |
Get the local MAC address. | |
virtual nsapi_error_t | set_network (const char *ip_address, const char *netmask, const char *gateway) |
Set a static IP address. | |
virtual nsapi_error_t | set_dhcp (bool dhcp) |
Enable or disable DHCP on the network. | |
virtual nsapi_error_t | gethostbyname (const char *host, SocketAddress *address, nsapi_version_t version=NSAPI_UNSPEC) |
Translates a hostname to an IP address with specific version. | |
virtual nsapi_error_t | add_dns_server (const SocketAddress &address) |
Add a domain name server to list of servers to query. | |
Protected Member Functions | |
virtual void | enable_hup (bool enable) |
Enable or disable hang-up detection. | |
virtual void | modem_init () |
Sets the modem up for powering on. | |
virtual void | modem_deinit () |
Sets the modem in unplugged state. | |
virtual void | modem_power_up () |
Powers up the modem. | |
virtual void | modem_power_down () |
Powers down the modem. | |
virtual NetworkStack * | get_stack () |
Provide access to the underlying stack. | |
bool | nwk_registration (uint8_t nwk_type=PACKET_SWITCHED) |
Starts network registration process. | |
Friends | |
class | Socket |
class | UDPSocket |
class | TCPSocket |
Detailed Description
PPPCellularInterface class.
This interface serves as the controller/driver for the Cellular modems (tested with UBLOX_C027 and MTS_DRAGONFLY_F411RE).
The driver will work with any generic FileHandle, and can be derived from in order to provide forms for specific interfaces, as well as adding extra power and reset controls alongside the FileHandle.
Definition at line 103 of file PPPCellularInterface.h.
Constructor & Destructor Documentation
PPPCellularInterface | ( | FileHandle * | fh, |
bool | debug = false |
||
) |
Constructor for a generic modem, using a single FileHandle for commands and PPP data.
The file handle pointer is not accessed within the constructor, only recorded for later use - this permits a derived class to pass a pointer to a not-yet-constructed member object.
Definition at line 255 of file PPPCellularInterface.cpp.
Member Function Documentation
nsapi_error_t add_dns_server | ( | const SocketAddress & | address ) | [virtual, inherited] |
Add a domain name server to list of servers to query.
- Parameters:
-
address Destination for the host address
- Returns:
- 0 on success, negative error code on failure
Definition at line 63 of file NetworkInterface.cpp.
nsapi_error_t connect | ( | ) | [virtual] |
Attempt to connect to the Cellular network.
Brings up the network interface. Connects to the Cellular Radio network and then brings up the underlying network stack to be used by the cellular modem over PPP interface.
If the SIM requires a PIN, and it is not set/invalid, NSAPI_ERROR_AUTH_ERROR is returned. For APN setup, default behaviour is to use 'internet' as APN string and assuming no authentication is required, i.e., username and password are not set. Optionally, a database lookup can be requested by turning on the APN database lookup feature. In order to do so, add 'MBED_CONF_PPP_CELL_IFACE_APN_LOOKUP' in your mbed_app.json. APN database is by no means exhaustive. It contains a short list of some public APNs with publicly available usernames and passwords (if required) in some particular countries only. Lookup is done using IMSI (International mobile subscriber identifier). Please note that even if 'MBED_CONF_PPP_CELL_IFACE_APN_LOOKUP' config option is set, 'set_credentials()' api still gets the precedence. If the aforementioned API is not used and the config option is set but no match is found in the lookup table then the driver tries to resort to default APN settings.
Preferred method is to setup APN using 'set_credentials()' API.
- Returns:
- 0 on success, negative error code on failure
Implements CellularBase.
Definition at line 556 of file PPPCellularInterface.cpp.
nsapi_error_t connect | ( | const char * | sim_pin, |
const char * | apn = 0 , |
||
const char * | uname = 0 , |
||
const char * | pwd = 0 |
||
) | [virtual] |
Start the interface.
Attempts to connect to a Cellular network. This driver is written mainly for data network connections as CellularInterface is NetworkInterface. That's why connect() call internally calls nwk_registration() method with parameter PACKET_SWITCHED network. Circuit switched hook and registration process is implemented and left in the driver for future extension/subclass support,e.g., an SMS or GPS extension.
- Parameters:
-
sim_pin PIN for the SIM card apn optionally, access point name uname optionally, Username pwd optionally, password
- Returns:
- NSAPI_ERROR_OK on success, or negative error code on failure
Implements CellularBase.
