
Rtos API example
CellularBase Class Reference
CellularBase class. More...
#include <CellularBase.h>
Inherits NetworkInterface.
Inherited by PPPCellularInterface.
Public Member Functions | |
virtual void | set_credentials (const char *apn, const char *uname=0, const char *pwd=0)=0 |
Set the Cellular network credentials. | |
virtual void | set_sim_pin (const char *sim_pin)=0 |
Set the pin code for SIM card. | |
virtual nsapi_error_t | connect (const char *sim_pin, const char *apn=0, const char *uname=0, const char *pwd=0)=0 |
Start the interface. | |
virtual nsapi_error_t | connect ()=0 |
Start the interface. | |
virtual nsapi_error_t | disconnect ()=0 |
Stop the interface. | |
virtual bool | is_connected ()=0 |
Check if the connection is currently established or not. | |
virtual const char * | get_ip_address ()=0 |
Get the local IP address. | |
virtual const char * | get_netmask ()=0 |
Get the local network mask. | |
virtual const char * | get_gateway ()=0 |
Get the local gateways. | |
virtual const char * | get_mac_address () |
Get the local MAC address. | |
virtual nsapi_error_t | set_network (const char *ip_address, const char *netmask, const char *gateway) |
Set a static IP address. | |
virtual nsapi_error_t | set_dhcp (bool dhcp) |
Enable or disable DHCP on the network. | |
virtual nsapi_error_t | gethostbyname (const char *host, SocketAddress *address, nsapi_version_t version=NSAPI_UNSPEC) |
Translates a hostname to an IP address with specific version. | |
virtual nsapi_error_t | add_dns_server (const SocketAddress &address) |
Add a domain name server to list of servers to query. | |
Protected Member Functions | |
virtual NetworkStack * | get_stack ()=0 |
Provide access to the NetworkStack object. | |
Friends | |
class | Socket |
class | UDPSocket |
class | TCPSocket |
Detailed Description
CellularBase class.
Common interface that is shared between Cellular interfaces
Definition at line 25 of file CellularBase.h.
Member Function Documentation
nsapi_error_t add_dns_server | ( | const SocketAddress & | address ) | [virtual, inherited] |
Add a domain name server to list of servers to query.
- Parameters:
-
address Destination for the host address
- Returns:
- 0 on success, negative error code on failure
Definition at line 63 of file NetworkInterface.cpp.
virtual nsapi_error_t connect | ( | ) | [pure virtual] |
Start the interface.
Attempts to connect to a Cellular network. If the SIM requires a PIN, and it is not set/invalid, NSAPI_ERROR_AUTH_ERROR is returned.
- Returns:
- NSAPI_ERROR_OK on success, or negative error code on failure
Implemented in PPPCellularInterface.
virtual nsapi_error_t connect | ( | const char * | sim_pin, |
const char * | apn = 0 , |
||
const char * | uname = 0 , |
||
const char * | pwd = 0 |
||
) | [pure virtual] |
Start the interface.
Attempts to connect to a Cellular network.
- Parameters:
-
sim_pin PIN for the SIM card apn optionally, access point name uname optionally, Username pwd optionally, password
- Returns:
- NSAPI_ERROR_OK on success, or negative error code on failure
Implemented in PPPCellularInterface.
virtual nsapi_error_t disconnect | ( | ) | [pure virtual] |
Stop the interface.
- Returns:
- 0 on success, or error code on failure
Implemented in PPPCellularInterface.
virtual const char* get_gateway | ( | ) | [pure virtual] |
Get the local gateways.
- Returns:
- Null-terminated representation of the local gateway or null if no network mask has been received
Implemented in PPPCellularInterface.
virtual const char* get_ip_address | ( | ) | [pure virtual] |
Get the local IP address.
- Returns:
- Null-terminated representation of the local IP address or null if no IP address has been received
Implemented in PPPCellularInterface.
const char * get_mac_address | ( | ) | [virtual, inherited] |
Get the local MAC address.
Provided MAC address is intended for info or debug purposes and may not be provided if the underlying network interface does not provide a MAC address
- Returns:
- Null-terminated representation of the local MAC address or null if no MAC address is available
Definition at line 23 of file NetworkInterface.cpp.
virtual const char* get_netmask | ( | ) | [pure virtual] |
Get the local network mask.
- Returns:
- Null-terminated representation of the local network mask or null if no network mask has been received
Implemented in PPPCellularInterface.
virtual NetworkStack* get_stack | ( | ) | [protected, pure virtual, inherited] |
Provide access to the NetworkStack object.
- Returns:
- The underlying NetworkStack object
nsapi_error_t gethostbyname | ( | const char * | host, |
SocketAddress * | address, | ||
nsapi_version_t | version = NSAPI_UNSPEC |
||
) | [virtual, inherited] |
Translates a hostname to an IP address with specific version.
The hostname may be either a domain name or an IP address. If the hostname is an IP address, no network transactions will be performed.
If no stack-specific DNS resolution is provided, the hostname will be resolve using a UDP socket on the stack.
- Parameters:
-
address Destination for the host SocketAddress host Hostname to resolve version IP version of address to resolve, NSAPI_UNSPEC indicates version is chosen by the stack (defaults to NSAPI_UNSPEC)
- Returns:
- 0 on success, negative error code on failure
Definition at line 58 of file NetworkInterface.cpp.
virtual bool is_connected | ( | ) | [pure virtual] |
Check if the connection is currently established or not.
- Returns:
- true/false If the cellular module have successfully acquired a carrier and is connected to an external packet data network using PPP, isConnected() API returns true and false otherwise.
Implemented in PPPCellularInterface.
virtual void set_credentials | ( | const char * | apn, |
const char * | uname = 0 , |
||
const char * | pwd = 0 |
||
) | [pure virtual] |
Set the Cellular network credentials.
Please check documentation of connect() for default behaviour of APN settings.
- Parameters:
-
apn Access point name uname optionally, Username pwd optionally, password
Implemented in PPPCellularInterface.
nsapi_error_t set_dhcp | ( | bool | dhcp ) | [virtual, inherited] |
Enable or disable DHCP on the network.
Enables DHCP on connecting the network. Defaults to enabled unless a static IP address has been assigned. Requires that the network is disconnected.
- Parameters:
-
dhcp True to enable DHCP
- Returns:
- 0 on success, negative error code on failure
Definition at line 48 of file NetworkInterface.cpp.
nsapi_error_t set_network | ( | const char * | ip_address, |
const char * | netmask, | ||
const char * | gateway | ||
) | [virtual, inherited] |
Set a static IP address.
Configures this network interface to use a static IP address. Implicitly disables DHCP, which can be enabled in set_dhcp. Requires that the network is disconnected.
- Parameters:
-
ip_address Null-terminated representation of the local IP address netmask Null-terminated representation of the local network mask gateway Null-terminated representation of the local gateway
- Returns:
- 0 on success, negative error code on failure
Definition at line 43 of file NetworkInterface.cpp.
virtual void set_sim_pin | ( | const char * | sim_pin ) | [pure virtual] |
Set the pin code for SIM card.
- Parameters:
-
sim_pin PIN for the SIM card
Implemented in PPPCellularInterface.
Generated on Sun Jul 17 2022 08:25:39 by
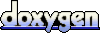