
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
PPPCellularInterface.h
00001 /* Copyright (c) 2017 ARM Limited 00002 * 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS, 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 */ 00015 00016 #ifndef PPP_CELLULAR_INTERFACE_ 00017 #define PPP_CELLULAR_INTERFACE_ 00018 00019 #include "CellularBase.h" 00020 #include "platform/ATCmdParser.h" 00021 #include "mbed.h" 00022 00023 #if NSAPI_PPP_AVAILABLE 00024 00025 // Forward declaration 00026 class NetworkStack; 00027 00028 /** 00029 * Network registration status 00030 * UBX-13001820 - AT Commands Example Application Note (Section 4.1.4.5) 00031 */ 00032 typedef enum { 00033 GSM=0, 00034 COMPACT_GSM=1, 00035 UTRAN=2, 00036 EDGE=3, 00037 HSDPA=4, 00038 HSUPA=5, 00039 HSDPA_HSUPA=6, 00040 LTE=7 00041 } radio_access_nwk_type; 00042 00043 /** 00044 * Used in registration process to tell which type of network 00045 * to connect. 00046 */ 00047 typedef enum { 00048 CIRCUIT_SWITCHED=0, 00049 PACKET_SWITCHED 00050 } nwk_type; 00051 00052 /** 00053 * Circuit Switched network registration status (CREG Usage) 00054 * UBX-13001820 - AT Commands Example Application Note (Section 7.10.3) 00055 */ 00056 typedef enum { 00057 CSD_NOT_REGISTERED_NOT_SEARCHING=0, 00058 CSD_REGISTERED=1, 00059 CSD_NOT_REGISTERED_SEARCHING=2, 00060 CSD_REGISTRATION_DENIED=3, 00061 CSD_UNKNOWN_COVERAGE=4, 00062 CSD_REGISTERED_ROAMING=5, 00063 CSD_SMS_ONLY=6, 00064 CSD_SMS_ONLY_ROAMING=7, 00065 CSD_CSFB_NOT_PREFERRED=9 00066 } nwk_registration_status_csd; 00067 00068 /** 00069 * Packet Switched network registration status (CGREG Usage) 00070 * UBX-13001820 - AT Commands Example Application Note (Section 18.27.3) 00071 */ 00072 typedef enum { 00073 PSD_NOT_REGISTERED_NOT_SEARCHING=0, 00074 PSD_REGISTERED=1, 00075 PSD_NOT_REGISTERED_SEARCHING=2, 00076 PSD_REGISTRATION_DENIED=3, 00077 PSD_UNKNOWN_COVERAGE=4, 00078 PSD_REGISTERED_ROAMING=5, 00079 PSD_EMERGENCY_SERVICES_ONLY=8 00080 } nwk_registration_status_psd; 00081 00082 typedef struct { 00083 char ccid[20+1]; //!< Integrated Circuit Card ID 00084 char imsi[15+1]; //!< International Mobile Station Identity 00085 char imei[15+1]; //!< International Mobile Equipment Identity 00086 char meid[18+1]; //!< Mobile Equipment IDentifier 00087 int flags; 00088 bool ppp_connection_up; 00089 radio_access_nwk_type rat; 00090 nwk_registration_status_csd reg_status_csd; 00091 nwk_registration_status_psd reg_status_psd; 00092 } device_info; 00093 00094 /** PPPCellularInterface class 00095 * 00096 * This interface serves as the controller/driver for the Cellular 00097 * modems (tested with UBLOX_C027 and MTS_DRAGONFLY_F411RE). 00098 * 00099 * The driver will work with any generic FileHandle, and can be 00100 * derived from in order to provide forms for specific interfaces, as well as 00101 * adding extra power and reset controls alongside the FileHandle. 00102 */ 00103 class PPPCellularInterface : public CellularBase { 00104 00105 public: 00106 00107 /** Constructor for a generic modem, using a single FileHandle for commands and PPP data. 00108 * 00109 * The file handle pointer is not accessed within the constructor, only recorded for later 00110 * use - this permits a derived class to pass a pointer to a not-yet-constructed member object. 00111 */ 00112 PPPCellularInterface(FileHandle *fh, bool debug = false); 00113 00114 virtual ~PPPCellularInterface(); 00115 00116 /** Set the Cellular network credentials 00117 * 00118 * Please check documentation of connect() for default behaviour of APN settings. 00119 * 00120 * @param apn Access point name 00121 * @param uname optionally, Username 00122 * @param pwd optionally, password 00123 */ 00124 virtual void set_credentials(const char *apn, const char *uname = 0, 00125 const char *pwd = 0); 00126 00127 /** Set the pin code for SIM card 00128 * 00129 * @param sim_pin PIN for the SIM card 00130 */ 00131 virtual void set_sim_pin(const char *sim_pin); 00132 00133 /** Start the interface 00134 * 00135 * Attempts to connect to a Cellular network. 00136 * This driver is written mainly for data network connections as CellularInterface 00137 * is NetworkInterface. That's why connect() call internally calls nwk_registration() 00138 * method with parameter PACKET_SWITCHED network. Circuit switched hook and registration 00139 * process is implemented and left in the driver for future extension/subclass support,e.g., 00140 * an SMS or GPS extension. 