Definition at line 533 of file PPPCellularInterface.cpp.
void connection_status_cb | ( | Callback< void(nsapi_error_t)> | cb ) |
Get notified if the connection gets lost.
- Parameters:
-
cb user defined callback
Definition at line 307 of file PPPCellularInterface.cpp.
nsapi_error_t disconnect | ( | ) | [virtual] |
Attempt to disconnect from the network.
User initiated disconnect.
Brings down the network interface. Shuts down the PPP interface of the underlying network stack. Does not bring down the Radio network
- Returns:
- 0 on success, negative error code on failure
Disconnects from PPP connection only and brings down the underlying network interface
Implements CellularBase.
Definition at line 700 of file PPPCellularInterface.cpp.
void enable_hup | ( | bool | enable ) | [protected, virtual] |
Enable or disable hang-up detection.
When in PPP data pump mode, it is helpful if the FileHandle will signal hang-up via POLLHUP, e.g., if the DCD line is deasserted on a UART. During command mode, this signaling is not desired. enable_hup() controls whether this function should be active.
Meant to be overridden.
Reimplemented in UARTCellularInterface.
Definition at line 277 of file PPPCellularInterface.cpp.
const char * get_gateway | ( | ) | [virtual] |
Get the local gateways.
- Returns:
- Null-terminated representation of the local gateway or null if no network mask has been recieved
Implements CellularBase.
Definition at line 721 of file PPPCellularInterface.cpp.
const char * get_ip_address | ( | ) | [virtual] |
Get the local IP address.
- Returns:
- Null-terminated representation of the local IP address or null if no IP address has been recieved
Implements CellularBase.
Definition at line 711 of file PPPCellularInterface.cpp.
const char * get_mac_address | ( | ) | [virtual, inherited] |
Get the local MAC address.
Provided MAC address is intended for info or debug purposes and may not be provided if the underlying network interface does not provide a MAC address
- Returns:
- Null-terminated representation of the local MAC address or null if no MAC address is available
Definition at line 23 of file NetworkInterface.cpp.
const char * get_netmask | ( | ) | [virtual] |
Get the local network mask.
- Returns:
- Null-terminated representation of the local network mask or null if no network mask has been recieved
Implements CellularBase.
Definition at line 716 of file PPPCellularInterface.cpp.
NetworkStack * get_stack | ( | void | ) | [protected, virtual] |
Provide access to the underlying stack.
Get a pointer to the underlying network stack.
- Returns:
- The underlying network stack
Definition at line 795 of file PPPCellularInterface.cpp.
nsapi_error_t gethostbyname | ( | const char * | host, |
SocketAddress * | address, | ||
nsapi_version_t | version = NSAPI_UNSPEC |
||
) | [virtual, inherited] |
Translates a hostname to an IP address with specific version.
The hostname may be either a domain name or an IP address. If the hostname is an IP address, no network transactions will be performed.
If no stack-specific DNS resolution is provided, the hostname will be resolve using a UDP socket on the stack.
- Parameters:
-
address Destination for the host SocketAddress host Hostname to resolve version IP version of address to resolve, NSAPI_UNSPEC indicates version is chosen by the stack (defaults to NSAPI_UNSPEC)
- Returns:
- 0 on success, negative error code on failure
Definition at line 58 of file NetworkInterface.cpp.
bool is_connected | ( | void | ) | [virtual] |
Check if the connection is currently established or not.
- Returns:
- true/false If the cellular module have successfully acquired a carrier and is connected to an external packet data network using PPP, isConnected() API returns true and false otherwise.
Implements CellularBase.
Definition at line 399 of file PPPCellularInterface.cpp.
void modem_debug_on | ( | bool | on ) |
Turn modem debug traces on.
- Parameters:
-
on set true to enable debug traces
Definition at line 302 of file PPPCellularInterface.cpp.
void modem_deinit | ( | ) | [protected, virtual] |
Sets the modem in unplugged state.
modem_deinit() will be equivalent to pulling the plug off of the device, i.e., detaching power and serial port. This puts the modem in lowest power state. It is meant to be overridden. If the modem is on-board, an implementation of onboard_modem_api.h will override this. If the the modem is a plugged-in device (i.e., a shield type component), the driver deriving from this class will override.
Reimplemented in OnboardCellularInterface.
Definition at line 287 of file PPPCellularInterface.cpp.
void modem_init | ( | ) | [protected, virtual] |
Sets the modem up for powering on.
modem_init() is equivalent to plugging in the device, e.g., attaching power and serial port. It is meant to be overridden. If the modem is on-board, an implementation of onboard_modem_api.h will override this. If the the modem is a plugged-in device (i.e., a shield type component), the driver deriving from this class will override.