00141 * 00142 * @param sim_pin PIN for the SIM card 00143 * @param apn optionally, access point name 00144 * @param uname optionally, Username 00145 * @param pwd optionally, password 00146 * @return NSAPI_ERROR_OK on success, or negative error code on failure 00147 */ 00148 virtual nsapi_error_t connect(const char *sim_pin, const char *apn = 0, 00149 const char *uname = 0, const char *pwd = 0); 00150 00151 /** Attempt to connect to the Cellular network 00152 * 00153 * Brings up the network interface. Connects to the Cellular Radio 00154 * network and then brings up the underlying network stack to be used 00155 * by the cellular modem over PPP interface. 00156 * 00157 * If the SIM requires a PIN, and it is not set/invalid, NSAPI_ERROR_AUTH_ERROR is returned. 00158 * For APN setup, default behaviour is to use 'internet' as APN string and assuming no authentication 00159 * is required, i.e., username and password are not set. Optionally, a database lookup can be requested 00160 * by turning on the APN database lookup feature. In order to do so, add 'MBED_CONF_PPP_CELL_IFACE_APN_LOOKUP' 00161 * in your mbed_app.json. APN database is by no means exhaustive. It contains a short list of some public 00162 * APNs with publicly available usernames and passwords (if required) in some particular countries only. 00163 * Lookup is done using IMSI (International mobile subscriber identifier). 00164 * Please note that even if 'MBED_CONF_PPP_CELL_IFACE_APN_LOOKUP' config option is set, 'set_credentials()' api still 00165 * gets the precedence. If the aforementioned API is not used and the config option is set but no match is found in 00166 * the lookup table then the driver tries to resort to default APN settings. 00167 * 00168 * Preferred method is to setup APN using 'set_credentials()' API. 00169 00170 * @return 0 on success, negative error code on failure 00171 */ 00172 virtual nsapi_error_t connect(); 00173 00174 /** Attempt to disconnect from the network 00175 * 00176 * Brings down the network interface. Shuts down the PPP interface 00177 * of the underlying network stack. Does not bring down the Radio network 00178 * 00179 * @return 0 on success, negative error code on failure 00180 */ 00181 virtual nsapi_error_t disconnect(); 00182 00183 /** Adds or removes a SIM facility lock 00184 * 00185 * Can be used to enable or disable SIM pin check at device startup. 00186 * This API sets up flags for the driver which would either enable or disable 00187 * SIM pin checking depending upon the user provided argument while establishing 00188 * connection. It doesn't do anything immediately other than setting up flags. 00189 * 00190 * @param set can be set to true if the SIM pin check is supposed to be enabled 00191 * and vice versa. 00192 */ 00193 void set_sim_pin_check(bool set); 00194 00195 /** Change the pin for the SIM card 00196 * 00197 * Provide the new pin for your SIM card with this API. Old pin code will be assumed to 00198 * be set using set_SIM_pin() API. This API have no immediate effect. While establishing 00199 * connection, driver will change the SIM pin for the next boot. 00200 * 00201 * @param new_pin new pin to be used in string format 00202 */ 00203 void set_new_sim_pin(const char *new_pin); 00204 00205 /** Check if the connection is currently established or not 00206 * 00207 * @return true/false If the cellular module have successfully acquired a carrier and is 00208 * connected to an external packet data network using PPP, isConnected() 00209 * API returns true and false otherwise. 