Reimplemented in OnboardCellularInterface.
Definition at line 282 of file PPPCellularInterface.cpp.
void modem_power_down | ( | ) | [protected, virtual] |
Powers down the modem.
modem_power_down() is equivalent to turning off the modem by button press. It is meant to be overridden. If the modem is on-board, an implementation of onboard_modem_api.h will override this. If the the modem is a plugged-in device (i.e., a shield type component), the driver deriving from this class will override.
Reimplemented in OnboardCellularInterface.
Definition at line 297 of file PPPCellularInterface.cpp.
void modem_power_up | ( | ) | [protected, virtual] |
Powers up the modem.
modem_power_up() is equivalent to pressing the soft power button. The driver may repeat this if the modem is not responsive to AT commands. It is meant to be overridden. If the modem is on-board, an implementation of onboard_modem_api.h will override this. If the the modem is a plugged-in device (i.e., a shield type component), the driver deriving from this class will override.
Reimplemented in OnboardCellularInterface.
Definition at line 292 of file PPPCellularInterface.cpp.
bool nwk_registration | ( | uint8_t | nwk_type = PACKET_SWITCHED ) |
[protected] |
Starts network registration process.
Potential users could be subclasses who are not network interface but would like to use CellularInterface infrastructure to register with a cellular network, e.g., an SMS extension to CellularInterface.
- Parameters:
-
nwk_type type of network to connect, defaults to packet switched network
- Returns:
- true if registration is successful
Definition at line 330 of file PPPCellularInterface.cpp.
void set_credentials | ( | const char * | apn, |
const char * | uname = 0 , |
||
const char * | pwd = 0 |
||
) | [virtual] |
Set the Cellular network credentials.
Please check documentation of connect() for default behaviour of APN settings.
- Parameters:
-
apn Access point name uname optionally, Username pwd optionally, password
Implements CellularBase.
Definition at line 496 of file PPPCellularInterface.cpp.
nsapi_error_t set_dhcp | ( | bool | dhcp ) | [virtual, inherited] |
Enable or disable DHCP on the network.
Enables DHCP on connecting the network. Defaults to enabled unless a static IP address has been assigned. Requires that the network is disconnected.
- Parameters:
-
dhcp True to enable DHCP
- Returns:
- 0 on success, negative error code on failure
Definition at line 48 of file NetworkInterface.cpp.
nsapi_error_t set_network | ( | const char * | ip_address, |
const char * | netmask, | ||
const char * | gateway | ||
) | [virtual, inherited] |
Set a static IP address.
Configures this network interface to use a static IP address. Implicitly disables DHCP, which can be enabled in set_dhcp. Requires that the network is disconnected.
- Parameters:
-
ip_address Null-terminated representation of the local IP address netmask Null-terminated representation of the local network mask gateway Null-terminated representation of the local gateway
- Returns:
- 0 on success, negative error code on failure
Definition at line 43 of file NetworkInterface.cpp.
void set_new_sim_pin | ( | const char * | new_pin ) |
Change the pin for the SIM card.
Public API.
Provide the new pin for your SIM card with this API. Old pin code will be assumed to be set using set_SIM_pin() API. This API have no immediate effect. While establishing connection, driver will change the SIM pin for the next boot.
- Parameters:
-
new_pin new pin to be used in string format
Sets up the flag for the driver to change pin code for SIM card
Definition at line 324 of file PPPCellularInterface.cpp.
void set_sim_pin | ( | const char * | sim_pin ) | [virtual] |
Set the pin code for SIM card.
- Parameters:
-
sim_pin PIN for the SIM card
Implements CellularBase.
Definition at line 442 of file PPPCellularInterface.cpp.
void set_sim_pin_check | ( | bool | check ) |
Adds or removes a SIM facility lock.
Public API.
Can be used to enable or disable SIM pin check at device startup. This API sets up flags for the driver which would either enable or disable SIM pin checking depending upon the user provided argument while establishing connection. It doesn't do anything immediately other than setting up flags.
- Parameters:
-
set can be set to true if the SIM pin check is supposed to be enabled and vice versa.
Sets up the flag for the driver to enable or disable SIM pin check at the next boot.
Definition at line 316 of file PPPCellularInterface.cpp.
Generated on Sun Jul 17 2022 08:25:42 by
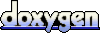