00210 */ 00211 virtual bool is_connected(); 00212 00213 /** Get the local IP address 00214 * 00215 * @return Null-terminated representation of the local IP address 00216 * or null if no IP address has been recieved 00217 */ 00218 virtual const char *get_ip_address(); 00219 00220 /** Get the local network mask 00221 * 00222 * @return Null-terminated representation of the local network mask 00223 * or null if no network mask has been recieved 00224 */ 00225 virtual const char *get_netmask(); 00226 00227 /** Get the local gateways 00228 * 00229 * @return Null-terminated representation of the local gateway 00230 * or null if no network mask has been recieved 00231 */ 00232 virtual const char *get_gateway(); 00233 00234 /** Get notified if the connection gets lost 00235 * 00236 * @param cb user defined callback 00237 */ 00238 void connection_status_cb(Callback<void(nsapi_error_t)> cb); 00239 00240 /** Turn modem debug traces on 00241 * 00242 * @param on set true to enable debug traces 00243 */ 00244 void modem_debug_on(bool on); 00245 00246 private: 00247 FileHandle *_fh; 00248 ATCmdParser *_at; 00249 const char *_new_pin; 00250 const char *_pin; 00251 const char *_apn; 00252 const char *_uname; 00253 const char *_pwd; 00254 bool _debug_trace_on; 00255 nsapi_ip_stack_t _stack; 00256 Callback<void(nsapi_error_t)> _connection_status_cb; 00257 void base_initialization(); 00258 void setup_at_parser(); 00259 void shutdown_at_parser(); 00260 nsapi_error_t initialize_sim_card(); 00261 nsapi_error_t setup_context_and_credentials(); 00262 bool power_up(); 00263 void power_down(); 00264 00265 protected: 00266 /** Enable or disable hang-up detection 00267 * 00268 * When in PPP data pump mode, it is helpful if the FileHandle will signal hang-up via 00269 * POLLHUP, e.g., if the DCD line is deasserted on a UART. During command mode, this 00270 * signaling is not desired. enable_hup() controls whether this function should be 00271 * active. 00272 * 00273 * Meant to be overridden. 00274 */ 00275 virtual void enable_hup(bool enable); 00276 00277 /** Sets the modem up for powering on 00278 * 00279 * modem_init() is equivalent to plugging in the device, e.g., attaching power and serial port. 00280 * It is meant to be overridden. 00281 * If the modem is on-board, an implementation of onboard_modem_api.h 00282 * will override this. 00283 * If the the modem is a plugged-in device (i.e., a shield type component), the driver deriving from this 00284 * class will override. 00285 */ 00286 virtual void modem_init(); 00287 00288 /** Sets the modem in unplugged state 00289 * 00290 * modem_deinit() will be equivalent to pulling the plug off of the device, i.e., detaching power 00291 * and serial port. This puts the modem in lowest power state. 00292 * It is meant to be overridden. 00293 * If the modem is on-board, an implementation of onboard_modem_api.h 00294 * will override this. 00295 * If the the modem is a plugged-in device (i.e., a shield type component), the driver deriving from this 00296 * class will override. 00297 */ 00298 virtual void modem_deinit(); 00299 00300 /** Powers up the modem 00301 * 00302 * modem_power_up() is equivalent to pressing the soft power button. 00303 * The driver may repeat this if the modem is not responsive to AT commands. 00304 * It is meant to be overridden. 00305 * If the modem is on-board, an implementation of onboard_modem_api.h 00306 * will override this. 00307 * If the the modem is a plugged-in device (i.e., a shield type component), the driver deriving from this 00308 * class will override. 00309 */ 00310 virtual void modem_power_up(); 00311 00312 /** Powers down the modem 00313 * 00314 * modem_power_down() is equivalent to turning off the modem by button press. 00315 * It is meant to be overridden. 00316 * If the modem is on-board, an implementation of onboard_modem_api.h 00317 * will override this. 00318 * If the the modem is a plugged-in device (i.e., a shield type component), the driver deriving from this 00319 * class will override. 00320 */ 00321 virtual void modem_power_down(); 00322 00323 /** Provide access to the underlying stack 00324 * 00325 * @return The underlying network stack 00326 */ 00327 virtual NetworkStack *get_stack(); 00328 00329 /** Starts network registration process. 00330 * 00331 * Potential users could be subclasses who are not network interface 00332 * but would like to use CellularInterface infrastructure to register 00333 * with a cellular network, e.g., an SMS extension to CellularInterface. 00334 * 00335 * @param nwk_type type of network to connect, defaults to packet switched network 00336 * 00337 * @return true if registration is successful 00338 */ 00339 bool nwk_registration(uint8_t nwk_type=PACKET_SWITCHED); 00340 00341 }; 00342 00343 #endif //NSAPI_PPP_AVAILABLE 00344 00345 #endif //PPP_CELLULAR_INTERFACE_
Generated on Sun Jul 17 2022 08:25:29 by
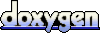